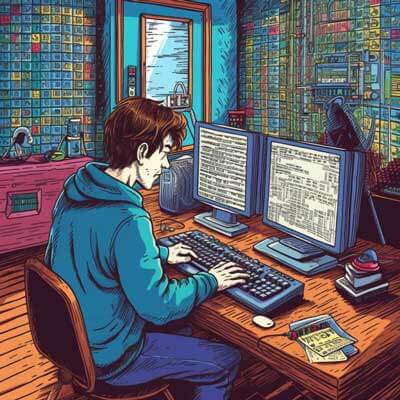
Converting a string to a number is a common task in TypeScript when working with user input or data coming from external sources. Fortunately, TypeScript provides several built-in methods and techniques for converting strings to numbers. In this post, we will explore different approaches to achieve this conversion.
1. Using the Number() Function
One straightforward way to convert a string to a number in TypeScript is by using the Number()
function. This function takes a string as an argument and returns a numeric representation of that string. Here’s an example:
const str = "42"; const num = Number(str); console.log(num); // Output: 42 console.log(typeof num); // Output: "number"
In the above example, we use the Number()
function to convert the string "42"
to the number 42
. The resulting value is then stored in the num
variable. We can verify the type of num
using the typeof
operator.
It is important to note that if the provided string cannot be parsed as a valid number, the Number()
function will return NaN
(Not a Number). For example:
const str = "Hello"; const num = Number(str); console.log(num); // Output: NaN
In this case, the string "Hello"
cannot be converted to a number, so the result is NaN
.
Related Article: TypeScript ETL (Extract, Transform, Load) Tutorial
2. Using the parseInt() and parseFloat() Functions
In addition to the Number()
function, TypeScript also provides the parseInt()
and parseFloat()
functions for converting strings to integers and floating-point numbers, respectively.
The parseInt()
function parses a string and returns an integer value. It takes two arguments: the string to parse and an optional radix (base) for parsing. Here’s an example:
const str = "10"; const num = parseInt(str); console.log(num); // Output: 10 console.log(typeof num); // Output: "number"
In this example, the string "10"
is parsed as an integer using the parseInt()
function, and the resulting value is 10
.
Similarly, the parseFloat()
function parses a string and returns a floating-point number. Here’s an example:
const str = "3.14"; const num = parseFloat(str); console.log(num); // Output: 3.14 console.log(typeof num); // Output: "number"
In this example, the string "3.14"
is parsed as a floating-point number using the parseFloat()
function, and the resulting value is 3.14
.
Both parseInt()
and parseFloat()
functions ignore any non-numeric characters after the initial numeric characters. For example:
const str = "42 is the answer"; const num = parseInt(str); console.log(num); // Output: 42
In this case, the parseInt()
function only considers the initial numeric characters "42"
and ignores the rest of the string.
3. Using the Unary Plus Operator
Another way to convert a string to a number in TypeScript is by using the unary plus operator (+
). This operator converts its operand to a number. Here’s an example:
const str = "42"; const num = +str; console.log(num); // Output: 42 console.log(typeof num); // Output: "number"
In this example, the unary plus operator is used to convert the string "42"
to the number 42
.
It is important to note that if the provided string cannot be parsed as a valid number, the result will be NaN
. For example:
const str = "Hello"; const num = +str; console.log(num); // Output: NaN
In this case, the string "Hello"
cannot be converted to a number, so the result is NaN
.
4. Using the parseInt() Method with a Radix
The parseInt()
function can also be used to convert strings to numbers with a specific radix (base). By providing a radix as the second argument to parseInt()
, we can control how the string is interpreted. For example:
const binaryStr = "1010"; const decimalNum = parseInt(binaryStr, 2); console.log(decimalNum); // Output: 10
In this example, the string "1010"
is interpreted as a binary number by specifying 2
as the radix. The resulting decimal number is 10
.
Similarly, we can convert hexadecimal strings to decimal numbers by specifying 16
as the radix:
const hexStr = "FF"; const decimalNum = parseInt(hexStr, 16); console.log(decimalNum); // Output: 255
In this example, the hexadecimal string "FF"
is interpreted as a hexadecimal number by specifying 16
as the radix. The resulting decimal number is 255
.
Using the appropriate radix is essential to correctly interpret the string. If the radix is not specified or set to 0
, the parseInt()
function will attempt to determine the radix automatically based on the string format.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
5. Handling Invalid Conversions
When converting a string to a number, it is important to handle cases where the conversion is not possible or results in NaN
. Here are a few strategies to consider:
– Check the result using the isNaN()
function:
const str = "Hello"; const num = Number(str); if (isNaN(num)) { console.log("Invalid number"); } else { console.log(num); }
In this example, the isNaN()
function is used to check if the num
value is NaN
. If it is NaN
, an error message is logged.
– Use default values for invalid conversions:
const str = "Hello"; const num = Number(str) || 0; console.log(num); // Output: 0
In this example, the logical OR (||
) operator is used to assign a default value of 0
if the conversion to a number fails.
– Validate the input string before conversion:
function convertToNumber(str: string): number | null { if (/^\d+$/.test(str)) { return Number(str); } return null; } const str = "42"; const num = convertToNumber(str); if (num === null) { console.log("Invalid number"); } else { console.log(num); }
In this example, a custom function convertToNumber()
is used to validate the input string using a regular expression (/^\d+$/
). If the string consists of only digits, it is converted to a number; otherwise, null
is returned.