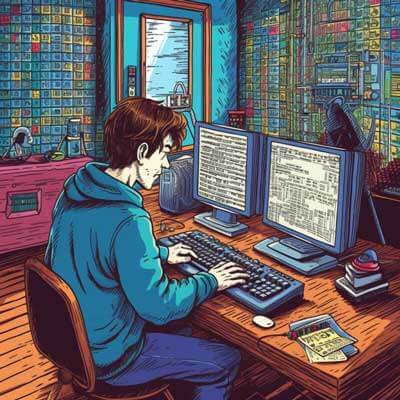
Table of Contents
Introduction to Design Patterns
Design patterns are reusable solutions to common problems that occur in software design and development. They provide a way to solve these problems in a structured and efficient manner. In this chapter, we will introduce the concept of design patterns and discuss their importance in software engineering.
Design patterns can be categorized into three main types: creational, structural, and behavioral. Creational patterns focus on object creation mechanisms, structural patterns deal with the composition of classes and objects, and behavioral patterns handle the interaction between objects and the delegation of responsibilities.
Let's take a look at a couple of examples to illustrate the use of design patterns in Java.
Related Article: Spring Boot Integration with Payment, Communication Tools
Example 1: Singleton Pattern
The Singleton pattern ensures that only a single instance of a class is created and provides a global point of access to it. This pattern is useful when you want to limit the number of instances of a class and ensure that there is only one instance throughout the application.
Here's an example of how to implement the Singleton pattern in Java:
public class Singleton { private static Singleton instance; private Singleton() { // Private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; }}
In this example, the Singleton class has a private constructor to prevent direct instantiation. The class also has a static method, getInstance()
, which returns the single instance of the class. The getInstance()
method uses double-checked locking to ensure thread-safety in a multi-threaded environment.
Example 2: Factory Pattern
The Factory pattern provides a way to create objects without specifying their exact classes. It encapsulates the object creation logic and allows the client to use the created objects without being aware of their concrete types.
Here's an example of how to implement the Factory pattern in Java:
public interface Animal { void makeSound();}public class Dog implements Animal { @Override public void makeSound() { System.out.println("Woof!"); }}public class Cat implements Animal { @Override public void makeSound() { System.out.println("Meow!"); }}public class AnimalFactory { public Animal createAnimal(String type) { if (type.equalsIgnoreCase("dog")) { return new Dog(); } else if (type.equalsIgnoreCase("cat")) { return new Cat(); } else { throw new IllegalArgumentException("Invalid animal type: " + type); } }}
In this example, we have an interface Animal
and two concrete classes Dog
and Cat
that implement the Animal
interface. The AnimalFactory
class has a method createAnimal()
that takes a string parameter indicating the type of animal to create. It returns an instance of the appropriate concrete class based on the provided type.
These examples demonstrate how design patterns can be used to solve specific problems in software design. In the following chapters, we will explore different design patterns in more detail and discuss their use cases, best practices, and real-world examples.
Structural Design Patterns Overview
Structural design patterns deal with the composition of classes and objects to form larger structures. They help ensure that the components of a system are structured in a flexible and scalable way. In this chapter, we will provide an overview of some commonly used structural design patterns in Java.
Related Article: How to Implement a Delay in Java Using java wait seconds
Adapter Pattern
The Adapter pattern allows objects with incompatible interfaces to collaborate. It acts as a bridge between two incompatible interfaces, converting the interface of one class into another interface that clients expect.
Here's an example of how to implement the Adapter pattern in Java:
public interface MediaPlayer { void play(String audioType, String fileName);}public interface AdvancedMediaPlayer { void playVlc(String fileName); void playMp4(String fileName);}public class VlcPlayer implements AdvancedMediaPlayer { @Override public void playVlc(String fileName) { System.out.println("Playing VLC file: " + fileName); } @Override public void playMp4(String fileName) { // Do nothing }}public class Mp4Player implements AdvancedMediaPlayer { @Override public void playVlc(String fileName) { // Do nothing } @Override public void playMp4(String fileName) { System.out.println("Playing MP4 file: " + fileName); }}public class MediaAdapter implements MediaPlayer { private AdvancedMediaPlayer advancedMediaPlayer; public MediaAdapter(String audioType) { if (audioType.equalsIgnoreCase("vlc")) { advancedMediaPlayer = new VlcPlayer(); } else if (audioType.equalsIgnoreCase("mp4")) { advancedMediaPlayer = new Mp4Player(); } } @Override public void play(String audioType, String fileName) { if (audioType.equalsIgnoreCase("vlc")) { advancedMediaPlayer.playVlc(fileName); } else if (audioType.equalsIgnoreCase("mp4")) { advancedMediaPlayer.playMp4(fileName); } }}public class AudioPlayer implements MediaPlayer { private MediaAdapter mediaAdapter; @Override public void play(String audioType, String fileName) { if (audioType.equalsIgnoreCase("mp3")) { System.out.println("Playing MP3 file: " + fileName); } else if (audioType.equalsIgnoreCase("vlc") || audioType.equalsIgnoreCase("mp4")) { mediaAdapter = new MediaAdapter(audioType); mediaAdapter.play(audioType, fileName); } else { System.out.println("Invalid media type: " + audioType); } }}
In this example, we have an interface MediaPlayer
that defines the play()
method. The AdvancedMediaPlayer
interface defines two methods, playVlc()
and playMp4()
. The VlcPlayer
and Mp4Player
classes implement the AdvancedMediaPlayer
interface.
The MediaAdapter
class acts as an adapter between the MediaPlayer
interface and the AdvancedMediaPlayer
interface. It takes the audio type as a parameter and creates an instance of the appropriate AdvancedMediaPlayer
implementation.
The AudioPlayer
class is the client that uses the MediaPlayer
interface. When the play()
method is called, it checks the audio type and creates an instance of the MediaAdapter
if the audio type is either "vlc" or "mp4". Otherwise, it plays the MP3 file directly.
This example demonstrates how the Adapter pattern can be used to play different types of audio files using a common interface.
Composite Pattern
The Composite pattern allows you to compose objects into tree structures to represent part-whole hierarchies. It lets clients treat individual objects and compositions of objects uniformly.
Here's an example of how to implement the Composite pattern in Java:
public interface Shape { void draw();}public class Circle implements Shape { @Override public void draw() { System.out.println("Drawing Circle"); }}public class Square implements Shape { @Override public void draw() { System.out.println("Drawing Square"); }}public class Triangle implements Shape { @Override public void draw() { System.out.println("Drawing Triangle"); }}public class Drawing implements Shape { private List<Shape> shapes = new ArrayList<>(); public void addShape(Shape shape) { shapes.add(shape); } public void removeShape(Shape shape) { shapes.remove(shape); } @Override public void draw() { for (Shape shape : shapes) { shape.draw(); } }}
In this example, we have an interface Shape
that defines the draw()
method. The Circle
, Square
, and Triangle
classes implement the Shape
interface.
The Drawing
class represents a composition of shapes. It has a list of shapes and provides methods to add and remove shapes. The draw()
method of the Drawing
class iterates over all the shapes and calls their draw()
methods.
This example demonstrates how the Composite pattern can be used to treat individual shapes and collections of shapes in a uniform manner.
These examples provide a glimpse into the world of structural design patterns in Java. In the following chapters, we will delve deeper into each of these patterns, discussing their use cases, best practices, real-world examples, and performance considerations.
Creational Design Patterns Overview
Creational design patterns focus on object creation mechanisms, providing ways to create objects in a flexible and decoupled manner. In this chapter, we will provide an overview of some commonly used creational design patterns in Java.
Singleton Pattern
The Singleton pattern ensures that only a single instance of a class is created and provides a global point of access to it. This pattern is useful when you want to limit the number of instances of a class and ensure that there is only one instance throughout the application.
Here's an example of how to implement the Singleton pattern in Java:
public class Singleton { private static Singleton instance; private Singleton() { // Private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; }}
In this example, the Singleton class has a private constructor to prevent direct instantiation. The class also has a static method, getInstance()
, which returns the single instance of the class. The getInstance()
method uses double-checked locking to ensure thread-safety in a multi-threaded environment.
Related Article: PHP vs Java: A Practical Comparison
Factory Pattern
The Factory pattern provides a way to create objects without specifying their exact classes. It encapsulates the object creation logic and allows the client to use the created objects without being aware of their concrete types.
Here's an example of how to implement the Factory pattern in Java:
public interface Animal { void makeSound();}public class Dog implements Animal { @Override public void makeSound() { System.out.println("Woof!"); }}public class Cat implements Animal { @Override public void makeSound() { System.out.println("Meow!"); }}public class AnimalFactory { public Animal createAnimal(String type) { if (type.equalsIgnoreCase("dog")) { return new Dog(); } else if (type.equalsIgnoreCase("cat")) { return new Cat(); } else { throw new IllegalArgumentException("Invalid animal type: " + type); } }}
In this example, we have an interface Animal
and two concrete classes Dog
and Cat
that implement the Animal
interface. The AnimalFactory
class has a method createAnimal()
that takes a string parameter indicating the type of animal to create. It returns an instance of the appropriate concrete class based on the provided type.
These examples provide a glimpse into the world of creational design patterns in Java. In the following chapters, we will delve deeper into each of these patterns, discussing their use cases, best practices, real-world examples, and performance considerations.
Behavioral Design Patterns Overview
Behavioral design patterns deal with the interaction between objects and the delegation of responsibilities. They help define communication patterns between objects and simplify the design of complex systems. In this chapter, we will provide an overview of some commonly used behavioral design patterns in Java.
Observer Pattern
The Observer pattern defines a one-to-many dependency between objects, where the state change of one object triggers the update of its dependent objects. It provides a way to notify multiple objects about any changes in the observed object.
Here's an example of how to implement the Observer pattern in Java:
import java.util.ArrayList;import java.util.List;public interface Observer { void update(String message);}public interface Subject { void attach(Observer observer); void detach(Observer observer); void notifyObservers(String message);}public class ConcreteSubject implements Subject { private List<Observer> observers = new ArrayList<>(); @Override public void attach(Observer observer) { observers.add(observer); } @Override public void detach(Observer observer) { observers.remove(observer); } @Override public void notifyObservers(String message) { for (Observer observer : observers) { observer.update(message); } }}public class ConcreteObserver implements Observer { private String name; public ConcreteObserver(String name) { this.name = name; } @Override public void update(String message) { System.out.println(name + " received message: " + message); }}
In this example, we have the Observer
interface that defines the update()
method, and the Subject
interface that defines the attach()
, detach()
, and notifyObservers()
methods. The ConcreteSubject
class implements the Subject
interface and maintains a list of observers. The ConcreteObserver
class implements the Observer
interface and defines how it handles the update message.
The ConcreteSubject
class notifies all its observers when a state change occurs by calling their update()
methods.
Strategy Pattern
The Strategy pattern allows you to define a family of algorithms, encapsulate each one as a separate class, and make them interchangeable at runtime. It provides a way to select an algorithm dynamically based on the specific context or requirements.
Here's an example of how to implement the Strategy pattern in Java:
public interface SortAlgorithm { void sort(int[] array);}public class BubbleSort implements SortAlgorithm { @Override public void sort(int[] array) { // Bubble sort implementation }}public class QuickSort implements SortAlgorithm { @Override public void sort(int[] array) { // Quick sort implementation }}public class Sorter { private SortAlgorithm sortAlgorithm; public Sorter(SortAlgorithm sortAlgorithm) { this.sortAlgorithm = sortAlgorithm; } public void setSortAlgorithm(SortAlgorithm sortAlgorithm) { this.sortAlgorithm = sortAlgorithm; } public void sort(int[] array) { sortAlgorithm.sort(array); }}
In this example, we have the SortAlgorithm
interface that defines the sort()
method. The BubbleSort
and QuickSort
classes implement the SortAlgorithm
interface with their respective sorting algorithms.
The Sorter
class uses composition to encapsulate the selected sorting algorithm. It has a method sort()
that delegates the sorting operation to the chosen SortAlgorithm
implementation.
This example demonstrates how the Strategy pattern can be used to dynamically select different sorting algorithms.
These examples provide a glimpse into the world of behavioral design patterns in Java. In the following chapters, we will delve deeper into each of these patterns, discussing their use cases, best practices, real-world examples, and performance considerations.
Related Article: How to Generate Random Numbers in Java
Singleton Pattern: Use Cases
The Singleton pattern is a creational design pattern that ensures that only a single instance of a class is created and provides a global point of access to it. This pattern is useful in various scenarios where you want to limit the number of instances of a class and ensure that there is only one instance throughout the application.
Here are some common use cases where the Singleton pattern can be applied:
1. Database Connection Pool: In applications that require database connections, a Singleton pattern can be used to manage a pool of database connections. The Singleton instance can handle the creation, management, and distribution of connections, ensuring efficient and controlled access to the database.
2. Configuration Settings: Singleton can be used to provide access to global configuration settings, such as application properties, environment variables, or user preferences. The Singleton instance can load and cache the configuration settings, making them accessible from anywhere in the application.
3. Logging: Singleton can be used to encapsulate a logging service that provides centralized logging functionality throughout the application. The Singleton instance can handle the initialization, configuration, and management of the logging service, ensuring consistent logging behavior.
4. Caching: Singleton can be used to implement a cache for frequently accessed data or expensive operations. The Singleton instance can manage the cache, including data insertion, retrieval, and eviction strategies. This can improve the performance of the application by reducing expensive computations or database queries.
These are just a few examples of the use cases for the Singleton pattern. The key idea is to use the Singleton pattern when you need to ensure that there is only one instance of a class and provide a global point of access to that instance.
Factory Pattern: Use Cases
The Factory pattern is a creational design pattern that provides a way to create objects without specifying their exact classes. It encapsulates the object creation logic and allows the client to use the created objects without being aware of their concrete types. The Factory pattern is useful in various scenarios where you want to decouple the client code from the specific implementation of the created objects.
Here are some common use cases where the Factory pattern can be applied:
1. Object Creation: When you have a complex object creation process involving multiple steps or dependencies, the Factory pattern can simplify the object creation code. The factory class can encapsulate the creation logic and handle the instantiation of the object, abstracting away the complexity from the client code.
2. Dependency Injection: In applications that use dependency injection frameworks, the Factory pattern can be used to provide the dependencies to the client classes. The factory class can create and inject the required dependencies based on the configuration or runtime conditions, allowing for flexible and dynamic dependency management.
3. Plugin System: The Factory pattern can be used to implement a plugin system where the client code needs to dynamically load and use different implementations of a common interface. The factory class can provide a way to discover, instantiate, and provide the appropriate plugin implementation based on the specific requirements or configurations.
4. Object Pooling: When you need to manage a pool of reusable objects, the Factory pattern can be used to create and manage the objects in the pool. The factory class can handle object creation, recycling, and maintenance, ensuring efficient and controlled access to the pooled objects.
These are just a few examples of the use cases for the Factory pattern. The key idea is to use the Factory pattern when you want to decouple the client code from the specific implementation of the created objects and provide a way to create objects in a flexible and abstract manner.
Decorator Pattern: Use Cases
The Decorator pattern is a structural design pattern that allows behavior to be added to an individual object dynamically, without affecting the behavior of other objects from the same class. It provides a way to extend the functionality of an object by wrapping it with one or more decorator objects. The Decorator pattern is useful in various scenarios where you want to add or modify the behavior of objects at runtime.
Here are some common use cases where the Decorator pattern can be applied:
1. Adding Responsibilities: When you want to add additional responsibilities or behaviors to an object dynamically, the Decorator pattern can be used. Each decorator class can add specific functionality to the wrapped object without affecting other objects of the same class. This allows for flexible and dynamic extension of an object's behavior.
2. Transparently Wrapping Objects: The Decorator pattern can be used to wrap objects transparently, without the client code being aware of the underlying decoration. This is useful when you want to modify the behavior of an object without changing its interface or impacting existing client code.
3. Dynamic Composition: The Decorator pattern allows for dynamic composition of objects at runtime. It provides a way to combine multiple decorators in different combinations to achieve the desired behavior. This allows for flexible and fine-grained control over the behavior of an object.
4. Legacy Code Integration: When integrating legacy code or third-party libraries into a new system, the Decorator pattern can be used to add additional functionality or adapt the existing code to meet the requirements of the new system. The decorator classes can wrap the legacy code and add the necessary modifications or enhancements.
These are just a few examples of the use cases for the Decorator pattern. The key idea is to use the Decorator pattern when you want to add or modify the behavior of objects dynamically and in a flexible manner, without affecting other objects or changing the existing code structure.
Singleton Pattern: Best Practices
The Singleton pattern is a widely used creational design pattern that ensures that only a single instance of a class is created and provides a global point of access to it. While the Singleton pattern can be useful in various scenarios, it should be used judiciously, considering the specific requirements and constraints of the application. Here are some best practices to keep in mind when using the Singleton pattern:
1. Thread Safety: Ensure that the Singleton implementation is thread-safe to prevent issues in multi-threaded environments. Consider using double-checked locking or other synchronization mechanisms to handle concurrent access to the Singleton instance.
2. Lazy Initialization: Consider using lazy initialization to create the Singleton instance only when it is actually needed. This can improve performance by avoiding unnecessary instantiation of the Singleton object.
3. Eager vs. Lazy Initialization: Choose between eager and lazy initialization based on the specific requirements of the application. Eager initialization creates the Singleton instance at the time of class loading, while lazy initialization creates the instance when it is first requested. Eager initialization provides immediate access to the Singleton instance but may result in unnecessary object creation, while lazy initialization defers object creation until it is needed but may introduce a slight overhead when accessing the Singleton instance.
4. Serialization: Consider implementing the Serializable interface and providing appropriate readResolve() method in the Singleton class to ensure correct serialization and deserialization of the Singleton instance. This is important if the Singleton instance needs to be serialized and restored across different JVMs or persisted in a storage medium.
5. Dependency Injection: Avoid using Singleton as a global state container or a replacement for proper dependency injection. Instead, consider using dependency injection frameworks or other appropriate mechanisms to manage dependencies and provide the necessary objects to the client classes.
6. Unit Testing: Design the Singleton class in such a way that it can be easily tested in isolation. Consider using dependency injection or other techniques to decouple the Singleton from its dependencies, allowing for easier unit testing of the client code.
7. Clear Documentation: Clearly document the intent and usage of the Singleton class to prevent misuse or misunderstanding by other developers. Make it clear that the class is intended to have a single instance and provide guidelines on how to access and use the Singleton instance correctly.
Related Article: How to Read a JSON File in Java Using the Simple JSON Lib
Factory Pattern: Best Practices
The Factory pattern is a creational design pattern that provides a way to create objects without specifying their exact classes. It encapsulates the object creation logic and allows the client to use the created objects without being aware of their concrete types. When using the Factory pattern, consider the following best practices:
1. Clear Separation of Concerns: Ensure that the factory class has a clear separation of concerns and is responsible only for creating objects. Avoid mixing object creation logic with other responsibilities, such as business logic or data manipulation. This promotes better maintainability and flexibility.
2. Encapsulation of Object Creation: Encapsulate the object creation logic within the factory class by providing dedicated factory methods or factory interfaces. This abstracts away the details of object creation, making the client code less dependent on specific implementations.
3. Use of Interfaces: Prefer using interfaces or abstract classes to define the factory method or factory interface. This allows for easier substitution of different factory implementations and promotes loose coupling between the client code and the concrete factory classes.
4. Selective Object Creation: Consider providing multiple factory methods or overloaded factory methods to create objects with different configurations or parameters. This allows for more flexibility and customization when creating objects.
5. Dependency Management: Consider using dependency injection or other appropriate mechanisms to manage dependencies between objects. This can help decouple the client code from the factory class and make the code more testable and maintainable.
6. Error Handling: Handle the scenarios where the factory class is unable to create the requested object gracefully. Consider throwing meaningful exceptions or returning default objects to handle such scenarios.
7. Documentation: Clearly document the intent and usage of the factory class, including the available factory methods, supported object types, and any restrictions or limitations. This helps other developers understand how to use the factory class correctly.
Decorator Pattern: Best Practices
The Decorator pattern is a structural design pattern that allows behavior to be added to an individual object dynamically, without affecting the behavior of other objects from the same class. When using the Decorator pattern, consider the following best practices:
1. Clear Separation of Concerns: Ensure that the decorator classes have a clear separation of concerns and are responsible only for adding or modifying specific behaviors. Avoid mixing decoration logic with other responsibilities, such as object creation or data manipulation. This promotes better maintainability and flexibility.
2. Interface Compliance: Decorator classes should implement the same interface or extend the same abstract class as the object being decorated. This ensures that the decorators can be used interchangeably with the original object and provides a consistent interface for the client code.
3. Transparent Decoration: The decoration should be transparent to the client code. The client code should not be aware of the specific decorators being applied to the object. This allows for easier integration and modification of the object's behavior without impacting the existing code structure.
4. Dynamic Composition: The Decorator pattern allows for dynamic composition of objects at runtime. Consider providing a way to dynamically add or remove decorators to achieve the desired behavior. This provides flexibility and fine-grained control over the behavior of the decorated object.
5. Order of Decoration: Consider the order in which decorators are applied. The order of decoration can affect the final behavior of the object. Make sure that the decorators are applied in a consistent and logical order to achieve the desired behavior.
6. Avoid Overlapping Decorators: Avoid creating decorators that overlap in terms of functionality. Each decorator should add or modify a unique behavior of the object. Overlapping decorators can lead to unnecessary complexity and confusion.
7. Documentation: Clearly document the intent and usage of the decorator classes, including the specific behaviors they add or modify. This helps other developers understand how to use the decorators correctly and promotes better code comprehension.
Singleton Pattern: Real World Examples
The Singleton pattern is a creational design pattern that ensures that only a single instance of a class is created and provides a global point of access to it. The Singleton pattern can be applied in various real-world scenarios to address specific requirements and constraints. Here are some examples of real-world use cases for the Singleton pattern:
1. Logging: In a logging system, you may want to have a single instance that handles the logging functionality throughout the application. The Singleton instance can provide methods to log messages, manage log levels, and handle log file rotation or other log-related operations.
2. Configuration Settings: In an application that requires global configuration settings, such as application properties or user preferences, a Singleton can be used to provide access to the configuration settings. The Singleton instance can load and cache the configuration settings, making them accessible from anywhere in the application.
3. Database Connection Pool: In applications that require database connections, a Singleton pattern can be used to manage a pool of database connections. The Singleton instance can handle the creation, management, and distribution of connections, ensuring efficient and controlled access to the database.
4. User Session Management: In a web application, you may want to have a single instance that manages user sessions. The Singleton instance can store user session data, handle session expiration, and provide methods to access and manipulate session-related information.
5. Print Spooler: In a print spooling system, you may want to have a single instance that manages the printing of documents. The Singleton instance can handle the queuing of print jobs, manage printer resources, and provide methods to control the printing process.
These examples demonstrate how the Singleton pattern can be applied in real-world scenarios to address specific requirements and constraints. By ensuring that only a single instance of a class is created and providing a global point of access to it, the Singleton pattern can help simplify the design and implementation of various systems.
Factory Pattern: Real World Examples
The Factory pattern is a creational design pattern that provides a way to create objects without specifying their exact classes. It encapsulates the object creation logic and allows the client to use the created objects without being aware of their concrete types. The Factory pattern can be applied in various real-world scenarios to address specific requirements and constraints. Here are some examples of real-world use cases for the Factory pattern:
1. GUI Component Creation: In a graphical user interface (GUI) framework, a factory can be used to create different types of GUI components, such as buttons, labels, or text fields. The factory can abstract away the specific implementation details and provide a common interface for creating and working with GUI components.
2. File Format Conversion: In a file conversion system, a factory can be used to create converters for different file formats. The factory can detect the input file format and dynamically create the appropriate converter object to perform the conversion. This allows for flexible and extensible file format conversion capabilities.
3. Plugin System: In an extensible application or framework, a factory can be used to create and manage plugins. The factory can provide a way to discover, instantiate, and configure the plugins based on the specific requirements or runtime conditions. This allows for dynamic extension of the application's functionality.
4. Dependency Injection: In applications that use dependency injection frameworks, a factory can be used to provide the dependencies to the client classes. The factory can create and inject the required dependencies based on the configuration or runtime conditions, allowing for flexible and dynamic dependency management.
5. Database Object Creation: In an object-relational mapping (ORM) system, a factory can be used to create database objects, such as entity objects or data access objects (DAOs). The factory can abstract away the specific database implementation details and provide a common interface for creating and working with database objects.
These examples demonstrate how the Factory pattern can be applied in real-world scenarios to address specific requirements and constraints. By encapsulating the object creation logic and providing a way to create objects in a flexible and decoupled manner, the Factory pattern promotes better code maintainability and reusability.
Related Article: How to Use Spring Configuration Annotation
Decorator Pattern: Real World Examples
The Decorator pattern is a structural design pattern that allows behavior to be added to an individual object dynamically, without affecting the behavior of other objects from the same class. The Decorator pattern can be applied in various real-world scenarios to add or modify the behavior of objects at runtime. Here are some examples of real-world use cases for the Decorator pattern:
1. Input/Output Streams: In the Java I/O framework, the Decorator pattern is used to add additional functionality to input/output streams. For example, the BufferedInputStream
and BufferedOutputStream
classes add buffering capabilities to the underlying input/output streams, improving performance.
2. Graphical User Interfaces: In GUI frameworks, the Decorator pattern is commonly used to add additional graphical effects or behaviors to user interface components. For example, a decorator can be used to add a border, shadow, or tooltip to a button component without changing the button's original behavior.
3. Encryption and Compression: In cryptography and data compression libraries, the Decorator pattern can be used to add encryption or compression functionality to underlying data streams or objects. Decorators can be stacked to provide multiple layers of encryption or compression with different algorithms or configurations.
4. Logging and Monitoring: In logging and monitoring systems, the Decorator pattern can be used to add additional logging or monitoring capabilities to objects or methods. Decorators can record method invocations, measure performance, or add additional logging information without modifying the original code.
5. Web Application Filters: In web application development, filters are used to intercept and modify incoming or outgoing requests and responses. Filters can be implemented using the Decorator pattern, allowing for flexible and reusable request or response processing logic.
These examples demonstrate how the Decorator pattern can be applied in real-world scenarios to add or modify the behavior of objects dynamically and in a transparent manner. By dynamically extending the functionality of objects, the Decorator pattern provides a flexible and reusable approach to software design.
Performance Considerations: Singleton Pattern
While the Singleton pattern provides a way to ensure that only a single instance of a class is created and provides a global point of access to it, it is important to consider the performance implications of using the Singleton pattern. Here are some performance considerations to keep in mind when using the Singleton pattern:
1. Object Creation Overhead: The Singleton pattern introduces an additional layer of object creation and initialization compared to directly instantiating objects. This can result in a slight overhead in terms of memory usage and CPU cycles, especially during the first creation of the Singleton instance.
2. Thread Safety Overhead: Ensuring thread safety in a multi-threaded environment can introduce additional synchronization overhead. Synchronization mechanisms, such as locks or atomic operations, may impact the performance of accessing the Singleton instance, especially in highly concurrent scenarios.
3. Eager vs. Lazy Initialization: The choice between eager and lazy initialization can have performance implications. Eager initialization creates the Singleton instance at the time of class loading, while lazy initialization defers object creation until it is actually needed. Eager initialization provides immediate access to the Singleton instance but may result in unnecessary object creation, while lazy initialization defers object creation until it is needed but may introduce a slight overhead when accessing the Singleton instance.
4. Serialization and Deserialization Overhead: If the Singleton instance needs to be serialized and deserialized, additional overhead may be incurred. Implementing the Serializable interface and providing appropriate readResolve() method can ensure correct serialization and deserialization of the Singleton instance but may impact performance.
5. Memory Consumption: The Singleton pattern can lead to increased memory consumption if the Singleton instance holds a large amount of data or resources. Care should be taken to manage the memory usage of the Singleton instance and avoid excessive resource consumption.
6. Scalability and Testability: Depending on the specific implementation of the Singleton pattern, it may introduce limitations or challenges when trying to scale the application or test it in isolation. Consider using dependency injection or other techniques to decouple the Singleton from its dependencies and make the code more scalable and testable.
It is important to measure and analyze the performance impact of using the Singleton pattern in the specific context of your application. While the Singleton pattern can provide benefits in terms of code organization and access to a single instance, it should be used judiciously, considering the performance requirements and constraints of the application.
Performance Considerations: Factory Pattern
The Factory pattern provides a way to create objects without specifying their exact classes, but it is important to consider the performance implications of using the Factory pattern. Here are some performance considerations to keep in mind when using the Factory pattern:
1. Object Creation Overhead: The Factory pattern introduces an additional layer of object creation and initialization compared to directly instantiating objects. This can result in a slight overhead in terms of memory usage and CPU cycles, especially if the factory logic is complex or involves external dependencies.
2. Dependency Resolution Overhead: If the factory needs to resolve dependencies or perform additional operations to create objects, this can introduce additional overhead. Dependency resolution mechanisms, such as dependency injection frameworks, may impact the performance of object creation in terms of CPU cycles and memory usage.
3. Flexibility vs. Performance Tradeoff: The Factory pattern provides flexibility and decoupling by abstracting away the specific implementation details of object creation. However, this abstraction can come at the cost of performance, as the factory needs to determine and instantiate the appropriate objects based on the specific requirements or configurations.
4. Eager vs. Lazy Initialization: The choice between eager and lazy initialization can have performance implications. Eager initialization creates objects at the time of factory creation or initialization, while lazy initialization defers object creation until it is actually needed. Eager initialization provides immediate access to the created objects but may result in unnecessary object creation, while lazy initialization defers object creation until it is needed but may introduce a slight overhead when creating the objects.
5. Caching and Object Reuse: Depending on the specific requirements of the application, the Factory pattern can be used to implement object caching or pooling mechanisms. Caching or reusing objects can improve performance by avoiding the overhead of object creation and initialization.
6. Scalability: Depending on the specific implementation of the Factory pattern, it may introduce limitations or challenges when trying to scale the application. Consider using scalable techniques, such as distributed factories or dependency injection frameworks, to handle large-scale object creation and management.
It is important to measure and analyze the performance impact of using the Factory pattern in the specific context of your application. While the Factory pattern provides benefits in terms of flexibility and decoupling, it should be used judiciously, considering the performance requirements and constraints of the application.
Performance Considerations: Decorator Pattern
The Decorator pattern allows behavior to be added to an individual object dynamically, but it is important to consider the performance implications of using the Decorator pattern. Here are some performance considerations to keep in mind when using the Decorator pattern:
1. Object Creation Overhead: The Decorator pattern introduces additional objects and their associated overhead compared to the original object. Each decorator object adds a layer of behavior to the object being decorated, which can result in increased memory usage and CPU cycles, especially when multiple decorators are used.
2. Method Invocation Overhead: Each method invocation on a decorated object may involve additional method calls on the decorators in the chain. This can result in increased method invocation overhead, especially when the decorators perform additional computations or operations.
3. Order of Decoration: The order in which decorators are applied can affect the final behavior and performance of the decorated object. Consider the order of decoration carefully, as some combinations of decorators may introduce unnecessary overhead or conflicts in behavior.
4. Transparent Decoration: The decoration should be transparent to the client code, but it may introduce additional complexity and overhead in terms of object creation and method invocation. Care should be taken to balance the flexibility and transparency of the decoration with the performance requirements of the application.
5. Caching and Memoization: Depending on the specific requirements of the application, caching or memoization techniques can be used to optimize the performance of decorated objects. Caching the results of expensive computations or operations in decorators can improve performance by avoiding unnecessary computations.
6. Scalability: Depending on the specific implementation of the Decorator pattern, it may introduce limitations or challenges when trying to scale the application. Consider using scalable techniques, such as distributed decorators or caching strategies, to handle large-scale object decoration and management.
It is important to measure and analyze the performance impact of using the Decorator pattern in the specific context of your application. While the Decorator pattern provides benefits in terms of flexibility and dynamic behavior modification, it should be used judiciously, considering the performance requirements and constraints of the application.
Related Article: Multiple Inheritance In Java: How to Use
Advanced Techniques: Singleton Pattern
The Singleton pattern is a widely used creational design pattern that ensures that only a single instance of a class is created and provides a global point of access to it. While the basic implementation of the Singleton pattern is straightforward, there are advanced techniques and variations that can be applied to enhance its functionality and address specific requirements. Here are some advanced techniques for implementing the Singleton pattern:
1. Lazy Initialization with Double-Checked Locking: The basic implementation of the Singleton pattern using lazy initialization may not be thread-safe in a multi-threaded environment. To ensure thread safety, the double-checked locking technique can be applied. This technique involves using a synchronized block to check and create the Singleton instance only when it is needed, eliminating unnecessary synchronization overhead in subsequent calls to the getInstance()
method.
2. Initialization-on-demand Holder Idiom: The Initialization-on-demand Holder (IODH) idiom is an advanced technique for implementing the Singleton pattern in a thread-safe manner without using explicit synchronization. It leverages the fact that inner classes are not loaded until they are referenced, and combines it with the initialization of static final fields to ensure that only a single instance of the Singleton class is created.
3. Enum Singleton: In Java, an enum type can be used to implement the Singleton pattern. Enum constants are guaranteed to be instantiated only once by the Java Virtual Machine, making them an effective way to implement singletons. Enum singletons are inherently thread-safe, serializable, and resistant to reflection attacks.
4. Singleton with Dependency Injection: The Singleton pattern can be combined with dependency injection to improve code maintainability and flexibility. Instead of directly accessing the Singleton instance from client code, dependencies can be injected into client classes using dependency injection frameworks or techniques. This allows for easier testing, decoupling of dependencies, and better separation of concerns.
5. Monostate Singleton: The Monostate pattern is an alternative implementation of the Singleton pattern where multiple instances of a class can be created, but they all share the same state. Each instance of the class behaves as if it were a Singleton, with all instances accessing and modifying the same shared state.
These advanced techniques provide additional options and variations for implementing the Singleton pattern. Depending on the specific requirements and constraints of the application, one or more of these techniques can be applied to enhance the functionality and performance of the Singleton instance.
Advanced Techniques: Factory Pattern
The Factory pattern is a creational design pattern that provides a way to create objects without specifying their exact classes. While the basic implementation of the Factory pattern is straightforward, there are advanced techniques and variations that can be applied to enhance its functionality and address specific requirements. Here are some advanced techniques for implementing the Factory pattern:
1. Abstract Factory: The Abstract Factory pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes. It is an extension of the basic Factory pattern that allows for the creation of multiple related objects in a consistent and interchangeable manner.
2. Parameterized Factory Methods: Instead of using a single factory method to create objects, parameterized factory methods can be used to create objects with different configurations or parameters. This allows for more flexibility and customization when creating objects, without the need for separate factory classes or complex logic within the factory.
3. Dependency Injection with Factory: The Factory pattern can be combined with dependency injection to improve code maintainability and flexibility. Instead of directly using the factory to create objects, dependencies can be injected into client classes using dependency injection frameworks or techniques. This allows for easier testing, decoupling of dependencies, and better separation of concerns.
4. Reflection-based Factory: Reflection can be used to dynamically create objects based on their class names or other runtime information. A reflection-based factory can use reflection mechanisms, such as Class.forName()
or Constructor.newInstance()
, to create objects at runtime based on the specific requirements or configurations.
5. Inversion of Control (IoC) Containers: Inversion of Control (IoC) containers, such as Spring Framework or Google Guice, provide advanced factory capabilities and dependency management. These containers can automatically create and manage objects based on configuration files or annotations, allowing for flexible and dynamic object creation and dependency injection.
These advanced techniques provide additional options and variations for implementing the Factory pattern. Depending on the specific requirements and constraints of the application, one or more of these techniques can be applied to enhance the functionality and flexibility of the object creation process.
Advanced Techniques: Decorator Pattern
The Decorator pattern is a structural design pattern that allows behavior to be added to an individual object dynamically. While the basic implementation of the Decorator pattern is straightforward, there are advanced techniques and variations that can be applied to enhance its functionality and address specific requirements. Here are some advanced techniques for implementing the Decorator pattern:
1. Dynamic Decoration: The basic implementation of the Decorator pattern involves statically defining decorators and their order of application. However, decorators can also be applied dynamically at runtime based on specific conditions or configurations. This allows for more flexibility and fine-grained control over the behavior of the decorated object.
2. Decorator Chains: Instead of using a single decorator, multiple decorators can be chained together to add or modify behavior in a layered manner. Decorator chains can be dynamically constructed or configured, allowing for complex and customizable behavior modification.
3. Aspect-Oriented Programming (AOP): The Decorator pattern can be combined with aspect-oriented programming techniques to provide additional cross-cutting functionality, such as logging, caching, or security. AOP frameworks, such as AspectJ or Spring AOP, can be used to apply decorators (aspects) to objects based on specific pointcuts or join points in the code.
4. Composite Decorators: Decorators can be combined with the Composite pattern to create complex hierarchies of decorated objects. Composite decorators can add or modify behavior at different levels of the object hierarchy, allowing for more granular control over the behavior of the decorated objects.
5. Conditional Decoration: Decorators can be conditionally applied based on specific conditions or criteria. This allows for selective decoration of objects, providing different behavior based on runtime conditions or configurations.
These advanced techniques provide additional options and variations for implementing the Decorator pattern. Depending on the specific requirements and constraints of the application, one or more of these techniques can be applied to enhance the functionality and flexibility of the object decoration process.
Code Snippet 1: Singleton Pattern
The following code snippet demonstrates the basic implementation of the Singleton pattern using lazy initialization and double-checked locking:
public class Singleton { private static volatile Singleton instance; private Singleton() { // Private constructor to prevent instantiation } public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; }}
In this code snippet, the getInstance()
method returns the single instance of the Singleton
class. The instance is lazily created when it is first requested and is guarded by a double-checked locking mechanism to ensure thread safety.
The volatile
keyword is used to ensure that the instance variable is correctly initialized across different threads. The outer if
condition checks if the instance is already created before acquiring the lock, while the inner if
condition checks again inside the synchronized block to prevent redundant object creation.
This implementation guarantees that only a single instance of the Singleton
class is created and provides a global point of access to it.
Related Article: How To Convert Java Objects To JSON With Jackson
Code Snippet 2: Factory Pattern
The following code snippet demonstrates the basic implementation of the Factory pattern using a factory class and factory methods:
public interface Animal { void makeSound();}public class Dog implements Animal { @Override public void makeSound() { System.out.println("Woof!"); }}public class Cat implements Animal { @Override public void makeSound() { System.out.println("Meow!"); }}public class AnimalFactory { public static Animal createDog() { return new Dog(); } public static Animal createCat() { return new Cat(); }}
In this code snippet, the Animal
interface defines the makeSound()
method, which is implemented by concrete classes Dog
and Cat
. The AnimalFactory
class provides factory methods createDog()
and createCat()
that return instances of the respective concrete classes.
Using the factory methods, client code can create Dog
or Cat
objects without being aware of their concrete types. This allows for decoupling between the client code and the specific implementation of the created objects.
Animal dog = AnimalFactory.createDog();dog.makeSound(); // Output: Woof!Animal cat = AnimalFactory.createCat();cat.makeSound(); // Output: Meow!
This implementation demonstrates how the Factory pattern can be used to create objects without specifying their exact classes, providing a flexible and abstract way to work with different types of objects.
Code Snippet 3: Decorator Pattern
The following code snippet demonstrates the basic implementation of the Decorator pattern using a component interface, concrete component class, and decorator classes:
public interface Component { void operation();}public class ConcreteComponent implements Component { @Override public void operation() { System.out.println("Executing operation in ConcreteComponent."); }}public abstract class Decorator implements Component { protected Component component; public Decorator(Component component) { this.component = component; } @Override public void operation() { component.operation(); }}public class ConcreteDecoratorA extends Decorator { public ConcreteDecoratorA(Component component) { super(component); } @Override public void operation() { super.operation(); System.out.println("Executing additional operation in ConcreteDecoratorA."); }}public class ConcreteDecoratorB extends Decorator { public ConcreteDecoratorB(Component component) { super(component); } @Override public void operation() { super.operation(); System.out.println("Executing additional operation in ConcreteDecoratorB."); }}
In this code snippet, the Component
interface defines the operation()
method, which is implemented by the ConcreteComponent
class. The Decorator
class is an abstract class that implements the Component
interface and holds a reference to the wrapped component. Concrete decorator classes, such as ConcreteDecoratorA
and ConcreteDecoratorB
, extend the Decorator
class and add additional behavior before or after calling the wrapped component's operation()
method.
Component component = new ConcreteComponent();component.operation(); // Output: Executing operation in ConcreteComponent.Component decoratedComponentA = new ConcreteDecoratorA(component);decoratedComponentA.operation();// Output:// Executing operation in ConcreteComponent.// Executing additional operation in ConcreteDecoratorA.Component decoratedComponentB = new ConcreteDecoratorB(component);decoratedComponentB.operation();// Output:// Executing operation in ConcreteComponent.// Executing additional operation in ConcreteDecoratorB.
This implementation demonstrates how the Decorator pattern can be used to add or modify the behavior of objects dynamically and in a transparent manner, without changing the original code structure.