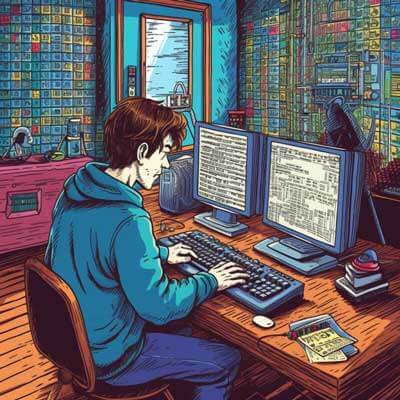
Table of Contents
Integrating Django with React
React is a popular JavaScript library for building user interfaces, and it can be seamlessly integrated with Django to create useful web applications. To integrate Django with React, follow these steps:
1. Install Django and create a new Django project:
$ pip install django $ django-admin startproject myproject
2. Create a new Django app:
$ python manage.py startapp myapp
3. Install the required dependencies for React:
$ npm install react react-dom
4. Create a new directory for your React code inside your Django app:
$ mkdir myapp/static/myapp/js
5. Create a new file called index.js
inside the js
directory and write your React code:
import React from 'react'; import ReactDOM from 'react-dom'; const App = () => { return ( <div> <h1>Hello, React!</h1> </div> ); }; ReactDOM.render(, document.getElementById('root'));
6. Create a new HTML template file called index.html
inside your Django app's templates
directory:
<title>My Django React App</title> <div id="root"></div>
7. Update your Django app's views.py
file to render the index.html
template:
from django.shortcuts import render def index(request): return render(request, 'myapp/index.html')
8. Update your Django app's urls.py
file to map the index
view to the root URL:
from django.urls import path from . import views urlpatterns = [ path('', views.index, name='index'), ]
9. Start the Django development server:
$ python manage.py runserver
Now, when you visit http://localhost:8000
, you should see your React app integrated with Django.
Related Article: Tutorial: Subprocess Popen in Python
Integrating Django with Vue
Vue is another popular JavaScript framework that can be integrated with Django to build modern web applications. Here's how you can integrate Django with Vue:
1. Install Django and create a new Django project:
$ pip install django $ django-admin startproject myproject
2. Create a new Django app:
$ python manage.py startapp myapp
3. Install Vue and the Vue CLI:
$ npm install vue vue-router $ npm install -g @vue/cli
4. Create a new Vue project inside your Django app's directory:
$ cd myapp $ vue create .
5. Choose the default preset and let the Vue CLI scaffold the project for you.
6. Update your Django app's views.py
file to render the Vue app:
from django.shortcuts import render def index(request): return render(request, 'myapp/index.html')
7. Update your Django app's urls.py
file to map the index
view to the root URL:
from django.urls import path from . import views urlpatterns = [ path('', views.index, name='index'), ]
8. Create a new HTML template file called index.html
inside your Django app's templates
directory:
<title>My Django Vue App</title> <div id="app"></div>
9. Update the main.js
file inside the src
directory of your Vue project:
import Vue from 'vue' import App from './App.vue' import router from './router' Vue.config.productionTip = false new Vue({ router, render: h => h(App) }).$mount('#app')
10. Start the Django development server:
$ python manage.py runserver
Now, when you visit http://localhost:8000
, you should see your Vue app integrated with Django.
Integrating Django with Angular
Angular is a useful frontend framework that can be integrated with Django to build scalable web applications. Here's how you can integrate Django with Angular:
1. Install Django and create a new Django project:
$ pip install django $ django-admin startproject myproject
2. Create a new Django app:
$ python manage.py startapp myapp
3. Install Angular CLI:
$ npm install -g @angular/cli
4. Create a new Angular project inside your Django app's directory:
$ cd myapp $ ng new frontend
5. Update your Django app's views.py
file to render the Angular app:
from django.shortcuts import render def index(request): return render(request, 'myapp/index.html')
6. Update your Django app's urls.py
file to map the index
view to the root URL:
from django.urls import path from . import views urlpatterns = [ path('', views.index, name='index'), ]
7. Create a new HTML template file called index.html
inside your Django app's templates
directory:
<title>My Django Angular App</title>
8. Start the Django development server:
$ python manage.py runserver
Now, when you visit http://localhost:8000
, you should see your Angular app integrated with Django.
Strategies for integrating single-page applications (SPA) with Django
Integrating single-page applications (SPA) with Django requires careful planning and consideration. Here are some strategies for integrating SPAs with Django:
1. Separate Backend and Frontend: In this strategy, the Django backend and the SPA frontend are developed and deployed separately. The frontend is hosted on a different server or on a static file hosting service, while the Django backend serves as the API backend for the SPA. The frontend communicates with the backend using RESTful APIs or GraphQL.
2. Django Template Integration: Django provides a useful templating engine that can be used to render server-side HTML templates. In this strategy, the Django backend serves the initial HTML page, which includes the necessary JavaScript and CSS files for the SPA. The SPA then takes over the client-side rendering and navigation.
3. Django REST framework: Django REST framework (DRF) is a popular library for building RESTful APIs in Django. Using DRF, you can build a robust API backend that can be consumed by any SPA frontend framework. The SPA communicates with the backend using AJAX requests to fetch and update data.
Related Article: How to Implement a Python Foreach Equivalent
Using WebSockets with Django using Channels and Daphne
WebSockets provide a bidirectional communication channel between the client and the server, allowing real-time updates and push notifications. Django provides a useful library called Channels for working with WebSockets. Here's how you can use WebSockets with Django using Channels and Daphne:
1. Install Channels and Daphne:
$ pip install channels daphne
2. Update your Django project's settings.py file to include Channels:
INSTALLED_APPS = [ ... 'channels', ... ] ASGI_APPLICATION = 'myproject.routing.application'
3. Create a new file called routing.py in your Django project's directory:
from channels.routing import ProtocolTypeRouter, URLRouter from django.urls import path from myapp.consumers import MyConsumer application = ProtocolTypeRouter({ 'http': get_asgi_application(), 'websocket': URLRouter([ path('ws/mywebsocket/', MyConsumer.as_asgi()), ]), })
4. Create a new file called consumers.py in your Django app's directory:
from channels.generic.websocket import AsyncWebsocketConsumer class MyConsumer(AsyncWebsocketConsumer): async def connect(self): await self.accept() async def disconnect(self, close_code): pass async def receive(self, text_data): await self.send(text_data=f"Received: {text_data}")
5. Start the Daphne server:
$ daphne myproject.routing:application
Now, you can use WebSockets in your Django app by connecting to ws://localhost:8000/ws/mywebsocket/
.
The role of Channels and Daphne in Django's WebSocket integration
Channels and Daphne play a crucial role in Django's WebSocket integration. Channels is a Django library that extends the capabilities of Django to handle WebSockets, while Daphne is a high-performance ASGI server that can serve Django applications, including those using Channels.
Channels provides a higher-level API for working with WebSockets in Django, making it easier to handle connections, send and receive messages, and manage state. It allows you to define WebSocket consumers, which are classes that handle WebSocket connections and events. Consumers can be asynchronous, allowing you to easily perform long-running tasks or interact with external services.
Daphne, on the other hand, is responsible for serving the Django application that uses Channels. It is designed to handle high-concurrency WebSocket connections efficiently and can scale to support thousands of connections.
Together, Channels and Daphne provide a useful and scalable solution for adding real-time capabilities to your Django applications.
Benefits of using GraphQL with Django
GraphQL is a query language for APIs that provides a flexible and efficient way to request and manipulate data. When used with Django, GraphQL offers several benefits:
1. Fine-grained control over data fetching: With GraphQL, clients can specify exactly what data they need, reducing over-fetching and under-fetching of data. This improves performance by minimizing the amount of data transferred over the network.
2. Reduced number of API requests: GraphQL allows clients to fetch multiple resources in a single request, reducing the number of round trips to the server. This improves efficiency and reduces latency.
3. Strong typing and introspection: GraphQL schemas are strongly typed, which provides better documentation and validation of the data. Clients can introspect the schema to discover available types and fields, making it easier to build robust and maintainable applications.
4. Rapid development and iteration: GraphQL's declarative nature allows frontend and backend developers to work independently and iterate quickly. Frontend developers can request the exact data they need without relying on backend changes, while backend developers can evolve the API schema without breaking existing clients.
5. Ecosystem and tooling: GraphQL has a vibrant ecosystem with a wide range of tools and libraries for client and server development. There are GraphQL clients available for various frontend frameworks, making it easy to integrate GraphQL with your preferred frontend technology.
Overall, using GraphQL with Django can improve the efficiency, flexibility, and developer experience of your web applications.
Implementing GraphQL in Django projects
To implement GraphQL in Django projects, follow these steps:
1. Install the required dependencies:
$ pip install graphene-django
2. Create a new Django app for your GraphQL schema:
$ python manage.py startapp mygraphqlapp
3. Define your GraphQL schema in a new file called schema.py
inside your Django app:
import graphene class Query(graphene.ObjectType): hello = graphene.String() def resolve_hello(self, info): return 'Hello, GraphQL!' schema = graphene.Schema(query=Query)
4. Update your Django app's urls.py
file to include the GraphQL view:
from django.urls import path from graphene_django.views import GraphQLView urlpatterns = [ path('graphql/', GraphQLView.as_view(graphiql=True)), ]
5. Start the Django development server:
$ python manage.py runserver
Now, when you visit http://localhost:8000/graphql/
, you can run GraphQL queries against your Django app.
Related Article: How to Use Inline If Statements for Print in Python
Advantages of using React, Vue, or Angular with Django
Integrating React, Vue, or Angular with Django offers several advantages:
1. Enhanced user experience: React, Vue, and Angular provide useful frontend capabilities that can enhance the user experience of your Django applications. These frameworks allow you to build interactive and dynamic user interfaces, providing a more engaging and responsive experience for your users.
2. Efficient development process: React, Vue, and Angular have a large ecosystem of libraries, tools, and community support, which can greatly simplify the development process. These frameworks provide well-defined patterns and best practices, making it easier to build scalable and maintainable code.
3. Separation of concerns: By separating the frontend and backend concerns, you can create a more modular and maintainable codebase. Django can focus on handling the server-side logic and data management, while React, Vue, or Angular can handle the presentation and user interface.
4. Reusability of components: React, Vue, and Angular promote the reusability of components, allowing you to build modular and composable UI elements. This can improve development speed and code quality by reducing duplication and promoting code reuse.
5. Ecosystem and community support: React, Vue, and Angular have large and active communities, which means you can find plenty of resources, tutorials, and libraries to help you in your development journey. These communities also provide ongoing support and updates, ensuring that your frontend framework remains up-to-date and secure.
Overall, using React, Vue, or Angular with Django can help you build modern and scalable web applications with improved user experience and development efficiency.
Differences between React, Vue, and Angular in Django integration
While React, Vue, and Angular can all be used to integrate with Django, there are some differences in how they are integrated and their respective features. Here are some key differences:
1. React: React is a JavaScript library for building user interfaces. It is known for its simplicity and flexibility. React can be easily integrated with Django using the Django templating system or by creating a separate frontend application that communicates with the Django backend using RESTful APIs or GraphQL. React provides a component-based architecture and a virtual DOM, which allows for efficient rendering and updates.
2. Vue: Vue is a JavaScript framework for building user interfaces. It is designed to be easy to learn and use, making it a popular choice for beginners. Vue can be integrated with Django using the Django templating system or by creating a separate frontend application that communicates with the Django backend using RESTful APIs or GraphQL. Vue provides a reactive data model and a component-based architecture, similar to React.
3. Angular: Angular is a full-featured frontend framework developed by Google. It provides a complete solution for building large-scale applications with a rich set of features. Angular can be integrated with Django by creating a separate frontend application that communicates with the Django backend using RESTful APIs or GraphQL. Angular has a useful dependency injection system and supports two-way data binding, making it suitable for complex applications.
The choice between React, Vue, and Angular depends on your specific requirements and preferences. React and Vue are often favored for their simplicity and ease of use, while Angular is preferred for its comprehensive feature set and scalability.
Best practices for integrating Django with frontend frameworks
When integrating Django with frontend frameworks like React, Vue, or Angular, it's important to follow best practices to ensure a smooth and maintainable development process. Here are some best practices to consider:
1. Separate backend and frontend concerns: Keep the backend and frontend code separate to achieve a clear separation of concerns. Use Django for server-side logic and data management, and the frontend framework for user interface and presentation logic.
2. Use APIs for communication: Use RESTful APIs or GraphQL to communicate between the Django backend and the frontend framework. This allows for loose coupling and enables different parts of the application to evolve independently.
3. Leverage Django's template system: If you prefer a server-side rendering approach, use Django's template system to render HTML templates that include the necessary JavaScript and CSS files for the frontend framework. This allows for easier integration and maintenance of the frontend.
4. Follow modular and component-based development: React, Vue, and Angular all promote modular and component-based development. Break down your application into reusable components to improve code reusability and maintainability.
5. Use package managers and build tools: Utilize package managers like npm or yarn to manage frontend dependencies. Use build tools like webpack or Parcel to bundle and optimize your frontend code.
6. Automate deployment and testing: Use automated deployment tools like Docker or CI/CD pipelines to streamline the deployment process. Implement automated testing for both the frontend and backend to ensure the stability and quality of your application.
Considerations when using WebSockets with Django
When using WebSockets with Django, there are several considerations to keep in mind:
1. Scalability: WebSockets can open a large number of concurrent connections, which can put a strain on your server's resources. Consider using a high-performance ASGI server like Daphne to handle the increased load.
2. Authentication and authorization: Ensure that your WebSocket connections are properly authenticated and authorized to prevent unauthorized access. Channels provides built-in support for authentication and authorization.
3. Real-time updates: WebSockets allow for real-time updates, which means that your server needs to handle and process updates efficiently. Consider using asynchronous programming techniques and optimizing your code for performance.
4. Message handling: WebSockets allow for bidirectional communication, which means that the server can send messages to the client and vice versa. Handle incoming messages carefully and validate them to prevent security vulnerabilities.
5. Compatibility: WebSockets may not be supported in all browsers or network environments. Consider providing fallback mechanisms or alternative communication channels for clients that do not support WebSockets.
6. Testing and debugging: WebSockets can be more challenging to test and debug compared to traditional HTTP requests. Use tools and libraries that provide WebSocket testing and debugging capabilities to simplify the process.
Related Article: How to Update and Upgrade Pip Inside a Python Virtual Environment
Additional Resources
- Django Channels and Daphne: WebSockets with Django
- Advantages of Using GraphQL with Django
- Alternatives to React, Vue, and Angular for Django SPA Integration