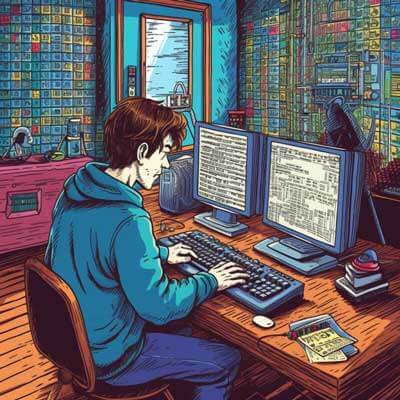
Table of Contents
Introduction
Parsing YAML files is a common task in Python when working with configuration files, data serialization, or any other situation where data needs to be stored and retrieved in a human-readable format. YAML (YAML Ain't Markup Language) is a popular data serialization language that is easy to read and write. In this guide, we will explore different methods to parse YAML files in Python.
Related Article: How to Create Multiline Comments in Python
Option 1: Using the PyYAML Library
One of the most popular libraries for parsing YAML files in Python is PyYAML. PyYAML is a YAML parser and emitter for Python, which allows you to easily load and dump YAML data. Follow the steps below to parse a YAML file using PyYAML:
1. Install the PyYAML library by running the following command:
pip install pyyaml
2. Import the yaml
module in your Python script:
import yaml
3. Use the yaml.load()
function to parse the YAML file and load its contents into a Python data structure. Here's an example:
with open('config.yaml', 'r') as file: data = yaml.load(file, Loader=yaml.FullLoader) # Access the YAML data print(data)
In the above example, we open the YAML file using the open() function and then pass it to the yaml.load()
function along with the Loader=yaml.FullLoader
argument. This argument ensures that the YAML file is loaded as a Python dictionary or list, rather than a custom object.
4. You can now access the parsed YAML data as a Python dictionary or list.
Option 2: Using the ruamel.yaml Library
Another option for parsing YAML files in Python is to use the ruamel.yaml library. ruamel.yaml is a YAML parser/emitter that is compatible with both YAML 1.1 and 1.2 specifications. Here's how you can parse a YAML file using ruamel.yaml:
1. Install the ruamel.yaml library by running the following command:
pip install ruamel.yaml
2. Import the necessary modules in your Python script:
import ruamel.yaml from ruamel.yaml import YAML
3. Create an instance of the YAML class:
yaml = YAML()
4. Use the yaml.load()
method to parse the YAML file and load its contents into a Python data structure. Here's an example:
with open('config.yaml', 'r') as file: data = yaml.load(file) # Access the YAML data print(data)
In the above example, we open the YAML file using the open() function and then pass it to the yaml.load()
method. The parsed YAML data is automatically converted to a Python dictionary or list.
5. You can now access the parsed YAML data as a Python dictionary or list.
Best Practices
When parsing YAML files in Python, it is important to follow some best practices to ensure the integrity and security of your application:
1. Always use a trusted YAML parsing library like PyYAML or ruamel.yaml. These libraries have been extensively tested and are widely used in the Python community.
2. Avoid using the yaml.load()
function without specifying the Loader
argument. This can lead to potential security vulnerabilities, as arbitrary code execution is possible if the YAML file contains malicious data.
3. Validate the YAML file before parsing it. Use a YAML linter or validator to check the syntax and structure of the file. This can help catch errors or inconsistencies before they cause issues in your application.
4. Handle errors gracefully when parsing YAML files. Use try-except blocks to catch any exceptions that may occur during the parsing process and handle them appropriately.