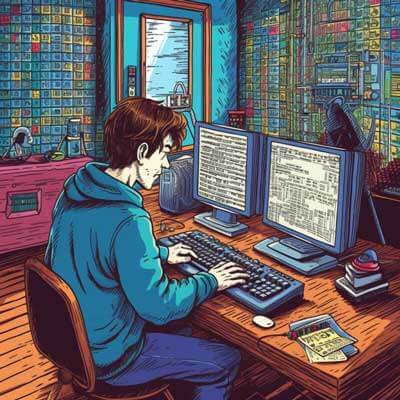
Table of Contents
Introduction to Redis Sharding
Redis Sharding is a technique used to horizontally scale Redis databases by distributing data across multiple Redis instances. It involves partitioning the data and assigning each partition to a different Redis instance. Sharding allows for increased storage capacity, improved performance, and higher availability.
To implement Redis sharding, a consistent hashing algorithm is used to determine which Redis instance should handle each key. This ensures that the data is evenly distributed across the instances.
Related Article: Tutorial on AWS Elasticache Redis Implementation
Use Cases for Redis Sharding
Redis sharding is particularly useful in scenarios where the data size exceeds the capacity of a single Redis instance or when high read/write throughput is required. Some common use cases for Redis sharding include:
1. High-traffic web applications: Redis sharding can help handle large amounts of data and heavy read/write loads, making it suitable for caching frequently accessed data or session management in web applications.
2. Real-time analytics: By distributing data across multiple Redis instances, sharding enables efficient processing and analysis of large datasets in real-time.
Example: Implementing Redis Sharding for a Web Application
To illustrate the implementation of Redis sharding in a web application, consider a scenario where a social media platform needs to store and retrieve user posts. The following code snippet demonstrates how Redis sharding can be implemented using the Python Redis library:
import redis # Redis Shard Configuration redis_shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), redis.Redis(host='shard3.example.com', port=6379) ] # Generate shard key based on the post ID def get_shard_key(post_id): return str(post_id % len(redis_shards)) # Save post to Redis shard def save_post(post_id, post_content): shard_key = get_shard_key(post_id) redis_shards[shard_key].set(post_id, post_content) # Retrieve post from Redis shard def get_post(post_id): shard_key = get_shard_key(post_id) return redis_shards[shard_key].get(post_id)
In this example, the redis_shards
list represents the different Redis instances used for sharding. The get_shard_key
function determines the shard key based on the post ID, and the save_post
and get_post
functions save and retrieve posts from the appropriate Redis shard based on the shard key.
Best Practices for Implementing Redis Sharding
When implementing Redis sharding, there are several best practices to consider:
1. Consistent Hashing: Use a consistent hashing algorithm to ensure even distribution of data across Redis instances. This helps prevent hotspots and provides better load balancing.
2. Monitoring: Implement monitoring and metrics collection to gain insights into the performance and health of each Redis shard. This can help identify potential bottlenecks or issues before they impact the application.
3. Error Handling: Implement proper error handling and retry mechanisms to handle failures or timeouts when interacting with Redis shards. This helps ensure the availability and reliability of the sharded system.
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Example: Error Handling in Redis Sharding
Here's an example of how error handling can be implemented in a Redis sharding scenario using the Python Redis library:
import redis redis_shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), redis.Redis(host='shard3.example.com', port=6379) ] # Function to handle Redis connection errors def handle_redis_error(func): def wrapper(*args, **kwargs): try: return func(*args, **kwargs) except redis.exceptions.RedisError as e: # Handle the error (e.g., retry, log, fallback) print(f"Redis error: {str(e)}") raise return wrapper @handle_redis_error def save_data(key, value): shard_key = get_shard_key(key) redis_shards[shard_key].set(key, value) @handle_redis_error def get_data(key): shard_key = get_shard_key(key) return redis_shards[shard_key].get(key)
In this example, the handle_redis_error
function is a decorator that wraps the Redis operations and handles any RedisError
exceptions. It provides a centralized place to handle errors and allows for custom error handling logic.
Real World Examples of Redis Sharding
Redis sharding is widely used in various real-world scenarios. Here are two examples:
1. Twitter: Twitter uses Redis sharding to handle the massive amount of tweet data generated by millions of users. By sharding the data across multiple Redis instances, Twitter ensures high availability and efficient data processing.
2. Pinterest: Pinterest leverages Redis sharding to manage user pins, boards, and other data. The sharding technique allows Pinterest to handle the substantial growth in user-generated content while maintaining fast response times.
Performance Considerations for Redis Sharding
When considering performance in Redis sharding, it is important to:
1. Monitor and optimize network latency: Network latency can impact the performance of Redis sharding. Minimizing network round trips and ensuring low-latency network connections between Redis instances can improve overall performance.
2. Cache Metadata: Caching shard metadata can reduce the overhead of determining which shard a key belongs to. This can be achieved by storing shard mappings in a centralized cache like Redis or using a distributed cache like Memcached.
Example: Caching Shard Metadata
import redis metadata_cache = redis.Redis(host='cache.example.com', port=6379) def get_shard_key(post_id): cached_shard_key = metadata_cache.get(post_id) if cached_shard_key is None: shard_key = calculate_shard_key(post_id) metadata_cache.set(post_id, shard_key) else: shard_key = cached_shard_key return shard_key
In this example, the metadata_cache
Redis instance is used to cache the shard mappings. When retrieving a post, the code first checks if the shard key for the given post ID is present in the cache. If not, it calculates the shard key and stores it in the cache for future use.
Related Article: Tutorial: Integrating Redis with Spring Boot
Error Handling in Redis Sharding
When working with Redis sharding, it is important to handle errors effectively. Common error scenarios in Redis sharding include network failures, Redis instance failures, and timeouts.
To handle errors in Redis sharding, consider the following approaches:
1. Retry Mechanism: Implement a retry mechanism to handle transient errors such as network connectivity issues. Retry with exponential backoff to avoid overwhelming the system with retries.
2. Error Logging: Log errors for troubleshooting and debugging purposes. Include relevant information such as the error message, timestamp, and the context in which the error occurred.
3. Graceful Degradation: Implement fallback mechanisms or alternative strategies in case of Redis sharding failures. For example, if a Redis shard becomes unavailable, the application can fall back to a different Redis shard or use a different data store.
Example: Retry Mechanism in Redis Sharding
import redis import time redis_shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), redis.Redis(host='shard3.example.com', port=6379) ] def retry_with_backoff(func, max_retries=3, initial_delay=0.1, max_delay=1.0): retries = 0 delay = initial_delay while retries < max_retries: try: return func() except redis.exceptions.RedisError as e: print(f"Redis error: {str(e)}. Retrying in {delay} seconds...") time.sleep(delay) delay = min(delay * 2, max_delay) retries += 1 raise Exception(f"Failed after {max_retries} retries") def save_data(key, value): def save(): shard_key = get_shard_key(key) redis_shards[shard_key].set(key, value) retry_with_backoff(save) def get_data(key): def get(): shard_key = get_shard_key(key) return redis_shards[shard_key].get(key) return retry_with_backoff(get)
In this example, the retry_with_backoff
function is used to implement a retry mechanism with exponential backoff. The save_data
and get_data
functions are wrapped with this retry mechanism to handle Redis errors. If a Redis error occurs, the function is retried with increasing delays between retries.
Code Snippet: Implementing Redis Sharding
Here's an example of how to implement Redis sharding using the Redisson library in Java:
import org.redisson.Redisson; import org.redisson.api.RedissonClient; import org.redisson.config.Config; import java.util.ArrayList; import java.util.List; public class RedisShardingExample { private static final int SHARD_COUNT = 3; public static void main(String[] args) { List<String> shardAddresses = new ArrayList<>(); shardAddresses.add("redis://shard1.example.com:6379"); shardAddresses.add("redis://shard2.example.com:6379"); shardAddresses.add("redis://shard3.example.com:6379"); List<RedissonClient> redissonShards = new ArrayList<>(); for (String address : shardAddresses) { Config config = new Config(); config.useSingleServer().setAddress(address); RedissonClient redisson = Redisson.create(config); redissonShards.add(redisson); } // Perform Redis sharding operations // ... } }
In this Java example, the Redisson library is used to create RedissonClient instances for each Redis shard. The shard addresses are specified in the shardAddresses
list, and the Redisson configuration is set accordingly. The resulting redissonShards
list contains the RedissonClient instances for each shard, which can be used for sharded operations.
Code Snippet: Scaling Redis Sharding
Scaling Redis sharding involves adding or removing Redis instances to accommodate increased or decreased data storage and processing requirements. Here's an example of how to scale Redis sharding using the Python Redis library:
import redis # Existing Redis shard configuration redis_shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), redis.Redis(host='shard3.example.com', port=6379) ] # Add a new Redis shard def add_redis_shard(host, port): new_shard = redis.Redis(host=host, port=port) redis_shards.append(new_shard) # Remove a Redis shard def remove_redis_shard(host, port): shard_to_remove = redis.Redis(host=host, port=port) redis_shards.remove(shard_to_remove)
In this example, the add_redis_shard
function adds a new Redis shard to the existing redis_shards
list. The remove_redis_shard
function removes a Redis shard from the list. By dynamically adding or removing Redis shards, the sharding capacity can be scaled up or down as needed.
Related Article: How to Configure a Redis Cluster
Code Snippet: Monitoring Redis Sharding
Proper monitoring of Redis sharding is essential to ensure its performance and availability. Here's an example of how to monitor Redis sharding using the Prometheus monitoring system:
scrape_configs: - job_name: 'redis_shards' metrics_path: '/metrics' static_configs: - targets: ['shard1.example.com:9121', 'shard2.example.com:9121', 'shard3.example.com:9121']
In this example, a Prometheus configuration file is used to scrape metrics from each Redis shard. The scrape_configs
section specifies the job name, metrics path, and the targets (Redis shard instances) to monitor. By collecting and analyzing these metrics, you can gain insights into the performance and health of your Redis sharding setup.
Advanced Technique: Consistent Hashing in Redis Sharding
Consistent hashing is an advanced technique used in Redis sharding to ensure even distribution of data across Redis instances while minimizing data movement when the number of instances changes. It achieves this by mapping each key to a point on a hash ring.
With consistent hashing, adding or removing a Redis instance only affects a fraction of the keys, reducing the need for data rebalancing. This makes it an efficient and scalable approach for Redis sharding.
Example: Consistent Hashing Algorithm
Redis clients typically use the CRC16 algorithm to calculate the hash slot for a given key. The following code snippet demonstrates how to implement a consistent hashing algorithm using CRC16 in Python:
import zlib def crc16(key): return zlib.crc32(key.encode()) & 0xFFFF class ConsistentHashing: def __init__(self, nodes, replicas=100): self.replicas = replicas self.circle = {} for node in nodes: self.add_node(node) def add_node(self, node): for i in range(self.replicas): key = f"{node}-{i}" slot = crc16(key) self.circle[slot] = node def remove_node(self, node): for i in range(self.replicas): key = f"{node}-{i}" slot = crc16(key) del self.circle[slot] def get_node(self, key): if not self.circle: return None slot = crc16(key) keys = list(self.circle.keys()) keys.sort() for k in keys: if k >= slot: return self.circle[k] return self.circle[keys[0]]
In this example, the ConsistentHashing
class implements consistent hashing using the CRC16 algorithm. The add_node
method adds a node (Redis instance) to the hash ring, while the remove_node
method removes a node. The get_node
method returns the node responsible for a given key based on its hash slot.
Advanced Technique: Data Partitioning in Redis Sharding
Data partitioning is another advanced technique used in Redis sharding to divide the data into logical partitions or shards. Each shard is responsible for a specific range of keys, allowing for efficient data storage and retrieval.
When implementing data partitioning in Redis sharding, consider the following aspects:
1. Key Distribution: Choose a partitioning scheme that evenly distributes keys across the partitions to avoid data hotspots.
2. Partitioning Strategy: Determine the strategy for assigning keys to partitions, such as range-based partitioning or hash-based partitioning.
Related Article: Exploring Alternatives to Redis
Example: Range-based Partitioning
Range-based partitioning is a common data partitioning strategy in Redis sharding. The following code snippet demonstrates how to implement range-based partitioning using the Python Redis library:
import redis redis_shards = { 'shard1': redis.Redis(host='shard1.example.com', port=6379), 'shard2': redis.Redis(host='shard2.example.com', port=6379), 'shard3': redis.Redis(host='shard3.example.com', port=6379) } def get_shard_key_range(shard_id): # Define key ranges for each shard if shard_id == 'shard1': return (0, 9999) elif shard_id == 'shard2': return (10000, 19999) elif shard_id == 'shard3': return (20000, 29999) def get_shard_id(key): # Determine the shard ID based on the key for shard_id in redis_shards.keys(): shard_key_range = get_shard_key_range(shard_id) if shard_key_range[0] <= key <= shard_key_range[1]: return shard_id raise Exception('No shard found for key') def save_data(key, value): shard_id = get_shard_id(key) redis_shards[shard_id].set(key, value) def get_data(key): shard_id = get_shard_id(key) return redis_shards[shard_id].get(key)
In this example, the get_shard_key_range
function defines the key ranges for each shard. The get_shard_id
function determines the shard ID based on the key by checking which key range it falls into. The save_data
and get_data
functions save and retrieve data from the appropriate Redis shard based on the shard ID.
Advanced Technique: Distributed Caching in Redis Sharding
Distributed caching is an advanced technique that leverages Redis sharding to improve the performance and scalability of caching systems. By distributing the cache across multiple Redis instances, it allows for higher cache capacity, reduced cache contention, and improved cache hit rates.
When implementing distributed caching in Redis sharding, consider the following:
1. Cache Key Distribution: Use consistent hashing to evenly distribute cache keys across Redis instances. This helps ensure balanced load distribution and efficient cache utilization.
2. Cache Invalidation: Implement a cache invalidation strategy to remove stale or outdated data from the cache. This can be achieved through time-based expiration or manual invalidation based on data updates.
Example: Distributed Caching with Redis Sharding
import redis redis_shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), redis.Redis(host='shard3.example.com', port=6379) ] def get_cache_key(key): return f"cache:{key}" def cache_get(key): cache_key = get_cache_key(key) shard_key = get_shard_key(key) return redis_shards[shard_key].get(cache_key) def cache_set(key, value, ttl=None): cache_key = get_cache_key(key) shard_key = get_shard_key(key) redis_shards[shard_key].set(cache_key, value, ex=ttl) def cache_delete(key): cache_key = get_cache_key(key) shard_key = get_shard_key(key) redis_shards[shard_key].delete(cache_key)
In this example, the get_cache_key
function prefixes the cache key with "cache:" to differentiate it from other keys in Redis. The cache_get
function retrieves data from the appropriate Redis shard based on the shard key calculated using consistent hashing. The cache_set
function sets data in the cache, and the cache_delete
function removes data from the cache.
These chapters provide a comprehensive overview of implementing Redis sharding, covering various aspects such as introduction, use cases, best practices, real-world examples, performance considerations, error handling, code snippets, and advanced techniques. With this knowledge, you can leverage Redis sharding to scale your Redis databases and enhance the performance and reliability of your applications.