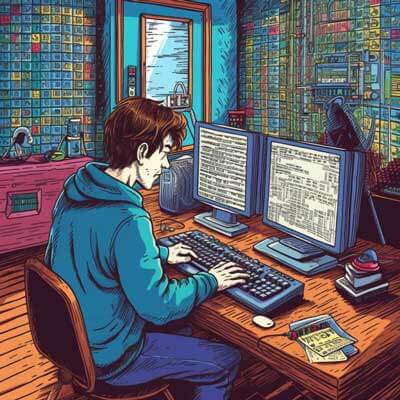
To match a space in regular expressions (regex) using Python, you can use the \s
escape sequence. The \s
pattern matches any whitespace character, including spaces, tabs, and newlines. This allows you to find and manipulate spaces in strings using regex patterns.
Here are two possible approaches to matching a space in regex using Python:
Approach 1: Using the \s Escape Sequence
One way to match a space in regex using Python is to use the \s
escape sequence. This sequence matches any whitespace character, including spaces, tabs, and newlines. You can use it in combination with other regex patterns to perform more complex matching operations.
Here’s an example of how to use the \s
escape sequence to match a space in a string:
import re # Example string string = "Hello World" # Match a space using \s matches = re.findall(r'\s', string) print(matches) # Output: [' ']
In this example, we import the re
module, which provides functions for working with regular expressions in Python. We define a string variable string
containing the phrase “Hello World”. We then use the re.findall()
function to find all occurrences of a space in the string using the regex pattern \s
. The result is stored in the matches
variable, which is then printed to the console.
The output of this code will be [' ']
, indicating that the space character was successfully matched.
Related Article: How to Execute a Program or System Command in Python
Approach 2: Using a Literal Space Character
Another way to match a space in regex using Python is to use the literal space character itself. This involves using a regular expression pattern that includes a space character within single quotes.
Here’s an example of how to use a literal space character to match a space in a string:
import re # Example string string = "Hello World" # Match a space using a literal space character matches = re.findall(r' ', string) print(matches) # Output: [' ']
In this example, we again import the re
module and define a string variable string
containing the phrase “Hello World”. We use the re.findall()
function to find all occurrences of a space in the string using the regex pattern ' '
. The result is stored in the matches
variable and printed to the console.
The output of this code will also be [' ']
, indicating that the space character was successfully matched.
Best Practices
When matching a space in regex using Python, it’s important to consider some best practices:
1. Use raw strings: To avoid issues with backslashes being interpreted as escape characters, it’s recommended to use raw strings when working with regex patterns in Python. Raw strings are denoted by prefixing the string literal with an r
. For example, r'\s'
is a raw string representing the regex pattern \s
.
2. Consider whitespace variations: Keep in mind that the \s
escape sequence matches any whitespace character, including spaces, tabs, and newlines. If you want to specifically match only spaces, you can use a literal space character as shown in approach 2.
3. Be mindful of word boundaries: When matching spaces in text, it’s important to consider word boundaries to avoid unintended matches. For example, if you want to match a standalone word surrounded by spaces, you can use the \b
word boundary anchor in your regex pattern. For example, r'\bword\b'
would match the exact word “word” surrounded by spaces.
4. Test and validate your regex patterns: Regular expressions can be complex and prone to mistakes. It’s important to thoroughly test and validate your regex patterns using sample inputs to ensure they match the intended spaces or characters.
Related Article: How to Use Python with Multiple Languages (Locale Guide)