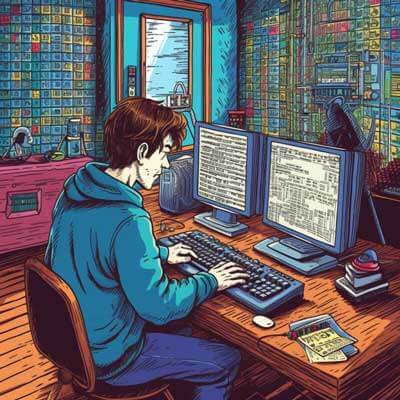
Table of Contents
To download a file over HTTP in Python, you can use the requests
library. Here are two possible ways to accomplish this:
Method 1: Using the requests
library
You can use the get()
function from the requests
library to download a file from a URL. Here's an example:
import requests url = "https://example.com/file.zip" response = requests.get(url) if response.status_code == 200: with open("file.zip", "wb") as file: file.write(response.content) print("File downloaded successfully!") else: print("Failed to download the file.")
In the above code snippet, we first import the requests
library. Then, we define the URL of the file we want to download. We use the get()
function from the requests
library to send a GET request to the specified URL. If the response status code is 200 (indicating a successful request), we open a file in binary write mode and write the content of the response to the file.
Related Article: How to Parallelize a Simple Python Loop
Method 2: Using the urllib
module
Another way to download a file over HTTP in Python is by using the urllib
module. Here's an example:
import urllib.request url = "https://example.com/file.zip" urllib.request.urlretrieve(url, "file.zip") print("File downloaded successfully!")
In the above code snippet, we import the urllib.request
module. We then use the urlretrieve()
function from the urllib.request
module to download the file from the specified URL and save it with the specified filename.
Both methods are commonly used to download files over HTTP in Python. The choice between them depends on your specific requirements and coding style.
Best Practices and Suggestions
Related Article: How to Use Python Super With Init Methods
When downloading files over HTTP in Python, consider the following best practices:
1. Error Handling: Always check the response status code to ensure the request was successful before proceeding with saving the file. Handle any potential errors or exceptions that may occur during the download process.
2. File Validation: If the file you are downloading is critical to your application or system, consider implementing additional checks to validate the integrity and authenticity of the file. You can calculate checksums or verify digital signatures to ensure the file has not been tampered with.
3. File Size and Progress: If you are dealing with large files, it may be beneficial to display the progress of the download or implement a mechanism to download the file in chunks. This can help prevent timeouts and provide a better user experience.
4. Security Considerations: Be cautious when downloading files from untrusted sources. Validate the URL and ensure it is from a trusted and secure source before initiating the download. Additionally, consider using HTTPS instead of HTTP for secure file transfers.
5. Performance Optimization: If you need to download multiple files or perform concurrent downloads, you can leverage asynchronous programming techniques or utilize libraries like aiohttp
to improve performance.
Overall, downloading files over HTTP in Python is a straightforward process using either the requests
library or the urllib
module. Choose the method that best suits your needs and consider implementing best practices to ensure a reliable and secure file download process.