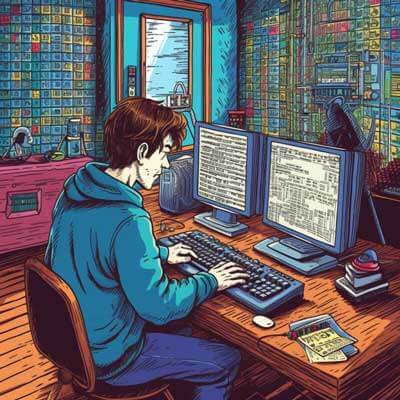
Table of Contents
In Python, the pickle.load
function is a useful tool for saving and loading objects. It allows you to serialize and deserialize Python objects, which means you can convert complex data structures into a byte stream that can be easily stored or transmitted and then converted back into objects when needed.
Step 1: Import the pickle module
To use the pickle.load
function, you first need to import the pickle
module. This module provides the necessary functions for serializing and deserializing Python objects.
import pickle
Related Article: How To Check If Key Exists In Python Dictionary
Step 2: Save an object to a file
Once you have imported the pickle
module, you can start saving objects to a file. The pickle.dump
function is used to serialize an object and save it to a file.
# Create an object to save my_object = {'name': 'John', 'age': 30} # Open a file in write mode with open('my_object.pickle', 'wb') as file: # Serialize and save the object to the file pickle.dump(my_object, file)
In the example above, we create a dictionary object called my_object
with some sample data. We then open a file called my_object.pickle
in write mode using the open
function. Finally, we use the pickle.dump
function to serialize and save the my_object
to the file.
Step 3: Load an object from a file
After saving an object to a file, you can later load it back into memory using the pickle.load
function. This function reads a serialized object from a file and converts it back into its original form.
# Open the file in read mode with open('my_object.pickle', 'rb') as file: # Deserialize and load the object from the file loaded_object = pickle.load(file) # Print the loaded object print(loaded_object)
In the example above, we open the my_object.pickle
file in read mode using the open
function. We then use the pickle.load
function to deserialize and load the object from the file. Finally, we print the loaded object, which should be the same as the original my_object
we saved.
Step 4: Handle exceptions
When working with the pickle.load
function, it's important to handle exceptions that may occur. For example, if the file you are trying to load from does not exist or if it contains invalid data, an exception will be raised.
try: with open('my_object.pickle', 'rb') as file: loaded_object = pickle.load(file) print(loaded_object) except FileNotFoundError: print("File not found") except pickle.UnpicklingError: print("Invalid data in file")
In the example above, we use a try-except
block to catch and handle potential exceptions. If the FileNotFoundError
exception is raised, it means that the file does not exist. If the pickle.UnpicklingError
exception is raised, it means that the file contains invalid data that cannot be deserialized.
Related Article: Working with Numpy Concatenate
Step 5: Best practices and considerations
When using the pickle.load
function, there are a few best practices and considerations to keep in mind:
- Only load objects from trusted sources: The
pickle.load
function can execute arbitrary code when deserializing an object, so it's important to only load objects from trusted sources to avoid potential security risks. - Use a binary file format: When saving objects to a file, it's recommended to use a binary file format (e.g.,
.pickle
) rather than a text file format. This ensures that the serialized data is not altered or corrupted when writing or reading the file. - Handle version compatibility: If you plan to load serialized objects in the future, make sure to handle version compatibility. If the structure of your objects changes between different versions of your code, you may encounter errors when trying to load older serialized objects.
- Consider alternative serialization libraries: While the
pickle
module is useful and convenient, it may not always be the most efficient or secure option for serializing objects. Depending on your specific requirements, you may want to consider alternative libraries like MessagePack or Apache Avro.