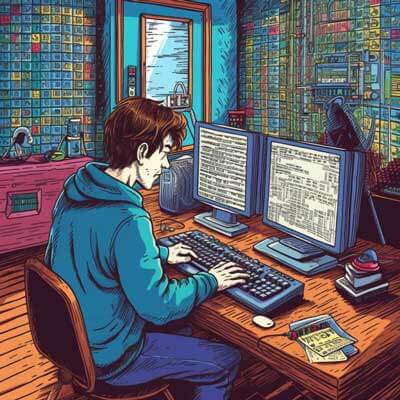
Python provides a flexible and useful warning system that helps developers identify potential issues or areas of improvement in their code. However, there may be situations where you want to suppress these warnings, either because they are irrelevant or because you have already addressed the underlying issues. In this guide, we will explore different methods to suppress Python warnings and discuss best practices for doing so.
Method 1: Using the warnings module
The simplest way to suppress Python warnings is by using the built-in warnings module. This module provides functions and classes for controlling the warning behavior in your code.
To suppress all warnings in a specific block of code, you can use the warnings.catch_warnings()
context manager along with the warnings.simplefilter()
function. Here’s an example:
import warnings def some_function(): # Code that generates warnings with warnings.catch_warnings(): warnings.simplefilter("ignore") some_function()
In this example, all warnings generated within the some_function()
will be ignored. The simplefilter("ignore")
call sets the warning filter to ignore mode.
It’s important to note that using the warnings
module to suppress warnings affects the entire Python process. If you want to suppress warnings only within a specific block of code, make sure to wrap that code in a context manager as shown above.
Related Article: How to Execute a Program or System Command in Python
Method 2: Using the -W command-line option
Another way to suppress Python warnings is by using the -W command-line option when executing your Python script. This option allows you to control the warning behavior without modifying your code.
To suppress all warnings, you can use the -W ignore
option. Here’s an example:
python -W ignore script.py
In this example, the script.py
file will be executed with all warnings ignored.
If you want to suppress specific types of warnings, you can use the -W
option followed by a comma-separated list of warning categories. For example, to suppress only the DeprecationWarning and PendingDeprecationWarning
warnings, you can use the following command:
python -W ignore::DeprecationWarning:PendingDeprecationWarning script.py
Best Practices for Suppressing Python Warnings
While suppressing warnings can be useful in certain situations, it’s important to use this feature judiciously. Here are some best practices to keep in mind:
1. Only suppress warnings that you have thoroughly investigated and determined to be safe to ignore. Ignoring warnings without proper understanding can lead to hidden bugs or unintended consequences.
2. If possible, address the underlying issues that cause the warnings instead of suppressing them. Warnings often indicate potential problems in your code that should be fixed to ensure robustness and maintainability.
3. Use context managers or command-line options to suppress warnings only in specific blocks of code or during specific executions. This helps minimize the impact on the overall codebase and makes it easier to track and fix warnings in the future.
4. Document the reasons for suppressing warnings in your code or in a project-wide documentation. This helps other developers understand the rationale behind the decision and reduces confusion or potential mistakes.
5. Regularly review and revisit the warnings you have suppressed. As your codebase evolves, the relevance and safety of suppressing certain warnings may change. Regularly reassess the need for suppressing warnings to ensure they remain valid.
Related Article: How to Use Python with Multiple Languages (Locale Guide)