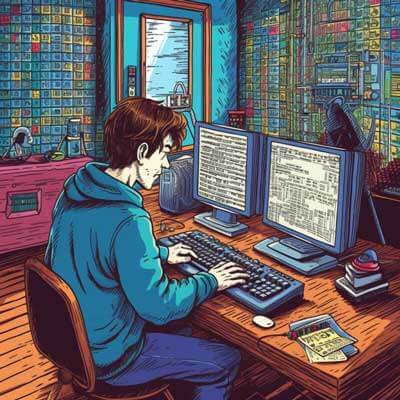
Table of Contents
What is typescript-eslint/eslint-plugin?
typescript-eslint/eslint-plugin is a plugin for ESLint that provides linting rules specifically tailored for TypeScript code. ESLint is a popular linting tool that helps catch code errors, enforce coding conventions, and improve code quality. However, ESLint originally focused on JavaScript code and had limited support for TypeScript. That's where typescript-eslint/eslint-plugin comes in.
By using typescript-eslint/eslint-plugin, you can leverage the power of ESLint to lint your TypeScript code and catch potential issues early on. The plugin provides a set of rules that take into account the unique features and syntax of TypeScript, allowing you to write cleaner, more maintainable code.
Related Article: How to Convert Strings to Booleans in TypeScript
Example:
// .eslintrc.js module.exports = { parser: '@typescript-eslint/parser', plugins: ['@typescript-eslint/eslint-plugin'], extends: ['plugin:@typescript-eslint/recommended'], };
In this example, we configure ESLint to use the @typescript-eslint/parser as the parser for TypeScript code and load the @typescript-eslint/eslint-plugin. We also extend the recommended rule set provided by the plugin, which includes a set of rules that are considered best practices for TypeScript code.
What is typescript-eslint/parser?
typescript-eslint/parser is the parser that allows ESLint to understand and analyze TypeScript code. It converts TypeScript code into an abstract syntax tree (AST), which is a data structure representing the code's syntax and structure.
The AST generated by typescript-eslint/parser is then used by ESLint to apply the configured linting rules. This allows ESLint to analyze TypeScript code and provide feedback on potential issues such as unused variables, missing type annotations, or incorrect imports.
Example:
// some-file.ts function greet(name: string) { console.log(`Hello, ${name}!`); } greet('John');
In this example TypeScript code, typescript-eslint/parser would parse the code and generate an AST that represents the structure of the code. This AST would then be used by ESLint to apply linting rules and provide feedback. For example, if there was a linting rule that enforces the use of type annotations for function parameters, ESLint would flag the lack of a type annotation for the name
parameter in the greet
function.
Related Article: How to Get an Object Value by Dynamic Keys in TypeScript
How does typescript-eslint work with TypeScript?
typescript-eslint works seamlessly with TypeScript by extending ESLint's capabilities to handle TypeScript-specific features and syntax. It consists of two main components: typescript-eslint/parser and typescript-eslint/eslint-plugin.
The typescript-eslint/parser is responsible for parsing TypeScript code and generating an AST that ESLint can understand. It leverages the TypeScript compiler's parser to ensure accurate parsing of TypeScript syntax.
The typescript-eslint/eslint-plugin provides a set of rules that are specific to TypeScript, allowing ESLint to analyze and lint TypeScript code. These rules cover a wide range of areas, including type checking, code style, and best practices for TypeScript development.
By using typescript-eslint, you can benefit from the useful linting capabilities of ESLint while also taking advantage of TypeScript's static type checking. This combination helps catch potential issues early on, improves code quality, and enhances the overall development experience.
Example:
// some-file.ts function add(a: number, b: number) { return a + b; } const result = add(1, '2'); // This will trigger a type error in TypeScript console.log(result);
In this example, typescript-eslint/parser would parse the TypeScript code and generate an AST, which would then be used by typescript-eslint/eslint-plugin to apply linting rules. In this case, the type error in the add
function call would be flagged by the TypeScript type checker, while ESLint could catch other issues such as unused variables or missing return statements.
What is ban-types in TypeScript?
ban-types is a TypeScript rule provided by typescript-eslint/eslint-plugin that helps enforce stricter type checking by discouraging the use of certain types. The rule is designed to prevent the use of overly generic types or types that may lead to unsafe code.
The ban-types rule can be configured to disallow specific types or type patterns. For example, you can configure it to ban the use of the any
type, which is often considered a code smell in TypeScript due to its lack of type safety. Similarly, you can ban the use of specific type literals or object types.
Example:
// .eslintrc.js module.exports = { rules: { '@typescript-eslint/ban-types': [ 'error', { types: { '{ String, Number }': { message: 'Avoid using object types with multiple properties.', fixWith: 'unknown', }, '{}': 'Avoid using empty object types.', }, }, ], }, };
In this example configuration, the ban-types rule is configured to disallow object types with multiple properties and empty object types. If such types are used, ESLint will raise an error and provide a suggested fix. In this case, the suggested fix is to use the unknown
type instead.
Related Article: Tutorial: Checking if a Value is in Enum in TypeScript
Why is type checking important in TypeScript?
TypeScript is a statically typed superset of JavaScript that brings type safety to the language. Type checking is a fundamental feature of TypeScript that ensures that the code is correct in terms of types. It helps catch potential errors and provides early feedback on incorrect or inconsistent usage of variables, functions, and objects.
By enforcing type checking, TypeScript helps prevent runtime errors and reduces the likelihood of encountering unexpected behavior. It improves code quality, readability, and maintainability by providing clarity on the expected types and interfaces of the code.
Type checking also enables better tooling support, such as autocompletion, refactoring, and code navigation. It allows developers to leverage the full power of modern IDEs and code editors, making development faster and more efficient.
Example:
// some-file.ts function multiply(a: number, b: number) { return a * b; } const result = multiply(2, '3'); // This will trigger a type error in TypeScript console.log(result);
In this example, the type checker in TypeScript would catch the type error in the multiply
function call, as the second argument is a string instead of a number. This type error would be caught at compile time, preventing a potential runtime error and providing early feedback to the developer.
How can we ensure type safety in TypeScript?
To ensure type safety in TypeScript, there are several best practices and techniques that can be followed:
1. Use explicit type annotations:
Explicitly annotating variables, function parameters, and return types with appropriate types helps ensure that the code is correctly typed. It provides clarity and prevents potential type errors.
// Explicit type annotations let name: string = 'John'; function add(a: number, b: number): number { return a + b; }
Related Article: How to Configure the Awesome TypeScript Loader
2. Avoid using the 'any' type:
The any
type in TypeScript disables type checking for a variable or expression, allowing it to have any type. However, overusing the any
type undermines the benefits of TypeScript's type system and can lead to unsafe code. It is recommended to avoid using the any
type and instead use more specific types.
// Avoid using the 'any' type let data: any = fetchData();
3. Use union and intersection types:
Union and intersection types allow combining multiple types, providing flexibility while maintaining type safety. Union types represent a value that can have one of several possible types, while intersection types represent a value that has all the properties of multiple types.
// Union and intersection types type User = { name: string; age: number; }; type Employee = { companyId: string; }; type UserEmployee = User & Employee; // Intersection type function processUser(user: User | Employee) { // ... }
4. Enable strictNullChecks:
Enabling the strictNullChecks compiler option in TypeScript ensures that variables can't have the value null
or undefined
unless explicitly allowed by using the union type T | null | undefined
. This helps eliminate potential null or undefined errors at runtime.
// tsconfig.json { "compilerOptions": { "strictNullChecks": true } }
5. Utilize type guards and assertions:
Type guards and assertions help narrow down the type of a variable within a conditional block, enabling more precise type checking and preventing type errors.
// Type guards and assertions function processValue(value: string | number) { if (typeof value === 'string') { // value is narrowed down to string console.log(value.toUpperCase()); } else { // value is narrowed down to number console.log(value.toFixed(2)); } }
By following these practices, developers can ensure type safety in TypeScript and minimize the likelihood of encountering type-related issues.
Related Article: Tutorial: Generating GUID in TypeScript
Understanding type annotations in TypeScript
Type annotations in TypeScript are used to explicitly specify the type of a variable, function parameter, or function return type. They provide clarity and help enforce type checking in the code.
Type annotations are written using the colon :
followed by the desired type. They can be applied to variables, function parameters, function return types, object properties, and more.
Example:
// Variable with type annotation let name: string = 'John'; // Function with type annotations for parameters and return type function add(a: number, b: number): number { return a + b; } // Object with type annotations for properties type Person = { name: string; age: number; }; const person: Person = { name: 'John', age: 30, };
In this example, the variable name
is explicitly annotated with the type string
, the function add
has type annotations for its parameters a
and b
(both number
) and its return type (number
), and the object person
has type annotations for its properties name
and age
.
How does type inference work in TypeScript?
TypeScript uses type inference to automatically determine the type of a variable based on its assigned value. Type inference analyzes the code and infers the most appropriate type based on the available information.
Type inference works by examining the value assigned to a variable and applying a set of rules to determine the type. It takes into account the literal value, contextual information, and any available type annotations.
Example:
// Type inference let name = 'John'; // Type inference infers the type 'string' let age = 30; // Type inference infers the type 'number' function add(a: number, b: number) { return a + b; // Type inference infers the return type 'number' } const person = { name: 'John', age: 30, }; // Type inference infers the type { name: string, age: number }
In this example, the variables name
and age
are assigned string and number values respectively. TypeScript uses type inference to infer that the types of name
and age
are string
and number
based on the assigned values.
Similarly, the function add
takes two parameters of type number
and returns the sum of the parameters, so TypeScript infers the return type of add
as number
.
The object person
is assigned an object literal with properties name
and age
. TypeScript infers the type of person
as { name: string, age: number }
based on the properties in the object literal.
Related Article: How to Exclude a Property in TypeScript
Configuring typescript-eslint in your project
To configure typescript-eslint in your project, follow these steps:
1. Install the required dependencies:
First, install the necessary dependencies:
npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
2. Create an ESLint configuration file:
Create an .eslintrc.js
configuration file in the root of your project:
// .eslintrc.js module.exports = { parser: '@typescript-eslint/parser', plugins: ['@typescript-eslint/eslint-plugin'], extends: ['plugin:@typescript-eslint/recommended'], };
In this example, we configure ESLint to use @typescript-eslint/parser
as the parser for TypeScript code and load the @typescript-eslint/eslint-plugin
. We also extend the recommended rule set provided by the plugin, which includes a set of rules that are considered best practices for TypeScript code.
3. Configure additional rules:
Customize the configuration by adding or modifying rules according to your project's requirements. You can find a list of available rules and their documentation in the typescript-eslint repository.
Related Article: How to Work with Dynamic Objects in TypeScript
4. Run ESLint:
Finally, run ESLint to lint your TypeScript code:
npx eslint .
This will lint all the TypeScript files in your project according to the configured rules.
Best practices for working with types in TypeScript
When working with types in TypeScript, it's important to follow best practices to ensure code quality, maintainability, and readability. Here are some best practices to consider:
1. Use meaningful and descriptive type names:
Choose type names that accurately describe the purpose and meaning of the type. This helps improve code readability and makes it easier for other developers to understand the code.
// Good type User = { name: string; age: number; }; // Bad: Unclear type name type P = { n: string; a: number; };
2. Prefer type aliases over inline types:
Use type aliases to define reusable types instead of inline types. Type aliases make the code more readable and maintainable by giving meaningful names to complex or commonly used types.
// Good type User = { name: string; age: number; }; // Bad: Inline type function processUser(user: { name: string; age: number }) { // ... }
Related Article: Building a Rules Engine with TypeScript
3. Use union and intersection types effectively:
Union and intersection types are useful features in TypeScript. Use them effectively to model complex types and improve code flexibility and expressiveness.
// Good type User = { name: string; age: number; }; type Employee = { companyId: string; }; type UserEmployee = User & Employee; // Bad: Redundant type definition type UserEmployee = { name: string; age: number; companyId: string; };
4. Enable strictNullChecks:
Enable the strictNullChecks compiler option in TypeScript to prevent null or undefined errors at runtime. This ensures that variables can't have the value null
or undefined
unless explicitly allowed by using the union type T | null | undefined
.
5. Regularly review and refactor types:
As your codebase evolves, regularly review and refactor your types to ensure they accurately represent the current state of the code. Remove unused or redundant types and update types as needed to reflect any changes in the code.
By following these best practices, you can ensure that your TypeScript code is well-typed, maintainable, and of high quality.
External Sources