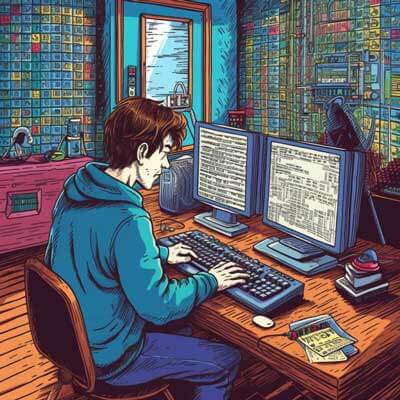
The subprocess module in Python provides a way to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. The subprocess.Popen
class is a useful tool that allows you to create and interact with subprocesses in Python. In this answer, we will explore how to utilize subprocess.Popen
effectively.
1. Importing the subprocess module
Before we can use subprocess.Popen
, we need to import the subprocess
module in our Python script. This can be done with the following line of code:
import subprocess
Related Article: How to Execute a Program or System Command in Python
2. Creating a subprocess with Popen
To create a new subprocess using subprocess.Popen
, we need to provide the command we want to run as a list of strings. Each element of the list represents a separate command-line argument. For example, to run the command ls -l
in the shell, we can use the following code:
import subprocess # Run the 'ls -l' command process = subprocess.Popen(['ls', '-l'])
This code creates a new subprocess that runs the ls -l
command. The process
variable now holds a handle to the subprocess, allowing us to interact with it further.
3. Communicating with the subprocess
Once we have created a subprocess using subprocess.Popen
, we can communicate with it by reading from its standard output and error streams and writing to its standard input stream. The subprocess.Popen
class provides several methods to facilitate this communication.
To read from the subprocess’s standard output or error stream, we can use the communicate
method. This method waits for the subprocess to complete and returns a tuple containing the output and error streams. Here’s an example:
import subprocess # Run the 'ls -l' command process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE) # Read the output and error streams output, error = process.communicate() # Print the output print(output.decode())
In this code, we redirect the standard output and error streams of the subprocess to subprocess.PIPE
, which allows us to capture them. The communicate
method then waits for the subprocess to complete and returns the captured output and error streams. We can use the decode
method to convert the byte strings to regular strings before printing them.
To write to the subprocess’s standard input stream, we can use the stdin
attribute of the subprocess.Popen
object. Here’s an example:
import subprocess # Run the 'grep' command process = subprocess.Popen(['grep', 'example'], stdin=subprocess.PIPE) # Write to the subprocess's standard input process.stdin.write(b'some example input\n') # Close the input stream process.stdin.close()
In this code, we create a subprocess that runs the grep
command. We then write to the subprocess’s standard input stream using the stdin.write
method. Note that we need to pass bytes as input, so we prefix the input string with b
. Finally, we close the input stream to indicate that we have finished writing.
4. Handling the subprocess’s return code
The return code of a subprocess indicates whether it completed successfully or encountered an error. A return code of 0
usually indicates success, while any other value indicates an error. We can obtain the return code of a subprocess by accessing the returncode
attribute of the subprocess.Popen
object.
Here’s an example that demonstrates how to handle the return code:
import subprocess # Run the 'ls' command process = subprocess.Popen(['ls']) # Wait for the subprocess to complete process.wait() # Check the return code if process.returncode == 0: print('Subprocess completed successfully') else: print(f'Subprocess failed with return code {process.returncode}')
In this code, we create a subprocess that runs the ls
command. We then use the wait
method to wait for the subprocess to complete. Finally, we check the return code of the subprocess and print an appropriate message based on the result.
Related Article: How to Use Python with Multiple Languages (Locale Guide)
5. Handling errors and exceptions
When working with subprocesses, it’s important to handle errors and exceptions properly. The subprocess.Popen
class raises various exceptions in different situations, such as when the command to be executed does not exist or when the subprocess encounters an error during execution.
To handle these exceptions, you can use a try-except block. Here’s an example:
import subprocess try: # Run the 'nonexistent-command' command process = subprocess.Popen(['nonexistent-command']) process.wait() except subprocess.CalledProcessError as e: print(f'Subprocess failed with return code {e.returncode}') except FileNotFoundError: print('Command not found')
In this code, we attempt to run a command that does not exist (nonexistent-command
). If the command is not found, the FileNotFoundError
exception is raised. If the command is found but encounters an error during execution, the subprocess.CalledProcessError
exception is raised. We catch these exceptions and handle them accordingly.
6. Security considerations
When using subprocess.Popen
, it’s important to be aware of security considerations. In particular, you should avoid passing user-supplied input directly to the subprocess.Popen
constructor without proper validation and sanitization. User-supplied input can potentially be used to execute arbitrary commands, leading to security vulnerabilities such as command injection.
To mitigate this risk, you should always validate and sanitize user input before using it in subprocess commands. One way to do this is by using functions like subprocess.list2cmdline
to properly escape and quote command-line arguments. Additionally, consider using input validation techniques such as regular expressions or whitelisting to ensure that user input adheres to expected patterns.