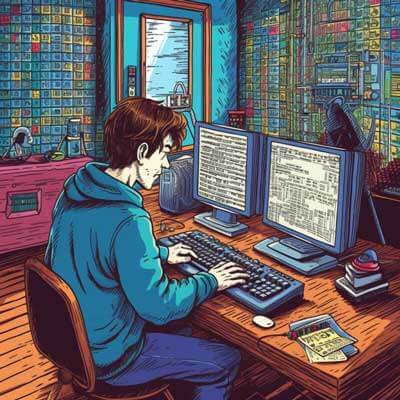
Table of Contents
When accessing a MySQL database from a Bash script, it's important to ensure that the access is secure and protected from unauthorized access. This involves implementing authentication and encryption mechanisms to prevent data breaches and unauthorized access to the database.
There are several best practices to follow when securely accessing a MySQL database from a Bash script:
1. Never hardcode database credentials in the script: Hardcoding sensitive information like database usernames and passwords in a script is a security risk. Instead, consider using environment variables or separate configuration files to store the credentials securely.
2. Use strong, unique passwords for database users: Ensure that the passwords used for database authentication are strong and unique. Avoid using common or easily guessable passwords.
3. Limit the privileges of the database user: When creating a database user for the Bash script, only grant the necessary privileges required for the script to perform its intended tasks. Restricting privileges helps minimize the impact of a potential security breach.
4. Implement SSL/TLS encryption for database connections: Secure Sockets Layer (SSL) or Transport Layer Security (TLS) encryption can be used to encrypt the communication between the Bash script and the MySQL server. This prevents unauthorized interception of sensitive data.
5. Regularly update and patch MySQL: Keeping your MySQL server up to date with the latest security patches and updates is crucial for maintaining a secure database environment.
Example 1: Securely Accessing MySQL from a Bash Script
Here's an example of a Bash script that securely accesses a MySQL database using environment variables for the credentials:
#!/bin/bash DB_USER="$MYSQL_USER" DB_PASSWORD="$MYSQL_PASSWORD" DB_NAME="database" # Rest of the script
In this example, instead of hardcoding the database credentials, the script uses environment variables ($MYSQL_USER
and $MYSQL_PASSWORD
) to store and retrieve the credentials. This approach allows for secure storage of sensitive information outside of the script.
Related Article: Adding Color to Bash Scripts in Linux
Example 2: Bash Script with SSL/TLS Encryption
Here's an example of a Bash script that uses SSL/TLS encryption to establish a secure connection with the MySQL server:
#!/bin/bash DB_HOST="localhost" DB_USER="username" DB_PASSWORD="password" DB_NAME="database" mysql --ssl-ca=/path/to/ca-cert.pem --ssl-cert=/path/to/client-cert.pem --ssl-key=/path/to/client-key.pem -h $DB_HOST -u $DB_USER -p$DB_PASSWORD $DB_NAME
In this example, the mysql
command is used to connect to the MySQL server with SSL/TLS encryption. The --ssl-ca
, --ssl-cert
, and --ssl-key
options are used to specify the paths to the CA certificate, client certificate, and client key files respectively. This ensures that the connection is established securely.
Best Practices for Securing MySQL Access in a Bash Script
When securing MySQL access in a Bash script, it's important to follow best practices to minimize the risk of security breaches and unauthorized access. These best practices include:
1. Use strong, unique passwords for MySQL users: Ensure that the passwords used for MySQL authentication are strong and unique. Avoid using common or easily guessable passwords.
2. Implement SSL/TLS encryption for MySQL connections: Enable SSL/TLS encryption for MySQL connections to encrypt the communication between the client and the server. This helps protect sensitive data from unauthorized interception.
3. Limit the privileges of MySQL users: When creating MySQL users for the Bash script, grant only the necessary privileges required for the script to perform its intended tasks. Restricting privileges helps minimize the impact of a potential security breach.
4. Regularly update and patch MySQL: Keep your MySQL server up to date with the latest security patches and updates to ensure that any known vulnerabilities are addressed.
Example 1: Using Strong Passwords for MySQL Users
When creating a MySQL user for the Bash script, it's important to use a strong, unique password. Here's an example of how to create a user with a strong password:
CREATE USER 'username'@'localhost' IDENTIFIED BY 'strong_password'; GRANT ALL PRIVILEGES ON database.* TO 'username'@'localhost'; FLUSH PRIVILEGES;
In this example, the CREATE USER
statement is used to create a MySQL user with the specified username and a strong password. The GRANT ALL PRIVILEGES
statement grants all privileges on the specified database to the user. Finally, the FLUSH PRIVILEGES
statement refreshes the MySQL privileges to apply the changes.
Related Article: Executing Scripts in Linux Without Bash Command Line
Example 2: Enabling SSL/TLS Encryption for MySQL Connections
To enable SSL/TLS encryption for MySQL connections, you need to configure the MySQL server and client accordingly. Here's an example of how to enable SSL/TLS encryption for MySQL connections:
1. Generate SSL/TLS certificates:
openssl req -x509 -nodes -days 365 -newkey rsa:2048 -keyout server-key.pem -out server-cert.pem
2. Configure MySQL server:
[mysqld] ssl-ca=/path/to/ca-cert.pem ssl-cert=/path/to/server-cert.pem ssl-key=/path/to/server-key.pem
3. Configure MySQL client (Bash script):
#!/bin/bash DB_HOST="localhost" DB_USER="username" DB_PASSWORD="password" DB_NAME="database" mysql --ssl-ca=/path/to/ca-cert.pem --ssl-cert=/path/to/client-cert.pem --ssl-key=/path/to/client-key.pem -h $DB_HOST -u $DB_USER -p$DB_PASSWORD $DB_NAME
In this example, the openssl
command is used to generate self-signed SSL/TLS certificates for the MySQL server. The server configuration file (my.cnf
) is then updated to specify the paths to the CA certificate, server certificate, and server key files. Finally, the Bash script is updated to use the appropriate SSL/TLS options when connecting to the MySQL server.
Setting Up an SSH Tunnel for Secure MySQL Access
Setting up an SSH tunnel is a secure way to access a remote MySQL server from a local machine. An SSH tunnel creates a secure connection between the local machine and the remote server, allowing MySQL traffic to be securely transmitted over the encrypted tunnel.
To set up an SSH tunnel for secure MySQL access, follow these steps:
1. Connect to the remote server via SSH:
ssh user@remote-server
2. Set up the SSH tunnel:
ssh -L 3306:localhost:3306 user@remote-server
3. Open a new terminal window and connect to the MySQL server using the local port:
mysql -h localhost -P 3306 -u username -p
4. Enter the MySQL password when prompted to establish the connection.
Setting up an SSH tunnel provides an additional layer of security by encrypting the connection between the local machine and the remote MySQL server. This ensures that sensitive data, including database credentials and query results, are protected from unauthorized access.
Example: Setting Up an SSH Tunnel for Secure MySQL Access
Here's an example of how to set up an SSH tunnel for secure MySQL access:
ssh -L 3306:localhost:3306 user@remote-server
In this example, the ssh
command is used to establish an SSH connection to the remote server (remote-server
) using the specified username (user
). The -L
option is used to set up the SSH tunnel, forwarding traffic from the local port 3306 to the remote server's port 3306. This allows MySQL traffic to be securely transmitted over the encrypted tunnel.
Once the SSH tunnel is established, you can use the mysql
command to connect to the MySQL server on the remote server using the local port:
mysql -h localhost -P 3306 -u username -p
In this example, the mysql
command is used to connect to the MySQL server on the localhost (-h localhost
) using the specified port (-P 3306
), username (-u username
), and password (-p
). The connection is established through the SSH tunnel, ensuring secure access to the remote MySQL server.