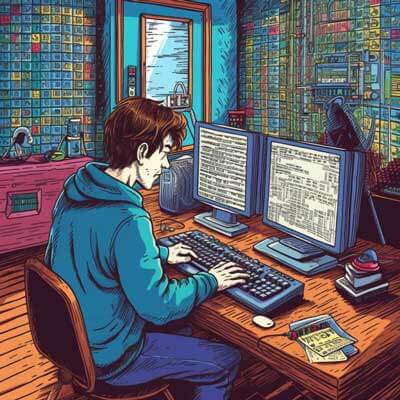
- Syntax for Importing HTML Files in TypeScript
- Using HTML Templates in TypeScript
- Importing External HTML Files in TypeScript
- Benefits of Importing HTML Templates in TypeScript
- Alternatives to Importing HTML Templates in TypeScript
- Dynamic Import of HTML Templates in TypeScript
- Libraries and Frameworks for Importing HTML Templates in TypeScript
- Step-by-Step Guide to Importing HTML in TypeScript
- External Sources
Syntax for Importing HTML Files in TypeScript
When working with TypeScript, importing HTML templates can be a useful feature to enhance the development process. By importing HTML files directly into TypeScript, you can leverage the benefits of type checking and code completion, making your code more maintainable and less error-prone.
To import HTML files in TypeScript, you can utilize the raw-loader
package, which allows you to load files as strings. First, you need to install the raw-loader
package using npm or yarn:
npm install --save-dev raw-loader
Once installed, you can use the import
statement to import HTML files. However, since TypeScript doesn’t recognize HTML as a valid module, you need to use a type declaration file to tell TypeScript how to handle the imported HTML. Create a file named html.d.ts
with the following content:
declare module '*.html' { const content: string; export default content; }
With this type declaration file in place, you can now import HTML files in your TypeScript code. For example, if you have an HTML file named template.html
, you can import it as follows:
import template from './template.html';
The template
variable will now contain the content of the HTML file as a string, which you can use in your code.
Related Article: Building a Rules Engine with TypeScript
Using HTML Templates in TypeScript
Once you have imported an HTML template in TypeScript, you can use it in various ways to enhance your application. One common use case is to dynamically generate HTML content based on the imported template.
Let’s say you have a template file named user.html
with the following content:
<div class="user"> <h1>{{ name }}</h1> <p>{{ email }}</p> </div>
You can import this template in your TypeScript code and use it to generate dynamic HTML content. Here’s an example:
import userTemplate from './user.html'; function renderUser(user: { name: string; email: string }) { const renderedTemplate = userTemplate .replace('{{ name }}', user.name) .replace('{{ email }}', user.email); document.getElementById('user-container').innerHTML = renderedTemplate; } const user = { name: 'John Doe', email: 'john@example.com' }; renderUser(user);
In this example, the renderUser
function takes a user object as input, replaces the placeholders in the imported template with the corresponding values, and then sets the rendered HTML as the content of an element with the ID user-container
. This allows you to dynamically generate HTML content based on the imported template.
Importing External HTML Files in TypeScript
In addition to importing HTML files from within your project, you can also import external HTML files in TypeScript. This can be useful when you want to reuse HTML templates across multiple projects or when working with third-party HTML templates.
To import an external HTML file in TypeScript, you can use the full URL of the file as the import path. For example:
import template from 'https://example.com/template.html';
Note that when importing external HTML files, you may need to configure your project to allow CORS (Cross-Origin Resource Sharing) if the HTML file is hosted on a different domain.
Benefits of Importing HTML Templates in TypeScript
Importing HTML templates in TypeScript offers several benefits that can improve the development experience and code quality:
1. Type Safety: TypeScript provides static type checking, which helps catch errors at compile-time rather than runtime. By importing HTML templates as strings, you can leverage TypeScript’s type system to ensure that you are using the correct template and avoid common HTML-related errors.
2. Code Completion: When you import HTML templates in TypeScript, your editor or IDE can provide code completion and suggestions based on the structure and content of the HTML template. This can significantly speed up development and reduce the chances of making mistakes.
3. Modularity: By separating HTML templates into individual files and importing them in TypeScript, you can promote modularity and reusability in your codebase. This makes it easier to manage and maintain your HTML templates, especially in larger projects.
4. Improved Collaboration: Importing HTML templates in TypeScript allows frontend developers to work more closely with backend developers. They can define and share HTML templates as separate files, making it easier to collaborate on the structure and content of the templates.
Related Article: Comparing Go with TypeScript
Alternatives to Importing HTML Templates in TypeScript
While importing HTML templates in TypeScript has its advantages, it’s important to note that there are alternative approaches that you can use depending on your specific use case. Here are a few alternatives worth considering:
1. Inline Templates: Instead of importing HTML templates as separate files, you can define them inline within your TypeScript code using template literals or JSX. This approach eliminates the need for separate files but may result in less maintainable code, especially for complex templates.
2. Server-Side Rendering: If you need to render HTML templates dynamically on the server-side, you can use server-side rendering frameworks like Next.js or Angular Universal. These frameworks allow you to write templates using HTML and dynamically inject data into them before sending the rendered HTML to the client.
3. Frontend Frameworks: If you’re working with a frontend framework like React, Vue.js, or Angular, these frameworks often provide their own mechanisms for defining and rendering templates. In many cases, you don’t need to import HTML templates directly into TypeScript but can instead use the framework’s component-based approach.
It’s important to evaluate your specific requirements and choose the approach that best fits your needs.
Dynamic Import of HTML Templates in TypeScript
In some cases, you may need to dynamically import HTML templates in TypeScript, for example, when you want to load templates based on user interactions or conditionally load templates based on certain conditions.
To dynamically import HTML templates in TypeScript, you can use the import()
function, which returns a promise that resolves to the imported module. Here’s an example:
async function loadTemplate(templateName: string) { const template = await import(`./templates/${templateName}.html`); return template.default; } const templateName = 'user'; const userTemplate = await loadTemplate(templateName);
In this example, the loadTemplate
function takes a template name as input and dynamically imports the corresponding HTML template. The import()
function is called with the template path as a template literal, allowing you to specify the template name dynamically. The imported module is then accessed using the default
property, which contains the template content as a string.
Libraries and Frameworks for Importing HTML Templates in TypeScript
While TypeScript itself doesn’t provide built-in support for importing HTML templates, there are several libraries and frameworks that can help you with this task. Here are a few popular options:
1. webpack: If you’re using webpack as your module bundler, you can use the raw-loader
package, as mentioned earlier, to import HTML templates as strings. webpack’s loaders allow you to preprocess files before bundling them, enabling you to import HTML templates in TypeScript.
2. Rollup: Rollup is another popular module bundler that supports importing HTML templates. You can use plugins like rollup-plugin-html
or rollup-plugin-string
to import HTML templates in TypeScript.
3. Lit: Lit is a lightweight library for building web components with TypeScript. It provides a html
tagged template literal function that allows you to define HTML templates directly in your TypeScript code. This eliminates the need for separate HTML files and enables code completion and type checking.
4. Angular: If you’re using Angular, the framework provides its own template system that allows you to define HTML templates inline within TypeScript files. Angular’s template system supports features like data binding, event handling, and component composition.
These are just a few examples, and there are many other libraries and frameworks available that can help you import HTML templates in TypeScript. Choose the one that best suits your project’s requirements and ecosystem.
Related Article: Fixing 'TypeScript Does Not Exist on Type Never' Errors
Step-by-Step Guide to Importing HTML in TypeScript
Now let’s walk through a step-by-step guide on how to import HTML templates in TypeScript:
1. Install the raw-loader
package using npm or yarn:
npm install --save-dev raw-loader
2. Create a type declaration file named html.d.ts
with the following content:
declare module '*.html' { const content: string; export default content; }
3. Import the HTML template in your TypeScript code:
import template from './template.html';
4. Use the imported template to generate dynamic HTML content:
function renderUser(user: { name: string; email: string }) { const renderedTemplate = template .replace('{{ name }}', user.name) .replace('{{ email }}', user.email); document.getElementById('user-container').innerHTML = renderedTemplate; } const user = { name: 'John Doe', email: 'john@example.com' }; renderUser(user);
Follow these steps, and you’ll be able to import and use HTML templates in TypeScript effectively.