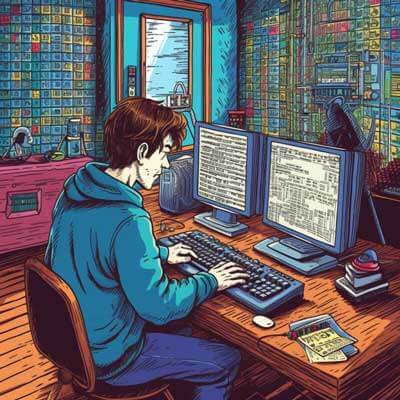
Table of Contents
Java Spring Boot
Java Spring Boot is a useful framework for building Java applications. It provides a streamlined way to create stand-alone, production-grade Spring-based applications. Spring Boot eliminates the need for complex configuration files and allows developers to focus on writing code that solves business problems.
With Spring Boot, developers can quickly set up a new project and get started with coding. It includes a wide range of features and libraries that simplify the development process. Spring Boot also provides built-in support for various deployment options, including traditional servers, cloud platforms, and containers.
To start using Spring Boot, you need to set up your development environment and create a new project. Here's an example of how to create a simple Spring Boot application:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class MyApp { public static void main(String[] args) { SpringApplication.run(MyApp.class, args); } }
In this example, we have a main class that is annotated with @SpringBootApplication
. This annotation enables auto-configuration and component scanning for our application. The main
method uses SpringApplication.run()
to start the Spring Boot application.
Spring Boot also provides a useful command-line interface (CLI) that allows you to quickly generate projects, run tests, and perform other tasks. You can install the CLI by following the instructions on the official Spring Boot website.
Overall, Spring Boot provides a convenient and efficient way to develop Java applications. Its simplicity and extensive ecosystem make it a popular choice among developers.
Related Article: How to Use Public Static Void Main String Args in Java
Security
Security is a critical aspect of any software application. It ensures that only authorized users can access sensitive information and perform actions that they are allowed to. In the context of Java Spring Boot applications, security can be implemented using the Spring Security framework.
Spring Security is a highly customizable and flexible security framework that provides a wide range of features for securing your applications. It integrates seamlessly with Spring Boot, making it easy to add authentication, authorization, and other security measures to your application.
Some of the key security features provided by Spring Security include:
- Authentication: Spring Security supports various authentication mechanisms, such as form-based authentication, HTTP Basic authentication, and OAuth2. It allows you to authenticate users based on their credentials or integrate with external authentication providers.
- Authorization: Spring Security provides a flexible authorization framework that allows you to define fine-grained access control rules. You can use annotations, expression-based access control, or custom authorization logic to secure your application's resources.
- Session management: Spring Security manages user sessions and provides features like session fixation protection, session concurrency control, and session timeout handling.
- Cross-site request forgery (CSRF) protection: Spring Security includes built-in protection against CSRF attacks. It generates and validates CSRF tokens to prevent malicious users from performing actions on behalf of authenticated users.
- Cross-site scripting (XSS) protection: Spring Security helps protect your application from XSS attacks by automatically escaping user input and providing features like Content Security Policy (CSP) and XSS prevention headers.
- Security headers: Spring Security allows you to easily configure security headers like Content-Security-Policy, X-Content-Type-Options, X-Frame-Options, and X-XSS-Protection to enhance the security of your application.
Overall, Spring Security provides a comprehensive set of features for securing Java Spring Boot applications. It allows you to customize and extend its functionality to meet the specific security requirements of your application.
Authentication
Authentication is the process of verifying the identity of a user or system. In the context of web applications, authentication typically involves validating user credentials, such as a username and password, before granting access to protected resources.
Spring Security provides a robust authentication framework that supports various authentication mechanisms. It allows you to authenticate users based on their credentials or integrate with external authentication providers like LDAP, Active Directory, or OAuth2.
To configure authentication in a Spring Boot application, you need to define an authentication manager and specify the authentication mechanism you want to use. Here's an example of how to configure form-based authentication in Spring Security:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception { auth.inMemoryAuthentication() .withUser("user") .password(encoder().encode("password")) .roles("USER"); } @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/public/**").permitAll() .anyRequest().authenticated() .and() .formLogin() .loginPage("/login") .defaultSuccessUrl("/dashboard") .permitAll() .and() .logout() .logoutUrl("/logout") .permitAll(); } @Bean public PasswordEncoder encoder() { return new BCryptPasswordEncoder(); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configureGlobal
method defines an in-memory authentication manager with a single user. The password is encoded using the BCryptPasswordEncoder.
The configure
method configures the HTTP security of our application. It specifies that requests to /public/**
should be allowed without authentication, while all other requests require authentication. It also configures the login page, default success URL, and logout URL.
Spring Security provides various other authentication mechanisms, such as HTTP Basic authentication, OAuth2, and JWT (JSON Web Token) authentication. You can choose the authentication mechanism that best suits your application's requirements.
Authorization
Authorization is the process of determining what actions a user is allowed to perform on a system or what resources they can access. In the context of web applications, authorization typically involves defining access control rules that restrict user access to certain parts of the application.
Spring Security provides a flexible authorization framework that allows you to define fine-grained access control rules. You can use annotations, expression-based access control, or custom authorization logic to secure your application's resources.
One common way to implement authorization in Spring Security is by using annotations. You can annotate your controller methods or service methods with @PreAuthorize
or @PostAuthorize
annotations to specify the access control rules. Here's an example:
@RestController @RequestMapping("/api") public class MyController { @PreAuthorize("hasRole('ADMIN')") @GetMapping("/admin") public String adminResource() { return "This resource is only accessible to admins"; } @PostAuthorize("returnObject.owner == authentication.name") @GetMapping("/my-resource") public MyResource getMyResource() { // Return a resource owned by the authenticated user } }
In this example, the adminResource
method is only accessible to users with the ADMIN
role. The getMyResource
method is accessible to all authenticated users, but it uses a post-authorization expression to ensure that the returned resource is owned by the authenticated user.
You can also configure access control rules using XML or Java configuration. Spring Security provides a rich set of expressions and methods that you can use to define complex access control rules.
In addition to annotations and expressions, Spring Security also supports custom authorization logic. You can create custom AccessDecisionVoter
or AccessDecisionManager
implementations to enforce your own authorization rules.
Overall, Spring Security provides a useful authorization framework that allows you to secure your application's resources and define fine-grained access control rules.
Related Article: How To Fix Java Certification Path Error
JSON Web Token (JWT)
JSON Web Token (JWT) is an open standard for securely transmitting information between parties as a JSON object. It is widely used for authentication and authorization in modern web applications.
A JWT consists of three parts: a header, a payload, and a signature. The header contains information about the type of token and the algorithm used to sign it. The payload contains the claims, which are statements about an entity (typically the user) and additional metadata. The signature is used to verify the integrity of the token.
JWTs are self-contained, meaning that all the necessary information to verify the token is included in the token itself. This eliminates the need for server-side storage of session data, resulting in a more scalable and stateless architecture.
Spring Security provides built-in support for JWT authentication. You can use JWTs to authenticate users and secure your RESTful APIs. Here's an example of how to configure JWT authentication in Spring Security:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .csrf().disable() .authorizeRequests() .antMatchers("/api/public/**").permitAll() .anyRequest().authenticated() .and() .addFilter(new JwtAuthenticationFilter(authenticationManager())) .addFilter(new JwtAuthorizationFilter(authenticationManager())); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the HTTP security of our application. It specifies that requests to /api/public/**
should be allowed without authentication, while all other requests require authentication.
The JwtAuthenticationFilter
and JwtAuthorizationFilter
are custom filters that handle JWT authentication and authorization, respectively. These filters extract the JWT from the request, validate it, and set the authenticated user in the Spring Security context.
To generate and sign JWTs, you can use libraries like jjwt or Nimbus JOSE+JWT. Here's an example of how to generate a JWT using jjwt:
import io.jsonwebtoken.Jwts; import io.jsonwebtoken.SignatureAlgorithm; String secretKey = "mySecretKey"; Date expirationDate = new Date(System.currentTimeMillis() + 86400000); // 1 day String token = Jwts.builder() .setSubject("user123") .setExpiration(expirationDate) .signWith(SignatureAlgorithm.HS256, secretKey) .compact();
In this example, we create a JWT with the subject "user123" and an expiration date of 1 day. We sign the token using the HS256 algorithm and our secret key.
JWTs provide a secure and scalable way to authenticate and authorize users in Spring Boot applications. They are widely used in microservices architectures and can be easily integrated with other authentication mechanisms like OAuth2.
OAuth2
OAuth2 is an open standard for authorization that allows users to grant third-party applications access to their resources without sharing their credentials. It is widely used by social media platforms, APIs, and other web services to provide secure access to user data.
In the context of Spring Boot applications, OAuth2 can be used to secure RESTful APIs and allow users to authenticate using their existing social media accounts or other identity providers.
Spring Security provides built-in support for OAuth2 authentication and authorization. You can use OAuth2 to authenticate users and secure your RESTful APIs. Here's an example of how to configure OAuth2 authentication in Spring Security:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .csrf().disable() .authorizeRequests() .antMatchers("/api/public/**").permitAll() .anyRequest().authenticated() .and() .oauth2Login() .userInfoEndpoint() .userAuthoritiesMapper(userAuthoritiesMapper()); } @Bean public GrantedAuthoritiesMapper userAuthoritiesMapper() { return (authorities) -> { Set mappedAuthorities = new HashSet(); // Map authorities based on your application's logic return mappedAuthorities; }; } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the HTTP security of our application. It specifies that requests to /api/public/**
should be allowed without authentication, while all other requests require authentication.
The oauth2Login
method configures OAuth2 authentication. It enables the OAuth2 login flow and configures the userInfoEndpoint
to map user authorities based on your application's logic.
To integrate with different OAuth2 providers, you can use Spring Boot's auto-configuration and provide the necessary client credentials in your application.properties or application.yml file. Here's an example of configuring OAuth2 with GitHub as the identity provider:
spring: security: oauth2: client: registration: github: client-id: your-client-id client-secret: your-client-secret
Once configured, you can use Spring Security's built-in @EnableOAuth2Client
and @EnableAuthorizationServer
annotations to enable OAuth2 support in your application.
Overall, OAuth2 provides a secure and scalable way to authenticate and authorize users in Spring Boot applications. It allows users to authenticate using their existing social media accounts or other identity providers, reducing the need for separate authentication systems.
Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is a security vulnerability that allows an attacker to trick a user into performing unwanted actions on a website in which they are authenticated. This vulnerability occurs when an attacker can force a user's browser to send a malicious request to a vulnerable website.
Spring Security provides built-in protection against CSRF attacks. It generates and validates CSRF tokens to prevent malicious users from performing actions on behalf of authenticated users.
To enable CSRF protection in a Spring Boot application, you need to configure it in your SecurityConfig
class. Here's an example:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .csrf() .csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse()); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the HTTP security of our application. It enables CSRF protection and uses the CookieCsrfTokenRepository
to store and validate CSRF tokens.
To ensure that CSRF protection is enabled on all forms in your application, you need to include the CSRF token in your form templates. Here's an example using Thymeleaf:
<!-- Other form fields --> <button type="submit">Submit</button>
In this example, we include a hidden input field with the CSRF token. When the form is submitted, the browser automatically includes the CSRF token in the request headers, and Spring Security validates it.
Overall, Spring Security's built-in CSRF protection helps prevent Cross-Site Request Forgery attacks in Spring Boot applications. It generates and validates CSRF tokens to ensure that only legitimate requests are processed.
Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a security vulnerability that allows an attacker to inject malicious scripts into web pages viewed by other users. This vulnerability occurs when a website does not properly validate and sanitize user input, allowing the execution of arbitrary code in the context of the vulnerable webpage.
Spring Security helps protect your application from XSS attacks by automatically escaping user input and providing features like Content Security Policy (CSP) and XSS prevention headers.
To enable XSS protection in a Spring Boot application, you need to configure it in your SecurityConfig
class. Here's an example:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .headers() .xssProtection().block(false) .contentSecurityPolicy("default-src 'self'"); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the HTTP security of our application. It enables XSS protection by disabling the XSS protection header's blocking mode. It also sets the Content Security Policy (CSP) to only allow resources from the same origin.
Spring Security automatically escapes user input when rendering views, preventing most XSS attacks. However, it's important to also properly sanitize user input before storing or displaying it in your application.
Additionally, you can use other security headers like X-Content-Type-Options
, X-Frame-Options
, and X-XSS-Protection
to further enhance the security of your application.
Overall, Spring Security's built-in XSS protection helps prevent Cross-Site Scripting attacks in Spring Boot applications. It automatically escapes user input and provides features like Content Security Policy and XSS prevention headers.
Related Article: How to Manage Collections Without a SortedList in Java
Custom Authentication Providers
Spring Security allows you to define custom authentication providers to authenticate users using your own logic or integrate with external authentication systems.
To create a custom authentication provider, you need to implement the AuthenticationProvider
interface and override the authenticate
method. Here's an example:
@Component public class CustomAuthenticationProvider implements AuthenticationProvider { @Override public Authentication authenticate(Authentication authentication) throws AuthenticationException { String username = authentication.getName(); String password = authentication.getCredentials().toString(); // Perform custom authentication logic if (isValidUser(username, password)) { List authorities = new ArrayList(); authorities.add(new SimpleGrantedAuthority("ROLE_USER")); return new UsernamePasswordAuthenticationToken(username, password, authorities); } else { throw new BadCredentialsException("Invalid username or password"); } } @Override public boolean supports(Class authentication) { return authentication.equals(UsernamePasswordAuthenticationToken.class); } private boolean isValidUser(String username, String password) { // Custom logic to validate username and password // Return true if the user is valid, false otherwise } }
In this example, we have a CustomAuthenticationProvider
class that implements the AuthenticationProvider
interface. The authenticate
method performs the custom authentication logic and returns an Authentication
object if the user is valid.
The supports
method specifies that this authentication provider supports the UsernamePasswordAuthenticationToken
class.
To use the custom authentication provider, you need to configure it in your SecurityConfig
class. Here's an example:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private CustomAuthenticationProvider authenticationProvider; @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.authenticationProvider(authenticationProvider); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the authentication manager to use our custom authentication provider.
Overall, Spring Security allows you to create custom authentication providers to authenticate users using your own logic or integrate with external authentication systems. Custom authentication providers give you full control over the authentication process and allow you to implement complex authentication mechanisms.
Success Handlers
Success handlers in Spring Security allow you to customize the behavior after a successful authentication. You can redirect the user to a specific page, display a success message, or perform other actions.
To create a custom success handler, you need to implement the AuthenticationSuccessHandler
interface and override the onAuthenticationSuccess
method. Here's an example:
@Component public class CustomSuccessHandler implements AuthenticationSuccessHandler { @Override public void onAuthenticationSuccess(HttpServletRequest request, HttpServletResponse response, Authentication authentication) throws IOException, ServletException { // Custom logic to handle successful authentication // For example, redirect the user to a specific page or display a success message response.sendRedirect("/dashboard"); } }
In this example, we have a CustomSuccessHandler
class that implements the AuthenticationSuccessHandler
interface. The onAuthenticationSuccess
method redirects the user to the /dashboard
page after a successful authentication.
To use the custom success handler, you need to configure it in your SecurityConfig
class. Here's an example:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private CustomSuccessHandler successHandler; @Override protected void configure(HttpSecurity http) throws Exception { http .formLogin() .successHandler(successHandler) .and() .logout(); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the form login to use our custom success handler. After a successful authentication, the user will be redirected to the specified page.
Overall, success handlers in Spring Security allow you to customize the behavior after a successful authentication. They give you the flexibility to perform actions like redirecting the user to a specific page, displaying a success message, or executing custom logic.
What is Spring Security?
Spring Security is a useful and highly customizable security framework for Java applications. It provides a comprehensive set of features for securing your applications, including authentication, authorization, session management, and protection against common web vulnerabilities.
Spring Security integrates seamlessly with Spring Boot, making it easy to add security to your applications. It allows you to secure your application's resources, control access to specific endpoints, and enforce fine-grained access control rules.
Spring Security follows the principle of "defense in depth" and provides multiple layers of security. It includes features like CSRF protection, XSS prevention, security headers, and secure session management to protect your application from various security threats.
With Spring Security, you can choose from various authentication mechanisms like form-based authentication, HTTP Basic authentication, OAuth2, and JWT authentication. It also supports custom authentication providers, allowing you to implement your own authentication logic or integrate with external authentication systems.
Additionally, Spring Security provides a flexible authorization framework that allows you to define access control rules using annotations, expressions, or custom logic. It supports role-based and attribute-based access control, giving you fine-grained control over who can access your application's resources.
Overall, Spring Security is a robust and versatile security framework that provides a wide range of features to secure your Java applications. Its integration with Spring Boot and extensive customization options make it a popular choice among developers.
How does Spring Security handle authentication?
Spring Security provides a flexible and extensible authentication framework that supports various authentication mechanisms. It allows you to authenticate users based on their credentials or integrate with external authentication providers.
When a user tries to access a protected resource, Spring Security intercepts the request and performs the authentication process. The authentication process typically involves verifying the user's credentials and checking if the user is authorized to access the requested resource.
Spring Security's authentication process can be summarized in the following steps:
1. User submits their credentials (e.g., username and password) to the application.
2. The application receives the authentication request and forwards it to the authentication manager.
3. The authentication manager delegates the authentication process to one or more authentication providers.
4. Each authentication provider performs the necessary authentication steps, such as verifying the user's credentials or integrating with external authentication systems.
5. If any of the authentication providers successfully authenticate the user, an Authentication
object is created and stored in the SecurityContext
.
6. The authenticated user is granted access to the requested resource, and the request is processed accordingly.
Spring Security supports various authentication providers out of the box, including in-memory authentication, JDBC authentication, LDAP authentication, and OAuth2 authentication. You can also create custom authentication providers to implement your own authentication logic or integrate with external authentication systems.
Overall, Spring Security's authentication framework provides a flexible and extensible way to authenticate users in Java applications. It allows you to choose the authentication mechanism that best suits your application's requirements and provides a seamless integration with Spring Boot.
Related Article: How to Generate Random Integers in a Range in Java
What is the purpose of JSON Web Tokens (JWT) in security?
JSON Web Tokens (JWT) are a popular method for securely transmitting information between parties as a JSON object. They are widely used for authentication and authorization in modern web applications.
The purpose of JWTs in security is to provide a secure and scalable way to authenticate and authorize users in distributed systems. JWTs have become a popular choice for authentication because they are self-contained, stateless, and do not require server-side session storage.
Here are some key purposes of JWTs in security:
1. Authentication: JWTs can be used to authenticate users by including user information in the token's payload. Once a user is authenticated, they can include the JWT in subsequent requests to access protected resources without the need for re-authentication.
2. Authorization: JWTs can be used to authorize access to specific resources or perform certain actions. The claims in the JWT's payload can include information about the user's roles, permissions, or other attributes that can be used to enforce access control rules.
3. Statelessness: JWTs are stateless, meaning that all the necessary information to verify the token is included in the token itself. This eliminates the need for server-side session storage and allows for a more scalable architecture.
4. Scalability: JWTs are widely used in microservices architectures, where multiple services need to authenticate and authorize users. Since JWTs are self-contained, each service can independently verify the token without relying on a centralized authentication server.
5. Interoperability: JWTs are based on open standards and are supported by a wide range of programming languages and frameworks. This makes them easy to implement and integrate with existing systems.
To use JWTs in a Spring Boot application, you need to configure JWT authentication and authorization in your SecurityConfig
class. You can generate and sign JWTs using libraries like jjwt or Nimbus JOSE+JWT, and validate them using Spring Security's built-in JWT support.
Overall, the purpose of JWTs in security is to provide a secure and scalable way to authenticate and authorize users in distributed systems. They are widely used in modern web applications and microservices architectures.
How does OAuth2 work in securing RESTful APIs?
OAuth2 is an open standard for authorization that allows users to grant third-party applications access to their resources without sharing their credentials. It is widely used to secure RESTful APIs and provide secure access to user data.
OAuth2 works by defining a set of roles and interactions between four parties: the resource owner (user), the client (third-party application), the authorization server, and the resource server (the API provider).
The OAuth2 flow typically involves the following steps:
1. User initiates the OAuth2 flow by requesting access to a protected resource on the client application.
2. The client application redirects the user to the authorization server, where the user is prompted to authenticate and authorize the client application.
3. If the user grants authorization, the authorization server generates an access token and redirects the user back to the client application.
4. The client application includes the access token in subsequent requests to the resource server to access protected resources.
5. The resource server verifies the access token with the authorization server and grants or denies access to the requested resource based on the token's validity and the user's permissions.
OAuth2 provides several grant types for different scenarios, including authorization code grant, implicit grant, client credentials grant, and resource owner password credentials grant. Each grant type has its own flow and security considerations.
To secure RESTful APIs with OAuth2 in a Spring Boot application, you need to configure OAuth2 authentication and authorization in your SecurityConfig
class. You can use Spring Boot's auto-configuration and provide the necessary client credentials in your application.properties or application.yml file.
Spring Security provides built-in support for OAuth2 authentication and authorization, making it easy to secure your RESTful APIs. You can also create custom OAuth2 providers or integrate with popular identity providers like Google, Facebook, or GitHub.
Overall, OAuth2 provides a secure and scalable way to secure RESTful APIs and allow users to grant third-party applications access to their resources. It allows for fine-grained access control and reduces the need for separate authentication systems.
Common Web Vulnerabilities and Spring Security
Web applications are susceptible to various security vulnerabilities that can be exploited by attackers. Spring Security provides built-in protection against common web vulnerabilities, helping to secure your applications.
Here are some common web vulnerabilities and how Spring Security addresses them:
1. Cross-Site Request Forgery (CSRF): Spring Security includes built-in protection against CSRF attacks. It generates and validates CSRF tokens to prevent malicious users from performing actions on behalf of authenticated users. You can configure CSRF protection by using the csrf()
method in your SecurityConfig
class.
2. Cross-Site Scripting (XSS): Spring Security helps protect your application from XSS attacks by automatically escaping user input and providing features like Content Security Policy (CSP) and XSS prevention headers. You can configure XSS protection by using the headers()
method in your SecurityConfig
class.
3. SQL Injection: Spring Security does not directly address SQL injection vulnerabilities. However, Spring Data JPA and other Spring modules provide features like parameterized queries and object-relational mapping that help prevent SQL injection attacks. It's important to properly sanitize and validate user input to mitigate the risk of SQL injection.
4. Session Fixation: Spring Security manages user sessions and provides protection against session fixation attacks. It generates a new session ID after successful authentication, preventing attackers from hijacking an existing session. Session fixation protection is enabled by default in Spring Security.
5. Clickjacking: Spring Security helps protect against clickjacking attacks by providing features like X-Frame-Options header and Content Security Policy (CSP). These features prevent malicious websites from embedding your application in a frame or iframe. You can configure clickjacking protection by using the headers()
method in your SecurityConfig
class.
These are just a few examples of common web vulnerabilities that Spring Security addresses. It's important to stay updated with the latest security best practices and regularly review and update your application's security measures.
Examples of Custom Authentication Providers in Spring Security
Spring Security allows you to create custom authentication providers to authenticate users using your own logic or integrate with external authentication systems.
Here are two examples of custom authentication providers in Spring Security:
1. Custom UserDetailsService:
@Component public class CustomUserDetailsService implements UserDetailsService { @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { // Retrieve user details from your database or external system User user = userRepository.findByUsername(username) .orElseThrow(() -> new UsernameNotFoundException("User not found")); return new org.springframework.security.core.userdetails.User( user.getUsername(), user.getPassword(), user.getAuthorities() ); } }
In this example, we have a CustomUserDetailsService
class that implements the UserDetailsService
interface. The loadUserByUsername
method retrieves user details from a database or external system and returns a UserDetails
object. This custom authentication provider integrates with an existing user repository.
To use the custom authentication provider, you need to configure it in your SecurityConfig
class:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private CustomUserDetailsService userDetailsService; @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.userDetailsService(userDetailsService); } }
2. Custom LDAP Authentication:
@Component public class CustomLdapAuthenticationProvider implements AuthenticationProvider { @Override public Authentication authenticate(Authentication authentication) throws AuthenticationException { // Retrieve user credentials from the authentication object String username = authentication.getName(); String password = authentication.getCredentials().toString(); // Authenticate against your LDAP server // ... // Return an Authentication object if the user is valid return new UsernamePasswordAuthenticationToken(username, password, new ArrayList()); } @Override public boolean supports(Class authentication) { return authentication.equals(UsernamePasswordAuthenticationToken.class); } }
In this example, we have a CustomLdapAuthenticationProvider
class that implements the AuthenticationProvider
interface. The authenticate
method retrieves user credentials from the authentication object and performs the necessary authentication against an LDAP server.
To use the custom authentication provider, you need to configure it in your SecurityConfig
class:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private CustomLdapAuthenticationProvider authenticationProvider; @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.authenticationProvider(authenticationProvider); } }
These are just two examples of custom authentication providers in Spring Security. You can create custom authentication providers to authenticate users using your own logic or integrate with external authentication systems. Custom authentication providers give you full control over the authentication process and allow you to implement complex authentication mechanisms.
Related Article: Java Serialization: How to Serialize Objects
The Role of Success Handlers in Spring Security
Success handlers in Spring Security allow you to customize the behavior after a successful authentication. They are invoked when a user successfully authenticates and can be used to redirect the user to a specific page, display a success message, or perform other actions.
However, you can create custom success handlers to override this default behavior and provide your own logic. Custom success handlers are useful when you want to redirect the user to a specific page, display a success message, or execute custom actions after authentication.
To create a custom success handler, you need to implement the AuthenticationSuccessHandler
interface and override the onAuthenticationSuccess
method. Here's an example:
@Component public class CustomSuccessHandler implements AuthenticationSuccessHandler { @Override public void onAuthenticationSuccess(HttpServletRequest request, HttpServletResponse response, Authentication authentication) throws IOException, ServletException { // Custom logic to handle successful authentication // For example, redirect the user to a specific page or display a success message response.sendRedirect("/dashboard"); } }
In this example, we have a CustomSuccessHandler
class that implements the AuthenticationSuccessHandler
interface. The onAuthenticationSuccess
method redirects the user to the /dashboard
page after a successful authentication.
To use the custom success handler, you need to configure it in your SecurityConfig
class:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private CustomSuccessHandler successHandler; @Override protected void configure(HttpSecurity http) throws Exception { http .formLogin() .successHandler(successHandler) .and() .logout(); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the form login to use our custom success handler. After a successful authentication, the user will be redirected to the specified page.
Overall, success handlers in Spring Security allow you to customize the behavior after a successful authentication. They give you the flexibility to perform actions like redirecting the user to a specific page, displaying a success message, or executing custom logic.
Securing RESTful APIs with JWT and OAuth2 using Spring Boot
Securing RESTful APIs is crucial to protect sensitive data and ensure that only authorized clients can access the APIs. In Spring Boot, you can use a combination of JSON Web Tokens (JWT) and OAuth2 to secure your RESTful APIs.
JWT provides a secure method for transmitting information between parties as a JSON object. It can be used for authentication and authorization in RESTful APIs. OAuth2, on the other hand, is an authorization framework that allows users to grant third-party applications access to their resources without sharing their credentials.
Here's an example of how to secure RESTful APIs with JWT and OAuth2 using Spring Boot:
1. Configure JWT Authentication:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .csrf().disable() .authorizeRequests() .antMatchers("/api/public/**").permitAll() .anyRequest().authenticated() .and() .addFilter(new JwtAuthenticationFilter(authenticationManager())) .addFilter(new JwtAuthorizationFilter(authenticationManager())); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the HTTP security of our application. It specifies that requests to /api/public/**
should be allowed without authentication, while all other requests require authentication.
The JwtAuthenticationFilter
and JwtAuthorizationFilter
are custom filters that handle JWT authentication and authorization, respectively. These filters extract the JWT from the request, validate it, and set the authenticated user in the Spring Security context.
2. Configure OAuth2 Authorization:
@Configuration @EnableAuthorizationServer public class OAuth2Config extends AuthorizationServerConfigurerAdapter { @Autowired private AuthenticationManager authenticationManager; @Override public void configure(ClientDetailsServiceConfigurer clients) throws Exception { clients .inMemory() .withClient("client") .secret("secret") .authorizedGrantTypes("authorization_code", "refresh_token") .scopes("read", "write") .redirectUris("http://localhost:8080/login/oauth2/code/") .accessTokenValiditySeconds(3600) .refreshTokenValiditySeconds(86400); } @Override public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception { endpoints.authenticationManager(authenticationManager); } }
In this example, we have an OAuth2Config
class that extends AuthorizationServerConfigurerAdapter
. The configure
method configures the OAuth2 authorization server. It specifies the client details, grant types, and scopes for OAuth2 clients. It also configures the authentication manager used for OAuth2 authentication.
3. Secure RESTful APIs:
@RestController @RequestMapping("/api") public class MyController { @GetMapping("/public") public String publicResource() { return "This is a public resource"; } @GetMapping("/private") public String privateResource() { return "This is a private resource"; } }
In this example, we have a MyController
class that defines two API endpoints: /api/public
and /api/private
. The /api/public
endpoint is accessible without authentication, while the /api/private
endpoint requires authentication.
To access the protected /api/private
endpoint, clients need to include a valid JWT in the Authorization
header of their requests.
Overall, securing RESTful APIs with JWT and OAuth2 using Spring Boot provides a secure and scalable way to authenticate and authorize clients. JWTs are used for authentication, while OAuth2 is used for authorization. This combination allows you to protect sensitive data and ensure that only authorized clients can access the APIs.
Preventing Cross-Site Request Forgery (CSRF) Attacks with Spring Security
Cross-Site Request Forgery (CSRF) is a security vulnerability that allows an attacker to trick a user into performing unwanted actions on a website in which they are authenticated. This vulnerability occurs when an attacker can force a user's browser to send a malicious request to a vulnerable website.
Spring Security provides built-in protection against CSRF attacks. It generates and validates CSRF tokens to prevent malicious users from performing actions on behalf of authenticated users.
To enable CSRF protection in a Spring Boot application, you need to configure it in your SecurityConfig
class. Here's an example:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .csrf().disable() .authorizeRequests() .antMatchers("/api/public/**").permitAll() .anyRequest().authenticated() .and() .formLogin() .loginPage("/login") .defaultSuccessUrl("/dashboard") .permitAll() .and() .logout() .logoutUrl("/logout") .permitAll(); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the HTTP security of our application. It specifies that requests to /api/public/**
should be allowed without authentication, while all other requests require authentication.
The csrf().disable()
method disables CSRF protection. This is done for simplicity in this example, but in a real-world application, you should keep CSRF protection enabled.
To ensure that CSRF protection is enabled on all forms in your application, you need to include the CSRF token in your form templates. Here's an example using Thymeleaf:
<!-- Other form fields --> <button type="submit">Submit</button>
In this example, we include a hidden input field with the CSRF token. When the form is submitted, the browser automatically includes the CSRF token in the request headers, and Spring Security validates it.
Spring Security's built-in CSRF protection helps prevent Cross-Site Request Forgery attacks in Spring Boot applications. It generates and validates CSRF tokens to ensure that only legitimate requests are processed.
Preventing Cross-Site Scripting (XSS) Attacks with Spring Security
Cross-Site Scripting (XSS) is a security vulnerability that allows an attacker to inject malicious scripts into web pages viewed by other users. This vulnerability occurs when a website does not properly validate and sanitize user input, allowing the execution of arbitrary code in the context of the vulnerable webpage.
Spring Security helps protect your application from XSS attacks by automatically escaping user input and providing features like Content Security Policy (CSP) and XSS prevention headers.
To enable XSS protection in a Spring Boot application, you need to configure it in your SecurityConfig
class. Here's an example:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .headers() .xssProtection().block(false) .contentSecurityPolicy("default-src 'self'"); } }
In this example, we have a SecurityConfig
class that extends WebSecurityConfigurerAdapter
. The configure
method configures the HTTP security of our application. It enables XSS protection by disabling the XSS protection header's blocking mode. It also sets the Content Security Policy (CSP) to only allow resources from the same origin.
Spring Security automatically escapes user input when rendering views, preventing most XSS attacks. However, it's important to also properly sanitize user input before storing or displaying it in your application.
Additionally, you can use other security headers like X-Content-Type-Options
, X-Frame-Options
, and X-XSS-Protection
to further enhance the security of your application.
Overall, Spring Security's built-in XSS protection helps prevent Cross-Site Scripting attacks in Spring Boot applications. It automatically escapes user input and provides features like Content Security Policy and XSS prevention headers.