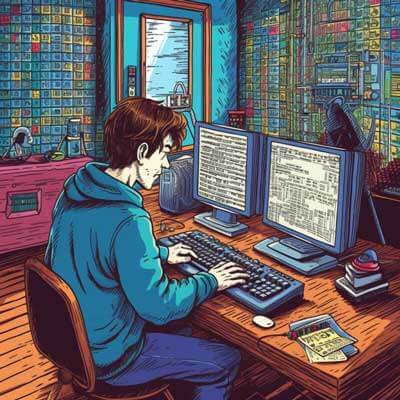
Table of Contents
To add a .gitignore
file for Python projects, follow these steps:
Step 1: Create a .gitignore
file
1. Open a text editor or your favorite code editor.
2. Create a new file in the root directory of your Python project.
3. Save the file with the name .gitignore
(including the dot at the beginning).
Related Article: Python Set Intersection Tutorial
Step 2: Specify files and directories to ignore
1. Inside the .gitignore
file, you can specify files, directories, or patterns that you want Git to ignore.
2. Each line in the .gitignore
file represents a pattern to ignore.
3. You can use wildcards, such as *
to match any characters, or ?
to match a single character.
4. You can also use the !
character to negate a pattern and include it in the repository.
5. Here are some common patterns to add to your .gitignore
file for Python projects:
# Ignore compiled Python bytecode files *.pyc # Ignore Python cache directories __pycache__/ # Ignore environment-specific settings .env # Ignore editor-specific files .vscode/ .idea/ *.sublime-*
Step 3: Save and commit the .gitignore
file
1. Save the changes to the .gitignore
file.
2. Commit the .gitignore
file to your Git repository.
3. From now on, Git will ignore the files and directories specified in the .gitignore
file.
Alternative Method: Using Git Templates
Another method to set up a .gitignore
file for Python projects is by using Git templates. Git templates allow you to set up default files for new repositories.
Related Article: How to Pip Install From a Git Repo Branch
Step 1: Create a Git template directory
1. Open a terminal and navigate to a directory where you want to store your Git templates.
2. Create a new directory called git-templates
or any other name you prefer.
3. Inside the git-templates
directory, create a subdirectory called templates
.
Step 2: Create a Python-specific template
1. Inside the templates
directory, create a new file called python.gitignore
.
2. Open the python.gitignore
file in a text editor.
3. Add the desired patterns to ignore specifically for Python projects.
4. Here are some example patterns for a Python-specific .gitignore
template:
# Ignore compiled Python bytecode files *.pyc # Ignore Python cache directories __pycache__/ # Ignore environment-specific settings .env # Ignore editor-specific files .vscode/ .idea/ *.sublime-*
Step 3: Configure Git to use the template
1. Open a terminal and navigate to the root directory of your Git repository.
2. Run the following command to set the core.templatesdir
configuration to the path of your git-templates
directory:
git config --global core.templatesdir /path/to/git-templates
3. Replace /path/to/git-templates
with the actual path to your git-templates
directory.
4. Now, whenever you initialize a new Git repository using git init
, Git will automatically copy the files from the template directory, including the Python-specific .gitignore
file.
Best Practices for Using .gitignore
in Python Projects
Here are some best practices to consider when using .gitignore
in Python projects:
1. Include the .gitignore
file in your repository to ensure that everyone working on the project follows the same ignore patterns.
2. Avoid committing sensitive information, such as API keys or passwords, by adding them to the .gitignore
file.
3. If you need to modify the .gitignore
file after committing it to the repository, make sure to communicate the changes to your team members to avoid confusion.
4. Use descriptive comments in the .gitignore
file to explain the purpose of ignored files or directories.
5. Regularly review and update your .gitignore
file to adapt to changes in your project's structure or requirements.
6. Consider using a global .gitignore
file if you have patterns that are common to all your projects. You can set up a global .gitignore
file by running:
git config --global core.excludesfile ~/.gitignore_global
7. Replace ~/.gitignore_global
with the path to your global .gitignore
file.
Related Article: Implementing a cURL command in Python
References
- Git documentation on ignoring files: https://git-scm.com/docs/gitignore
- GitHub's collection of useful .gitignore
templates: https://github.com/github/gitignore