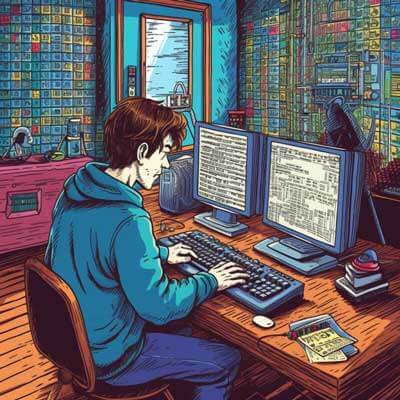
Table of Contents
To measure the elapsed time in Python, you can use the built-in time
module or the more advanced datetime
module. Both modules provide functions and classes to work with time-related operations in Python. In this answer, we will explore both approaches and provide examples of how to measure elapsed time using each method.
Using the time module
The time
module in Python provides a straightforward way to measure elapsed time. It includes a function called time()
that returns the current time in seconds since the epoch (January 1, 1970). By calling this function at the beginning and end of a section of code, you can calculate the time difference and determine the elapsed time.
Here's an example that demonstrates how to measure elapsed time using the time
module:
import time # Start the timer start_time = time.time() # Perform some time-consuming operation # Replace this with your actual code time.sleep(2) # Calculate the elapsed time elapsed_time = time.time() - start_time # Print the elapsed time print("Elapsed time:", elapsed_time, "seconds")
In this example, we import the time
module and use the time()
function to get the current time at the beginning and end of the code block we want to measure. We then calculate the difference between the two times to get the elapsed time in seconds.
Related Article: How To Check If a File Exists In Python
Using the datetime module
The datetime
module in Python provides more advanced functionality for working with dates and times. It includes a class called datetime
that represents a point in time with microsecond precision. By creating datetime
objects at the beginning and end of a section of code, you can calculate the time difference and determine the elapsed time.
Here's an example that demonstrates how to measure elapsed time using the datetime
module:
from datetime import datetime # Start the timer start_time = datetime.now() # Perform some time-consuming operation # Replace this with your actual code time.sleep(2) # Calculate the elapsed time elapsed_time = datetime.now() - start_time # Print the elapsed time print("Elapsed time:", elapsed_time.total_seconds(), "seconds")
In this example, we import the datetime
class from the datetime
module and create datetime
objects at the beginning and end of the code block we want to measure. We then subtract the start time from the end time to get a timedelta
object representing the time difference. Finally, we use the total_seconds()
method of the timedelta
object to get the elapsed time in seconds.
Alternative ideas for measuring elapsed time
There are also other ways to measure elapsed time in Python, depending on your specific use case. Here are a few alternative ideas:
1. Using the timeit
module: The timeit
module provides a convenient way to measure the execution time of small code snippets. It allows you to run a statement or function multiple times and returns the average execution time. This can be useful for benchmarking and performance testing.
2. Using a performance profiling tool: Python provides various performance profiling tools, such as cProfile
and line_profiler
, which can be used to measure the execution time of different parts of your code. These tools provide detailed information on the time spent in each function or line of code, helping you identify bottlenecks and optimize your code.
3. Using a dedicated library: There are several third-party libraries available that specialize in measuring elapsed time and providing advanced timing functionality. One popular library is perf_counter
, which provides a high-resolution timer with better accuracy than the standard time
module.
Best practices for measuring elapsed time
When measuring elapsed time in Python, keep the following best practices in mind:
1. Measure the specific section of code you are interested in: Instead of measuring the entire program execution time, focus on the specific section of code that you want to optimize or benchmark. This will give you more accurate and relevant timing information.
2. Repeat the measurement multiple times: To get a more accurate measurement, it's often a good idea to repeat the timing operation multiple times and take the average. This helps smooth out any variations in system load or other external factors that may affect the timing results.
3. Use appropriate timing functions: Depending on your specific use case, choose the appropriate timing function or method. The time
module is suitable for basic timing needs, while the datetime
module provides more advanced functionality. If you need high-resolution timing or more advanced features, consider using a dedicated library like perf_counter
.
4. Consider the overhead of the timing operation: Keep in mind that the act of measuring elapsed time itself takes some time. If the section of code you are measuring is very fast, the overhead of the timing operation may affect the measurement accuracy. In such cases, consider using a dedicated profiling tool or a library with lower overhead.