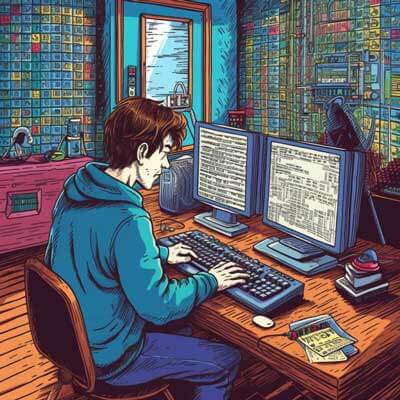
To get today’s date in the YYYY MM DD format in Python, you can use the datetime
module. Here are two possible ways to achieve this:
Method 1: Using the strftime() Method
You can use the strftime()
method from the datetime
module to format the current date according to your desired format. The strftime()
method allows you to specify a format string that defines how the date should be displayed.
Here’s an example that shows how to get today’s date in the YYYY MM DD format:
from datetime import datetime # Get today's date today = datetime.today() # Format the date as YYYY MM DD formatted_date = today.strftime("%Y %m %d") # Print the formatted date print(formatted_date)
This will output the current date in the format “YYYY MM DD”, such as “2022 01 01”.
Related Article: How To Limit Floats To Two Decimal Points In Python
Method 2: Using the strptime() and strftime() Methods
Another approach is to use the strptime()
method to parse the current date as a string and then use the strftime()
method to format it.
Here’s an example that demonstrates this approach:
from datetime import datetime # Get the current date as a string current_date_str = datetime.now().strftime("%Y-%m-%d") # Parse the current date string current_date = datetime.strptime(current_date_str, "%Y-%m-%d") # Format the parsed date as YYYY MM DD formatted_date = current_date.strftime("%Y %m %d") # Print the formatted date print(formatted_date)
In this example, we first use strftime()
to get the current date as a string in the format “YYYY-MM-DD”. Then, we use strptime()
to parse the date string and convert it to a datetime
object. Finally, we use strftime()
again to format the datetime
object as “YYYY MM DD”.
Best Practices and Suggestions
When working with dates in Python, it’s important to keep the following best practices in mind:
1. Import the datetime
module: Before using any of the date-related functions and classes, make sure to import the datetime
module.
2. Use the appropriate format codes: When formatting dates using the strftime()
method, you need to use the appropriate format codes to represent different date components. For example, %Y
represents the four-digit year, %m
represents the zero-padded month, and %d
represents the zero-padded day.
3. Be aware of the system’s default locale: The strftime()
method can be affected by the system’s default locale settings. To ensure consistent date formatting, you can set the LC_TIME
environment variable to a specific locale before running your Python script. For example, you can set it to “C” for the default POSIX locale.
4. Consider using third-party libraries: If you need to perform more advanced date manipulation or handle time zones, you might consider using third-party libraries like dateutil
or pytz
. These libraries provide additional functionality and make certain tasks easier.
Related Article: How To Rename A File With Python