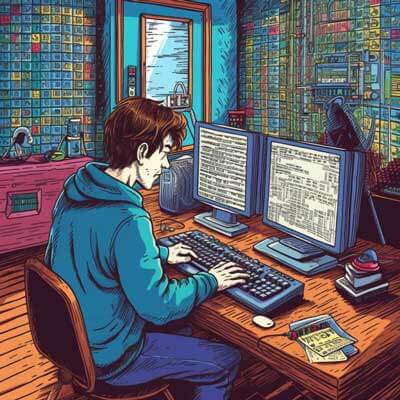
Checking if a variable exists in Python is a common task in programming. There are several ways to accomplish this, depending on the specific scenario and requirements. In this answer, we will explore two common approaches to checking the existence of a variable in Python.
Using the ‘in’ Operator
One straightforward way to check if a variable exists in Python is by using the ‘in’ operator. The ‘in’ operator is typically used to check if a value exists in a sequence, such as a list or a string. However, it can also be used to check if a variable exists in the current scope.
Here’s an example that demonstrates the usage of the ‘in’ operator to check if a variable exists:
if 'my_variable' in locals(): print("The variable 'my_variable' exists.") else: print("The variable 'my_variable' does not exist.")
In this code snippet, we use the ‘locals()’ function, which returns a dictionary containing all local variables in the current scope. We check if the variable ‘my_variable’ exists in this dictionary using the ‘in’ operator.
Related Article: String Comparison in Python: Best Practices and Techniques
Using the ‘try-except’ Block
Another approach to checking if a variable exists in Python is by using a ‘try-except’ block. This method involves trying to access the variable and catching the ‘NameError’ exception if it does not exist.
Here’s an example that demonstrates the usage of the ‘try-except’ block to check if a variable exists:
try: my_variable print("The variable 'my_variable' exists.") except NameError: print("The variable 'my_variable' does not exist.")
In this code snippet, we attempt to access the variable ‘my_variable’ directly. If the variable does not exist, a ‘NameError’ exception will be raised, and we catch it using the ‘except’ block to handle the case where the variable does not exist.
Best Practices
When checking if a variable exists in Python, it is important to consider the scope of the variable. The ‘in’ operator and the ‘try-except’ block both operate within the current scope. If you need to check for the existence of a variable in an outer or global scope, you can use the ‘globals()’ function instead of ‘locals()’ in the ‘in’ operator approach.
It is generally recommended to use the ‘try-except’ block approach when you actually need to use the variable after checking its existence. This approach avoids unnecessary redundant checks and ensures that the variable is accessible within the ‘try’ block.
Related Article: How To Limit Floats To Two Decimal Points In Python