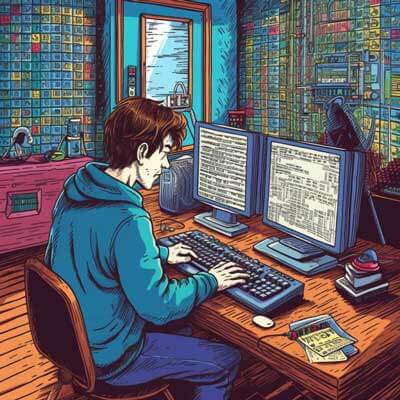
- Introduction to Scikit Learn
- Installing and Configuring Scikit Learn
- Code Snippet: Installing Scikit Learn
- Code Snippet: Importing Scikit Learn
- Data Preprocessing with Scikit Learn
- Handling Missing Values
- Scaling Features
- Supervised Learning: Regression
- Linear Regression
- Decision Tree Regression
- Supervised Learning: Classification
- Logistic Regression
- Decision Tree Classification
- Unsupervised Learning: Clustering
- K-means Clustering
- DBSCAN
- Unsupervised Learning: Dimensionality Reduction
- Principal Component Analysis (PCA)
- t-distributed Stochastic Neighbor Embedding (t-SNE)
- Model Evaluation Metrics
- Classification Metrics
- Regression Metrics
- Cross-validation in Scikit Learn
- Using cross_val_score
- Using KFold
- Hyperparameters and Grid Search
- Using GridSearchCV
- Utilizing Pipelines in Scikit Learn
- Creating a Pipeline
- Code Snippet: Creating a Pipeline
- Code Snippet: Regression with Scikit Learn
- Code Snippet: Classification with Scikit Learn
- Code Snippet: Clustering with Scikit Learn
- Code Snippet: Dimensionality Reduction with Scikit Learn
- Code Snippet: Cross-validation with Scikit Learn
- Use Case: Predicting House Prices
- Code Snippet: Predicting House Prices with Linear Regression
- Code Snippet: Predicting House Prices with Decision Tree Regression
- Use Case: Image Recognition
- Code Snippet: Image Recognition with Logistic Regression
- Code Snippet: Image Recognition with Convolutional Neural Networks (CNN)
- Use Case: Text Classification
- Code Snippet: Text Classification with Logistic Regression
- Code Snippet: Text Classification with Support Vector Machines
- Best Practice: Data Scaling
- Standardization
- Normalization
- Best Practice: Handling Imbalanced Data
- Oversampling
- Undersampling
- Using Class Weights
- Best Practice: Feature Selection
- Univariate Feature Selection
- Recursive Feature Elimination
- Real World Example: Predicting Credit Card Fraud
- Code Snippet: Predicting Credit Card Fraud with Logistic Regression
- Code Snippet: Predicting Credit Card Fraud with Random Forest
- Real World Example: Recommender System
- Code Snippet: Collaborative Filtering
- Code Snippet: Content-based Filtering
- Real World Example: Predicting Customer Churn
- Code Snippet: Predicting Customer Churn with Logistic Regression
- Code Snippet: Predicting Customer Churn with Support Vector Machines
- Performance Consideration: Algorithm Choice
- Regression
- Classification
- Clustering
- Dimensionality Reduction
- Performance Consideration: Data Size
- Stochastic Gradient Descent
- Mini-batch Learning
- Incremental Learning
- Performance Consideration: Hardware Considerations
- Parallel Processing
- GPU Acceleration
- Advanced Technique: Ensemble Methods
- Bagging
- Boosting
- Stacking
- Advanced Technique: Feature Engineering
- Polynomial Features
- Interaction Terms
- Advanced Technique: Neural Networks
- Code Snippet: Neural Network for Regression
- Code Snippet: Neural Network for Classification
- Error Handling in Scikit Learn
- Handling Missing Values
- Handling Incompatible Shapes
- Handling Invalid Parameter Values
Introduction to Scikit Learn
Scikit Learn is a powerful and popular machine learning library in Python. It provides a wide range of tools and algorithms for data preprocessing, feature selection, model training, and evaluation. Whether you are a beginner or an experienced data scientist, Scikit Learn offers a user-friendly interface and comprehensive documentation to help you solve real-world problems.
Related Article: How to Plot a Histogram in Python Using Matplotlib with List Data
Installing and Configuring Scikit Learn
To install Scikit Learn, you can use pip, the package installer for Python. Simply open your command prompt or terminal and run the following command:
pip install scikit-learn
Scikit Learn has some dependencies, such as NumPy and SciPy, which will be automatically installed if not already present.
Once Scikit Learn is installed, you can import it in your Python script or notebook using the following code:
import sklearn
Code Snippet: Installing Scikit Learn
To install Scikit Learn, open your command prompt or terminal and run the following command:
pip install scikit-learn
Code Snippet: Importing Scikit Learn
To import Scikit Learn in your Python script or notebook, use the following code:
import sklearn
Data Preprocessing with Scikit Learn
Data preprocessing is an essential step in any machine learning project. Scikit Learn provides a variety of preprocessing techniques to handle missing values, scale features, encode categorical variables, and more.
Handling Missing Values
Missing values in a dataset can be problematic for machine learning algorithms. Scikit Learn provides the SimpleImputer
class to handle missing values by replacing them with a suitable value. Here’s an example of how to use it:
from sklearn.impute import SimpleImputer # Create an instance of SimpleImputer imputer = SimpleImputer(strategy='mean') # Fit the imputer on the training data imputer.fit(X_train) # Transform the training and testing data X_train = imputer.transform(X_train) X_test = imputer.transform(X_test)
Scaling Features
When the features in your dataset have different scales, it can negatively impact the performance of certain machine learning algorithms. Scikit Learn provides various scaling techniques, such as standardization and normalization, to address this issue. Here’s an example of how to perform feature scaling using the StandardScaler
class:
from sklearn.preprocessing import StandardScaler # Create an instance of StandardScaler scaler = StandardScaler() # Fit the scaler on the training data scaler.fit(X_train) # Transform the training and testing data X_train = scaler.transform(X_train) X_test = scaler.transform(X_test)
Supervised Learning: Regression
Regression is a type of supervised learning where the goal is to predict a continuous target variable. Scikit Learn provides a wide range of regression algorithms, including linear regression, decision tree regression, and support vector regression.
Linear Regression
Linear regression is a simple yet powerful algorithm for predicting a continuous target variable based on one or more features. Scikit Learn provides the LinearRegression
class to perform linear regression. Here’s an example:
from sklearn.linear_model import LinearRegression # Create an instance of LinearRegression regressor = LinearRegression() # Fit the regressor on the training data regressor.fit(X_train, y_train) # Predict the target variable for the testing data y_pred = regressor.predict(X_test)
Decision Tree Regression
Decision tree regression is a non-parametric algorithm that makes predictions by partitioning the feature space into regions and assigning a constant value to each region. Scikit Learn provides the DecisionTreeRegressor
class to perform decision tree regression. Here’s an example:
from sklearn.tree import DecisionTreeRegressor # Create an instance of DecisionTreeRegressor regressor = DecisionTreeRegressor() # Fit the regressor on the training data regressor.fit(X_train, y_train) # Predict the target variable for the testing data y_pred = regressor.predict(X_test)
Supervised Learning: Classification
Classification is a type of supervised learning where the goal is to predict the class or category of a target variable. Scikit Learn provides various classification algorithms, such as logistic regression, decision tree classification, and random forest classification.
Logistic Regression
Logistic regression is a popular algorithm for binary classification. It models the probability of the target variable belonging to a certain class using a logistic function. Scikit Learn provides the LogisticRegression
class to perform logistic regression. Here’s an example:
from sklearn.linear_model import LogisticRegression # Create an instance of LogisticRegression classifier = LogisticRegression() # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class probabilities for the testing data y_pred_proba = classifier.predict_proba(X_test) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Decision Tree Classification
Decision tree classification is a non-parametric algorithm that assigns class labels to instances based on their feature values. Scikit Learn provides the DecisionTreeClassifier
class to perform decision tree classification. Here’s an example:
from sklearn.tree import DecisionTreeClassifier # Create an instance of DecisionTreeClassifier classifier = DecisionTreeClassifier() # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Unsupervised Learning: Clustering
Clustering is an unsupervised learning technique that groups similar instances together based on their feature similarity. Scikit Learn provides various clustering algorithms, such as K-means clustering, hierarchical clustering, and DBSCAN.
K-means Clustering
K-means clustering is a popular algorithm that partitions instances into K clusters based on their feature similarity. Scikit Learn provides the KMeans
class to perform K-means clustering. Here’s an example:
from sklearn.cluster import KMeans # Create an instance of KMeans kmeans = KMeans(n_clusters=3) # Fit the k-means model on the training data kmeans.fit(X_train) # Predict the cluster labels for the testing data y_pred = kmeans.predict(X_test)
DBSCAN
DBSCAN (Density-Based Spatial Clustering of Applications with Noise) is a density-based clustering algorithm that can discover clusters of arbitrary shape. Scikit Learn provides the DBSCAN
class to perform DBSCAN clustering. Here’s an example:
from sklearn.cluster import DBSCAN # Create an instance of DBSCAN dbscan = DBSCAN(eps=0.5, min_samples=5) # Fit the DBSCAN model on the training data dbscan.fit(X_train) # Predict the cluster labels for the testing data y_pred = dbscan.fit_predict(X_test)
Unsupervised Learning: Dimensionality Reduction
Dimensionality reduction is a technique used to reduce the number of features in a dataset while preserving its essential information. Scikit Learn provides various dimensionality reduction algorithms, such as principal component analysis (PCA) and t-distributed stochastic neighbor embedding (t-SNE).
Principal Component Analysis (PCA)
PCA is a popular technique for dimensionality reduction that transforms the original features into a new set of uncorrelated variables called principal components. Scikit Learn provides the PCA
class to perform PCA. Here’s an example:
from sklearn.decomposition import PCA # Create an instance of PCA pca = PCA(n_components=2) # Fit the PCA model on the training data pca.fit(X_train) # Transform the training and testing data X_train_pca = pca.transform(X_train) X_test_pca = pca.transform(X_test)
t-distributed Stochastic Neighbor Embedding (t-SNE)
t-SNE is a nonlinear dimensionality reduction technique that is particularly useful for visualizing high-dimensional data in a low-dimensional space. Scikit Learn provides the TSNE
class to perform t-SNE. Here’s an example:
from sklearn.manifold import TSNE # Create an instance of TSNE tsne = TSNE(n_components=2) # Fit the t-SNE model on the training data tsne.fit(X_train) # Transform the training and testing data X_train_tsne = tsne.transform(X_train) X_test_tsne = tsne.transform(X_test)
Model Evaluation Metrics
Model evaluation metrics are used to assess the performance of machine learning models. Scikit Learn provides a wide range of metrics for both classification and regression tasks, including accuracy, precision, recall, F1 score, mean squared error, and R-squared.
Classification Metrics
When evaluating classification models, metrics such as accuracy, precision, recall, and F1 score are commonly used. Here’s an example of how to calculate these metrics using Scikit Learn:
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score # Calculate accuracy accuracy = accuracy_score(y_true, y_pred) # Calculate precision precision = precision_score(y_true, y_pred) # Calculate recall recall = recall_score(y_true, y_pred) # Calculate F1 score f1 = f1_score(y_true, y_pred)
Regression Metrics
For regression models, metrics such as mean squared error (MSE) and R-squared are commonly used. Here’s an example of how to calculate these metrics using Scikit Learn:
from sklearn.metrics import mean_squared_error, r2_score # Calculate mean squared error mse = mean_squared_error(y_true, y_pred) # Calculate R-squared r2 = r2_score(y_true, y_pred)
Cross-validation in Scikit Learn
Cross-validation is a technique used to assess the performance of a machine learning model on unseen data. Scikit Learn provides various functions and classes for performing cross-validation, such as cross_val_score
, KFold
, and StratifiedKFold
.
Using cross_val_score
The cross_val_score
function is a convenient way to perform cross-validation and obtain the evaluation scores for each fold. Here’s an example:
from sklearn.model_selection import cross_val_score from sklearn.linear_model import LogisticRegression # Create an instance of LogisticRegression classifier = LogisticRegression() # Perform cross-validation scores = cross_val_score(classifier, X, y, cv=5) # Print the scores for each fold for fold, score in enumerate(scores): print(f"Fold {fold + 1}: {score}")
Using KFold
The KFold
class allows you to define the number of folds and control how the data is split into train and test sets for each fold. Here’s an example:
from sklearn.model_selection import KFold from sklearn.linear_model import LinearRegression # Create an instance of KFold kf = KFold(n_splits=5) # Iterate over the folds for train_index, test_index in kf.split(X): X_train, X_test = X[train_index], X[test_index] y_train, y_test = y[train_index], y[test_index] # Create an instance of LinearRegression regressor = LinearRegression() # Fit the regressor on the training data regressor.fit(X_train, y_train) # Evaluate the regressor on the testing data score = regressor.score(X_test, y_test) print(f"Score: {score}")
Hyperparameters and Grid Search
Hyperparameters are the parameters of a machine learning model that are not learned from the data but set by the user. Grid search is a technique used to find the best combination of hyperparameters for a model by exhaustively searching through a specified parameter grid. Scikit Learn provides the GridSearchCV
class to perform grid search.
Using GridSearchCV
The GridSearchCV
class takes a model and a dictionary of hyperparameters as input and performs an exhaustive search over all possible combinations of hyperparameters. Here’s an example:
from sklearn.model_selection import GridSearchCV from sklearn.svm import SVC # Create an instance of SVC classifier = SVC() # Define the parameter grid param_grid = { 'C': [0.1, 1, 10], 'kernel': ['linear', 'rbf'] } # Perform grid search grid_search = GridSearchCV(classifier, param_grid, cv=5) # Fit the grid search on the training data grid_search.fit(X_train, y_train) # Print the best hyperparameters print("Best hyperparameters:", grid_search.best_params_) # Print the best score print("Best score:", grid_search.best_score_)
Utilizing Pipelines in Scikit Learn
Pipelines are a convenient way to chain multiple preprocessing steps and a machine learning model into a single object. Scikit Learn provides the Pipeline
class to create pipelines.
Creating a Pipeline
To create a pipeline, you need to define a list of steps, where each step is a tuple containing a name and an instance of a transformer or an estimator. Here’s an example of how to create a pipeline for preprocessing and classification:
from sklearn.pipeline import Pipeline from sklearn.preprocessing import StandardScaler from sklearn.svm import SVC # Define the steps steps = [ ('scaler', StandardScaler()), ('classifier', SVC()) ] # Create the pipeline pipeline = Pipeline(steps) # Fit the pipeline on the training data pipeline.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = pipeline.predict(X_test)
Code Snippet: Creating a Pipeline
To create a pipeline, define a list of steps where each step is a tuple containing a name and an instance of a transformer or an estimator. Here’s an example:
from sklearn.pipeline import Pipeline from sklearn.preprocessing import StandardScaler from sklearn.svm import SVC # Define the steps steps = [ ('scaler', StandardScaler()), ('classifier', SVC()) ] # Create the pipeline pipeline = Pipeline(steps)
Code Snippet: Regression with Scikit Learn
Here’s an example of how to perform regression using Scikit Learn:
from sklearn.linear_model import LinearRegression # Create an instance of LinearRegression regressor = LinearRegression() # Fit the regressor on the training data regressor.fit(X_train, y_train) # Predict the target variable for the testing data y_pred = regressor.predict(X_test)
Code Snippet: Classification with Scikit Learn
Here’s an example of how to perform classification using Scikit Learn:
from sklearn.linear_model import LogisticRegression # Create an instance of LogisticRegression classifier = LogisticRegression() # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Code Snippet: Clustering with Scikit Learn
Here’s an example of how to perform clustering using Scikit Learn:
from sklearn.cluster import KMeans # Create an instance of KMeans kmeans = KMeans(n_clusters=3) # Fit the k-means model on the training data kmeans.fit(X_train) # Predict the cluster labels for the testing data y_pred = kmeans.predict(X_test)
Code Snippet: Dimensionality Reduction with Scikit Learn
Here’s an example of how to perform dimensionality reduction using Scikit Learn:
from sklearn.decomposition import PCA # Create an instance of PCA pca = PCA(n_components=2) # Fit the PCA model on the training data pca.fit(X_train) # Transform the training and testing data X_train_pca = pca.transform(X_train) X_test_pca = pca.transform(X_test)
Code Snippet: Cross-validation with Scikit Learn
Here’s an example of how to perform cross-validation using Scikit Learn:
from sklearn.model_selection import cross_val_score from sklearn.linear_model import LogisticRegression # Create an instance of LogisticRegression classifier = LogisticRegression() # Perform cross-validation scores = cross_val_score(classifier, X, y, cv=5) # Print the scores for each fold for fold, score in enumerate(scores): print(f"Fold {fold + 1}: {score}")
Use Case: Predicting House Prices
Predicting house prices is a common use case in the field of machine learning. Given a set of features such as the number of bedrooms, the area of the house, and the location, the goal is to predict the sale price of a house. Scikit Learn provides various regression algorithms that can be used for this task, such as linear regression, decision tree regression, and random forest regression.
Code Snippet: Predicting House Prices with Linear Regression
Here’s an example of how to predict house prices using linear regression in Scikit Learn:
from sklearn.linear_model import LinearRegression # Create an instance of LinearRegression regressor = LinearRegression() # Fit the regressor on the training data regressor.fit(X_train, y_train) # Predict the house prices for the testing data y_pred = regressor.predict(X_test)
Code Snippet: Predicting House Prices with Decision Tree Regression
Here’s an example of how to predict house prices using decision tree regression in Scikit Learn:
from sklearn.tree import DecisionTreeRegressor # Create an instance of DecisionTreeRegressor regressor = DecisionTreeRegressor() # Fit the regressor on the training data regressor.fit(X_train, y_train) # Predict the house prices for the testing data y_pred = regressor.predict(X_test)
Use Case: Image Recognition
Image recognition is a popular application of machine learning that involves identifying and classifying objects or patterns in images. Scikit Learn provides various classification algorithms that can be used for image recognition, such as logistic regression, support vector machines, and convolutional neural networks (CNN).
Code Snippet: Image Recognition with Logistic Regression
Here’s an example of how to perform image recognition using logistic regression in Scikit Learn:
from sklearn.linear_model import LogisticRegression # Create an instance of LogisticRegression classifier = LogisticRegression() # Flatten the images into a 1D array X_train_flat = X_train.reshape(X_train.shape[0], -1) X_test_flat = X_test.reshape(X_test.shape[0], -1) # Fit the classifier on the training data classifier.fit(X_train_flat, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test_flat)
Code Snippet: Image Recognition with Convolutional Neural Networks (CNN)
Here’s an example of how to perform image recognition using a convolutional neural network (CNN) in Scikit Learn:
from sklearn.neural_network import MLPClassifier # Create an instance of MLPClassifier with convolutional layers classifier = MLPClassifier(hidden_layer_sizes=(64, 128, 64), activation='relu', solver='adam', max_iter=100) # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Use Case: Text Classification
Text classification is the task of assigning predefined categories or labels to text documents. It has many applications, such as sentiment analysis, spam detection, and topic classification. Scikit Learn provides various algorithms for text classification, such as logistic regression, support vector machines, and naive Bayes.
Code Snippet: Text Classification with Logistic Regression
Here’s an example of how to perform text classification using logistic regression in Scikit Learn:
from sklearn.linear_model import LogisticRegression from sklearn.feature_extraction.text import TfidfVectorizer # Create an instance of TfidfVectorizer vectorizer = TfidfVectorizer() # Convert the text documents to a matrix of TF-IDF features X_train_tfidf = vectorizer.fit_transform(X_train) X_test_tfidf = vectorizer.transform(X_test) # Create an instance of LogisticRegression classifier = LogisticRegression() # Fit the classifier on the training data classifier.fit(X_train_tfidf, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test_tfidf)
Code Snippet: Text Classification with Support Vector Machines
Here’s an example of how to perform text classification using support vector machines (SVM) in Scikit Learn:
from sklearn.svm import SVC from sklearn.feature_extraction.text import TfidfVectorizer # Create an instance of TfidfVectorizer vectorizer = TfidfVectorizer() # Convert the text documents to a matrix of TF-IDF features X_train_tfidf = vectorizer.fit_transform(X_train) X_test_tfidf = vectorizer.transform(X_test) # Create an instance of SVC classifier = SVC() # Fit the classifier on the training data classifier.fit(X_train_tfidf, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test_tfidf)
Best Practice: Data Scaling
Data scaling is an important step in machine learning to ensure that features are on a similar scale. Scikit Learn provides various scaling techniques, such as standardization and normalization, to preprocess the data before training a model.
Standardization
Standardization is a scaling technique that transforms the data such that it has zero mean and unit variance. Scikit Learn provides the StandardScaler
class to perform standardization. Here’s an example:
from sklearn.preprocessing import StandardScaler # Create an instance of StandardScaler scaler = StandardScaler() # Fit the scaler on the training data scaler.fit(X_train) # Transform the training and testing data X_train_scaled = scaler.transform(X_train) X_test_scaled = scaler.transform(X_test)
Normalization
Normalization is a scaling technique that transforms the data such that it lies within a specific range, usually between 0 and 1. Scikit Learn provides the MinMaxScaler
class to perform normalization. Here’s an example:
from sklearn.preprocessing import MinMaxScaler # Create an instance of MinMaxScaler scaler = MinMaxScaler() # Fit the scaler on the training data scaler.fit(X_train) # Transform the training and testing data X_train_scaled = scaler.transform(X_train) X_test_scaled = scaler.transform(X_test)
Best Practice: Handling Imbalanced Data
Imbalanced data refers to a situation where the classes in a classification problem are not represented equally. Scikit Learn provides various techniques to handle imbalanced data, such as oversampling, undersampling, and using class weights.
Oversampling
Oversampling is a technique that increases the number of instances in the minority class to balance the class distribution. Scikit Learn provides the RandomOverSampler
class to perform oversampling. Here’s an example:
from imblearn.over_sampling import RandomOverSampler # Create an instance of RandomOverSampler oversampler = RandomOverSampler() # Resample the training data X_train_resampled, y_train_resampled = oversampler.fit_resample(X_train, y_train)
Undersampling
Undersampling is a technique that decreases the number of instances in the majority class to balance the class distribution. Scikit Learn provides the RandomUnderSampler
class to perform undersampling. Here’s an example:
from imblearn.under_sampling import RandomUnderSampler # Create an instance of RandomUnderSampler undersampler = RandomUnderSampler() # Resample the training data X_train_resampled, y_train_resampled = undersampler.fit_resample(X_train, y_train)
Using Class Weights
Class weights are used to assign higher weights to the minority class and lower weights to the majority class during model training. Scikit Learn provides the class_weight
parameter in various classifiers to automatically handle class weights. Here’s an example:
from sklearn.svm import SVC # Create an instance of SVC with balanced class weights classifier = SVC(class_weight='balanced') # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Best Practice: Feature Selection
Feature selection is the process of selecting a subset of relevant features from a dataset to improve the performance of a machine learning model. Scikit Learn provides various feature selection techniques, such as univariate feature selection, recursive feature elimination, and feature importance.
Univariate Feature Selection
Univariate feature selection selects the best features based on univariate statistical tests. Scikit Learn provides the SelectKBest
class to perform univariate feature selection. Here’s an example:
from sklearn.feature_selection import SelectKBest, f_regression # Create an instance of SelectKBest selector = SelectKBest(score_func=f_regression, k=5) # Fit the selector on the training data selector.fit(X_train, y_train) # Transform the training and testing data X_train_selected = selector.transform(X_train) X_test_selected = selector.transform(X_test)
Recursive Feature Elimination
Recursive feature elimination selects features by recursively considering smaller and smaller subsets of features. Scikit Learn provides the RFECV
class to perform recursive feature elimination. Here’s an example:
from sklearn.feature_selection import RFECV from sklearn.linear_model import LinearRegression # Create an instance of LinearRegression regressor = LinearRegression() # Create an instance of RFECV selector = RFECV(regressor) # Fit the selector on the training data selector.fit(X_train, y_train) # Transform the training and testing data X_train_selected = selector.transform(X_train) X_test_selected = selector.transform(X_test)
Real World Example: Predicting Credit Card Fraud
Predicting credit card fraud is a challenging task in the field of machine learning. The goal is to predict whether a credit card transaction is fraudulent or not based on various features such as the transaction amount, time, and location. Scikit Learn provides various classification algorithms that can be used for this task, such as logistic regression, random forest, and gradient boosting.
Code Snippet: Predicting Credit Card Fraud with Logistic Regression
Here’s an example of how to predict credit card fraud using logistic regression in Scikit Learn:
from sklearn.linear_model import LogisticRegression # Create an instance of LogisticRegression classifier = LogisticRegression() # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Code Snippet: Predicting Credit Card Fraud with Random Forest
Here’s an example of how to predict credit card fraud using random forest in Scikit Learn:
from sklearn.ensemble import RandomForestClassifier # Create an instance of RandomForestClassifier classifier = RandomForestClassifier() # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Real World Example: Recommender System
Recommender systems are widely used in e-commerce and entertainment platforms to provide personalized recommendations to users. Scikit Learn provides various algorithms for building recommender systems, such as collaborative filtering, content-based filtering, and matrix factorization.
Code Snippet: Collaborative Filtering
Here’s an example of how to build a recommender system using collaborative filtering in Scikit Learn:
from sklearn.metrics.pairwise import cosine_similarity # Compute the item-item similarity matrix item_similarity = cosine_similarity(X.T) # Compute the user-item recommendation matrix user_recommendation = np.dot(user_ratings, item_similarity) / np.sum(item_similarity, axis=1) # Get the top recommendations for a user user_id = 1 top_recommendations = np.argsort(user_recommendation[user_id])[::-1][:10]
Code Snippet: Content-based Filtering
Here’s an example of how to build a recommender system using content-based filtering in Scikit Learn:
from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.metrics.pairwise import cosine_similarity # Create an instance of TfidfVectorizer vectorizer = TfidfVectorizer() # Convert the item descriptions to a matrix of TF-IDF features item_features = vectorizer.fit_transform(item_descriptions) # Compute the item-item similarity matrix item_similarity = cosine_similarity(item_features) # Get the top recommendations for a user user_id = 1 user_profile = user_preferences[user_id] user_recommendation = np.dot(user_profile, item_similarity) / np.sum(item_similarity, axis=1) top_recommendations = np.argsort(user_recommendation)[::-1][:10]
Real World Example: Predicting Customer Churn
Predicting customer churn is an important problem in customer relationship management. The goal is to predict whether a customer is likely to churn or not based on various features such as their purchase history, customer service interactions, and demographic information. Scikit Learn provides various classification algorithms that can be used for this task, such as logistic regression, support vector machines, and random forest.
Code Snippet: Predicting Customer Churn with Logistic Regression
Here’s an example of how to predict customer churn using logistic regression in Scikit Learn:
from sklearn.linear_model import LogisticRegression # Create an instance of LogisticRegression classifier = LogisticRegression() # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Code Snippet: Predicting Customer Churn with Support Vector Machines
Here’s an example of how to predict customer churn using support vector machines (SVM) in Scikit Learn:
from sklearn.svm import SVC # Create an instance of SVC classifier = SVC() # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Performance Consideration: Algorithm Choice
The choice of algorithm can have a significant impact on the performance of a machine learning model. Scikit Learn provides a wide range of algorithms for different tasks, such as regression, classification, clustering, and dimensionality reduction. It is important to choose the right algorithm based on the specific problem and dataset.
Regression
For regression tasks, linear regression is a simple and often effective algorithm. However, if the relationship between the features and the target variable is nonlinear, decision tree regression or support vector regression may be more appropriate.
Classification
For binary classification tasks, logistic regression and support vector machines are commonly used algorithms. For multi-class classification tasks, algorithms such as decision tree classification, random forest classification, and gradient boosting can be effective.
Clustering
For clustering tasks, K-means clustering is a popular algorithm that is easy to implement. However, if the clusters have irregular shapes or different sizes, algorithms such as hierarchical clustering or DBSCAN may be more suitable.
Dimensionality Reduction
For dimensionality reduction tasks, PCA is a widely used algorithm that is particularly effective when the data has a linear structure. However, if the data has a nonlinear structure, algorithms such as t-SNE or UMAP may be more appropriate.
Performance Consideration: Data Size
The size of the dataset can have a significant impact on the performance of a machine learning model. Scikit Learn provides various techniques to handle large datasets, such as stochastic gradient descent, mini-batch learning, and incremental learning.
Stochastic Gradient Descent
Stochastic gradient descent is an optimization technique that updates the model parameters using a single instance or a small batch of instances at a time. It is particularly effective for large datasets as it can efficiently update the model parameters without requiring the entire dataset to be loaded into memory.
Mini-batch Learning
Mini-batch learning is a variation of stochastic gradient descent that updates the model parameters using a small random subset of the training data at a time. It strikes a balance between the efficiency of stochastic gradient descent and the stability of batch learning.
Incremental Learning
Incremental learning is a technique that updates the model parameters as new data becomes available. It is useful for handling streaming data or situations where the dataset cannot fit into memory. Scikit Learn provides the partial_fit
method in various classifiers to perform incremental learning.
Performance Consideration: Hardware Considerations
The performance of a machine learning model can also be influenced by the hardware on which it is running. Scikit Learn provides support for parallel processing and GPU acceleration to speed up model training and inference.
Parallel Processing
Parallel processing is a technique that divides the workload across multiple processors or cores to speed up computation. Scikit Learn provides the n_jobs
parameter in various functions and classes to enable parallel processing. By setting n_jobs
to -1
, Scikit Learn will automatically utilize all available processors.
GPU Acceleration
GPU acceleration is a technique that uses the computational power of graphics processing units (GPUs) to speed up model training and inference. Scikit Learn provides support for GPU acceleration through libraries such as CuPy and scikit-cuda. However, not all algorithms in Scikit Learn have GPU support, so it is important to check the documentation for each specific algorithm.
Advanced Technique: Ensemble Methods
Ensemble methods combine multiple individual models to make more accurate predictions. Scikit Learn provides various ensemble methods, such as bagging, boosting, and stacking.
Bagging
Bagging is an ensemble method that combines multiple models trained on different subsets of the training data. Scikit Learn provides the BaggingRegressor
and BaggingClassifier
classes to perform bagging for regression and classification tasks, respectively.
Boosting
Boosting is an ensemble method that combines multiple weak models into a strong model by sequentially training each model to correct the mistakes of the previous models. Scikit Learn provides the AdaBoostRegressor
and AdaBoostClassifier
classes to perform boosting for regression and classification tasks, respectively.
Stacking
Stacking is an ensemble method that combines multiple models by training a meta-model on their predictions. Scikit Learn provides the StackingRegressor
and StackingClassifier
classes to perform stacking for regression and classification tasks, respectively.
Advanced Technique: Feature Engineering
Feature engineering is the process of creating new features from existing ones to improve the performance of a machine learning model. Scikit Learn provides various feature engineering techniques, such as polynomial features, interaction terms, and feature selection.
Polynomial Features
Polynomial features are created by taking the powers and interactions of existing features. Scikit Learn provides the PolynomialFeatures
class to generate polynomial features. Here’s an example:
from sklearn.preprocessing import PolynomialFeatures # Create an instance of PolynomialFeatures poly = PolynomialFeatures(degree=2) # Generate polynomial features X_train_poly = poly.fit_transform(X_train) X_test_poly = poly.transform(X_test)
Interaction Terms
Interaction terms are created by multiplying pairs of existing features. Scikit Learn provides the PolynomialFeatures
class with the interaction_only
parameter set to True
to generate interaction terms. Here’s an example:
from sklearn.preprocessing import PolynomialFeatures # Create an instance of PolynomialFeatures poly = PolynomialFeatures(degree=2, interaction_only=True) # Generate interaction terms X_train_interaction = poly.fit_transform(X_train) X_test_interaction = poly.transform(X_test)
Advanced Technique: Neural Networks
Neural networks are a powerful class of machine learning models that are capable of learning complex patterns and relationships in data. Scikit Learn provides the MLPRegressor
and MLPClassifier
classes to build neural networks for regression and classification tasks, respectively.
Code Snippet: Neural Network for Regression
Here’s an example of how to build a neural network for regression using Scikit Learn:
from sklearn.neural_network import MLPRegressor # Create an instance of MLPRegressor regressor = MLPRegressor(hidden_layer_sizes=(64, 128, 64), activation='relu', solver='adam', max_iter=100) # Fit the regressor on the training data regressor.fit(X_train, y_train) # Predict the target variable for the testing data y_pred = regressor.predict(X_test)
Code Snippet: Neural Network for Classification
Here’s an example of how to build a neural network for classification using Scikit Learn:
from sklearn.neural_network import MLPClassifier # Create an instance of MLPClassifier classifier = MLPClassifier(hidden_layer_sizes=(64, 128, 64), activation='relu', solver='adam', max_iter=100) # Fit the classifier on the training data classifier.fit(X_train, y_train) # Predict the class labels for the testing data y_pred = classifier.predict(X_test)
Error Handling in Scikit Learn
Scikit Learn provides various error handling mechanisms to handle common issues that may arise during model training and evaluation, such as missing values, incompatible shapes, and invalid parameter values.
Handling Missing Values
Scikit Learn provides various techniques to handle missing values, such as imputation and removal. The SimpleImputer
class can be used to replace missing values with a suitable value, while the Dropna
class can be used to remove instances or features with missing values.
Handling Incompatible Shapes
Incompatible shapes occur when the dimensions of the input data and the model parameters do not match. Scikit Learn provides helpful error messages that indicate the mismatched dimensions, allowing you to identify and fix the issue.
Handling Invalid Parameter Values
Invalid parameter values occur when you pass incorrect values to the parameters of Scikit Learn functions and classes. Scikit Learn provides informative error messages that highlight the invalid values, helping you to correct them.
These error handling mechanisms ensure that potential issues are detected early and provide guidance on how to resolve them, allowing you to build more robust and reliable machine learning models.