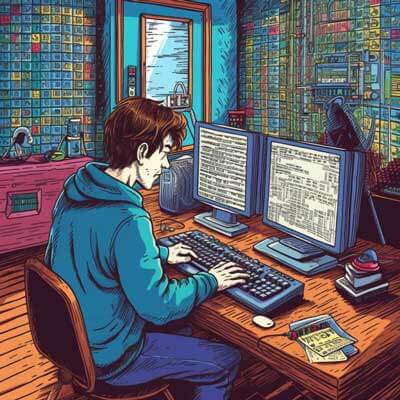
- What is the Maximum Number of Arguments a Bash Script Can Have?
- How to Handle Optional Arguments in a Bash Script?
- What Happens if I Exceed the Maximum Number of Arguments in a Bash Script?
- How to Check if a Bash Script Received a Specific Argument?
- Are There Any Restrictions on the Length of Arguments in a Bash Script?
- Can I Pass Arguments to a Bash Script Using Environment Variables?
- Is it Possible to Pass Arguments to a Bash Script Using a File?
- How to Handle Spaces and Special Characters in Bash Script Arguments?
- What is the Difference Between Positional Parameters and Named Parameters in a Bash Script?
What is the Maximum Number of Arguments a Bash Script Can Have?
The maximum number of arguments a bash script can have depends on various factors, including the system’s resources and limitations. In most cases, the maximum number of arguments is determined by the operating system’s maximum command-line length.
On Linux systems, the maximum command-line length is typically defined by the ARG_MAX
constant, which represents the maximum number of bytes that can be passed as arguments and environment variables to a new process. You can check the value of ARG_MAX
on your system by running the following command:
$ getconf ARG_MAX
The output will be the maximum number of bytes that can be passed as arguments.
However, it is important to note that the actual number of arguments that can be passed to a bash script may be lower than the maximum command-line length. This is because the length of each argument, as well as other factors such as environment variables and command-line options, also contribute to the overall command-line length. Therefore, the maximum number of arguments that can be passed to a bash script may vary depending on the length of the arguments and other factors.
In general, it is recommended to keep the number of arguments to a reasonable limit to ensure the script’s usability and avoid potential issues with exceeding system limitations.
Related Article: How To Echo a Newline In Bash
How to Handle Optional Arguments in a Bash Script?
In some cases, you may want to provide optional arguments to a bash script. Optional arguments allow users to customize the behavior of the script without necessarily requiring them to provide values for every argument. This can make the script more flexible and user-friendly.
To handle optional arguments in a bash script, you can make use of conditional logic and default values. One common approach is to check if an argument is provided and use a default value if it is not. This can be done using conditional statements such as if
and else
.
Let’s consider an example where we have a script that accepts two arguments: a filename and an optional delimiter. If the delimiter is not provided, we want to use a comma as the default delimiter. Here’s how the script can be implemented:
#!/bin/bash filename=$1 delimiter=${2:-,} echo "Filename: $filename" echo "Delimiter: $delimiter"
In this script, the second argument (delimiter) is assigned a default value of comma (,) using the ${2:-,}
syntax. This means that if the second argument is not provided, the value of $delimiter
will be set to comma (,). If the second argument is provided, its value will be used instead.
Now, let’s execute the script with and without the delimiter argument:
$ ./my_script.sh data.txt
Output:
Filename: data.txt Delimiter: ,
$ ./my_script.sh data.txt ;
Output:
Filename: data.txt Delimiter: ;
As you can see, the script handles the optional delimiter argument by assigning a default value if it is not provided.
It’s worth noting that you can have multiple optional arguments in a script by extending this approach. Simply assign default values to each optional argument using the ${n:-default}
syntax, where n
represents the position of the argument.
What Happens if I Exceed the Maximum Number of Arguments in a Bash Script?
If you exceed the maximum number of arguments in a bash script, you may encounter various issues depending on the specific scenario and system limitations. Some potential consequences of exceeding the maximum number of arguments include:
1. Error messages: When you exceed the maximum number of arguments, the script may produce error messages indicating that the command-line length has been exceeded. These error messages can help identify the issue and provide information on how to resolve it.
2. Unexpected behavior: Exceeding the maximum number of arguments can lead to unexpected behavior in your script. This can include incorrect results, crashes, or other issues that may arise due to the excessive input.
3. Performance degradation: Handling a large number of arguments can impact the performance of your script, especially if it involves complex operations or requires significant memory usage. Exceeding the maximum number of arguments can exacerbate performance degradation and result in slower execution times.
To avoid exceeding the maximum number of arguments, it is recommended to carefully design your script and consider alternative approaches if you anticipate a large number of inputs. This may involve using input files, environment variables, or other methods to pass and handle the required data.
How to Check if a Bash Script Received a Specific Argument?
In some cases, you may want to check if a bash script received a specific argument to perform certain actions or make decisions within the script’s logic. Bash provides several ways to check for specific arguments, such as using conditional statements and the $#
variable.
The $#
variable in bash represents the total number of arguments passed to a script. By comparing the value of $#
with a desired number, you can determine if a specific argument was provided.
Let’s consider an example where we have a script that expects a single argument representing a filename. We want to check if the script received the argument and display a message accordingly. Here’s how the script can be implemented:
#!/bin/bash filename=$1 if [ $# -eq 1 ]; then echo "File name provided: $filename" else echo "No file name provided" fi
In this script, we use the conditional statement [ $# -eq 1 ]
to check if the number of arguments is equal to 1. If it is, we assume that a file name argument was provided and display a message indicating the provided file name. Otherwise, we display a message indicating that no file name was provided.
Now, let’s execute the script with and without a file name argument:
$ ./my_script.sh data.txt
Output:
File name provided: data.txt
$ ./my_script.sh
Output:
No file name provided
As you can see, the script checks if a specific argument (in this case, the file name) was provided and takes appropriate action based on the result.
It’s worth noting that you can check for multiple specific arguments by extending this approach. Simply add additional conditional statements or use a case
statement to check for different arguments and perform corresponding actions.
Related Article: How to Use If-Else Statements in Shell Scripts
Are There Any Restrictions on the Length of Arguments in a Bash Script?
As mentioned earlier, the maximum length of arguments in a bash script is typically determined by the operating system’s maximum command-line length. This maximum length can vary depending on the system, but it is important to note that there are limitations on the length of individual arguments as well.
The length of an argument in a bash script is subject to the maximum length of a string that can be stored in memory. This maximum length can depend on various factors such as the system architecture, available memory, and system settings.
In general, the maximum length of an argument in a bash script is limited by the maximum length of a string in the system’s environment. This maximum length can vary, but it is typically in the range of several thousand characters.
It is important to consider these limitations when designing your bash scripts and handling arguments. If you anticipate arguments with very long lengths, it may be necessary to use alternative methods such as passing arguments through files or using environment variables to avoid exceeding the maximum length.
Can I Pass Arguments to a Bash Script Using Environment Variables?
Yes, you can pass arguments to a bash script using environment variables. Environment variables are a way to store and pass information to processes, including bash scripts.
To pass arguments using environment variables, you can set the desired environment variables before executing the script. The script can then access the values of these variables within its logic.
Let’s consider an example where we have a script that expects two arguments: a username and a password. Instead of passing the arguments directly, we can use environment variables to store and pass the values. Here’s how the script can be implemented:
#!/bin/bash username=$USERNAME password=$PASSWORD echo "Username: $username" echo "Password: $password"
In this script, we assign the values of the environment variables $USERNAME
and $PASSWORD
to the variables $username
and $password
, respectively. These environment variables can be set before executing the script using the export
command.
Now, let’s set the environment variables and execute the script:
$ export USERNAME=johndoe $ export PASSWORD=secretpassword $ ./my_script.sh
Output:
Username: johndoe Password: secretpassword
As you can see, the script accesses the values of the environment variables and uses them as the script’s arguments.
Using environment variables to pass arguments can be useful in situations where you want to provide sensitive information, such as passwords, without directly exposing them in the command-line arguments.
It’s worth noting that environment variables are not limited to passing arguments to a script. They can also be used to store and access other types of information that may be required by the script during its execution.
Is it Possible to Pass Arguments to a Bash Script Using a File?
Yes, it is possible to pass arguments to a bash script using a file. This can be useful when you have a large number of arguments or when the arguments contain special characters that may be difficult to pass through the command line.
To pass arguments using a file, you can store the arguments in a text file, with each argument on a separate line. The script can then read the contents of the file and process the arguments accordingly.
Let’s consider an example where we have a script that expects multiple arguments. Instead of passing the arguments directly, we can use a file to store the arguments. Here’s how the script can be implemented:
#!/bin/bash filename=$1 arguments_file=$2 echo "Filename: $filename" echo "Arguments:" while IFS= read -r argument do echo "- $argument" done < "$arguments_file"
In this script, we use the while
loop and the read
command to read each argument from the file specified as the second argument. The IFS= read -r argument
line reads each line from the file and assigns it to the $argument
variable. The loop then processes each argument by echoing it with a hyphen prefix.
Now, let’s create a file with the arguments and execute the script:
$ echo "argument1" > arguments.txt $ echo "argument2" >> arguments.txt $ ./my_script.sh data.txt arguments.txt
Output:
Filename: data.txt Arguments: - argument1 - argument2
As you can see, the script reads the arguments from the file and processes them accordingly.
Using a file to pass arguments can be useful in situations where you have a large number of arguments or when the arguments contain special characters that may be difficult to pass through the command line. Additionally, it provides a convenient way to store and manage arguments separately from the script’s execution.
It’s worth noting that you can use different file formats or structures to store the arguments, depending on your specific requirements. For example, you can use JSON, YAML, or other structured data formats to pass arguments in a more structured manner.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
How to Handle Spaces and Special Characters in Bash Script Arguments?
Handling spaces and special characters in bash script arguments can be challenging because these characters can be interpreted by the shell and lead to unexpected behavior. However, there are techniques that can be used to handle spaces and special characters effectively.
One common technique is to use quotes to group arguments and treat them as a single entity. There are three types of quotes that can be used in bash: single quotes (''
), double quotes (" "
), and backticks. Each type of quote has different behavior when it comes to handling spaces and special characters.
Single quotes (''
) treat everything within them as a literal string, ignoring any variable expansions or special characters. This means that spaces and special characters within single quotes are treated as regular characters.
Double quotes (" "
) allow variable expansions within them and treat most special characters as literal characters. However, they still interpret some special characters, such as the dollar sign ($), backticks, and backslashes (\), which have special meaning within double quotes.
Backticks also known as backquotes, are used to execute commands and substitute their output into the argument. They are less commonly used for handling spaces and special characters directly in arguments.
Let’s consider an example where we have a script that expects a single argument containing spaces. We want to handle the argument correctly, regardless of the spaces it contains. Here’s how the script can be implemented:
#!/bin/bash argument=$1 echo "Argument: $argument"
Now, let’s execute the script with different arguments containing spaces:
$ ./my_script.sh 'hello world'
Output:
Argument: hello world
$ ./my_script.sh "hello world"
Output:
Argument: hello world
As you can see, using quotes allows us to handle spaces within the argument correctly.
To handle special characters within arguments, you can also use escape sequences. An escape sequence starts with a backslash (\) and is used to represent special characters as literal characters. For example, to include a space or a dollar sign within an argument, you can escape it by preceding it with a backslash (\).
Let’s consider an example where we have a script that expects a single argument containing a dollar sign ($). We want to handle the argument correctly, treating the dollar sign as a literal character. Here’s how the script can be implemented:
#!/bin/bash argument=$1 echo "Argument: $argument"
Now, let’s execute the script with an argument containing a dollar sign:
$ ./my_script.sh 'hello $world'
Output:
Argument: hello $world
As you can see, using quotes and escape sequences allows us to handle special characters within the argument correctly.
It’s worth noting that the specific approach for handling spaces and special characters may vary depending on the context and requirements of your script. It is recommended to test and validate the behavior of your script with different arguments to ensure that it handles spaces and special characters correctly.
What is the Difference Between Positional Parameters and Named Parameters in a Bash Script?
In bash scripts, parameters are values that are passed to a script when it is executed. Parameters can be accessed within the script using positional parameters or named parameters.
Positional parameters refer to the arguments that are passed to the script based on their position in the command-line. The first argument is accessed using $1
, the second argument using $2
, and so on. Positional parameters provide a simple and straightforward way to access and use arguments within a script.
Named parameters, on the other hand, are variables that are assigned specific values based on their names. Named parameters are typically used when you want to provide more descriptive and meaningful names for the arguments, rather than relying on their positions.
To use named parameters in a bash script, you can assign values to variables using the =
operator. These variables can then be accessed and used within the script’s logic.
Let’s consider an example where we have a script that expects two named parameters: --input
and --output
. Here’s how the script can be implemented:
#!/bin/bash while [[ $# -gt 0 ]]; do key="$1" case $key in --input) input_file="$2" shift shift ;; --output) output_file="$2" shift shift ;; *) echo "Unknown option: $1" shift ;; esac done echo "Input file: $input_file" echo "Output file: $output_file"
In this script, we use a while
loop and a case
statement to iterate through the named parameters. The key="$1"
line assigns the current parameter to the $key
variable. The case
statement then checks the value of $key
and assigns the corresponding value to the appropriate variable ($input_file
or $output_file
). The shift
command is used to move to the next parameter and discard the current one.
Now, let’s execute the script with named parameters:
$ ./my_script.sh --input data.txt --output output.txt
Output:
Input file: data.txt Output file: output.txt
As you can see, using named parameters allows us to provide more descriptive names for the arguments and make the script more readable and user-friendly.
It’s worth noting that positional parameters and named parameters can be used together in a bash script. This provides flexibility in how arguments can be passed and accessed within the script. You can choose the appropriate approach based on the specific requirements and context of your script.