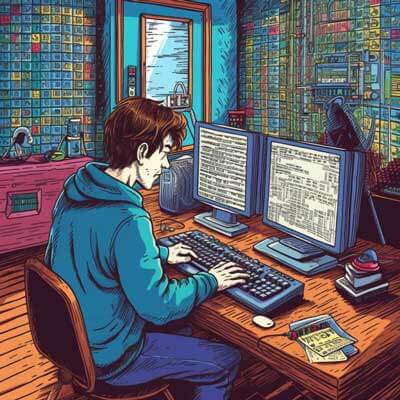
Sending emails using Python is a common task in many applications. Python provides a built-in module called smtplib
that makes it easy to send emails using the Simple Mail Transfer Protocol (SMTP). In this guide, we will walk through the steps to send an email using Python.
Step 1: Import the required modules
To get started, you need to import the necessary modules in your Python script. The smtplib
module provides the functionality to send emails, while the email
module helps in defining the email message.
import smtplib from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart
Related Article: String Comparison in Python: Best Practices and Techniques
Step 2: Set up the email parameters
Next, you need to set up the parameters for your email. This includes the sender’s email address, the recipient’s email address, the subject of the email, and the body of the email.
sender_email = "your_email@example.com" receiver_email = "recipient@example.com" subject = "Hello from Python!" body = "This is the body of the email."
Step 3: Create the email message
After setting up the email parameters, you can create the email message using the MIMEMultipart
class from the email.mime.multipart
module. This allows you to attach files or HTML content to the email if needed. In this example, we will create a simple text-based email.
message = MIMEMultipart() message["From"] = sender_email message["To"] = receiver_email message["Subject"] = subject message.attach(MIMEText(body, "plain"))
Step 4: Connect to the SMTP server
To send the email, you need to connect to an SMTP server. This server is responsible for sending the email on your behalf. You will need the SMTP server address and port number to establish a connection.
smtp_server = "smtp.example.com" smtp_port = 587
Next, you can establish a connection to the SMTP server using the smtplib.SMTP
class.
with smtplib.SMTP(smtp_server, smtp_port) as server: server.starttls() server.login(sender_email, "your_password") server.send_message(message)
Related Article: How To Limit Floats To Two Decimal Points In Python
Step 5: Sending the email
Once the connection is established and you have logged in to the SMTP server, you can send the email using the server.send_message(message)
method.
server.send_message(message)
After sending the email, you can close the connection to the SMTP server.
server.quit()
Alternative Approach: Using a third-party library
While the built-in smtplib
module is sufficient for sending basic emails, you may encounter more complex requirements in your projects. In such cases, using a third-party library like yagmail
or smtplib
can provide additional features and simplify the email sending process.
For example, with yagmail
, you can send emails without having to enter your email password in your code. It uses keyring and OAuth2 to securely authenticate with the email provider.
Here’s an example of how to send an email using yagmail
:
import yagmail sender_email = "your_email@example.com" receiver_email = "recipient@example.com" subject = "Hello from Python!" body = "This is the body of the email." yag = yagmail.SMTP(sender_email) yag.send(receiver_email, subject, body)
Make sure to install the yagmail
library using pip before using it:
pip install yagmail
Best Practices
When sending emails using Python, it’s important to follow best practices to ensure a successful delivery and avoid being flagged as spam:
1. Use a valid sender email address: Make sure the sender’s email address is valid and belongs to a domain that you have permission to send emails from.
2. Provide a meaningful subject: The subject of the email should accurately reflect the content and purpose of the message.
3. Personalize the email: If possible, personalize the email by including the recipient’s name or other relevant information.
4. Handle exceptions: When sending emails, exceptions can occur due to network issues or incorrect email configurations. Make sure to handle these exceptions gracefully to avoid unexpected errors in your application.
5. Test thoroughly: Before deploying your email-sending code to production, thoroughly test it in a development or staging environment to ensure it works as expected.