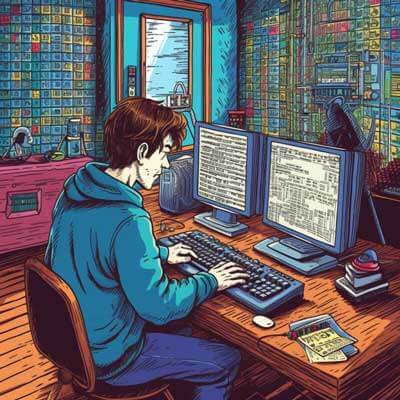
Table of Contents
Introduction to Multiprocessing
The Python Multiprocessing module provides a way to execute multiple processes concurrently, allowing for improved performance and utilization of multiple CPU cores. In this chapter, we will explore the basics of multiprocessing and how it can be used to enhance the execution of Python programs.
Related Article: Python Priority Queue Tutorial
Code Snippet: Creating Processes
import multiprocessing def worker(): print("Worker process") if __name__ == "__main__": process = multiprocessing.Process(target=worker) process.start()
The above code snippet demonstrates how to create a new process using the multiprocessing.Process
class. The target
argument specifies the function to be executed in the new process. By calling the start()
method, the process is launched and the function is executed concurrently.
Code Snippet: Managing Process Execution
import multiprocessing def worker(): print("Worker process") if __name__ == "__main__": processes = [] for _ in range(5): process = multiprocessing.Process(target=worker) processes.append(process) process.start() for process in processes: process.join()
In this code snippet, we create multiple processes and store them in a list. By calling the start()
method for each process, they are executed concurrently. The join()
method is used to wait for all processes to complete before proceeding.
Examining the Multiprocessing Module
The multiprocessing module provides a rich set of features and functionalities for managing and executing processes in Python. In this chapter, we will explore the various components of the multiprocessing module and how they can be utilized to achieve parallelism.
Related Article: Python Bitwise Operators Tutorial
Code Snippet: Inter-Process Communication
import multiprocessing def worker(queue): result = 10 + 20 queue.put(result) if __name__ == "__main__": queue = multiprocessing.Queue() process = multiprocessing.Process(target=worker, args=(queue,)) process.start() process.join() result = queue.get() print("Result:", result)
The code snippet above demonstrates how to perform inter-process communication using a multiprocessing Queue. The Queue
class provides a thread-safe way to exchange data between processes. In this example, the worker
function calculates a result and puts it into the queue, which is then retrieved and printed in the main process.
Code Snippet: Synchronizing Processes
import multiprocessing def worker(lock): with lock: print("Worker process") if __name__ == "__main__": lock = multiprocessing.Lock() process = multiprocessing.Process(target=worker, args=(lock,)) process.start() process.join()
In this code snippet, a multiprocessing Lock is used to synchronize access to a shared resource. The with
statement is used to acquire and release the lock, ensuring that only one process can access the critical section at a time. This helps prevent race conditions and ensures data integrity.
The Theory Behind Multiprocessing
To effectively use the multiprocessing module, it is important to understand the underlying theory and concepts behind multiprocessing. This chapter provides an overview of the theory behind multiprocessing and how it enables parallel execution of code.
Code Snippet: Using a Process Pool
import multiprocessing def worker(number): return number * 2 if __name__ == "__main__": numbers = [1, 2, 3, 4, 5] with multiprocessing.Pool() as pool: results = pool.map(worker, numbers) print("Results:", results)
The above code snippet demonstrates the usage of a process pool to execute a function concurrently on multiple inputs. The Pool
class provides a convenient way to distribute the workload across multiple processes. In this example, the worker
function is applied to each number in the numbers
list using the map
method of the process pool, resulting in a list of results.
Related Article: How to Read Xlsx File Using Pandas Library in Python
Code Snippet: Synchronization Primitives
import multiprocessing def worker(event): event.wait() print("Worker process") if __name__ == "__main__": event = multiprocessing.Event() process = multiprocessing.Process(target=worker, args=(event,)) process.start() event.set() process.join()
In this code snippet, a multiprocessing Event is used to synchronize the execution of multiple processes. The wait
method blocks the process until the event is set, allowing for coordinated execution. By calling the set
method, the event is triggered, allowing the worker process to proceed.
Setting Up Your Environment for Multiprocessing
Before diving into using multiprocessing in Python, it is important to set up your environment correctly. This chapter provides guidance on how to prepare your development environment to effectively utilize multiprocessing.
Code Snippet: Setting the Number of Processes
import multiprocessing if __name__ == "__main__": num_processes = multiprocessing.cpu_count() print("Number of processes:", num_processes)
The above code snippet demonstrates how to determine the number of CPU cores available on the system using the cpu_count
function from the multiprocessing module. This information can be used to optimize the number of processes to be created, ensuring efficient utilization of system resources.
Code Snippet: Specifying Process Start Method
import multiprocessing if __name__ == "__main__": multiprocessing.set_start_method("spawn") # Rest of the code
In this code snippet, the set_start_method
function is used to specify the method by which new processes are created. The "spawn" method is recommended for most platforms as it provides the highest level of isolation and compatibility.
Related Article: Implementing Security Practices in Django
Use Case: Data Analysis with Multiprocessing
The multiprocessing module can greatly accelerate data analysis tasks by distributing the workload across multiple processes. In this chapter, we will explore a use case where multiprocessing is leveraged to perform data analysis tasks efficiently.
Code Snippet: Parallelizing Data Processing
import multiprocessing def process_data(data): # Process data here return processed_data if __name__ == "__main__": data = [...] # Input data with multiprocessing.Pool() as pool: results = pool.map(process_data, data) # Process the results
The code snippet above demonstrates how to parallelize data processing using the Pool
class from the multiprocessing module. The process_data
function is applied to each element in the input data list using the map
method. The results are collected and can be further processed as needed.
Code Snippet: Concurrent Data Aggregation
import multiprocessing def aggregate_data(data): # Aggregate data here return aggregated_data if __name__ == "__main__": data = [...] # Input data with multiprocessing.Pool() as pool: results = pool.map(aggregate_data, data) final_result = merge_results(results) # Process the final result
In this code snippet, the multiprocessing module is used to concurrently perform data aggregation tasks. The aggregate_data
function is applied to each element in the input data list, and the results are collected using the map
method. The final result is obtained by merging the individual results and can be further processed.
Use Case: Web Crawling with Multiprocessing
Web crawling is a computationally intensive task that can benefit greatly from parallel execution. In this chapter, we will explore a use case where multiprocessing is applied to enhance web crawling performance.
Related Article: Intro to Payment Processing in Django Web Apps
Code Snippet: Concurrent URL Retrieval
import multiprocessing import requests def retrieve_url(url): response = requests.get(url) return response.content if __name__ == "__main__": urls = [...] # List of URLs to crawl with multiprocessing.Pool() as pool: results = pool.map(retrieve_url, urls) # Process the results
The above code snippet demonstrates how to use multiprocessing to concurrently retrieve multiple URLs. The retrieve_url
function uses the requests library to fetch the content of each URL. The map
method of the process pool is used to apply the function to each URL, resulting in a list of response contents that can be further processed.
Code Snippet: Parallel Link Extraction
import multiprocessing import requests from bs4 import BeautifulSoup def extract_links(url): response = requests.get(url) soup = BeautifulSoup(response.content, "html.parser") links = [a["href"] for a in soup.find_all("a")] return links if __name__ == "__main__": urls = [...] # List of URLs to crawl with multiprocessing.Pool() as pool: results = pool.map(extract_links, urls) # Process the results
In this code snippet, multiprocessing is used to parallelize the extraction of links from multiple webpages. The extract_links
function fetches the content of each URL, parses it using BeautifulSoup, and extracts all the links. The map
method of the process pool is used to apply the function to each URL, resulting in a list of link lists that can be further processed.
Best Practice: Managing Processes
Effectively managing processes is crucial when working with multiprocessing in Python. This chapter provides best practices for managing processes and ensuring efficient execution.
Code Snippet: Process Termination
import multiprocessing import time def worker(): print("Worker process") time.sleep(5) if __name__ == "__main__": process = multiprocessing.Process(target=worker) process.start() time.sleep(2) process.terminate() process.join()
The above code snippet demonstrates how to terminate a process using the terminate
method. In this example, the worker process is sleeping for 5 seconds. After 2 seconds, the main process terminates the worker process by calling the terminate
method. The join
method is then used to wait for the process to finish.
Related Article: How To Limit Floats To Two Decimal Points In Python
Code Snippet: Process Exit Status
import multiprocessing import time def worker(): print("Worker process") time.sleep(5) if __name__ == "__main__": process = multiprocessing.Process(target=worker) process.start() process.join() exit_code = process.exitcode print("Exit code:", exit_code)
In this code snippet, the exitcode
attribute of a process is used to retrieve its exit status. After the process has finished executing, the join
method is called to wait for its completion. The exit code is then obtained and printed, indicating the status of the process.
Best Practice: Sharing State Between Processes
When working with multiprocessing, it may be necessary to share data between processes. This chapter provides best practices for sharing state between processes and avoiding common pitfalls.
Code Snippet: Shared Memory
import multiprocessing def worker(shared_list): shared_list.append(1) if __name__ == "__main__": manager = multiprocessing.Manager() shared_list = manager.list() processes = [] for _ in range(5): process = multiprocessing.Process(target=worker, args=(shared_list,)) processes.append(process) process.start() for process in processes: process.join() print("Shared list:", shared_list)
The above code snippet demonstrates how to share a list between multiple processes using the Manager
class from the multiprocessing module. The Manager
class provides a way to create shared objects that can be accessed by multiple processes. In this example, a shared list is created and each process appends a value to it. The final contents of the shared list are printed.
Code Snippet: Shared Counter
import multiprocessing def worker(shared_counter): shared_counter.value += 1 if __name__ == "__main__": manager = multiprocessing.Manager() shared_counter = manager.Value("i", 0) processes = [] for _ in range(5): process = multiprocessing.Process(target=worker, args=(shared_counter,)) processes.append(process) process.start() for process in processes: process.join() print("Shared counter:", shared_counter.value)
In this code snippet, a shared counter is implemented using the Value
class from the multiprocessing module. The Value
class allows for the creation of shared variables that can be accessed and modified by multiple processes. Each process increments the counter, and the final value is printed.
Related Article: How to Manage Relative Imports in Python 3
Real World Example: Concurrent Web Scraper
To illustrate the power of multiprocessing, let's explore a real-world example of a concurrent web scraper. This chapter provides a detailed example of how multiprocessing can be used to scrape data from multiple websites simultaneously.
Code Snippet: Concurrent Web Scraping
import multiprocessing import requests from bs4 import BeautifulSoup def scrape_website(url): response = requests.get(url) soup = BeautifulSoup(response.content, "html.parser") # Scrape data from the webpage return scraped_data if __name__ == "__main__": urls = [...] # List of URLs to scrape with multiprocessing.Pool() as pool: results = pool.map(scrape_website, urls) # Process the results
The above code snippet demonstrates how to implement a concurrent web scraper using multiprocessing. The scrape_website
function retrieves the content of each URL, parses it using BeautifulSoup, and extracts the desired data. The map
method of the process pool is used to apply the function to each URL, resulting in a list of scraped data that can be further processed.
Code Snippet: Throttling Requests
import multiprocessing import requests import time from bs4 import BeautifulSoup def scrape_website(url): response = requests.get(url) soup = BeautifulSoup(response.content, "html.parser") # Scrape data from the webpage return scraped_data if __name__ == "__main__": urls = [...] # List of URLs to scrape with multiprocessing.Pool() as pool: results = [] for url in urls: time.sleep(0.5) # Throttle requests result = pool.apply_async(scrape_website, (url,)) results.append(result) scraped_data = [result.get() for result in results] # Process the scraped data
In this code snippet, requests are throttled to avoid overwhelming the web server. The time.sleep
function is used to introduce a delay between requests, allowing for a more controlled rate of scraping. The apply_async
method of the process pool is used to asynchronously apply the scrape_website
function to each URL, and the results are collected and further processed.
Real World Example: Parallel Image Processor
Another practical application of multiprocessing is parallel image processing. This chapter provides a real-world example of how multiprocessing can be used to process images concurrently, enabling faster image manipulation tasks.
Related Article: How to Use Python's Numpy.Linalg.Norm Function
Code Snippet: Concurrent Image Processing
import multiprocessing from PIL import Image def process_image(image_path): image = Image.open(image_path) # Process the image processed_image = image.rotate(90) return processed_image if __name__ == "__main__": image_paths = [...] # List of image file paths with multiprocessing.Pool() as pool: results = pool.map(process_image, image_paths) # Process the results
The above code snippet demonstrates how to utilize multiprocessing to process images concurrently. The process_image
function opens each image file, applies the desired image processing operations, and returns the processed image. The map
method of the process pool is used to apply the function to each image path, resulting in a list of processed images that can be further processed.
Code Snippet: Parallel Thumbnail Generation
import multiprocessing from PIL import Image def generate_thumbnail(image_path, thumbnail_size): image = Image.open(image_path) thumbnail = image.resize(thumbnail_size) return thumbnail if __name__ == "__main__": image_paths = [...] # List of image file paths thumbnail_size = (100, 100) # Desired thumbnail size with multiprocessing.Pool() as pool: results = pool.starmap(generate_thumbnail, [(path, thumbnail_size) for path in image_paths]) # Process the results
In this code snippet, multiprocessing is used to generate thumbnails of multiple images in parallel. The generate_thumbnail
function opens each image file, resizes it to the desired thumbnail size, and returns the thumbnail image. The starmap
method of the process pool is used to apply the function to each image path and thumbnail size pair, resulting in a list of thumbnail images that can be further processed.
Performance Considerations: Process Overhead
While multiprocessing can greatly improve performance, it is important to consider the overhead associated with creating and managing processes. This chapter explores the impact of process overhead on performance and provides insights on how to optimize multiprocessing performance.
Code Snippet: Measuring Process Creation Time
import multiprocessing import time def worker(): time.sleep(1) if __name__ == "__main__": start_time = time.time() processes = [] for _ in range(1000): process = multiprocessing.Process(target=worker) processes.append(process) process.start() for process in processes: process.join() end_time = time.time() total_time = end_time - start_time print("Total time:", total_time)
The above code snippet demonstrates how to measure the time taken to create and execute multiple processes. In this example, 1000 processes are created and started in a loop. The join
method is used to wait for all processes to complete. The total time taken for process creation and execution is then calculated and printed.
Related Article: Python Super Keyword Tutorial
Code Snippet: Using Process Pools
import multiprocessing import time def worker(): time.sleep(1) if __name__ == "__main__": start_time = time.time() with multiprocessing.Pool() as pool: for _ in range(1000): pool.apply_async(worker) pool.close() pool.join() end_time = time.time() total_time = end_time - start_time print("Total time:", total_time)
In this code snippet, the performance impact of process overhead is reduced by using a process pool. Instead of creating and starting individual processes, the apply_async
method of the process pool is used to asynchronously apply the worker
function to the pool. The close
and join
methods are used to manage the processes in the pool and ensure their completion.
Performance Considerations: Inter-Process Communication
Inter-process communication (IPC) is an essential aspect of multiprocessing. However, it can introduce performance overhead. In this chapter, we explore the impact of inter-process communication on performance and discuss strategies for optimizing IPC.
Code Snippet: Measuring IPC Overhead
import multiprocessing import time def worker(queue): for _ in range(100000): queue.put(1) if __name__ == "__main__": queue = multiprocessing.Queue() start_time = time.time() processes = [] for _ in range(4): process = multiprocessing.Process(target=worker, args=(queue,)) processes.append(process) process.start() for process in processes: process.join() end_time = time.time() total_time = end_time - start_time print("Total time:", total_time)
The above code snippet demonstrates how to measure the time taken for inter-process communication using a multiprocessing Queue. In this example, multiple processes are used to put items into the queue. The total time taken for the processes to complete is then calculated and printed.
Code Snippet: Using Shared Memory
import multiprocessing import time def worker(shared_list): for _ in range(100000): shared_list.append(1) if __name__ == "__main__": manager = multiprocessing.Manager() shared_list = manager.list() start_time = time.time() processes = [] for _ in range(4): process = multiprocessing.Process(target=worker, args=(shared_list,)) processes.append(process) process.start() for process in processes: process.join() end_time = time.time() total_time = end_time - start_time print("Total time:", total_time)
In this code snippet, the impact of inter-process communication on performance is reduced by using shared memory. The Manager
class from the multiprocessing module is used to create a shared list that can be accessed and modified by multiple processes. Each process appends items to the shared list, resulting in reduced inter-process communication overhead.
Related Article: Converting Integer Scalar Arrays To Scalar Index In Python
Advanced Technique: Process Pools
Process pools provide a convenient way to manage and distribute the workload across multiple processes. This chapter explores the advanced technique of using process pools to achieve efficient execution and resource utilization.
Code Snippet: Dynamic Workload Distribution
import multiprocessing def worker(number): return number * 2 if __name__ == "__main__": numbers = [1, 2, 3, 4, 5] with multiprocessing.Pool() as pool: results = pool.map(worker, numbers) print("Results:", results)
The above code snippet demonstrates how to use a process pool to distribute the workload dynamically. In this example, the worker
function is applied to each number in the numbers
list using the map
method of the process pool. The workload is automatically divided among the available processes, resulting in efficient workload distribution.
Code Snippet: Limiting the Number of Processes
import multiprocessing def worker(number): return number * 2 if __name__ == "__main__": numbers = [1, 2, 3, 4, 5] with multiprocessing.Pool(processes=2) as pool: results = pool.map(worker, numbers) print("Results:", results)
In this code snippet, the number of processes in the process pool is limited using the processes
argument of the Pool
constructor. By specifying the desired number of processes, the workload is distributed among the specified number of processes, ensuring optimal resource utilization.
Advanced Technique: Synchronization Primitives
Synchronization primitives provide a way to coordinate the execution of multiple processes and ensure data integrity. This chapter explores advanced techniques for using synchronization primitives in multiprocessing.
Related Article: How to Normalize a Numpy Array to a Unit Vector in Python
Code Snippet: Using a Lock
import multiprocessing def worker(lock): with lock: print("Worker process") if __name__ == "__main__": lock = multiprocessing.Lock() with multiprocessing.Pool() as pool: pool.apply(worker, (lock,)) pool.apply(worker, (lock,))
The above code snippet demonstrates how to use a Lock to synchronize access to a shared resource. The with
statement is used to acquire and release the lock, ensuring that only one process can access the critical section at a time. In this example, the worker
function is applied to the process pool twice, and the lock ensures that the print statement is executed by only one process at a time.
Code Snippet: Using a Semaphore
import multiprocessing def worker(semaphore): with semaphore: print("Worker process") if __name__ == "__main__": semaphore = multiprocessing.Semaphore(2) with multiprocessing.Pool() as pool: pool.apply(worker, (semaphore,)) pool.apply(worker, (semaphore,)) pool.apply(worker, (semaphore,))
In this code snippet, a Semaphore is used to limit the number of processes that can access a shared resource simultaneously. The Semaphore
class allows for the initialization of a semaphore with a specified value. In this example, the semaphore is initialized with a value of 2, allowing up to 2 processes to enter the critical section simultaneously.
Code Snippet: Creating Processes
import multiprocessing def worker(): print("Worker process") if __name__ == "__main__": process = multiprocessing.Process(target=worker) process.start()
Code Snippet: Managing Process Execution
import multiprocessing def worker(): print("Worker process") if __name__ == "__main__": processes = [] for _ in range(5): process = multiprocessing.Process(target=worker) processes.append(process) process.start() for process in processes: process.join()
Related Article: How to Work with Encoding & Multiple Languages in Django
Code Snippet: Inter-Process Communication
import multiprocessing def worker(queue): result = 10 + 20 queue.put(result) if __name__ == "__main__": queue = multiprocessing.Queue() process = multiprocessing.Process(target=worker, args=(queue,)) process.start() process.join() result = queue.get() print("Result:", result)
Code Snippet: Synchronizing Processes
import multiprocessing def worker(lock): with lock: print("Worker process") if __name__ == "__main__": lock = multiprocessing.Lock() process = multiprocessing.Process(target=worker, args=(lock,)) process.start() process.join()
Code Snippet: Using a Process Pool
import multiprocessing def worker(number): return number * 2 if __name__ == "__main__": numbers = [1, 2, 3, 4, 5] with multiprocessing.Pool() as pool: results = pool.map(worker, numbers) print("Results:", results)
Error Handling: Common Pitfalls
When working with multiprocessing, it is important to be aware of common pitfalls and errors that can occur. This chapter highlights some common pitfalls and provides guidance on how to avoid them.
Related Article: How to Use Global Variables in a Python Function
Code Snippet: Handling Exceptions in Processes
import multiprocessing def worker(): raise Exception("Error in worker process") if __name__ == "__main__": try: process = multiprocessing.Process(target=worker) process.start() process.join() except Exception as e: print("Exception:", e)
The above code snippet demonstrates how to handle exceptions raised in processes. In this example, the worker process raises an exception. By wrapping the process creation and execution code in a try-except block, the exception can be caught and handled appropriately.
Code Snippet: Preventing Deadlocks
import multiprocessing def worker(lock1, lock2): with lock1: print("Worker process acquired lock1") with lock2: print("Worker process acquired lock2") if __name__ == "__main__": lock1 = multiprocessing.Lock() lock2 = multiprocessing.Lock() process1 = multiprocessing.Process(target=worker, args=(lock1, lock2)) process2 = multiprocessing.Process(target=worker, args=(lock2, lock1)) process1.start() process2.start() process1.join() process2.join()
In this code snippet, two processes attempt to acquire multiple locks in different orders, leading to a deadlock. The program will hang indefinitely because each process is waiting for a lock that the other process holds. To prevent deadlocks, it is important to carefully manage the order in which locks are acquired.
Error Handling: Debugging Techniques
When working with multiprocessing, debugging can be challenging due to the concurrent nature of processes. This chapter provides debugging techniques and strategies for effectively identifying and resolving issues in multiprocessing code.
Code Snippet: Printing Debug Information
import multiprocessing def worker(): print("Worker process") if __name__ == "__main__": multiprocessing.log_to_stderr() logger = multiprocessing.get_logger() logger.setLevel(multiprocessing.SUBDEBUG) process = multiprocessing.Process(target=worker) process.start() process.join()
The above code snippet demonstrates how to print debug information from multiprocessing code. By calling log_to_stderr
and setting the logger level to SUBDEBUG
, detailed debug information will be printed to the console. This can help identify issues and understand the execution flow of the multiprocessing code.
Related Article: How to Detect Duplicates in a Python List
Code Snippet: Using Logging
import multiprocessing import logging def worker(): logging.info("Worker process") if __name__ == "__main__": multiprocessing.log_to_stderr() logger = multiprocessing.get_logger() logger.setLevel(logging.INFO) process = multiprocessing.Process(target=worker) process.start() process.join()
In this code snippet, the logging module is used to log information from multiprocessing code. By configuring the logger to an appropriate level, log messages can be used to track the execution flow and identify issues. The log messages can be directed to various outputs, such as the console or a log file, depending on the logging configuration.