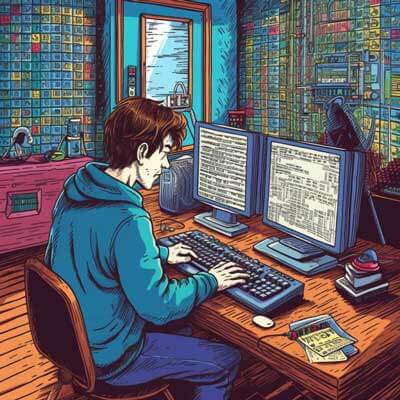
- Syntax for Using Variables in If Statements
- Variable Substitution in If Statements
- Variable Expansion in If Statements
- Comparing Variables in If Statements
- Conditional Expressions in If Statements
- Examples of Using Variables in If Statements
- Example 1: Checking if a File Exists
- Example 2: Checking if a Number is Even or Odd
- Best Practices for Using Variables in If Statements
- Limitations and Restrictions of Using Variables in If Statements
In Bash scripting, variables are an essential component for storing and manipulating data. They allow you to store values and use them in different parts of your script. One common use case for variables is within if statements, where you can evaluate conditions and perform different actions based on the result.
Syntax for Using Variables in If Statements
To use variables in if statements, you need to follow a specific syntax. The basic structure of an if statement in Bash is as follows:
if [ condition ] then # code to be executed if the condition is true fi
To use a variable in the condition, you can simply include the variable name within the square brackets. For example:
if [ $variable -eq 10 ] then echo "The variable is equal to 10" fi
In this example, the if statement checks if the value of the variable is equal to 10. If the condition is true, the code inside the if block will be executed.
Related Article: How To Echo a Newline In Bash
Variable Substitution in If Statements
Variable substitution is a useful feature in Bash that allows you to replace a variable with its value when using it in a command or expression. This can be particularly useful when working with if statements.
Consider the following example:
name="John" if [ "$name" == "John" ] then echo "Hello, John!" fi
In this example, the variable $name
is substituted with its value, which is “John”. The if statement then checks if the value is equal to “John”. If the condition is true, the message “Hello, John!” will be printed.
Variable Expansion in If Statements
Variable expansion is another feature in Bash that allows you to perform operations on variables before using them in if statements. One common use case is when you want to check if a variable is empty or not.
Here’s an example:
name="John" if [ -z "$name" ] then echo "The name is empty" else echo "The name is not empty" fi
In this example, the -z
flag is used to check if the length of the variable $name
is zero. If the condition is true, it means the variable is empty, and the message “The name is empty” will be printed. Otherwise, the message “The name is not empty” will be printed.
Comparing Variables in If Statements
In addition to checking if a variable is equal to a specific value, you can also compare variables against each other in if statements. Bash provides several comparison operators that you can use for this purpose.
Here are some examples:
a=10 b=20 if [ $a -lt $b ] then echo "a is less than b" fi if [ $a -eq $b ] then echo "a is equal to b" fi if [ $a -gt $b ] then echo "a is greater than b" fi
In these examples, the -lt
operator is used to check if $a
is less than $b
, the -eq
operator is used to check if they are equal, and the -gt
operator is used to check if $a
is greater than $b
.
Related Article: How to Use If-Else Statements in Shell Scripts
Conditional Expressions in If Statements
Conditional expressions provide a more flexible way of evaluating conditions in if statements. They allow you to combine multiple conditions using logical operators such as &&
(AND) and ||
(OR).
Here’s an example:
a=10 b=20 c=30 if [ $a -lt $b ] && [ $b -lt $c ] then echo "a is less than b and b is less than c" fi
In this example, the if statement checks if both conditions are true: $a
is less than $b
and $b
is less than $c
. If both conditions are true, the message “a is less than b and b is less than c” will be printed.
Examples of Using Variables in If Statements
Now let’s look at some practical examples of using variables in if statements.
Example 1: Checking if a File Exists
filename="example.txt" if [ -f "$filename" ] then echo "The file $filename exists" else echo "The file $filename does not exist" fi
In this example, the if statement checks if the file with the name stored in the variable $filename
exists. If the condition is true, the message “The file example.txt exists” will be printed. Otherwise, the message “The file example.txt does not exist” will be printed.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Example 2: Checking if a Number is Even or Odd
number=10 if [ $(($number % 2)) -eq 0 ] then echo "The number $number is even" else echo "The number $number is odd" fi
In this example, the if statement uses the modulus operator %
to check if the number stored in the variable $number
is divisible by 2. If the condition is true, it means the number is even, and the message “The number 10 is even” will be printed. Otherwise, the message “The number 10 is odd” will be printed.
Best Practices for Using Variables in If Statements
When using variables in if statements, it’s important to follow some best practices to ensure your code is clear, maintainable, and error-free.
1. Always quote your variables: It’s a good practice to enclose your variables in double quotes to prevent issues with whitespace and special characters. For example, [ "$variable" == "value" ]
instead of [ $variable == "value" ]
.
2. Use descriptive variable names: Choose meaningful variable names that clearly indicate their purpose. This will make your code easier to understand and maintain.
3. Comment your code: Add comments to explain the purpose of your if statements and any complex conditions. This will make it easier for others (and yourself) to understand your code in the future.
4. Test your conditions: Before running your script, test your if statements with different values to ensure they are working as expected. This will help you catch any errors or unexpected behavior.
Limitations and Restrictions of Using Variables in If Statements
While variables are a useful tool in Bash scripting, there are some limitations and restrictions to keep in mind when using them in if statements.
1. Variable scoping: Variables in Bash have a global scope by default, which means they can be accessed from any part of the script. However, if you declare a variable inside a function, it will have a local scope and can only be accessed within that function.
2. Variable types: Bash does not have explicit variable types, so you need to be careful when comparing variables in if statements. For example, comparing a string variable with an integer variable may lead to unexpected results.
3. Variable names: Bash has some restrictions on variable names. They can only contain alphanumeric characters and underscores, and they cannot start with a number.
4. Missing variables: If a variable used in an if statement is not defined or empty, it will produce an error or unexpected behavior. It’s important to handle such cases properly to avoid issues.