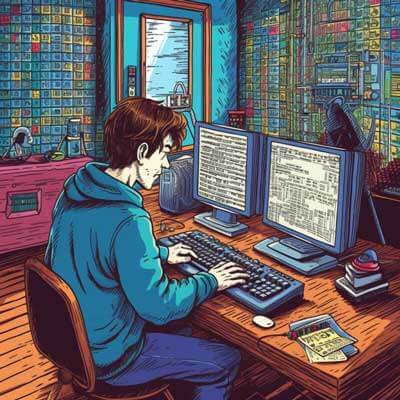
Table of Contents
To get the logical XOR of two variables in Python, you can use the "^" operator. The XOR operation returns True if one and only one of the operands is true, and False otherwise. Here are two possible ways to perform the XOR operation in Python:
Using the "^" Operator
You can use the "^" operator to perform the logical XOR operation between two variables. Here's an example:
a = True b = False result = a ^ b print(result) # Output: True
In this example, the variable "a" is assigned the value True and the variable "b" is assigned the value False. The "^" operator is then used to perform the XOR operation between the two variables, and the result is assigned to the variable "result". Finally, the result is printed, which in this case is True.
Related Article: Handling Large Volumes of Data in FastAPI
Using the "!=" Operator
Another way to get the logical XOR of two variables is by using the "!=" operator. The "!=" operator returns True if the operands are not equal, and False otherwise. By using this operator twice, you can perform the XOR operation. Here's an example:
a = True b = False result = (a != b) print(result) # Output: True
In this example, the "!=" operator is used to compare the values of variables "a" and "b". Since "a" is True and "b" is False, the result of the comparison is True. The variable "result" is then assigned the value of the comparison, which in this case is True. Finally, the result is printed.
Best Practices
When performing the XOR operation in Python, it is important to keep the following best practices in mind:
1. Use meaningful variable names: Choose variable names that accurately represent the values they hold. This will make your code more readable and maintainable.
2. Comment your code: Add comments to explain the purpose of the XOR operation and any other important details. This will make it easier for other developers (including yourself) to understand the code in the future.
3. Use parentheses for clarity: When performing complex XOR operations, it is a good practice to use parentheses to make the order of operations clear. This will help prevent any confusion or unintended results.
a = True b = False c = True result = (a ^ b) ^ c print(result) # Output: False
In this example, parentheses are used to group the XOR operations. The XOR operation between "a" and "b" is performed first, and then the result is XORed with "c". The final result is False.
Alternative Ideas
While the "^" operator and the "!=" operator are commonly used to perform the XOR operation in Python, there are alternative approaches you can consider:
1. Using the "not" operator: You can use the "not" operator to invert the result of a logical operation, and then use the "and" operator to combine the inverted results. Here's an example:
a = True b = False result = (a and not b) or (not a and b) print(result) # Output: True
In this example, the "not" operator is used to invert the values of "a" and "b". Then, the "and" operator is used to combine the inverted results. The final result is True.
2. Using the "int" function: You can convert the boolean values to integers (0 for False and 1 for True) and then use the bitwise XOR operator "&". Here's an example:
a = True b = False result = bool(int(a) ^ int(b)) print(result) # Output: True
In this example, the "int" function is used to convert the boolean values to integers. The "^" operator is then used to perform the bitwise XOR operation between the integers. Finally, the result is converted back to a boolean value using the "bool" function.
These alternative ideas can be useful in certain scenarios where you need to perform the XOR operation in a different way or if you prefer a different coding style. However, the "^" operator and the "!=" operator are widely recognized and commonly used for performing the logical XOR operation in Python.