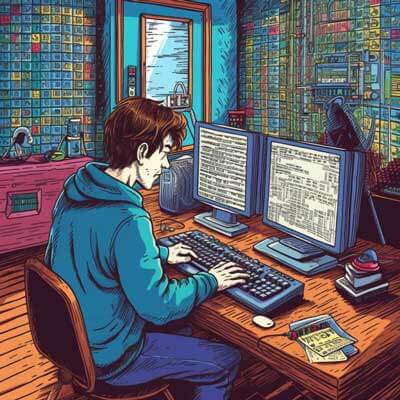
- Introduction to Pandas and Excel files
- Installing and Importing Libraries
- Reading Excel Files Using Pandas
- Different Methods to Load Excel Files
- Data Manipulation after Reading Excel Files
- Use Case: Analyzing Sales Data
- Use Case: Cleaning and Processing Survey Data
- Best Practice: Efficient Data Loading
- Best Practice: Handling Missing Data
- Real World Example: Financial Data Analysis
- Real World Example: Educational Data Processing
- Performance Consideration: Memory Usage
- Performance Consideration: Speed Optimization
- Advanced Technique: Custom Data Parsing
- Advanced Technique: Multi-indexing with Excel Files
- Code Snippet: Read Excel file with Pandas
- Code Snippet: Selecting Columns after Reading
- Code Snippet: Filtering Rows after Reading
- Code Snippet: Applying Functions to Data
- Code Snippet: Saving Processed Data to New Excel File
- Handling Errors while Reading Excel Files
Introduction to Pandas and Excel files
Pandas is a powerful data manipulation library in Python that provides easy-to-use data structures and data analysis tools. It is widely used for tasks such as data cleaning, data transformation, and data analysis. One common task in data analysis is working with Excel files, as Excel is a popular format for storing and organizing data.
In this chapter, we will explore how to use Pandas to read Excel files in Python. We will cover various methods to load Excel files, manipulate the data after reading, and handle common scenarios encountered while working with Excel data.
Related Article: How To Read JSON From a File In Python
Installing and Importing Libraries
Before we can start working with Pandas and Excel files, we need to ensure that the necessary libraries are installed and imported into our Python environment.
To install Pandas, we can use pip, the Python package installer, by running the following command in the terminal:
pip install pandas
In addition to Pandas, we also need to install the openpyxl library, which provides support for reading and writing Excel files in the .xlsx format. We can install it by running the following command:
pip install openpyxl
Once we have installed the required libraries, we can import them into our Python script using the following import statements:
import pandas as pd import openpyxl
Now that we have Pandas and openpyxl installed and imported, we are ready to start reading Excel files using Pandas.
Reading Excel Files Using Pandas
Pandas provides a convenient function called read_excel()
that allows us to read Excel files into a Pandas DataFrame. The read_excel()
function supports various parameters to customize the import process, such as specifying the sheet name, skipping rows or columns, and selecting specific columns to import.
Here is an example of how to use the read_excel()
function to read an Excel file named “data.xlsx” located in the current directory:
df = pd.read_excel("data.xlsx")
This will read the contents of the Excel file into a DataFrame named df
.
Different Methods to Load Excel Files
In addition to the basic read_excel()
function, Pandas provides several other methods to load Excel files with different configurations and options. Let’s explore two common methods: loading specific sheets and skipping rows.
Loading Specific Sheets
Sometimes, an Excel file contains multiple sheets, and we may only be interested in loading a specific sheet. We can achieve this by passing the sheet name or index to the read_excel()
function.
Here is an example of how to load the sheet named “Sheet1” from an Excel file:
df = pd.read_excel("data.xlsx", sheet_name="Sheet1")
Skipping Rows
In some cases, an Excel file may have header rows or other irrelevant rows that we want to skip during the import process. We can use the skiprows
parameter to specify the number of rows to skip.
Here is an example of how to skip the first two rows of an Excel file:
df = pd.read_excel("data.xlsx", skiprows=2)
These are just a few examples of the different methods available to load Excel files using Pandas. Depending on the specific requirements of your data, you can select the most appropriate method to load the data efficiently.
Related Article: How to Convert JSON to CSV in Python
Data Manipulation after Reading Excel Files
Once we have loaded an Excel file into a Pandas DataFrame, we can perform various data manipulation tasks on the data. Pandas provides a rich set of functions and methods to handle common data manipulation operations, such as filtering rows, selecting columns, applying functions to data, and more.
In this chapter, we will explore some of the most commonly used data manipulation techniques using Pandas after reading Excel files.
Use Case: Analyzing Sales Data
Let’s consider a scenario where we have an Excel file containing sales data for a company. The data includes columns such as “Date”, “Product”, “Quantity”, and “Revenue”. Our goal is to analyze the sales data and gain insights into the company’s performance.
Selecting Columns after Reading
To select specific columns from a DataFrame, we can use the square bracket notation and pass a list of column names.
Here is an example of how to select the “Date” and “Revenue” columns from the sales data:
selected_columns = df[["Date", "Revenue"]]
Filtering Rows after Reading
To filter rows based on specific conditions, we can use boolean indexing. We create a boolean mask by applying a condition to a column, and then use that mask to filter the DataFrame.
Here is an example of how to filter the sales data to only include rows where the revenue is greater than 1000:
filtered_data = df[df["Revenue"] > 1000]
Applying Functions to Data
Pandas allows us to apply functions to columns or rows of a DataFrame using the apply()
method. We can pass a custom function or a built-in function to perform calculations or transformations on the data.
Here is an example of how to calculate the total revenue for each product:
def calculate_total_revenue(row): return row["Quantity"] * row["Revenue"] df["Total Revenue"] = df.apply(calculate_total_revenue, axis=1)
These are just a few examples of the data manipulation techniques that can be applied after reading Excel files using Pandas. Depending on the specific requirements of your data analysis task, you can explore the rich functionality provided by Pandas to manipulate and transform the data effectively.
Use Case: Cleaning and Processing Survey Data
In addition to analyzing sales data, Pandas can also be used for cleaning and processing survey data. Surveys often contain missing values, inconsistent formatting, and other data quality issues that need to be addressed before analysis.
Let’s consider a scenario where we have an Excel file containing survey responses. The data includes columns such as “Name”, “Age”, “Gender”, and “Response”. Our goal is to clean the data and extract meaningful insights from the survey responses.
Handling Missing Data
Missing data is a common issue in survey data. Pandas provides various functions and methods to handle missing data, such as fillna()
to fill missing values and dropna()
to drop rows or columns with missing values.
Here is an example of how to fill missing values in the “Age” column with the mean age:
mean_age = df["Age"].mean() df["Age"].fillna(mean_age, inplace=True)
Efficient Data Loading
When working with large Excel files, loading the entire file into memory can be memory-intensive and slow. Pandas provides options to load data in chunks using the chunksize
parameter of the read_excel()
function.
Here is an example of how to load data in chunks of 1000 rows:
chunk_iter = pd.read_excel("data.xlsx", chunksize=1000) for chunk in chunk_iter: # Perform data processing on each chunk ...
These are just a few examples of the techniques that can be applied to clean and process survey data using Pandas after reading Excel files. Pandas provides a comprehensive set of tools to handle various data quality issues and extract meaningful insights from survey data.
Related Article: How to Read Xlsx File Using Pandas Library in Python
Best Practice: Efficient Data Loading
Efficient data loading is crucial when working with large Excel files or when dealing with limited computing resources. Loading data efficiently can help reduce memory usage and improve processing speed.
In this chapter, we will explore some best practices for efficient data loading using Pandas when reading Excel files.
Specify Data Types
By default, Pandas infers the data types of columns while reading Excel files. However, inferring data types can be time-consuming and memory-intensive for large datasets. To improve performance, we can specify the data types of columns explicitly using the dtype
parameter of the read_excel()
function.
Here is an example of how to specify the data types of columns while reading an Excel file:
df = pd.read_excel("data.xlsx", dtype={"Quantity": int, "Revenue": float})
Use Filters to Load Relevant Data
In some cases, an Excel file may contain multiple sheets or a large number of rows and columns. Loading the entire file into memory may not be necessary if we only need a subset of the data. We can use filters to specify the relevant sheets, rows, or columns to load, reducing the memory footprint.
Here is an example of how to load a specific sheet and select only the required columns from an Excel file:
df = pd.read_excel("data.xlsx", sheet_name="Sheet1", usecols=["Date", "Revenue"])
These are just a few examples of the best practices for efficient data loading using Pandas when reading Excel files. By following these practices, we can optimize the loading process and improve the performance of our data analysis tasks.
Best Practice: Handling Missing Data
Missing data is a common issue in data analysis tasks, including when working with Excel files. Pandas provides various techniques to handle missing data effectively and ensure accurate analysis results.
In this chapter, we will explore some best practices for handling missing data when reading Excel files using Pandas.
Identify Missing Values
Before handling missing data, it is essential to identify and understand the missing values in the dataset. Pandas provides the isna()
and isnull()
functions to detect missing values in a DataFrame.
Here is an example of how to identify missing values in a DataFrame:
missing_values = df.isna().sum()
Fill Missing Values
One approach to handling missing data is to fill the missing values with appropriate values. Pandas provides the fillna()
function to fill missing values with a specified value or using various filling techniques, such as forward filling or backward filling.
Here is an example of how to fill missing values in a DataFrame with the mean value of the column:
mean_values = df.mean() df.fillna(mean_values, inplace=True)
Drop Missing Values
Another approach to handling missing data is to drop rows or columns with missing values. Pandas provides the dropna()
function to remove rows or columns with missing values.
Here is an example of how to drop rows with missing values in a DataFrame:
df.dropna(axis=0, inplace=True)
These are just a few examples of the best practices for handling missing data when reading Excel files using Pandas. By understanding and addressing missing values appropriately, we can ensure reliable and accurate data analysis results.
Real World Example: Financial Data Analysis
To demonstrate how to apply Pandas to real-world scenarios, let’s consider an example of analyzing financial data from an Excel file. The data includes columns such as “Date”, “Stock”, “Price”, and “Volume”. Our goal is to calculate various financial metrics and gain insights into the stock performance.
Calculating Daily Returns
One common financial metric is the daily return, which measures the percentage change in stock price from one day to the next. We can calculate the daily returns using the pct_change()
method in Pandas.
Here is an example of how to calculate the daily returns for a stock:
df["Daily Return"] = df["Price"].pct_change()
Calculating Moving Averages
Moving averages are used to smooth out fluctuations in stock prices and identify trends over a specific period. We can calculate moving averages using the rolling()
method in Pandas.
Here is an example of how to calculate the 30-day moving average for a stock:
df["30-day Moving Average"] = df["Price"].rolling(window=30).mean()
These are just a few examples of the financial analysis techniques that can be applied using Pandas after reading financial data from an Excel file. By leveraging the powerful data manipulation capabilities of Pandas, we can gain valuable insights into financial data and make informed investment decisions.
Related Article: How To Write Pandas Dataframe To CSV File
Real World Example: Educational Data Processing
In addition to financial data analysis, Pandas can also be used to process educational data. Let’s consider an example of processing student performance data from an Excel file. The data includes columns such as “Name”, “Age”, “Subject”, and “Grade”. Our goal is to analyze the student performance and identify trends based on different subjects.
Grouping and Aggregating Data
To analyze student performance by subject, we can group the data by the “Subject” column and calculate various statistics using the groupby()
and agg()
methods in Pandas.
Here is an example of how to calculate the average grade for each subject:
subject_average = df.groupby("Subject")["Grade"].mean()
Plotting Data
Visualizing educational data can provide valuable insights into student performance. Pandas integrates with popular data visualization libraries like Matplotlib and Seaborn to create various types of plots, such as bar plots, line plots, and scatter plots.
Here is an example of how to create a bar plot to visualize the average grade for each subject:
import matplotlib.pyplot as plt subject_average.plot(kind="bar") plt.xlabel("Subject") plt.ylabel("Average Grade") plt.title("Average Grade by Subject") plt.show()
These are just a few examples of how Pandas can be used to process and analyze educational data after reading it from an Excel file. By leveraging the data manipulation and visualization capabilities of Pandas, we can gain insights into student performance and make data-driven decisions in the educational domain.
Performance Consideration: Memory Usage
When working with large Excel files or limited computing resources, it is essential to consider memory usage and optimize it to ensure efficient data processing. Pandas provides several techniques to reduce memory usage when reading Excel files.
Specify Data Types
Specifying data types explicitly while reading Excel files can help reduce memory usage. By default, Pandas infers the data types, which may result in larger memory allocations. We can use the dtype
parameter of the read_excel()
function to specify the data types of columns.
Here is an example of how to specify the data types while reading an Excel file:
df = pd.read_excel("data.xlsx", dtype={"Quantity": int, "Revenue": float})
Use Categorical Data Type
When working with columns that have a limited number of unique values, converting them to the categorical data type can significantly reduce memory usage. The categorical data type represents the data more efficiently by storing the unique values internally and using integer codes to represent the values.
Here is an example of how to convert a column to the categorical data type:
df["Category"] = df["Category"].astype("category")
These are just a few examples of the techniques that can be applied to reduce memory usage when reading Excel files using Pandas. By optimizing memory usage, we can process larger datasets and improve the overall performance of our data analysis tasks.
Performance Consideration: Speed Optimization
In addition to memory usage, optimizing speed is crucial when working with large Excel files or performing time-sensitive data analysis tasks. Pandas provides various techniques to improve processing speed when reading Excel files.
Use read_excel() Parameters
The read_excel()
function in Pandas provides several parameters that can be tweaked to improve speed. For example, setting the engine
parameter to “openpyxl” can often provide faster performance compared to the default engine.
Here is an example of how to specify the engine while reading an Excel file:
df = pd.read_excel("data.xlsx", engine="openpyxl")
Use Chunked Reading
For extremely large Excel files, loading the entire file into memory may not be feasible. In such cases, we can use the chunksize
parameter of the read_excel()
function to read the data in smaller chunks.
Here is an example of how to read an Excel file in chunks:
chunk_iter = pd.read_excel("data.xlsx", chunksize=1000) for chunk in chunk_iter: # Perform data processing on each chunk ...
These are just a few examples of the techniques that can be applied to optimize speed when reading Excel files using Pandas. By fine-tuning the import process and using chunked reading, we can significantly improve the performance of our data analysis tasks.
Advanced Technique: Custom Data Parsing
Pandas provides powerful built-in functionality for parsing various data types from Excel files. However, in some cases, the default parsing may not be sufficient for specialized data formats. In such cases, we can use custom data parsing techniques to extract the desired information.
Using Custom Functions
Pandas allows us to define custom functions and apply them to the data during the import process. By leveraging the flexibility of Python, we can implement complex parsing logic and extract specific data elements.
Here is an example of how to use a custom function to parse a specific column during the import process:
def parse_custom_data(value): # Custom parsing logic ... df = pd.read_excel("data.xlsx", converters={"CustomColumn": parse_custom_data})
Using Regular Expressions
Regular expressions can be powerful tools for data parsing. Pandas provides the read_excel()
function with the converters
parameter, which allows us to specify regular expressions to extract desired patterns from the data.
Here is an example of how to use a regular expression to parse a specific column during the import process:
import re def parse_custom_data(value): pattern = r"(\d+)" match = re.search(pattern, value) if match: return match.group(1) else: return None df = pd.read_excel("data.xlsx", converters={"CustomColumn": parse_custom_data})
These are just a few examples of the advanced techniques that can be applied to perform custom data parsing when reading Excel files using Pandas. By utilizing custom functions and regular expressions, we can extract specific information from complex data formats.
Advanced Technique: Multi-indexing with Excel Files
Multi-indexing allows us to work with hierarchical index structures, which can be useful for organizing and analyzing complex datasets. Pandas provides robust support for multi-indexing, including reading and writing Excel files with multi-indexing.
Creating Multi-index
To create a multi-index, we can use the pd.MultiIndex.from_arrays()
or pd.MultiIndex.from_tuples()
functions in Pandas. These functions allow us to specify the levels and labels for each level of the index.
Here is an example of how to create a multi-index from arrays:
import pandas as pd index_data = [["A", "A", "B", "B"], [1, 2, 1, 2]] multi_index = pd.MultiIndex.from_arrays(index_data, names=["Letter", "Number"])
Reading Excel Files with Multi-indexing
When reading Excel files with multi-indexing, we need to specify the header
parameter of the read_excel()
function to indicate the row(s) that contain the column names. We can set the header
parameter to an integer or a list of integers to skip the appropriate number of rows.
Here is an example of how to read an Excel file with multi-indexing:
df = pd.read_excel("data.xlsx", header=[0, 1])
Accessing Multi-indexed Data
Once we have a multi-indexed DataFrame, we can access and manipulate the data using the loc
indexer. The loc
indexer allows us to specify the levels and labels of the index to select specific rows or columns.
Here is an example of how to access data from a multi-indexed DataFrame:
selected_data = df.loc[("A", 1), "Column1"]
These are just a few examples of the advanced techniques that can be applied to work with multi-indexing in Excel files using Pandas. By leveraging the powerful multi-indexing capabilities of Pandas, we can organize and analyze complex datasets effectively.
Code Snippet: Read Excel file with Pandas
import pandas as pd df = pd.read_excel("data.xlsx")
This code snippet demonstrates how to read an Excel file named “data.xlsx” using Pandas. After reading the Excel file, the data is stored in a Pandas DataFrame named df
, which we can then manipulate and analyze further.
Code Snippet: Selecting Columns after Reading
selected_columns = df[["Column1", "Column2"]]
This code snippet demonstrates how to select specific columns from a DataFrame named df
after reading an Excel file. The selected columns are specified using the square bracket notation and a list of column names.
Code Snippet: Filtering Rows after Reading
filtered_data = df[df["Column1"] > 100]
This code snippet demonstrates how to filter rows based on a condition after reading an Excel file. The condition is applied to the “Column1” column of the DataFrame df
, resulting in a new DataFrame named filtered_data
that only includes rows where the “Column1” value is greater than 100.
Code Snippet: Applying Functions to Data
def custom_function(row): return row["Column1"] * 2 df["NewColumn"] = df.apply(custom_function, axis=1)
This code snippet demonstrates how to apply a custom function to each row of a DataFrame named df
after reading an Excel file. The custom function is defined to multiply the value in the “Column1” column by 2, and the result is stored in a new column named “NewColumn”.
Code Snippet: Saving Processed Data to New Excel File
df.to_excel("processed_data.xlsx", index=False)
This code snippet demonstrates how to save a DataFrame named df
containing processed data to a new Excel file named “processed_data.xlsx”. The to_excel()
function is used, and the index
parameter is set to False
to exclude the index from the saved Excel file.
Handling Errors while Reading Excel Files
When reading Excel files using Pandas, it is essential to handle errors that may occur during the import process. Errors can arise due to various reasons, such as file not found, incorrect file format, or incompatible data types.
To handle errors while reading Excel files, we can use exception handling techniques in Python. By wrapping the reading code in a try-except block, we can catch and handle specific types of errors that may occur.
Here is an example of how to handle errors while reading an Excel file:
try: df = pd.read_excel("data.xlsx") except FileNotFoundError: print("File not found!") except pd.errors.ParserError: print("Error while parsing the Excel file!") except Exception as e: print("An error occurred:", str(e))
In this example, we catch specific types of errors, such as FileNotFoundError
and ParserError
, using separate except
blocks. For any other types of errors, we catch them using the general Exception
class and print the error message.
These are just a few examples of how to handle errors while reading Excel files using Pandas. By implementing robust error handling mechanisms, we can ensure that our code gracefully handles any unexpected situations and provides meaningful feedback to the user.