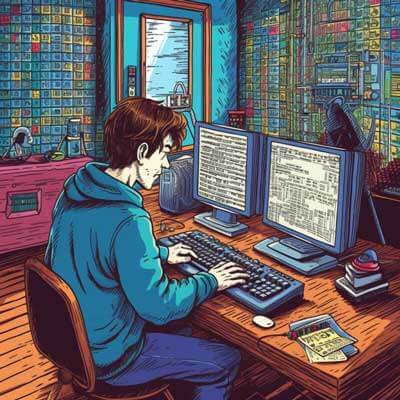
Table of Contents
The @JsonProperty
annotation is a useful tool in Java for customizing the serialization and deserialization process of JSON objects. It allows you to map a Java object's field or property to a specific JSON property, providing flexibility and control over the JSON representation.
Purpose of @JsonProperty
The main purpose of the @JsonProperty
annotation is to define a custom name for a Java object's field or property when it is serialized to JSON or deserialized from JSON. By default, the name of the Java field or property is used as the corresponding JSON property name. However, there may be cases where you want to use a different name in the JSON representation.
For example, consider a Java class Person
with a field firstName
that you want to serialize as first_name
in the resulting JSON. You can achieve this by using the @JsonProperty
annotation:
public class Person { @JsonProperty("first_name") private String firstName; // getter and setter methods }
In this example, the firstName
field will be serialized as first_name
in the JSON representation.
Related Article: How to Generate Random Numbers in Java
Usage of @JsonProperty
The @JsonProperty
annotation can be used in various ways to customize the serialization and deserialization process. Here are some common use cases:
1. Mapping Java fields to JSON properties
You can use the @JsonProperty
annotation to map a Java field to a specific JSON property. This is useful when the names of the Java fields and JSON properties are different.
public class Person { @JsonProperty("first_name") private String firstName; // getter and setter methods }
In this example, the firstName
field will be serialized as first_name
in the JSON representation.
2. Ignoring a Java field during serialization or deserialization
Sometimes, you may want to exclude a specific Java field from the serialization or deserialization process. This can be achieved by using the @JsonIgnore
annotation along with @JsonProperty
.
public class Person { @JsonIgnore private String secretField; @JsonProperty("public_field") private String publicField; // getter and setter methods }
In this example, the secretField
will be ignored during serialization and deserialization, while the publicField
will be serialized and deserialized as public_field
in the JSON representation.
3. Handling optional JSON properties
If a JSON property is optional and may or may not be present in the JSON payload, you can use the required
attribute of the @JsonProperty
annotation to handle such cases.
public class Person { @JsonProperty(value = "first_name", required = false) private String firstName; // getter and setter methods }
In this example, the firstName
field is marked as not required. During deserialization, if the first_name
JSON property is missing, the firstName
field will be assigned a null value.
4. Handling default values
You can also specify a default value for a Java field when the corresponding JSON property is missing using the defaultValue
attribute of the @JsonProperty
annotation.
public class Person { @JsonProperty(value = "age", defaultValue = "18") private int age; // getter and setter methods }
In this example, if the age
JSON property is missing, the age
field will be assigned a default value of 18 during deserialization.
5. Customizing property order
The order in which properties are serialized or deserialized can be customized using the index
attribute of the @JsonProperty
annotation.
public class Person { @JsonProperty(value = "first_name", index = 1) private String firstName; @JsonProperty(value = "last_name", index = 2) private String lastName; // getter and setter methods }
In this example, the firstName
field will be serialized or deserialized before the lastName
field, as specified by the index
attribute.
Best Practices
Related Article: Java String Substring Tutorial
When using the @JsonProperty
annotation, it is recommended to follow these best practices:
- Use meaningful and descriptive names for both Java fields and JSON properties to improve code readability.
- Keep the mapping between Java fields and JSON properties consistent and intuitive to avoid confusion.
- Document the purpose and usage of the @JsonProperty
annotations in your code to make it clear how the JSON serialization and deserialization is customized.