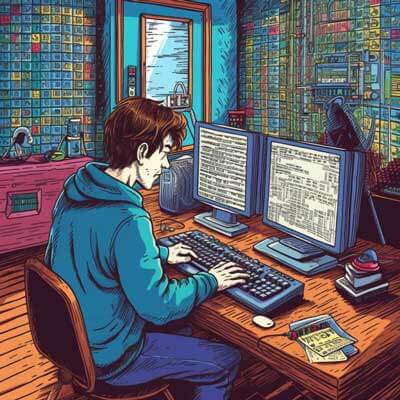
To execute a curl command using Python, you can utilize the requests
library, which is a popular HTTP library for Python. The requests
library provides a simple and intuitive way to send HTTP requests and handle responses. This section will guide you through the process of executing a curl command using Python.
Step 1: Install the requests library
Before you can use the requests
library, you need to install it. You can install the requests
library using pip
, the package installer for Python. Open your terminal or command prompt and run the following command:
pip install requests
Related Article: How to Execute a Program or System Command in Python
Step 2: Import the requests library
Once you have installed the requests
library, you need to import it into your Python script. You can do this by adding the following line at the top of your script:
import requests
Step 3: Execute the curl command
To execute a curl command using Python, you need to translate the curl command into a Python code snippet using the requests
library. The basic structure of the code snippet is as follows:
import requests response = requests.('url', )
Replace with the appropriate HTTP method for your curl command, such as
get
, post
, put
, delete
, etc. Replace 'url'
with the URL you want to send the request to. You can also include optional parameters, such as headers, query parameters, or request body, depending on your curl command.
Here are a few examples to help you understand how to execute different types of curl commands using Python:
Example 1: GET request
If your curl command is a GET request without any parameters, you can execute it in Python as follows:
import requests response = requests.get('https://api.example.com/users') print(response.text)
This code snippet sends a GET request to the specified URL and prints the response content.
Example 2: POST request with headers and JSON payload
If your curl command is a POST request with headers and a JSON payload, you can execute it in Python as follows:
import requests import json headers = { 'Content-Type': 'application/json', 'Authorization': 'Bearer ' } payload = { 'name': 'John Doe', 'email': 'john.doe@example.com' } response = requests.post('https://api.example.com/users', headers=headers, data=json.dumps(payload)) print(response.json())
This code snippet sends a POST request to the specified URL with the headers and JSON payload specified. The response is printed as a JSON object.
Example 3: PUT request with query parameters
If your curl command is a PUT request with query parameters, you can execute it in Python as follows:
import requests params = { 'id': 123, 'name': 'John Doe' } response = requests.put('https://api.example.com/users', params=params) print(response.status_code)
This code snippet sends a PUT request to the specified URL with the query parameters specified. The response status code is printed.
Step 4: Handle the response
After executing the curl command using Python, you can handle the response according to your requirements. The response
object returned by the requests
library provides various attributes and methods to access and manipulate the response data.
Here are a few common tasks you might perform with the response:
– Access the response content: You can access the response content using the response.text
attribute. For example, print(response.text)
prints the response content.
– Access the response headers: You can access the response headers using the response.headers
attribute. For example, print(response.headers)
prints the response headers.
– Check the response status code: You can check the response status code using the response.status_code
attribute. For example, print(response.status_code)
prints the response status code.
– Parse the response as JSON: If the response content is in JSON format, you can parse it as a JSON object using the response.json()
method. For example, data = response.json()
parses the response content as a JSON object and assigns it to the data
variable.
Related Article: How to Use Python with Multiple Languages (Locale Guide)
Step 5: Error handling
When executing curl commands using Python, it’s important to handle errors and exceptions that may occur during the request. The requests
library provides built-in error handling capabilities.
Here’s an example of how you can handle errors when executing a curl command using Python:
import requests try: response = requests.get('https://api.example.com/users') response.raise_for_status() # Raises an exception if the request was unsuccessful print(response.text) except requests.exceptions.HTTPError as e: print('HTTP error occurred:', e) except requests.exceptions.RequestException as e: print('An error occurred:', e)
In this example, the try
block attempts to execute the curl command using the requests
library. If the request is unsuccessful (e.g., returns a non-2xx status code), an exception is raised. The except
blocks catch and handle different types of exceptions that may occur.
Step 6: Other libraries and alternatives
While the requests
library is a popular choice for executing curl commands using Python, there are other libraries and alternatives available as well. Some of these alternatives include:
– urllib
: The urllib
module is a built-in Python library that provides functions for making HTTP requests. It is part of the Python Standard Library and does not require any additional installation.
– http.client
: The http.client
module is another built-in Python library that provides a low-level interface for making HTTP requests. It is part of the Python Standard Library and does not require any additional installation.
– curlify
: The curlify
library is a Python library that allows you to convert Python requests into curl commands. It provides a convenient way to generate curl commands from Python code.
– subprocess
: The subprocess
module is a built-in Python library that allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. It can be used to execute curl commands using the system’s curl executable.
Consider exploring these alternatives if they better suit your specific requirements or preferences.