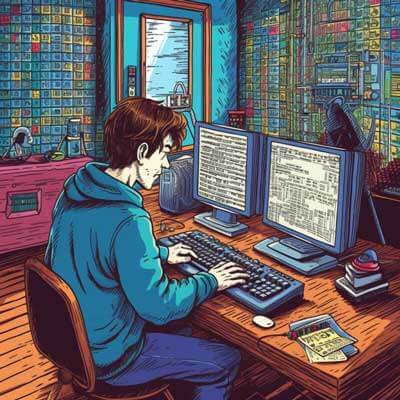
Table of Contents
The ternary operator in Python is a useful shorthand way to write conditional expressions. It allows you to write a concise if-else statement in a single line of code. In this answer, we will explore the syntax and usage of the ternary operator in Python.
Why is the ternary operator used in Python?
The ternary operator is commonly used in Python to simplify conditional expressions. It provides a concise way to write if-else statements, especially when the conditions are simple and the resulting expressions are short.
The ternary operator is particularly useful in situations where you need to assign a value or perform a simple operation based on a condition. It can improve code readability and reduce the number of lines of code, making the code more compact and easier to understand.
Related Article: How to Check for an Empty String in Python
Syntax of the ternary operator in Python
The syntax of the ternary operator in Python is as follows:
value_if_true if condition else value_if_false
Here, condition
is the expression that evaluates to either True
or False
. If the condition is True
, the expression value_if_true
is evaluated and returned. Otherwise, the expression value_if_false
is evaluated and returned.
The ternary operator is a concise alternative to writing if-else statements in Python. It allows you to perform a simple conditional check and return a value based on the result of that check.
Examples of using the ternary operator in Python
Let's look at some examples to understand how to use the ternary operator in Python.
Example 1: Assigning a value based on a condition
x = 10 result = "Even" if x % 2 == 0 else "Odd" print(result) # Output: Even
In this example, we use the ternary operator to assign the value "Even" to the variable result
if the value of x
is even (i.e., x % 2
equals 0). Otherwise, the value "Odd" is assigned to result
. The output of this code snippet is "Even" because 10 is an even number.
Example 2: Performing a simple operation based on a condition
x = 5 result = x * 2 if x > 0 else 0 print(result) # Output: 10
In this example, we use the ternary operator to multiply the value of x
by 2 if x
is greater than 0. Otherwise, the value 0 is assigned to result
. The output of this code snippet is 10 because 5 is greater than 0, and result
is assigned the value of x * 2
, which is 10.
Best practices and considerations
When using the ternary operator in Python, it is important to keep the following best practices and considerations in mind:
1. Keep the expressions short and simple: The ternary operator is most effective when the conditions and expressions are simple and concise. If the expressions become too complex, it can make the code harder to read and understand. In such cases, it is recommended to use if-else statements instead.
2. Use parentheses for clarity: It is a good practice to use parentheses around the conditions to improve readability and avoid potential confusion. For example:
result = (value_if_true if condition else value_if_false)
3. Avoid nesting ternary operators: While it is possible to nest ternary operators, it can quickly become unreadable and difficult to follow. It is generally better to use if-else statements or separate lines of code when dealing with complex conditions or expressions.
4. Consider using if-else statements for multiple conditions: If you have multiple conditions to check, it may be more readable to use if-else statements instead of chaining multiple ternary operators. This can make the code easier to understand and maintain.
5. Use meaningful variable and expression names: When using the ternary operator, it is important to use meaningful variable names and expressions to improve code clarity. This will make it easier for others (and yourself) to understand the purpose and behavior of the code.