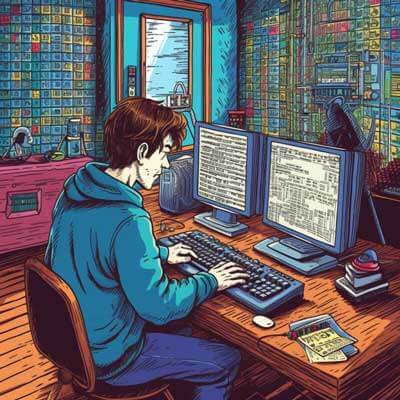
Table of Contents
To post JSON data with Curl in Linux, you can follow the steps below:
Step 1: Install Curl
Before you can use Curl to post JSON data, you need to ensure that it is installed on your Linux system. Most Linux distributions come with Curl pre-installed, but if it is not available, you can install it using your package manager. For example, on Ubuntu, you can run the following command:
sudo apt-get install curl
Related Article: Locating and Moving Files in Bash Scripting on Linux
Step 2: Prepare the JSON Data
To post JSON data with Curl, you first need to prepare the JSON payload that you want to send. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and for machines to parse and generate. You can create the JSON payload using a text editor or by using a programming language that supports JSON serialization, such as Python or JavaScript.
Here's an example of a JSON payload:
{ "name": "John Doe", "email": "johndoe@example.com", "age": 30}
Make sure that your JSON payload is valid and well-formed. You can use online JSON validators to check the validity of your JSON data.
Step 3: Use Curl to Post JSON Data
Once you have prepared the JSON payload, you can use Curl to post it to a server. The basic syntax for posting JSON data with Curl is as follows:
curl -X POST -H "Content-Type: application/json" -d '<json_data>' <url>
Let's break down the different parts of the command:
- -X POST
: Specifies that the HTTP request method is POST.
- -H "Content-Type: application/json"
: Sets the Content-Type
header to application/json
, indicating that the request payload is in JSON format.
- -d '<json_data>'
: Specifies the JSON payload to be sent in the request. Replace <json_data>
with the actual JSON data.
- <url>
: Specifies the URL of the server to which the request should be sent. Replace <url>
with the actual URL.
Here's an example command that posts the JSON payload to a server:
curl -X POST -H "Content-Type: application/json" -d '{ "name": "John Doe", "email": "johndoe@example.com", "age": 30}' https://api.example.com/users
Make sure to replace the URL with the actual server endpoint where you want to send the JSON data.
Step 4: Handle the Response
After sending the JSON data with Curl, the server will process the request and send a response back. To handle the response, you can redirect the output of the Curl command to a file or use additional Curl options to extract specific information from the response.
For example, to save the response to a file named response.json
, you can use the following command:
curl -X POST -H "Content-Type: application/json" -d '{ "name": "John Doe", "email": "johndoe@example.com", "age": 30}' https://api.example.com/users -o response.json
Alternatively, you can use the -i
option to include the response headers in the output:
curl -X POST -H "Content-Type: application/json" -d '{ "name": "John Doe", "email": "johndoe@example.com", "age": 30}' https://api.example.com/users -i
This will display both the response headers and the response body in the output.
Related Article: How to Set LD_LIBRARY_PATH in Linux
Step 5: Best Practices and Tips
- Use proper error handling: When posting JSON data with Curl, make sure to handle any potential errors that may occur during the request. Check the response status code and handle different scenarios accordingly.
- Validate the JSON payload: Before sending the JSON data, validate it to ensure that it is valid and well-formed. This will help prevent issues when parsing the data on the server-side.
- Use HTTPS for secure communication: If you are sending sensitive data in the JSON payload, it is recommended to use HTTPS instead of HTTP to ensure secure communication between the client and the server.
- Use command-line options to customize the request: Curl provides a wide range of options that you can use to customize the request. For example, you can set custom headers, specify authentication credentials, or set request timeouts.
Alternative Approach: Using a File for JSON Data
Instead of specifying the JSON payload directly in the Curl command, you can also use a file to store the JSON data and pass it to Curl using the -d
option. This can be useful when dealing with large or complex JSON payloads.
Here's an example:
1. Create a file named data.json
and store the JSON payload in it:
{ "name": "John Doe", "email": "johndoe@example.com", "age": 30}
2. Use the following Curl command to post the JSON data from the file:
curl -X POST -H "Content-Type: application/json" -d @data.json https://api.example.com/users
This will read the JSON data from the data.json
file and send it as the request payload.
Using a file for the JSON data can be convenient when you have multiple requests or need to reuse the JSON payload in different Curl commands.