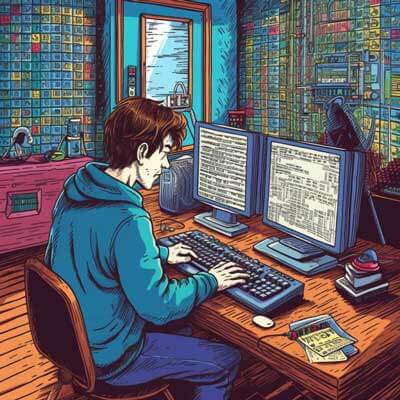
- Understanding Color Codes for Bash Scripting
- Syntax Highlighting in Bash Scripts
- Colorizing Terminal Output in Bash Scripts
- Recommended Terminal Color Schemes for Bash Scripting
- Libraries and Tools for Adding Color to Bash Scripts
- Using Color Escape Sequences in Bash Scripting
- Colorizing Specific Parts of Output in Bash Scripts
- Best Practices for Using Color in Bash Scripts
- Customizing Terminal Color Scheme for Bash Scripting
- Additional Resources
Understanding Color Codes for Bash Scripting
When it comes to adding color to bash scripts in Linux, it’s important to understand how color codes work. In bash scripting, color codes are represented by special escape sequences that are interpreted by the terminal emulator. These escape sequences begin with the escape character, followed by a bracket, and end with a lowercase letter or number. The escape character is represented by “\033” or “\e”.
To add color to your bash scripts, you need to use these escape sequences along with specific color codes. Here are some commonly used color codes:
– Black: \e[30m
– Red: \e[31m
– Green: \e[32m
– Yellow: \e[33m
– Blue: \e[34m
– Magenta: \e[35m
– Cyan: \e[36m
– White: \e[37m
For example, to print the text “Hello, World!” in red, you can use the following code snippet:
echo -e "\e[31mHello, World!\e[0m"
In this example, the escape sequence “\e[31m” sets the color to red, and the escape sequence “\e[0m” resets the color back to the default.
It’s important to note that these color codes only affect the text that follows them. If you want to apply color to a specific part of the output, you can use these color codes accordingly.
Related Article: How to Calculate the Sum of Inputs in Bash Scripts
Syntax Highlighting in Bash Scripts
Syntax highlighting is a useful feature that helps to improve the readability of code by highlighting different elements in different colors. While syntax highlighting is commonly associated with text editors and IDEs, you can also enable it in bash scripts to make them more visually appealing.
To enable syntax highlighting in bash scripts, you can use a tool called Pygments. Pygments is a syntax highlighter written in Python that supports a wide range of programming languages, including bash.
First, you need to install Pygments on your system. You can do this by running the following command:
pip install Pygments
Once Pygments is installed, you can use the pygmentize
command to generate syntax-highlighted output. For example, to syntax highlight a bash script file called script.sh
, you can use the following command:
pygmentize -f terminal256 -O style=native -l bash script.sh
In this example, the -f terminal256
option specifies the output format as 256-color terminal, the -O style=native
option sets the color scheme to the native terminal color scheme, and the -l bash
option specifies the language as bash.
You can also customize the color scheme by specifying a different style. Pygments provides a wide range of built-in styles, such as monokai
, tango
, vs
, and more. You can specify a different style using the -O style=<style>
option.
Colorizing Terminal Output in Bash Scripts
In addition to adding color to specific parts of your bash script output, you can also colorize the entire terminal output. This can be useful for distinguishing different types of output or highlighting important information.
To colorize the terminal output in bash scripts, you can use the tput
command along with ANSI color codes. The tput
command is a terminal utility that allows you to interact with the terminal’s capabilities, such as changing text color.
Here’s an example of how to colorize the terminal output in a bash script:
#!/bin/bash # Set color variables RED=$(tput setaf 1) GREEN=$(tput setaf 2) YELLOW=$(tput setaf 3) RESET=$(tput sgr0) # Print colored output echo "${RED}Error:${RESET} Something went wrong." echo "${GREEN}Success:${RESET} Operation completed successfully." echo "${YELLOW}Warning:${RESET} Proceed with caution."
In this example, we use the tput setaf <color>
command to set the foreground color. The <color>
argument can be a number between 0 and 255, representing the desired color. The tput sgr0
command resets the color back to the default.
You can also use other tput
commands to change other text attributes, such as bold, underline, and blink. These attributes can further enhance the appearance of your terminal output.
Recommended Terminal Color Schemes for Bash Scripting
Choosing the right color scheme for your bash scripts can greatly enhance the readability and aesthetics of your code. Here are some recommended terminal color schemes that work well for bash scripting:
1. Solarized: Solarized is a popular color scheme that provides a balanced and soothing color palette. It offers both light and dark variants, making it suitable for different lighting conditions. You can find the Solarized color scheme for various terminal emulators, such as iTerm, GNOME Terminal, and Konsole.
2. Monokai: Monokai is a vibrant color scheme that is widely used in code editors and terminals. It features bold and contrasting colors that make the code stand out. Monokai is available for popular terminal emulators, including iTerm, GNOME Terminal, and Terminator.
3. Tomorrow: Tomorrow is a set of four color schemes that are designed to be easy on the eyes and provide good contrast. These color schemes are available for various terminal emulators, such as iTerm, GNOME Terminal, and Alacritty.
4. Dracula: Dracula is a dark color scheme that is known for its high contrast and vibrant colors. It is available for a wide range of terminal emulators, including iTerm, GNOME Terminal, and Konsole.
To install and use these color schemes, you can follow the instructions provided by the respective terminal emulator’s documentation or refer to the official websites of these color schemes.
Related Article: Locating Largest Memory in Bash Script on Linux
Libraries and Tools for Adding Color to Bash Scripts
While manually adding escape sequences and color codes to your bash scripts can be effective, it can also be time-consuming and error-prone. Fortunately, there are several libraries and tools available that can simplify the process of adding color to bash scripts.
1. BashColors: BashColors is a lightweight bash library that provides an easy-to-use interface for adding colors to your script output. It allows you to use simple function calls instead of manually typing escape sequences. BashColors supports both foreground and background colors, as well as text attributes like bold and underline. You can find the library and usage examples on GitHub.
2. Bash-Snippets: Bash-Snippets is a collection of useful bash scripts and snippets, including a script for adding color to your script output. The colorize
script in Bash-Snippets allows you to colorize specific parts of your output using a simple syntax. It supports a wide range of colors and text attributes. You can find the repository and usage examples on GitHub.
3. Colorize: Colorize is a command-line tool that allows you to colorize text from standard input or files. It supports a wide range of colors and text attributes, and it can be easily integrated into your bash scripts. Colorize is available as a package for various Linux distributions, and you can find the source code and usage examples on GitHub.
These libraries and tools provide convenient ways to add color to your bash scripts without the need for manual escape sequence handling. You can choose the one that best fits your needs and integrate it into your scripts to enhance their appearance.
Using Color Escape Sequences in Bash Scripting
Color escape sequences are special codes that can be used to change the color of text in bash scripts. These sequences are interpreted by the terminal emulator and can be used to add color to the output of your scripts.
Here are some examples of color escape sequences that you can use in your bash scripts:
– Black: \033[30m
– Red: \033[31m
– Green: \033[32m
– Yellow: \033[33m
– Blue: \033[34m
– Magenta: \033[35m
– Cyan: \033[36m
– White: \033[37m
To use these escape sequences, you can use the echo
command with the -e
option to enable interpretation of escape sequences. For example, to print the text “Hello, World!” in red, you can use the following code:
echo -e "\033[31mHello, World!\033[0m"
In this example, the escape sequence \033[31m
sets the color to red, and the escape sequence \033[0m
resets the color back to the default.
You can also combine multiple escape sequences to change the color of different parts of your output. For example, to print the text “Error: Something went wrong.” in red and “Success: Operation completed successfully.” in green, you can use the following code:
echo -e "\033[31mError:\033[0m \033[1mSomething went wrong.\033[0m" echo -e "\033[32mSuccess:\033[0m \033[1mOperation completed successfully.\033[0m"
In this example, the escape sequence \033[31m
sets the color to red, \033[32m
sets the color to green, and \033[1m
makes the text bold. The escape sequence \033[0m
resets the color and text attributes back to the default.
Colorizing Specific Parts of Output in Bash Scripts
In some cases, you may want to colorize specific parts of the output in your bash scripts, such as highlighting error messages or emphasizing important information. You can achieve this by using color escape sequences selectively.
Here’s an example of how to colorize specific parts of the output in a bash script:
#!/bin/bash # Set color variables ERROR_COLOR="\033[31m" SUCCESS_COLOR="\033[32m" RESET_COLOR="\033[0m" # Print output with color echo -e "Processing files..." # Simulate an error echo -e "${ERROR_COLOR}Error:${RESET_COLOR} File not found: file.txt" # Simulate a success message echo -e "${SUCCESS_COLOR}Success:${RESET_COLOR} File processed successfully."
In this example, we define color variables for error and success messages using color escape sequences. We then use these color variables to selectively colorize parts of the output. The ${RESET_COLOR}
escape sequence is used to reset the color back to the default.
Related Article: Terminate Bash Script Loop via Keyboard Interrupt in Linux
Best Practices for Using Color in Bash Scripts
While adding color to your bash scripts can make them more visually appealing and easier to read, it’s important to use color judiciously and follow some best practices. Here are some best practices for using color in bash scripts:
1. Use color sparingly: Avoid excessive use of color, as it can make the output overwhelming and hard to read. Reserve color for highlighting important information or distinguishing different types of output.
2. Consider color blindness: Keep in mind that some users may have color blindness or other visual impairments. When using color, make sure to provide alternative ways of conveying the same information, such as using different text styles or symbols.
3. Use meaningful colors: Choose colors that have clear meanings and associations. For example, use red for errors or warnings, green for success messages, and yellow for informational messages. This will make it easier for users to understand the meaning of the colored output.
4. Test on different terminals: Colors may appear differently on different terminal emulators or operating systems. Make sure to test your bash scripts on different terminals to ensure consistent and readable output.
5. Provide fallback options: If you’re using color escape sequences or libraries that rely on them, make sure to provide fallback options for terminals that do not support colors. This can be done by checking the terminal capabilities and conditionally enabling or disabling color output.
Customizing Terminal Color Scheme for Bash Scripting
If you want to customize the color scheme of your terminal specifically for bash scripting, you can modify the settings of your terminal emulator to suit your preferences. The exact steps for customizing the color scheme will depend on the terminal emulator you are using.
Here are some general steps for customizing the terminal color scheme:
1. Open the preferences or settings of your terminal emulator. This can usually be done by right-clicking on the terminal window and selecting “Preferences” or “Settings”.
2. Look for the color settings or themes section. This is where you can customize the color scheme of your terminal.
3. Choose a color scheme that you like or create a custom color scheme. Most terminal emulators provide a range of pre-defined color schemes that you can choose from. Alternatively, you can create a custom color scheme by selecting individual colors for different elements.
4. Save the changes and apply the new color scheme. Once you have customized the color scheme to your liking, save the changes and apply the new color scheme to your terminal.