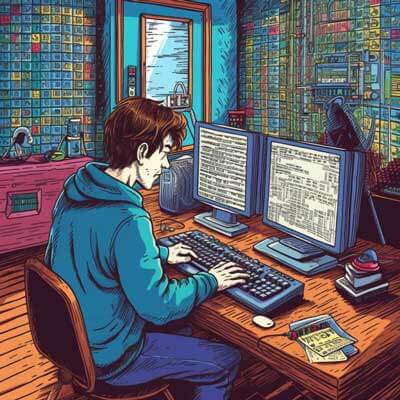
- Comparing Dates in TypeScript
- Comparing Dates Without Considering Time in TypeScript
- Comparison Operators for Dates in TypeScript
- Comparing Dates with Different Timezones in TypeScript
- Checking if One Date is Before Another in TypeScript
- Checking if One Date is After Another in TypeScript
- External Sources
Comparing Dates in TypeScript
When working with dates in TypeScript, there are several ways to compare them. The Date object in TypeScript provides methods and operators that allow you to compare dates based on different criteria such as equality, order, and range.
To compare two dates in TypeScript, you can use the following methods and operators:
1. The equality operator (== or ===) to check if two dates are the same.
2. The greater than operator (>) to check if one date is after another.
3. The less than operator (<) to check if one date is before another. 4. The greater than or equal to operator (>=) to check if one date is after or the same as another.
5. The less than or equal to operator (<=) to check if one date is before or the same as another.
Here are some examples of how to compare dates in TypeScript:
const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-02'); console.log(date1 === date2); // false console.log(date1 < date2); // true console.log(date1 > date2); // false console.log(date1 <= date2); // true console.log(date1 >= date2); // false
In the above example, we create two Date objects, date1
and date2
, representing different dates. We then use the comparison operators to compare these dates and log the results to the console.
Related Article: How to Implement and Use Generics in Typescript
Comparing Dates Without Considering Time in TypeScript
When comparing dates in TypeScript, it is often necessary to ignore the time portion of the dates and only compare the dates based on their year, month, and day values.
To compare dates without considering time in TypeScript, you can use the setHours
, setMinutes
, setSeconds
, and setMilliseconds
methods of the Date object to set the time portion of the dates to the same values.
Here is an example of how to compare dates without considering time in TypeScript:
const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-01'); date1.setHours(0, 0, 0, 0); date2.setHours(0, 0, 0, 0); console.log(date1 === date2); // true
In the above example, we create two Date objects, date1
and date2
, representing the same date. We then use the setHours
method to set the time portion of both dates to 0. Finally, we compare the dates using the equality operator and log the result to the console.
Comparison Operators for Dates in TypeScript
As mentioned earlier, TypeScript provides several comparison operators that can be used to compare dates based on different criteria. These operators include:
1. The equality operator (== or ===) to check if two dates are the same.
2. The greater than operator (>) to check if one date is after another.
3. The less than operator (<) to check if one date is before another. 4. The greater than or equal to operator (>=) to check if one date is after or the same as another.
5. The less than or equal to operator (<=) to check if one date is before or the same as another.
These operators can be used to compare dates directly or in combination with other logic operators.
Here are some examples of how to use comparison operators for dates in TypeScript:
const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-02'); console.log(date1 === date2); // false console.log(date1 < date2); // true console.log(date1 > date2); // false console.log(date1 <= date2); // true console.log(date1 >= date2); // false
In the above example, we create two Date objects, date1
and date2
, representing different dates. We then use the comparison operators to compare these dates and log the results to the console.
Comparing Dates with Different Timezones in TypeScript
When comparing dates with different time zones in TypeScript, it is important to consider the time zone offset of each date. The time zone offset represents the difference, in minutes, between the local time and UTC (Coordinated Universal Time).
To compare dates with different time zones in TypeScript, you can use the getTimezoneOffset
method of the Date object to get the time zone offset of each date. You can then adjust the dates based on their time zone offsets before comparing them.
Here is an example of how to compare dates with different time zones in TypeScript:
const date1 = new Date('2022-01-01T00:00:00-07:00'); // Date in UTC-7 time zone const date2 = new Date('2022-01-01T00:00:00+02:00'); // Date in UTC+2 time zone const offset1 = date1.getTimezoneOffset(); const offset2 = date2.getTimezoneOffset(); date1.setMinutes(date1.getMinutes() + offset1); date2.setMinutes(date2.getMinutes() + offset2); console.log(date1 === date2); // true
In the above example, we create two Date objects, date1
and date2
, representing the same date but in different time zones. We then use the getTimezoneOffset
method to get the time zone offsets of both dates. Finally, we adjust the dates based on their time zone offsets and compare them using the equality operator.
Related Article: How to Check If a String is in an Enum in TypeScript
Checking if One Date is Before Another in TypeScript
To check if one date is before another in TypeScript, you can use the less than operator (<) or the greater than operator (>) depending on the desired comparison.
Here is an example of how to check if one date is before another in TypeScript:
const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-02'); console.log(date1 < date2); // true
In the above example, we create two Date objects, date1
and date2
, representing different dates. We then use the less than operator to check if date1
is before date2
and log the result to the console.
Checking if One Date is After Another in TypeScript
To check if one date is after another in TypeScript, you can use the greater than operator (>) or the less than operator (<) depending on the desired comparison. Here is an example of how to check if one date is after another in TypeScript: typescript const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-02'); console.log(date1 > date2); // false
<pre>{{EJS5}}</pre>
In the above example, we create two Date objects,
date1 and
date2, representing the same date. We then use the
method to set the time portion of both dates to 0. Finally, we compare the dates using the equality operator and log the result to the console.
<h2>Comparing a Date with Today's Date in TypeScript</h2>
To compare a date with today's date in TypeScript, you can create a new Date object representing today's date and compare it with the desired date using the desired comparison operator.
Here is an example of how to compare a date with today's date in TypeScript:
<pre>{{EJS6}}</pre>
In the above example, we create a Date object
representing a specific date. We then create a new Date object
representing today's date. Finally, we use the less than operator to check if
is before
and log the result to the console.
<h2>Calculating the Difference Between Two Dates in TypeScript</h2>
To calculate the difference between two dates in TypeScript, you can <a href="https://www.squash.io/tutorial-date-subtraction-in-typescript/">subtract one date</a> from another, which will result in the difference in milliseconds. You can then convert the difference to the desired unit (e.g., days, hours, minutes) as needed.
Here is an example of how to calculate the difference between two dates in TypeScript:
<pre>{{EJS7}}</pre>
In the above example, we create two Date objects,
date1 and
date2, representing different dates. We then subtract
date1 from
date2 to get the difference in milliseconds. We then convert the difference to seconds, minutes, hours, and days by dividing by the appropriate conversion factors. Finally, we log the difference in days to the console.
<h2>Checking if a Date is Within a Specific Range in TypeScript</h2>
To check if a date is within a specific range in TypeScript, you can use the greater than or equal to operator (>=) and the less than or equal to operator (<=) in combination. Here is an example of how to check if a date is within a specific range in TypeScript:
const date = new Date(‘2022-01-01’); const startDate = new Date(‘2022-01-01’); const endDate = new Date(‘2022-01-31’); console.log(date >= startDate && date <= endDate); // true
In the above example, we create a Date object
representing a specific date. We also create two Date objects
and
representing the start and end dates of the desired range. Finally, we use the greater than or equal to operator and the less than or equal to operator to check if
is within the range defined by
and
`, and log the result to the console.
External Sources
– Mozilla Developer Network: Date
– TypeScript Documentation: Date
– MDN Web Docs: Comparing Dates