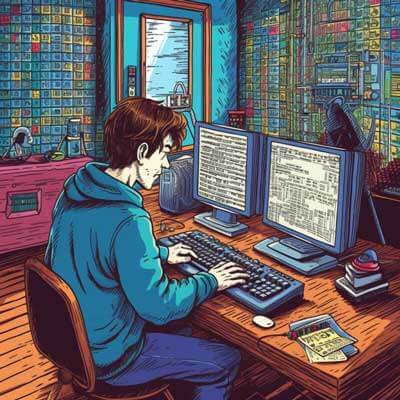
Table of Contents
A progress bar is a graphical representation of the progress of a task. It is often used to provide feedback to users about the completion status of a long-running process. In Python, there are several ways to implement a progress bar. In this answer, we will explore two popular methods: using the tqdm
library and using the progressbar2
library.
Method 1: Using the tqdm Library
The tqdm
library is a popular choice for implementing progress bars in Python. It provides a simple and easy-to-use interface for displaying progress bars in the command line.
To use the tqdm
library, you first need to install it. You can install it using pip
by running the following command:
pip install tqdm
Once you have installed the tqdm
library, you can use it in your Python code as follows:
from tqdm import tqdm import time # Define the number of iterations total_iterations = 100 # Create a progress bar object progress_bar = tqdm(total=total_iterations) # Perform the iterations for i in range(total_iterations): # Perform some task time.sleep(0.1) # Update the progress bar progress_bar.update(1) # Close the progress bar progress_bar.close()
In this example, we import the tqdm
module and the time
module. We then define the total number of iterations and create a progress bar object using the tqdm
function. Inside the loop, we perform some task (in this case, a simple sleep for demonstration purposes) and update the progress bar using the update
method. Finally, we close the progress bar using the close
method.
The tqdm
library also provides additional features such as estimating the remaining time, displaying the progress as a percentage, and displaying a progress bar with a specific format. You can find more information about these features in the tqdm
documentation.
Related Article: Tutorial of Trimming Strings in Python
Method 2: Using the progressbar2 Library
Another popular library for implementing progress bars in Python is progressbar2
. Similar to the tqdm
library, it provides a straightforward way to create and update progress bars in the command line.
To use the progressbar2
library, you first need to install it. You can install it using pip
by running the following command:
pip install progressbar2
Once you have installed the progressbar2
library, you can use it in your Python code as follows:
import progressbar import time # Define the number of iterations total_iterations = 100 # Create a progress bar widget progress_bar = progressbar.ProgressBar(max_value=total_iterations) # Perform the iterations for i in range(total_iterations): # Perform some task time.sleep(0.1) # Update the progress bar progress_bar.update(i + 1) # Finish the progress bar progress_bar.finish()
In this example, we import the progressbar
module and the time
module. We then define the total number of iterations and create a progress bar widget using the ProgressBar
class. Inside the loop, we perform some task (in this case, a simple sleep for demonstration purposes) and update the progress bar using the update
method with the current iteration number. Finally, we finish the progress bar using the finish
method.
The progressbar2
library also provides additional features such as displaying the progress as a percentage, displaying the elapsed time, and displaying a progress bar with a specific format. You can find more information about these features in the progressbar2
documentation.
Alternative Ideas and Best Practices
Related Article: How to Unzip Files in Python
- If you are working with a specific framework or library that has its own progress bar implementation, it is recommended to use the built-in progress bar functionality provided by that framework or library. This can help ensure compatibility and consistency within your codebase.
- When implementing a progress bar, it is important to consider the performance impact. Updating the progress bar too frequently can slow down your code, especially if the task being performed is computationally intensive. It is recommended to update the progress bar at regular intervals or after completing a significant portion of the task.
- In addition to displaying the progress bar in the command line, you can also integrate it into graphical user interfaces (GUIs) or web applications. This can provide a more interactive and visually appealing user experience.
- When implementing a progress bar for a long-running process, it is a good practice to provide an option for the user to cancel or interrupt the process. This can be achieved by listening for user input or integrating with a signal handling mechanism.
- Consider using context managers or decorators to encapsulate the progress bar functionality and handle resource cleanup automatically. This can help improve code readability and maintainability.