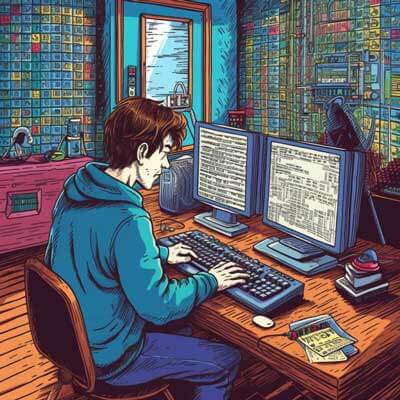
- What is SQLite and how is it used in Linux?
- How can I execute multiple SQLite statements in a bash script?
- Are there any limitations or considerations when executing multiple SQLite statements in a bash script?
- What are the advantages of using a bash script to execute multiple SQLite statements?
- Can I execute multiple SQLite statements directly from the command line?
- Is it possible to execute multiple SQLite statements in a shell script without using a bash script?
- What are some alternatives to executing multiple SQLite statements in a bash script?
- Are there any best practices or recommended approaches for executing multiple SQLite statements in a bash script?
- Additional Resources
What is SQLite and how is it used in Linux?
SQLite is a lightweight, file-based, embedded relational database management system (RDBMS) that is widely used in various applications and platforms. It is designed to be self-contained, serverless, and zero-configuration, making it easy to use and deploy. SQLite stores data in a single, portable file, making it suitable for small to medium-sized databases.
To use SQLite in Linux, you need to have the SQLite package installed on your system. You can check if SQLite is installed by running the following command in your terminal:
sqlite3 --version
If SQLite is not installed, you can install it using the package manager for your Linux distribution. For example, on Ubuntu, you can install SQLite by running the following command:
sudo apt-get install sqlite3
Once SQLite is installed, you can create and manage databases using the sqlite3
command-line tool. You can execute SQL statements, create tables, insert data, query data, and perform other database operations using SQLite commands.
Related Article: Executing Bash Scripts with Chef Apply in Linux
How can I execute multiple SQLite statements in a bash script?
To execute multiple SQLite statements in a bash script, you can make use of the sqlite3
command-line tool. The sqlite3
tool allows you to interact with SQLite databases by executing SQL statements.
Here’s an example of a bash script that executes multiple SQLite statements:
#!/bin/bash sqlite3 my_database.db <<EOF CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER); INSERT INTO users (name, age) VALUES ('John Doe', 30); INSERT INTO users (name, age) VALUES ('Jane Smith', 25); SELECT * FROM users; EOF
In this example, the script creates a table named users
in the my_database.db
database, inserts two rows of data into the users
table, and then selects and displays all the rows from the users
table.
The <<EOF
syntax is called a “here-document” or “here-script” in bash. It allows you to include multiple lines of input within the script without the need for external files. The EOF
at the end of the script signifies the end of the input.
When you run the script, it will execute each SQLite statement sequentially, producing the desired result.
Are there any limitations or considerations when executing multiple SQLite statements in a bash script?
When executing multiple SQLite statements in a bash script, there are a few limitations and considerations to keep in mind:
1. Syntax: Each SQLite statement should be written on a separate line and terminated with a semicolon (;). The statements should be properly formatted and follow the correct SQL syntax.
2. Error handling: It is important to handle any potential errors that may occur during the execution of the SQLite statements. You can use conditional statements and error checking mechanisms to ensure the script behaves as expected.
3. Input validation: When accepting user input or dynamic values in the SQLite statements, it is essential to validate and sanitize the input to prevent SQL injection attacks or unexpected behavior.
4. Transaction management: By default, each SQLite statement in a bash script is executed as a separate transaction. If you need to perform a series of statements as a single transaction, you can use the BEGIN TRANSACTION
, COMMIT
, and ROLLBACK
statements.
5. Performance: Executing multiple SQLite statements in a bash script can impact performance, especially when dealing with large databases or complex operations. It is important to optimize the script and consider using indexes, query optimization techniques, and other performance-enhancing strategies.
What are the advantages of using a bash script to execute multiple SQLite statements?
Using a bash script to execute multiple SQLite statements offers several advantages:
1. Automation: Bash scripts allow you to automate repetitive tasks and execute multiple SQLite statements in a single run. This saves time and effort compared to manually typing and executing each statement.
2. Scriptability: Bash scripts are highly scriptable and can be easily modified and extended. You can add conditional statements, loops, and other programming constructs to make the script more useful and flexible.
3. Easy integration: Bash scripts can be easily integrated into existing workflows and pipelines. They can be executed from the command line, scheduled as cron jobs, or triggered by other scripts or applications.
4. Portability: Bash scripts are portable across different Linux distributions and platforms. As long as the required dependencies (such as SQLite) are available, the script can be executed on any compatible system without modification.
5. Version control: Bash scripts can be version-controlled using tools like Git, allowing you to track changes, collaborate with others, and revert to previous versions if needed.
6. Debugging and error handling: Bash scripts provide mechanisms for error handling, debugging, and logging. You can handle errors gracefully, log output for troubleshooting, and capture exit codes to determine the success or failure of the script.
Overall, using a bash script to execute multiple SQLite statements provides a flexible and efficient way to interact with databases and automate data management tasks.
Can I execute multiple SQLite statements directly from the command line?
Yes, you can execute multiple SQLite statements directly from the command line using the sqlite3
command-line tool. The sqlite3
tool allows you to open and interact with SQLite databases, and you can pass SQL statements as arguments to the tool.
Here’s an example of executing multiple SQLite statements from the command line:
sqlite3 my_database.db " CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER); INSERT INTO users (name, age) VALUES ('John Doe', 30); INSERT INTO users (name, age) VALUES ('Jane Smith', 25); SELECT * FROM users; "
In this example, the SQLite statements are enclosed within double quotes and passed as a single argument to the sqlite3
command. The statements are executed sequentially, producing the desired result.
Executing multiple SQLite statements directly from the command line can be useful for quick ad hoc queries or when you need to perform simple operations on a database without the need for a separate script.
Is it possible to execute multiple SQLite statements in a shell script without using a bash script?
Yes, it is possible to execute multiple SQLite statements in a shell script without using a bash script. Shell scripts are more general-purpose scripts that can be written in various scripting languages, including Bash, Python, Perl, and more.
Here’s an example of executing multiple SQLite statements in a shell script written in Bash:
#!/bin/sh sqlite3 my_database.db <<EOF CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER); INSERT INTO users (name, age) VALUES ('John Doe', 30); INSERT INTO users (name, age) VALUES ('Jane Smith', 25); SELECT * FROM users; EOF
In this example, the script is written in the Bourne shell (sh
) syntax, which is a more portable shell scripting language. The SQLite statements are enclosed within the here-document (<<EOF
) and executed using the sqlite3
command.
While Bash scripts provide additional features and flexibility, it is possible to execute multiple SQLite statements in a shell script using the appropriate syntax for the chosen scripting language.
What are some alternatives to executing multiple SQLite statements in a bash script?
While executing multiple SQLite statements in a bash script is a common approach, there are alternative methods to interact with SQLite databases:
1. SQLite shell: Instead of using a bash script, you can directly use the SQLite shell (sqlite3
) to execute statements interactively or from a file. This provides a convenient way to work with SQLite databases without the need for a separate script.
2. SQLite API: If you are working with a programming language like Python, Java, or C, you can use the SQLite API to execute statements programmatically. These APIs provide a higher level of control and flexibility, allowing you to integrate SQLite functionality directly into your code.
3. SQLite GUI tools: There are various GUI tools available for SQLite that provide a graphical interface to interact with databases. These tools allow you to execute statements, visualize data, and perform database management tasks without the need for scripting.
4. ORMs (Object-Relational Mappers): If you are working with a framework or library that includes an ORM, such as Django or SQLAlchemy in Python, you can leverage the ORM’s functionality to execute SQLite statements. ORMs provide a higher-level abstraction for database operations and can simplify the process of working with SQLite.
The choice of method depends on the specific requirements of your project, the complexity of the tasks you need to perform, and your familiarity with the tools and languages involved.
Are there any best practices or recommended approaches for executing multiple SQLite statements in a bash script?
When executing multiple SQLite statements in a bash script, it is important to follow best practices and recommended approaches to ensure the script’s reliability and maintainability:
1. Use proper error handling: Include error handling mechanisms in your script to handle potential errors that may occur during the execution of SQLite statements. This can help prevent unexpected behavior and provide useful feedback in case of failures.
2. Sanitize input: If your script accepts user input or dynamic values to be used in SQLite statements, make sure to properly sanitize and validate the input to prevent SQL injection attacks or unintended behavior.
3. Use transactions when necessary: If you need to perform a series of SQLite statements as a single transaction, use the BEGIN TRANSACTION
, COMMIT
, and ROLLBACK
statements to ensure data integrity and consistency.
4. Optimize performance: Consider optimizing the performance of your script by using indexes, optimizing queries, and employing other performance-enhancing techniques. This can be particularly important when dealing with large databases or complex operations.
5. Modularize your script: Break down your script into modular functions or sections to improve readability and maintainability. This can make it easier to understand and modify the script in the future.
6. Version control your script: Use a version control system, such as Git, to track changes to your script and collaborate with others. This can help in managing different versions of the script and reverting to previous versions if needed.
7. Document your script: Include comments and documentation within your script to explain its purpose, usage, and any important considerations. This can make it easier for others to understand and use your script.