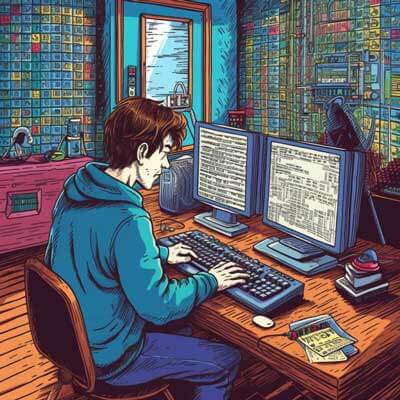
Creating a standalone Python executable allows you to distribute your Python applications to users who do not have Python installed on their systems. In this guide, we will explore two different methods to achieve this.
Method 1: pyInstaller
pyInstaller is a popular Python library that converts Python scripts into standalone executables. It is compatible with Windows, macOS, and Linux.
Here are the steps to create a standalone Python executable using pyInstaller:
1. Install pyInstaller by running the following command:
pip install pyinstaller
2. Navigate to the directory where your Python script is located using the command line.
3. Run the following command to generate the standalone executable:
pyinstaller your_script.py
Replace your_script.py
with the name of your Python script.
4. pyInstaller will generate the standalone executable in a new dist
directory within your current directory.
Note: pyInstaller may generate additional files and directories along with the executable. These files are necessary for the executable to function properly and should not be deleted or modified.
Related Article: How to Execute a Program or System Command in Python
Method 2: cx_Freeze
cx_Freeze is another popular Python library that can be used to create standalone executables. It supports Windows, macOS, and Linux as well.
Follow these steps to create a standalone Python executable using cx_Freeze:
1. Install cx_Freeze by running the following command:
pip install cx_Freeze
2. Create a setup script file (e.g., setup.py
) in the same directory as your Python script. Open the file in a text editor and add the following code:
import sys from cx_Freeze import setup, Executable base = None if sys.platform == "win32": base = "Win32GUI" # Use this option for GUI applications setup( name="YourApplication", version="1.0", description="Description of your application", executables=[Executable("your_script.py", base=base)] )
Replace YourApplication
with the name of your application, 1.0
with the version number, Description of your application
with a brief description, and your_script.py
with the name of your Python script.
3. Open the command line and navigate to the directory where your setup script (setup.py
) is located.
4. Run the following command to generate the standalone executable:
python setup.py build
cx_Freeze will generate the standalone executable in a new build
directory within your current directory.
Best Practices
– Before creating a standalone executable, make sure your Python script runs correctly on your own system.
– Consider including any external dependencies required by your script within the executable. This can be achieved using pyInstaller or cx_Freeze’s options for including additional files and directories.
– Test the standalone executable on different platforms to ensure compatibility.
– Keep your Python script and the standalone executable in separate directories to avoid confusion.
– Update your standalone executable whenever you make changes to your Python script to ensure users have the latest version.
Creating a standalone Python executable allows you to distribute your Python applications easily. Whether you choose pyInstaller or cx_Freeze, both methods provide a convenient way to package your Python scripts into standalone executables for different platforms.
Related Article: How to Use Python with Multiple Languages (Locale Guide)