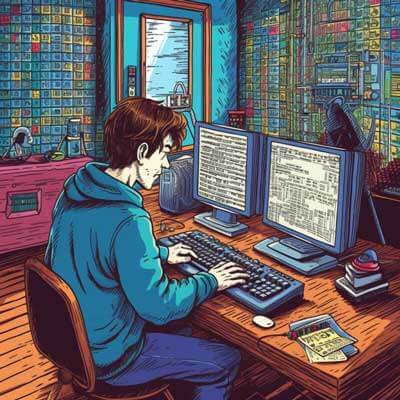
Table of Contents
To get the row count of a Pandas DataFrame in Python, you have multiple options. Here are two possible answers:
Answer 1: Using the len() function
One simple way to get the row count of a Pandas DataFrame is by using the len() function. The len() function returns the number of elements in an object, so when applied to a DataFrame, it will give you the row count.
Here's an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Jane', 'Alice', 'Bob'], 'Age': [25, 30, 35, 40]} df = pd.DataFrame(data) # Get the row count using len() row_count = len(df) print("Row Count:", row_count)
Output:
Row Count: 4
In this example, we create a DataFrame with two columns: 'Name' and 'Age'. We then use the len() function to get the row count of the DataFrame and store it in the variable 'row_count'. Finally, we print the row count.
Related Article: How to Install Specific Package Versions With Pip in Python
Answer 2: Using the shape attribute
Another way to get the row count of a Pandas DataFrame is by using the shape attribute. The shape attribute returns a tuple representing the dimensions of the DataFrame, where the first element corresponds to the number of rows.
Here's an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Jane', 'Alice', 'Bob'], 'Age': [25, 30, 35, 40]} df = pd.DataFrame(data) # Get the row count using the shape attribute row_count = df.shape[0] print("Row Count:", row_count)
Output:
Row Count: 4
In this example, we create a DataFrame with two columns: 'Name' and 'Age'. We then use the shape attribute to access the dimensions of the DataFrame and retrieve the number of rows by accessing the first element of the tuple (shape[0]). Finally, we print the row count.
Why is the question asked?
The question "How to get the row count of a Pandas DataFrame?" is commonly asked by Python developers working with data analysis or data manipulation tasks using Pandas. Knowing the row count of a DataFrame is essential for various purposes, such as understanding the size of the dataset, performing data quality checks, or determining the number of iterations for data processing tasks.
By having the ability to obtain the row count, developers can better analyze and manipulate their data, and make informed decisions based on the dataset size.
Potential Reasons for Asking the Question
There are several potential reasons why someone might ask the question "How to get the row count of a Pandas DataFrame?". Some of these reasons include:
1. Data Analysis: When performing data analysis tasks, it is often necessary to know the size of the dataset. The row count provides an essential metric for understanding the volume of data and can help in making decisions regarding data analysis techniques, resource allocation, or statistical calculations.
2. Data Cleaning: Before cleaning or preprocessing a dataset, it is useful to know the number of rows present. This information allows developers to assess the impact of data cleaning operations on the dataset size and identify potential issues such as missing data or duplicated records.
3. Loop Iterations: In certain scenarios, developers may need to iterate over the rows of a DataFrame using loops. Having the row count beforehand allows developers to set the correct number of iterations and avoid errors such as index out of range.
4. Performance Optimization: Understanding the row count can be helpful for optimizing the performance of data processing tasks. By knowing the size of the dataset, developers can estimate the time complexity of their operations and make adjustments accordingly.
Related Article: How to Change Column Type in Pandas
Suggestions and Alternative Ideas
While the above answers provide simple and straightforward ways to obtain the row count of a Pandas DataFrame, there are also alternative ideas and suggestions that can be considered:
1. Using the info() method: The info() method provides a concise summary of the DataFrame, including the row count, column count, and data types. If you are interested in obtaining additional information about the DataFrame, such as memory usage or data types, using the info() method can be a convenient option.
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Jane', 'Alice', 'Bob'], 'Age': [25, 30, 35, 40]} df = pd.DataFrame(data) # Use the info() method to get the DataFrame summary df.info()
Output:
RangeIndex: 4 entries, 0 to 3 Data columns (total 2 columns): # Column Non-Null Count Dtype --- ------ -------------- ----- 0 Name 4 non-null object 1 Age 4 non-null int64 dtypes: int64(1), object(1) memory usage: 192.0+ bytes
2. Using the count() method: The count() method returns the number of non-null values for each column in the DataFrame. By selecting any column and retrieving its count, you can obtain the row count.
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Jane', 'Alice', 'Bob'], 'Age': [25, 30, 35, 40]} df = pd.DataFrame(data) # Get the row count using the count() method on a column row_count = df['Name'].count() print("Row Count:", row_count)
Output:
Row Count: 4
In this example, we use the count() method on the 'Name' column, which returns the number of non-null values in that column. Since all rows have a non-null value for the 'Name' column, the count will be equal to the row count of the DataFrame.
Best Practices
When working with Pandas DataFrames and needing to obtain the row count, it's recommended to follow these best practices:
1. Use the most straightforward and concise method: The simplest and most direct way to get the row count is by using the len() function or the shape attribute. These methods are widely known and understood, making the code more readable and maintainable.
2. Consider the performance implications: If performance is a concern, using the shape attribute is generally faster than using len() or count() methods. The shape attribute retrieves the row count directly from the DataFrame's internal metadata without iterating over the rows or columns.
3. Handle missing data appropriately: Depending on the requirements of your analysis or application, it's important to handle missing data appropriately. If your dataset contains missing values, consider using methods like dropna() or fillna() before retrieving the row count, to ensure accurate results.
4. Document your code: As with any code, it's crucial to add comments or documentation to explain the purpose of obtaining the row count. This will make it easier for other developers to understand the code and its context.