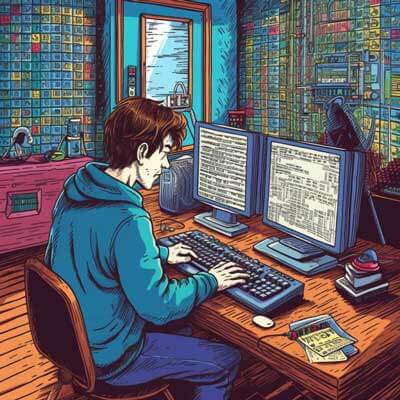
In Python, line break and line continuation are used to split long lines of code into multiple lines for better readability and maintainability. Line breaks are used to split a single line of code into multiple lines, while line continuation is used to continue a statement onto the next line without causing a syntax error.
Implementing Line Break in Python
To implement line breaks in Python, you can make use of backslashes (\) at the end of each line. The backslash tells Python that the line should continue onto the next line. Here’s an example:
print("This is a long line of code \ that spans multiple lines.")
This code will produce the following output:
This is a long line of code that spans multiple lines.
Alternatively, you can also make use of parentheses to implement line breaks. Here’s an example:
print("This is a long line of code " "that spans multiple lines.")
This code will produce the same output as the previous example.
Related Article: How To Limit Floats To Two Decimal Points In Python
Implementing Line Continuation in Python
Line continuation allows you to continue a statement onto the next line without causing a syntax error. It is particularly useful when you have long expressions or function calls that would otherwise exceed the recommended line length limit.
To implement line continuation in Python, you can make use of the backslash (\) character at the end of each line. Here’s an example:
result = 10 + 20 + 30 + \ 40 + 50
This code will assign the value 150 to the variable result
.
Alternatively, you can also make use of parentheses to implement line continuation. Here’s an example:
result = (10 + 20 + 30 + 40 + 50)
This code will produce the same result as the previous example.
Best Practices for Line Break and Line Continuation in Python
When implementing line breaks and line continuation in Python, it is important to follow some best practices to ensure clean and readable code. Here are some best practices to keep in mind:
1. Limit line length: It is recommended to limit the length of each line to 79 characters. This helps improve code readability and avoids horizontal scrolling.
2. Use indentation: When continuing a statement onto the next line, make sure to indent the continued line to clearly indicate that it is a continuation of the previous line.
3. Be consistent: Choose a consistent approach for line breaks and line continuation throughout your codebase. Stick to either using backslashes (\) or parentheses for line breaks and line continuation.
4. Avoid excessive line breaks: While line breaks can improve code readability, excessive use of line breaks can make the code harder to follow. Use line breaks sparingly and only when necessary to improve readability.
5. Use parentheses for complex expressions: When you have complex expressions or function calls that span multiple lines, using parentheses can help improve code readability.
Related Article: How To Rename A File With Python