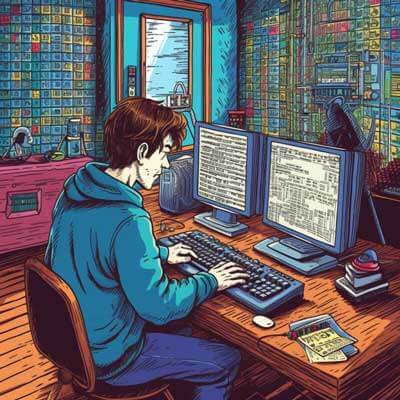
Table of Contents
Introduction to Redis and Spring Boot Integration
Redis is an open-source, in-memory data structure store that can be used as a database, cache, or message broker. It provides high performance and scalability, making it a popular choice for various use cases in modern applications. Spring Boot, on the other hand, is a framework that simplifies the development of Java applications. In this chapter, we will explore how Redis can be integrated with Spring Boot to leverage its features and enhance the functionality of our applications.
Related Article: How to Retrieve Current Date and Time in Java
Setting Up Redis for Spring Boot Application
Before we can start integrating Redis with a Spring Boot application, we need to set up a Redis server and configure our project to connect to it. Here are the steps to follow:
Step 1: Install Redis
First, we need to install Redis on our local machine or set up a Redis server in the cloud. You can refer to the official Redis documentation for installation instructions specific to your operating system.
Step 2: Configure Redis Connection
Once Redis is installed, we need to configure our Spring Boot application to connect to the Redis server. We can do this by adding the necessary dependencies and configuring the connection properties in the application.properties or application.yml file.
Here is an example of configuring Redis connection in the application.properties file:
spring.redis.host=localhost spring.redis.port=6379
Related Article: Java Design Patterns Tutorial
Basic Usage of Redis in Spring Boot
Now that we have set up Redis and configured our Spring Boot application to connect to it, let's explore the basic usage of Redis in Spring Boot. Redis provides various data structures and operations that can be used to store and retrieve data efficiently. In this section, we will cover some of the commonly used features of Redis in Spring Boot.
Storing and Retrieving Data
One of the primary use cases of Redis is storing and retrieving data. Redis supports various data structures like strings, lists, sets, sorted sets, and hashes. Let's see how we can use these data structures in a Spring Boot application.
To store a value in Redis, we can use the ValueOperations
class provided by Spring Data Redis. Here's an example:
@Autowired private RedisTemplate redisTemplate; public void storeData(String key, String value) { ValueOperations valueOperations = redisTemplate.opsForValue(); valueOperations.set(key, value); } public String retrieveData(String key) { ValueOperations valueOperations = redisTemplate.opsForValue(); return valueOperations.get(key); }
Working with Lists
Redis lists are ordered collections of strings. They can be used to implement a queue, stack, or a simple list of items. Let's see how we can use Redis lists in a Spring Boot application.
@Autowired private RedisTemplate redisTemplate; public void addToList(String key, String value) { ListOperations listOperations = redisTemplate.opsForList(); listOperations.leftPush(key, value); } public List getList(String key) { ListOperations listOperations = redisTemplate.opsForList(); return listOperations.range(key, 0, -1); }
Advanced Usage of Redis in Spring Boot
In addition to the basic usage, Redis provides advanced features that can be leveraged in a Spring Boot application. In this section, we will explore some of these advanced features and how they can be integrated into our application.
Related Article: How to Fix the Java NullPointerException
Code Snippet: Caching with Redis
Caching is a common technique used to improve the performance of applications by storing frequently accessed data in memory. Redis can be used as a cache store in a Spring Boot application to speed up data retrieval. Here's an example of how to enable caching with Redis in Spring Boot:
@Configuration @EnableCaching public class RedisCacheConfig extends CachingConfigurerSupport { @Autowired private RedisConnectionFactory redisConnectionFactory; @Bean @Override public CacheManager cacheManager() { RedisCacheManager redisCacheManager = RedisCacheManager.builder(redisConnectionFactory) .cacheDefaults(RedisCacheConfiguration.defaultCacheConfig().entryTtl(Duration.ofMinutes(5))) .build(); return redisCacheManager; } }
Code Snippet: Pub/Sub Messaging with Redis
Redis provides a publish/subscribe messaging pattern that allows multiple clients to subscribe to channels and receive messages. This feature can be useful for implementing real-time communication or event-driven architectures. Let's see how we can use pub/sub messaging with Redis in a Spring Boot application:
@Autowired private RedisTemplate redisTemplate; public void sendMessage(String channel, String message) { redisTemplate.convertAndSend(channel, message); } @RedisListener(channels = "myChannel") public void receiveMessage(String message) { // Process received message }
Use Case: Session Management with Redis
Session management is an important aspect of web applications, and Redis can be used as a session store to improve scalability and reliability. In this section, we will explore how to use Redis for session management in a Spring Boot application.
Configuring Redis as Session Store
To configure Redis as the session store in a Spring Boot application, we need to add the necessary dependencies and configure the session properties. Here's an example of configuring Redis as the session store in the application.properties file:
spring.session.store-type=redis spring.session.redis.namespace=mySession
Related Article: Tutorial on Redis Docker Compose
Using Redis for Session Storage
Once Redis is configured as the session store, Spring Session will automatically store session data in Redis. We can retrieve and manipulate session data using the HttpSession
object. Here's an example of using Redis for session storage in a Spring Boot application:
@GetMapping("/storeData") public void storeData(HttpSession session) { session.setAttribute("key", "value"); } @GetMapping("/retrieveData") public String retrieveData(HttpSession session) { return (String) session.getAttribute("key"); }
Use Case: Distributed Locking with Redis
Distributed locking is a technique used to coordinate access to shared resources in a distributed environment. Redis provides the necessary data structures and operations to implement distributed locking. In this section, we will explore how to use Redis for distributed locking in a Spring Boot application.
Code Snippet: Implementing Distributed Locking
To implement distributed locking with Redis, we can use the Redisson
library, which provides a simple and reliable way to acquire and release locks. Here's an example of implementing distributed locking with Redis in a Spring Boot application using Redisson:
@Autowired private RedissonClient redissonClient; public void acquireLock(String lockKey) { RLock lock = redissonClient.getLock(lockKey); lock.lock(); // Perform operations under lock lock.unlock(); }
Use Case: Real-time Analytics with Redis
Real-time analytics is a crucial requirement for many applications, especially those dealing with large amounts of data. Redis provides features like sorted sets and hyperloglogs that can be used to implement real-time analytics in a Spring Boot application. Let's explore how to leverage Redis for real-time analytics.
Related Article: Storing Contact Information in Java Data Structures
Code Snippet: Implementing Real-time Analytics
Redis sorted sets are ideal for storing and querying ordered data. We can use sorted sets to store timestamps and values, allowing us to perform real-time analytics on the data. Here's an example of how to implement real-time analytics with Redis in a Spring Boot application:
@Autowired private RedisTemplate redisTemplate; public void trackEvent(String event, long timestamp) { String key = "analytics:" + event; redisTemplate.opsForZSet().add(key, String.valueOf(timestamp), timestamp); } public long countEvents(String event, long startTimestamp, long endTimestamp) { String key = "analytics:" + event; return redisTemplate.opsForZSet().count(key, startTimestamp, endTimestamp); }
Best Practices for Redis Integration in Spring Boot
When integrating Redis with Spring Boot, it's important to follow best practices to ensure optimal performance, reliability, and maintainability. In this section, we will discuss some best practices that can be followed when integrating Redis in a Spring Boot application.
Use Connection Pooling
To improve performance and reduce the overhead of establishing connections to the Redis server, it is recommended to use connection pooling. Spring Boot provides support for connection pooling through the Lettuce
or Jedis
libraries. Here's an example of configuring connection pooling with Lettuce
in the application.properties file:
spring.redis.jedis.pool.max-active=10 spring.redis.jedis.pool.max-idle=5 spring.redis.jedis.pool.min-idle=2
Serialize and Deserialize Objects
When storing objects in Redis, it's important to serialize and deserialize them properly to ensure data integrity. Spring Boot provides support for serialization through the RedisTemplate
class. By default, Spring Boot uses Java serialization, but it's recommended to use a more efficient serialization format like JSON or Protobuf. Here's an example of configuring serialization with JSON in a Spring Boot application:
@Configuration public class RedisConfig { @Bean public RedisTemplate redisTemplate(RedisConnectionFactory redisConnectionFactory) { RedisTemplate template = new RedisTemplate(); template.setConnectionFactory(redisConnectionFactory); template.setDefaultSerializer(new GenericJackson2JsonRedisSerializer()); return template; } }
Related Article: How to Use the JsonProperty in Java
Error Handling in Redis Integration with Spring Boot
When integrating Redis with Spring Boot, it's important to handle errors and exceptions gracefully to ensure the stability and reliability of the application. Redis operations can fail due to various reasons like network issues, server errors, or data corruption. In this section, we will explore how to handle errors in Redis integration with Spring Boot.
Handling Redis Exceptions
Spring Boot provides built-in exception handling for Redis operations through the RedisSystemException
class. This exception can be caught and handled to perform appropriate error handling logic. Here's an example of handling Redis exceptions in a Spring Boot application:
try { // Redis operation } catch (RedisSystemException ex) { // Handle Redis exception }
Performance Considerations for Redis Integration
When integrating Redis with Spring Boot, it's important to consider performance aspects to ensure optimal application performance. Redis is known for its high performance, but there are certain factors that can impact its performance. In this section, we will discuss some performance considerations for Redis integration in a Spring Boot application.
Optimizing Redis Operations
To maximize performance, it's important to optimize Redis operations by reducing the number of round trips to the Redis server and minimizing data transfer. This can be achieved by using batch operations, pipelining, and minimizing the size of data stored in Redis. Here's an example of using batch operations with Redis in a Spring Boot application:
@Autowired private RedisTemplate redisTemplate; public void performBatchOperations() { redisTemplate.executePipelined((RedisConnection connection) -> { // Perform multiple Redis operations connection.set("key1".getBytes(), "value1".getBytes()); connection.set("key2".getBytes(), "value2".getBytes()); return null; }); }
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Advanced Techniques for Redis Integration with Spring Boot
In addition to the basic and advanced features discussed earlier, there are several advanced techniques that can be used to further enhance Redis integration in a Spring Boot application. In this section, we will explore some of these advanced techniques.
Redis Lua Scripting
Redis provides support for Lua scripting, which allows us to perform complex operations on the server side. Lua scripts can be executed atomically and can access the full power of Redis commands. This can be useful for implementing complex business logic or performing bulk operations efficiently. Here's an example of executing a Lua script in a Spring Boot application:
@Autowired private RedisScript luaScript; @Autowired private StringRedisTemplate redisTemplate; public boolean executeLuaScript(String key, String value) { List keys = Arrays.asList(key); List values = Arrays.asList(value); return redisTemplate.execute(luaScript, keys, values); }
Real World Example: Building a Chat Application with Redis and Spring Boot
To demonstrate the practical application of Redis integration in a Spring Boot application, let's build a chat application that leverages Redis Pub/Sub messaging. In this section, we will discuss the architecture, implementation, and key components of the chat application.
Architecture Overview
The chat application will consist of a chat server and multiple chat clients. The chat server will use Redis Pub/Sub messaging to handle real-time communication between clients. Each client will connect to the chat server using a WebSocket connection and will be able to send and receive messages. Redis will be used as a message broker to handle the Pub/Sub messaging.
Related Article: Saving Tree Data Structure in Java File: A Discussion
Implementation Details
The chat server will be implemented using Spring Boot and Spring WebSockets. Redis Pub/Sub messaging will be handled using Spring Data Redis. Each chat client will be a web application that connects to the chat server using a WebSocket connection.
The chat server will publish incoming messages to a Redis channel, and all connected clients will subscribe to this channel to receive messages. When a client sends a message, it will be published to the Redis channel, and all subscribed clients will receive the message.
Here's an example of configuring Redis Pub/Sub messaging in a Spring Boot application:
@Configuration @EnableWebSocketMessageBroker public class WebSocketConfig implements WebSocketMessageBrokerConfigurer { @Autowired private RedisConnectionFactory redisConnectionFactory; @Override public void configureMessageBroker(MessageBrokerRegistry registry) { RedisMessageListenerContainer container = new RedisMessageListenerContainer(); container.setConnectionFactory(redisConnectionFactory); container.addMessageListener(new RedisMessageListener(), new ChannelTopic("chatChannel")); registry.enableSimpleBroker("/topic"); registry.setApplicationDestinationPrefixes("/app"); } }