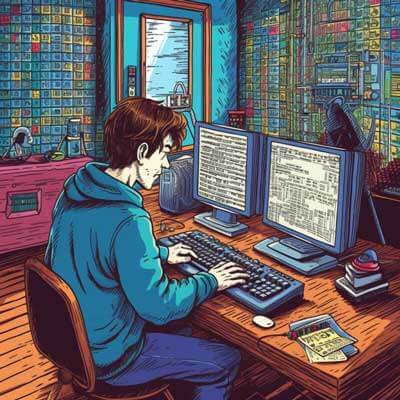
Table of Contents
Bash, the default shell interpreter on Linux systems, provides a straightforward way to access the seconds since the epoch (January 1, 1970, 00:00:00 UTC). This value, often referred to as the "Unix timestamp," is a widely used representation of time in Unix-like operating systems.
In this article, we will explore various methods to access the seconds since the epoch in Bash scripts on Linux. We will cover topics such as converting epoch time to a readable date format, getting the current Unix timestamp, calculating time differences between two epoch timestamps, converting specific date and time to epoch time, and more.
How can I convert epoch time to a readable date format in bash?
Converting epoch time to a human-readable date format is a common requirement when working with timestamps in Bash scripts. The date
command, available in most Linux distributions, provides a simple way to achieve this.
To convert an epoch timestamp to a readable date format, you can use the -d
option followed by the timestamp value. Here's an example:
$ date -d @1628600000
This command will output the corresponding date and time for the given epoch timestamp. The @
symbol is used to indicate that the provided value is an epoch timestamp.
Additionally, you can specify a specific date format using the +
option followed by the desired format. For example, to display the date in the format "YYYY-MM-DD HH:MM:SS", you can use the following command:
$ date -d @1628600000 +"%Y-%m-%d %H:%M:%S"
This will output the date in the specified format: "2021-08-10 12:00:00".
Related Article: Tutorial on Traceroute in Linux
What is the command to get the current Unix timestamp in a bash script?
To get the current Unix timestamp in a Bash script, you can use the date
command with the +%s
option. The %s
format specifier represents the number of seconds since the epoch.
Here's an example:
$ date +%s
This command will output the current Unix timestamp.
You can also store the current timestamp in a variable within a Bash script for later use. Here's an example:
timestamp=$(date +%s) echo "Current timestamp: $timestamp"
This will store the current Unix timestamp in the timestamp
variable and then print it.
How do I calculate the time difference in seconds between two epoch timestamps in a bash script?
Calculating the time difference in seconds between two epoch timestamps can be useful in various scenarios. Bash provides several ways to perform this calculation.
One approach is to subtract the two timestamps and then use the bc
command-line calculator to perform the arithmetic operation. Here's an example:
timestamp1=1628600000 timestamp2=1628600500 difference=$(echo "$timestamp2 - $timestamp1" | bc) echo "Time difference in seconds: $difference"
This will output the time difference in seconds between the two timestamps.
Another approach is to use the $(( ))
arithmetic expansion in Bash. Here's an example:
timestamp1=1628600000 timestamp2=1628600500 difference=$((timestamp2 - timestamp1)) echo "Time difference in seconds: $difference"
Both approaches will yield the same result.
Is there a way to convert a specific date and time to epoch time in a bash script?
Yes, you can convert a specific date and time to epoch time in a Bash script using the date
command. The date
command allows you to specify a custom date and time using the --date
or -d
option.
Here's an example:
date_string="2021-08-10 12:00:00" epoch_time=$(date -d "$date_string" +%s) echo "Epoch time for $date_string: $epoch_time"
This script will convert the specified date and time to epoch time and print the result.
It's important to note that the date and time format must be valid and conform to the expected format. In the example above, the date format is "YYYY-MM-DD HH:MM:SS".
Related Article: How to Fix Openssl Error: Self Signed Certificate in Certificate Chain on Linux
What is the syntax for using the date command to display seconds since epoch in a bash script?
To display the seconds since the epoch using the date
command in a Bash script, you can use the +%s
option. The %s
format specifier represents the number of seconds since the epoch.
Here's an example:
epoch_time=$(date +%s) echo "Seconds since the epoch: $epoch_time"
This script will store the current epoch time in the epoch_time
variable and then print it.
How can I add or subtract a certain number of seconds from the current epoch time in a bash script?
To add or subtract a certain number of seconds from the current epoch time in a Bash script, you can use the date
command along with the --date
or -d
option.
To add seconds, you can specify the desired number of seconds using the +
operator. For example, to add 60 seconds to the current epoch time, you can use the following command:
new_epoch_time=$(date -d "+60 seconds" +%s)
To subtract seconds, you can use the -
operator. For example, to subtract 60 seconds from the current epoch time, you can use the following command:
new_epoch_time=$(date -d "-60 seconds" +%s)
These commands will calculate the new epoch time based on the addition or subtraction of the specified number of seconds.
What are some common use cases for accessing seconds since epoch in bash scripts?
Accessing seconds since the epoch in Bash scripts can be useful in various scenarios. Some common use cases include:
- Timestamping logs or events: Epoch timestamps provide a standardized way to record timestamps for events or log entries, allowing for easy sorting and analysis.
- Calculating time differences: By accessing epoch timestamps, you can calculate the time difference between two events or measure the duration of specific operations.
- Scheduling tasks: Epoch timestamps enable precise scheduling of tasks based on specific time criteria, such as running a script at a certain epoch time.
- Working with APIs: Many APIs require epoch timestamps for time-related parameters, such as retrieving data within a specific time range.
These are just a few examples of how accessing seconds since the epoch can be beneficial in Bash scripts. The flexibility and ease of use of epoch timestamps make them a valuable tool in various scripting scenarios.
Are there any built-in functions or variables in bash for working with epoch time?
Bash does not have any built-in functions or variables specifically designed for working with epoch time. However, the date
command, as mentioned earlier, provides extensive capabilities for manipulating and formatting epoch timestamps.
In addition to the date
command, Bash also offers a range of arithmetic and string manipulation operators that can be used to perform calculations and conversions on epoch timestamps stored in variables.
For example, you can use the arithmetic expansion $(( ))
to perform mathematical operations on epoch timestamps. Here's an example:
timestamp1=1628600000 timestamp2=1628600500 difference=$((timestamp2 - timestamp1))
This script calculates the time difference in seconds between two epoch timestamps using the arithmetic expansion.
While Bash itself does not have built-in functions or variables dedicated to epoch time, its extensive set of tools and operators allows for flexible and useful manipulation of epoch timestamps within scripts.
Related Article: Appending Dates to Filenames in Bash Scripts
How can I convert a given date and time to epoch time using a bash script?
To convert a given date and time to epoch time using a Bash script, you can use the date
command along with the --date
or -d
option.
Here's an example script that converts a specific date and time to epoch time:
date_string="2021-08-10 12:00:00" epoch_time=$(date -d "$date_string" +%s) echo "Epoch time for $date_string: $epoch_time"
This script takes the date_string
variable, which represents the desired date and time, and converts it to epoch time using the date
command. The resulting epoch time is then printed.
Make sure to provide the date and time in the correct format, such as "YYYY-MM-DD HH:MM:SS", to ensure accurate conversion.
Is there a way to display the current time in seconds since epoch without using the date command in bash?
While the date
command is the most common way to access the current time in seconds since the epoch, there is an alternative method using Bash's built-in printf
function.
The printf
function allows you to format and print data, including the current time in seconds since the epoch. Here's an example:
current_time=$(printf '%(%s)T\n' -1) echo "Current time in seconds since the epoch: $current_time"
In this script, the printf
function is used with the %s
format specifier to print the current time in seconds since the epoch. The -1
argument represents the current time.
This method can be useful in scenarios where the date
command is not available or if you prefer to use Bash's built-in functionality.
Additional Resources
- Converting Unix timestamp to readable date format in bash