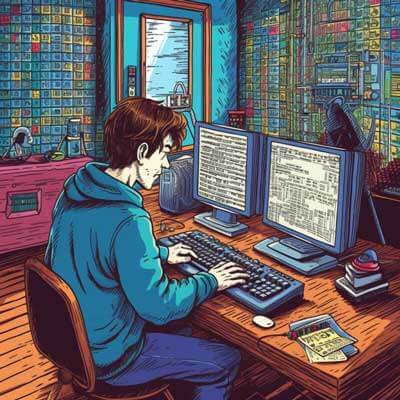
Table of Contents
Introduction
The Flask framework is a popular choice for building web applications in Python. While Flask provides a solid foundation for development, it's important to consider the security implications of your Flask apps. In this article, we will explore various security vulnerabilities that Flask apps may be susceptible to, and discuss best practices for fortifying your Flask apps against these vulnerabilities.
Related Article: Calculating Averages with Numpy in Python
SQL Injection Vulnerabilities in Flask Apps
Understanding SQL Injection Vulnerabilities
SQL Injection is a common web application vulnerability that occurs when an attacker is able to manipulate an application's database queries. This can lead to unauthorized access, data breaches, and even complete compromise of the application and underlying systems.
To demonstrate the risk of SQL Injection vulnerabilities in Flask apps, let's consider a simple example of a user login functionality:
from flask import Flask, request import sqlite3 app = Flask(__name__) conn = sqlite3.connect('database.db') cursor = conn.cursor() @app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] query = "SELECT * FROM users WHERE username = '{}' AND password = '{}'".format(username, password) cursor.execute(query) result = cursor.fetchone() if result: return 'Login successful' else: return 'Invalid credentials' if __name__ == '__main__': app.run()
In this example, the user's input is directly concatenated into the SQL query string. This leaves the application vulnerable to SQL Injection attacks. An attacker can craft malicious input that alters the query and bypasses authentication, gaining unauthorized access to the application.
Preventing SQL Injection in Flask Apps
To prevent SQL Injection vulnerabilities in Flask apps, it is important to use parameterized queries or prepared statements. This ensures that user input is properly sanitized and treated as data, rather than executable SQL code.
Here's an updated version of the previous example that uses parameterized queries:
from flask import Flask, request import sqlite3 app = Flask(__name__) conn = sqlite3.connect('database.db') cursor = conn.cursor() @app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] query = "SELECT * FROM users WHERE username = ? AND password = ?" cursor.execute(query, (username, password)) result = cursor.fetchone() if result: return 'Login successful' else: return 'Invalid credentials' if __name__ == '__main__': app.run()
Related Article: Python Command Line Arguments: How to Use Them
Cross-site Scripting (XSS) Vulnerabilities in Flask Apps
Understanding XSS Vulnerabilities
Cross-site Scripting (XSS) is a web application vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. These scripts can be used to steal sensitive information, manipulate user sessions, or deface the website.
Flask apps can be vulnerable to XSS attacks if they fail to properly sanitize and validate user input before displaying it in web pages.
Consider the following Flask app that displays user-generated content without proper input validation:
from flask import Flask, request, render_template_string app = Flask(__name__) @app.route('/post', methods=['POST']) def post(): content = request.form['content'] return render_template_string('<p>{{ content }}</p>', content=content) if __name__ == '__main__': app.run()
In this example, the user-generated content is directly inserted into the HTML template using the {{ content }}
syntax. If an attacker submits malicious JavaScript code as the content, it will be executed by the browser when the page is loaded, leading to an XSS vulnerability.
Preventing XSS Vulnerabilities in Flask Apps
To prevent XSS vulnerabilities in Flask apps, it is important to properly sanitize and validate user input before displaying it in web pages. Flask provides a built-in mechanism for escaping HTML characters using the escape()
function from the flask
module.
Here's an updated version of the previous example that escapes HTML characters in the user-generated content:
from flask import Flask, request, render_template_string, escape app = Flask(__name__) @app.route('/post', methods=['POST']) def post(): content = request.form['content'] return render_template_string('<p>{{ content }}</p>', content=escape(content)) if __name__ == '__main__': app.run()
Cross-site Request Forgery (CSRF) Protection in Flask
Related Article: How to Manage Memory with Python
Understanding CSRF Vulnerabilities
Cross-site Request Forgery (CSRF) is a web application vulnerability that allows an attacker to perform unauthorized actions on behalf of a victim user. This occurs when an attacker tricks a victim into unknowingly submitting a malicious request, exploiting the victim's authenticated session.
Flask apps can be vulnerable to CSRF attacks if they do not implement proper CSRF protection mechanisms.
Consider the following Flask app that allows users to update their profile information:
from flask import Flask, request, render_template, session, redirect, url_for app = Flask(__name__) app.secret_key = 'your_secret_key' @app.route('/profile', methods=['GET', 'POST']) def profile(): if request.method == 'POST': session['username'] = request.form['username'] return redirect(url_for('profile')) else: username = session.get('username') return render_template('profile.html', username=username) if __name__ == '__main__': app.run()
In this example, the user's username is stored in the session and displayed in the profile page. However, there is no mechanism in place to verify the origin of the request. An attacker can create a malicious web page that automatically submits a POST request to the /profile
endpoint, changing the victim's username without their knowledge or consent.
Implementing CSRF Protection in Flask
To protect Flask apps against CSRF attacks, it is important to implement CSRF tokens. A CSRF token is a unique token that is generated for each user session and included in every form submission. The server verifies the submitted token to ensure that the request was intentionally made by the user.
Flask provides a built-in mechanism for generating and validating CSRF tokens using the csrf
module from the flask_wtf
package.
Here's an updated version of the previous example that implements CSRF protection:
from flask import Flask, request, render_template, session, redirect, url_for from flask_wtf import FlaskForm from wtforms import StringField, SubmitField from wtforms.csrf.session import SessionCSRF from wtforms.validators import DataRequired app = Flask(__name__) app.secret_key = 'your_secret_key' app.config['WTF_CSRF_SECRET_KEY'] = 'your_csrf_secret_key' class ProfileForm(FlaskForm): username = StringField('Username', validators=[DataRequired()]) submit = SubmitField('Save') @app.route('/profile', methods=['GET', 'POST']) def profile(): form = ProfileForm() if form.validate_on_submit(): session['username'] = form.username.data return redirect(url_for('profile')) form.username.data = session.get('username') return render_template('profile.html', form=form) if __name__ == '__main__': app.run()
In this updated example, we define a ProfileForm
class that extends FlaskForm
from the flask_wtf
package. The ProfileForm
includes a CSRF token field, which generates and validates CSRF tokens for each form submission. By using the validate_on_submit()
method, we ensure that the form is only processed if the CSRF token is valid and the form data passes any additional validation rules.
Clickjacking Vulnerabilities and Countermeasures in Flask Apps
Understanding Clickjacking Vulnerabilities
Clickjacking is a web application vulnerability that allows an attacker to trick users into clicking on invisible or disguised elements on a web page. This can lead to unintended actions performed by the user, such as unknowingly submitting form data or clicking on malicious links.
Flask apps can be vulnerable to clickjacking attacks if they do not implement proper clickjacking protection mechanisms.
Consider the following Flask app that displays sensitive user information:
from flask import Flask, render_template app = Flask(__name__) @app.route('/profile') def profile(): return render_template('profile.html') if __name__ == '__main__': app.run()
In this example, the profile.html
template is rendered without any clickjacking protection mechanisms. An attacker can create a malicious web page that embeds the Flask app within an iframe, tricking the user into unknowingly performing actions on the app.
Related Article: How to Work with CSV Files in Python: An Advanced Guide
Countermeasures for Clickjacking Vulnerabilities in Flask Apps
To protect Flask apps against clickjacking attacks, it is important to implement the X-Frame-Options HTTP header. The X-Frame-Options header allows you to control whether or not your Flask app can be embedded within an iframe on another website.
Flask provides a built-in mechanism for setting the X-Frame-Options header using the after_request
decorator.
Here's an updated version of the previous example that sets the X-Frame-Options header:
from flask import Flask, render_template app = Flask(__name__) @app.route('/profile') def profile(): return render_template('profile.html') @app.after_request def set_x_frame_options(response): response.headers['X-Frame-Options'] = 'SAMEORIGIN' return response if __name__ == '__main__': app.run()
In this updated example, we use the after_request
decorator to set the X-Frame-Options header to SAMEORIGIN
. This ensures that the Flask app can only be embedded within an iframe on the same origin (i.e., the same website). This effectively prevents clickjacking attacks by disallowing the app from being embedded in iframes on malicious websites.
Session Hijacking Prevention in Flask Apps
Understanding Session Hijacking
Session hijacking is a web application vulnerability that allows an attacker to steal or manipulate a user's session identifier, gaining unauthorized access to the user's account. This can lead to account compromise, unauthorized actions, and exposure of sensitive information.
Flask apps can be vulnerable to session hijacking if they do not properly secure session identifiers and implement mechanisms to prevent session hijacking.
Consider the following Flask app that uses the default session management provided by Flask:
from flask import Flask, session, request app = Flask(__name__) app.secret_key = 'your_secret_key' @app.route('/') def index(): session['username'] = 'admin' return 'Hello, {}'.format(session['username']) @app.route('/profile') def profile(): return 'Welcome to your profile, {}'.format(session['username']) if __name__ == '__main__': app.run()
In this example, the default Flask session management is used, which stores session identifiers in client-side cookies. However, these session identifiers are not properly secured, making them vulnerable to session hijacking attacks.
Securing Session Identifiers in Flask Apps
To secure session identifiers in Flask apps and prevent session hijacking, it is important to use secure session management mechanisms, such as using secure cookies and rotating session identifiers.
Flask provides a built-in mechanism for configuring secure session management using the SESSION_COOKIE_SECURE
and SESSION_COOKIE_HTTPONLY
configuration options.
Here's an updated version of the previous example that configures secure session management:
from flask import Flask, session, request app = Flask(__name__) app.secret_key = 'your_secret_key' app.config['SESSION_COOKIE_SECURE'] = True app.config['SESSION_COOKIE_HTTPONLY'] = True @app.route('/') def index(): session['username'] = 'admin' return 'Hello, {}'.format(session['username']) @app.route('/profile') def profile(): return 'Welcome to your profile, {}'.format(session['username']) if __name__ == '__main__': app.run()
In this updated example, we set the SESSION_COOKIE_SECURE
option to True
, which ensures that session cookies are only transmitted over HTTPS. We also set the SESSION_COOKIE_HTTPONLY
option to True
, which prevents client-side JavaScript from accessing the session cookie, reducing the risk of session hijacking through XSS attacks.
Related Article: How to Check If Something Is Not In A Python List
Brute Force Attack Prevention in Flask Apps
Understanding Brute Force Attacks
Brute force attacks are a type of cyber attack where an attacker attempts to gain unauthorized access to an application or system by systematically trying all possible combinations of usernames and passwords. Brute force attacks can be a serious threat to Flask apps if they do not implement proper mechanisms to prevent or mitigate these attacks.
Consider the following Flask app that implements a login functionality:
from flask import Flask, request, session app = Flask(__name__) app.secret_key = 'your_secret_key' @app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] if username == 'admin' and password == 'password': session['username'] = 'admin' return 'Login successful' else: return 'Invalid credentials' if __name__ == '__main__': app.run()
In this example, the login functionality is susceptible to brute force attacks as there are no mechanisms in place to prevent multiple login attempts or rate limiting.
Preventing Brute Force Attacks in Flask Apps
To prevent brute force attacks in Flask apps, it is important to implement mechanisms such as rate limiting, account lockouts, and strong password policies.
There are several libraries and techniques available to enhance the security of Flask apps against brute force attacks.
One popular library is Flask-Limiter, which provides rate limiting capabilities for Flask apps. Here's an example of using Flask-Limiter to implement rate limiting:
from flask import Flask, request, session from flask_limiter import Limiter from flask_limiter.util import get_remote_address app = Flask(__name__) app.secret_key = 'your_secret_key' limiter = Limiter(app, key_func=get_remote_address) @app.route('/login', methods=['POST']) @limiter.limit("5/minute") def login(): username = request.form['username'] password = request.form['password'] if username == 'admin' and password == 'password': session['username'] = 'admin' return 'Login successful' else: return 'Invalid credentials' if __name__ == '__main__': app.run()
In this updated example, we use Flask-Limiter to rate limit the /login
endpoint to a maximum of 5 requests per minute. This helps prevent brute force attacks by limiting the number of login attempts an attacker can make within a given time period.
Additionally, it is important to enforce strong password policies, such as requiring a minimum password length, complexity requirements, and implementing password hashing and salting.
Man-in-the-Middle (MITM) Attack Mitigation in Flask
Related Article: How To Filter Dataframe Rows Based On Column Values
Understanding Man-in-the-Middle (MITM) Attacks
Man-in-the-Middle (MITM) attacks are a type of cyber attack where an attacker intercepts and potentially alters communications between two parties without their knowledge or consent. MITM attacks can be a serious threat to Flask apps if they do not implement proper encryption and authentication mechanisms.
Consider the following Flask app that sends sensitive data over an unencrypted HTTP connection:
from flask import Flask, request app = Flask(__name__) @app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] # Perform login logic return 'Login successful' if __name__ == '__main__': app.run()
In this example, the login functionality is susceptible to MITM attacks as the sensitive data (i.e., the username and password) is transmitted in clear text over an unencrypted connection. An attacker who can intercept the communication can easily capture and potentially manipulate the data.
Mitigating Man-in-the-Middle (MITM) Attacks in Flask
To mitigate MITM attacks in Flask apps, it is important to implement proper encryption and authentication mechanisms, such as using HTTPS and implementing certificate validation.
To enable HTTPS in Flask, you will need to obtain an SSL certificate and configure your web server (e.g., Nginx, Apache) to handle HTTPS requests. Additionally, you can use libraries such as Flask-SSLify to automatically redirect HTTP requests to HTTPS.
Here's an example of using Flask-SSLify to enforce HTTPS:
from flask import Flask, request from flask_sslify import SSLify app = Flask(__name__) sslify = SSLify(app) @app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] # Perform login logic return 'Login successful' if __name__ == '__main__': app.run()
In this updated example, we use Flask-SSLify to automatically redirect HTTP requests to HTTPS. This ensures that all communication between the client and server is encrypted, mitigating the risk of MITM attacks.
It is also important to validate the server's SSL certificate to prevent attacks such as SSL/TLS certificate spoofing. Flask provides the verify_x509_certificate()
function in the ssl
module for this purpose.
Denial of Service (DoS) Attack Mitigation in Flask
Understanding Denial of Service (DoS) Attacks
Denial of Service (DoS) attacks are a type of cyber attack where an attacker overwhelms a target system with a large volume of traffic or resource-intensive requests, rendering the system unavailable to legitimate users. DoS attacks can be a serious threat to Flask apps if they do not implement proper mitigation mechanisms.
Consider the following Flask app that performs a computationally expensive task:
from flask import Flask, request app = Flask(__name__) @app.route('/process', methods=['POST']) def process(): data = request.form['data'] # Perform computationally expensive task return 'Processing complete' if __name__ == '__main__': app.run()
In this example, the /process
endpoint performs a computationally expensive task. An attacker can exploit this by sending a large number of requests with resource-intensive input, potentially overwhelming the server and causing a denial of service for legitimate users.
Related Article: How To Use Matplotlib Inline In Python
Mitigating Denial of Service (DoS) Attacks in Flask
To mitigate Denial of Service (DoS) attacks in Flask apps, it is important to implement mechanisms such as rate limiting, request validation, and resource allocation.
Flask-Limiter, as mentioned earlier, can be used to implement rate limiting to prevent excessive requests from overwhelming the server.
Additionally, it is important to validate and sanitize user input to prevent resource-intensive requests. This can be achieved using libraries such as WTForms for form validation and input sanitization.
Here's an example of using WTForms to validate and sanitize user input:
from flask import Flask, request from wtforms import Form, StringField, validators app = Flask(__name__) class ProcessForm(Form): data = StringField('Data', validators=[validators.DataRequired()]) @app.route('/process', methods=['POST']) def process(): form = ProcessForm(request.form) if form.validate(): data = form.data.data # Perform computationally expensive task return 'Processing complete' else: return 'Invalid input' if __name__ == '__main__': app.run()
In this updated example, we use WTForms to define a ProcessForm
class that includes a StringField
for the data
input. The validators.DataRequired()
validator ensures that the input is not empty. By validating and sanitizing user input, we can prevent resource-intensive requests and mitigate the risk of DoS attacks.
JSON Web Token (JWT) Implementation in Flask API
Understanding JSON Web Tokens (JWT)
JSON Web Tokens (JWT) are a popular method for securely transmitting information between parties as a JSON object. JWTs are commonly used for authentication and authorization in web APIs.
Flask apps can implement JWT-based authentication and authorization mechanisms using libraries such as Flask-JWT-Extended.
Consider the following Flask app that implements JWT-based authentication:
from flask import Flask, request, jsonify from flask_jwt_extended import ( JWTManager, jwt_required, create_access_token, get_jwt_identity ) app = Flask(__name__) app.config['JWT_SECRET_KEY'] = 'your_secret_key' jwt = JWTManager(app) @app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] # Perform authentication logic access_token = create_access_token(identity=username) return jsonify(access_token=access_token) @app.route('/protected', methods=['GET']) @jwt_required() def protected(): current_user = get_jwt_identity() return 'Welcome, {}'.format(current_user) if __name__ == '__main__': app.run()
In this example, the /login
endpoint performs authentication and returns a JWT access token upon successful authentication. The /protected
endpoint requires a valid access token and returns a protected resource if the token is valid.
Implementing JWT in Flask API
To implement JWT-based authentication and authorization in Flask apps, you can use the Flask-JWT-Extended library. Flask-JWT-Extended provides a comprehensive set of features for working with JWTs in Flask apps.
To get started, you will need to install Flask-JWT-Extended:
pip install flask-jwt-extended
Here's an example of implementing JWT in a Flask API using Flask-JWT-Extended:
from flask import Flask, request, jsonify from flask_jwt_extended import ( JWTManager, jwt_required, create_access_token, get_jwt_identity ) app = Flask(__name__) app.config['JWT_SECRET_KEY'] = 'your_secret_key' jwt = JWTManager(app) @app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] # Perform authentication logic access_token = create_access_token(identity=username) return jsonify(access_token=access_token) @app.route('/protected', methods=['GET']) @jwt_required() def protected(): current_user = get_jwt_identity() return 'Welcome, {}'.format(current_user) if __name__ == '__main__': app.run()
In this example, we initialize the Flask app and configure the JWT secret key. The /login
endpoint performs authentication and returns a JWT access token. The /protected
endpoint is protected with the @jwt_required()
decorator, which ensures that a valid access token is required to access the endpoint. The get_jwt_identity()
function retrieves the current user's identity from the access token.
Related Article: How to Use Regex to Match Any Character in Python
Two-Factor Authentication (2FA) in Flask Apps
Understanding Two-Factor Authentication (2FA)
Two-Factor Authentication (2FA) is an additional layer of security that requires users to provide two forms of identification before gaining access to an application or system. This typically involves something the user knows (e.g., a password) and something the user possesses (e.g., a physical token or a mobile device).
Flask apps can implement 2FA using libraries such as Flask-Login and Flask-OTP.
Consider the following Flask app that implements 2FA using Flask-Login and Flask-OTP:
from flask import Flask, request, render_template, redirect, url_for from flask_login import ( LoginManager, UserMixin, login_user, current_user, login_required, logout_user ) from flask_otp import OTP from flask_otp.login import OTPLoginManager, OTPRequired app = Flask(__name__) app.secret_key = 'your_secret_key' login_manager = LoginManager(app) otp = OTP(app) otp_login_manager = OTPLoginManager(app) class User(UserMixin): def __init__(self, id): self.id = id def get_otp_secret(self): # Retrieve OTP secret for the user from the database return 'your_otp_secret' def verify_otp(self, otp_value): # Verify the OTP value for the user return otp.verify_totp(self.get_otp_secret(), otp_value) @login_manager.user_loader def load_user(user_id): return User(user_id) @app.route('/login', methods=['GET', 'POST']) def login(): if current_user.is_authenticated: return redirect(url_for('profile')) if request.method == 'POST': username = request.form['username'] password = request.form['password'] otp_value = request.form['otp'] user = User(username) if user.verify_otp(otp_value) and password == 'password': login_user(user) return redirect(url_for('profile')) else: return 'Invalid credentials' return render_template('login.html') @app.route('/profile') @login_required @OTPRequired def profile(): return 'Welcome, {}'.format(current_user.id) @app.route('/logout') @login_required def logout(): logout_user() return redirect(url_for('login')) if __name__ == '__main__': app.run()
In this example, we define a User
class that extends UserMixin
from Flask-Login. The User
class implements the necessary methods for handling OTP authentication. The /login
endpoint checks the username, password, and OTP value before authenticating the user using login_user()
. The /profile
endpoint requires the user to be authenticated and have a valid OTP session.
Implementing Two-Factor Authentication (2FA) in Flask Apps
To implement Two-Factor Authentication (2FA) in Flask apps, you can use libraries such as Flask-Login and Flask-OTP.
To get started, you will need to install Flask-Login and Flask-OTP:
pip install flask-login flask-otp
Here's an example of implementing 2FA in a Flask app using Flask-Login and Flask-OTP:
from flask import Flask, request, render_template, redirect, url_for from flask_login import ( LoginManager, UserMixin, login_user, current_user, login_required, logout_user ) from flask_otp import OTP from flask_otp.login import OTPLoginManager, OTPRequired app = Flask(__name__) app.secret_key = 'your_secret_key' login_manager = LoginManager(app) otp = OTP(app) otp_login_manager = OTPLoginManager(app) class User(UserMixin): def __init__(self, id): self.id = id def get_otp_secret(self): # Retrieve OTP secret for the user from the database return 'your_otp_secret' def verify_otp(self, otp_value): # Verify the OTP value for the user return otp.verify_totp(self.get_otp_secret(), otp_value) @login_manager.user_loader def load_user(user_id): return User(user_id) @app.route('/login', methods=['GET', 'POST']) def login(): if current_user.is_authenticated: return redirect(url_for('profile')) if request.method == 'POST': username = request.form['username'] password = request.form['password'] otp_value = request.form['otp'] user = User(username) if user.verify_otp(otp_value) and password == 'password': login_user(user) return redirect(url_for('profile')) else: return 'Invalid credentials' return render_template('login.html') @app.route('/profile') @login_required @OTPRequired def profile(): return 'Welcome, {}'.format(current_user.id) @app.route('/logout') @login_required def logout(): logout_user() return redirect(url_for('login')) if __name__ == '__main__': app.run()
In this example, we initialize the Flask app and configure the secret key. We define a User
class that extends UserMixin
from Flask-Login and implements the necessary methods for handling OTP authentication. We use the @login_manager.user_loader
decorator to load the user from the database. The /login
endpoint checks the username, password, and OTP value before authenticating the user using login_user()
. The /profile
endpoint requires the user to be authenticated and have a valid OTP session.
Additional Resources
- Flask Web Security Best Practices