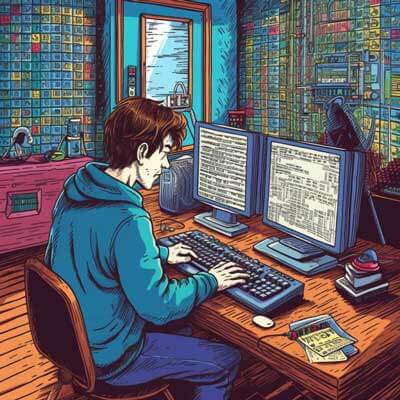
To prettyprint a JSON file in Python, you can use the built-in json
module. This module provides functions for working with JSON data, including parsing JSON strings into Python objects and serializing Python objects into JSON strings. The json
module also provides the dump() and dumps() functions for prettyprinting JSON data.
Using the dump() function to prettyprint a JSON file
The dump()
function from the json
module allows you to write JSON data to a file while prettyprinting it. Here is an example of how to use dump()
to prettyprint a JSON file:
import json # JSON data data = { "name": "John Doe", "age": 30, "city": "New York" } # Open a file for writing with open("data.json", "w") as file: # <a href="https://www.squash.io/how-to-convert-json-to-csv-in-python/">Write JSON</a> data to the file with indentation json.dump(data, file, indent=4)
In this example, we create a dictionary data
representing JSON data. We then open a file named data.json
in write mode using the open()
function. We pass the file object to the dump()
function along with the JSON data and specify the indent
parameter as 4
to indicate the desired indentation level. The dump()
function writes the prettyprinted JSON data to the file.
Related Article: How to Execute a Program or System Command in Python
Using the dumps() function to prettyprint a JSON file
If you don’t need to write the prettyprinted JSON data to a file and just want to obtain a string representation, you can use the dumps()
function. Here is an example:
import json # JSON data data = { "name": "John Doe", "age": 30, "city": "New York" } # Get the prettyprinted JSON string pretty_json = json.dumps(data, indent=4) # Print the prettyprinted JSON string print(pretty_json)
In this example, we use the dumps()
function to convert the data
dictionary into a prettyprinted JSON string. We specify the indent
parameter as 4
to indicate the desired indentation level. The resulting JSON string is assigned to the variable pretty_json
, which can be used or printed as needed.
Alternative ideas
If you prefer a third-party library, you can use the pprint
module from the pprint package. The pprint
module provides a pprint()
function that can be used to prettyprint JSON data. Here is an example:
from pprint import pprint # JSON data data = { "name": "John Doe", "age": 30, "city": "New York" } # Prettyprint the JSON data pprint(data, indent=4)
In this example, we import the pprint
function from the pprint
module. We then pass the data
dictionary to the pprint()
function along with the indent
parameter set to 4
. The pprint()
function prettyprints the JSON data to the console.
Best practices
When prettyprinting JSON data, it is common to use an indentation level of 4 spaces. This indentation level helps improve readability by visually separating nested elements. It is also important to ensure that the JSON data is properly formatted before attempting to prettyprint it. Invalid JSON data may result in errors or unexpected output.
Related Article: How to Use Python with Multiple Languages (Locale Guide)