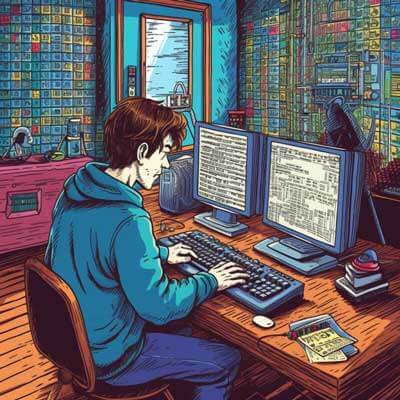
Table of Contents
Introduction to Flask
Flask is a lightweight and flexible web framework written in Python. It allows developers to build web applications quickly and easily. Flask follows the Model-View-Controller (MVC) architectural pattern and provides a simple and intuitive way to handle HTTP requests, route URLs, render templates, and interact with databases.
To get started with Flask, you first need to install it. Open your terminal and run the following command:
pip install flask
Once Flask is installed, you can create a new Flask application by creating a Python file with the .py
extension. In this file, you can define routes, views, and other application logic.
Here's an example of a basic Flask application:
from flask import Flask app = Flask(__name__) @app.route('/') def hello(): return 'Hello, World!' if __name__ == '__main__': app.run()
In this example, we import the Flask
class from the flask
module. We create an instance of the Flask
class and define a route for the root URL ("/"). When a user visits this URL in their browser, the hello()
function is called, which returns the string "Hello, World!". Finally, we run the application using the run()
method.
Flask also supports URL parameters and dynamic routing. You can define routes with variables enclosed in brackets. These variables can then be accessed in your view functions.
@app.route('/users/') def get_user(username): return f'Hello, {username}!'
In this example, if a user visits "/users/john", the get_user()
function will be called with 'john'
as the value of the username
parameter.
Flask provides many other features such as template rendering using Jinja2, form handling, file uploads, and more. It also supports various extensions and libraries that can be easily integrated into your Flask application.
Related Article: How To Convert a Dictionary To JSON In Python
Setting up the Development Environment
Before you start building a web app with Flask, you need to set up your development environment. Here are the steps to get started:
1. Install Python: Flask is written in Python, so you'll need to have Python installed on your machine. You can download the latest version of Python from the official website (https://www.python.org/) and follow the installation instructions for your operating system.
2. Create a Virtual Environment: It's a best practice to create a virtual environment for each project to isolate its dependencies from other projects. Open your terminal or command prompt and run the following commands:
python -m venv myenv
This will create a new directory called "myenv" which contains the necessary files for the virtual environment.
3. Activate the Virtual Environment: Once you've created the virtual environment, you need to activate it before installing any packages or running your Flask application. Run the following command:
For Windows:
myenv\Scripts\activate
For Unix/Linux:
source myenv/bin/activate
4. Install Flask: With your virtual environment activated, you can now install Flask using pip, which is a package manager for Python. Run the following command:
pip install flask
5. Install Other Dependencies: Depending on your specific web app requirements, you may need additional packages or libraries such as MongoDB driver, Reactjs, Bootstrap, etc. Use pip to install these dependencies as needed.
Now that your development environment is set up and ready to go, you can proceed with creating your Flask application.
Creating the Backend with Flask
Flask provides a simple and elegant way to create the backend of your web application. You can define routes, handle HTTP requests, and interact with databases using Flask's built-in functionalities.
Here's an example of creating a basic backend API using Flask:
from flask import Flask, jsonify, request app = Flask(__name__) @app.route('/api/users', methods=['GET']) def get_users(): # Retrieve users from the database users = [ {'id': 1, 'name': 'John Doe'}, {'id': 2, 'name': 'Jane Smith'} ] return jsonify(users) @app.route('/api/users', methods=['POST']) def create_user(): # Create a new user based on request data data = request.get_json() user = {'id': data['id'], 'name': data['name']} # Save the new user to the database # ... return jsonify(user), 201 if __name__ == '__main__': app.run()
In this example, we define two routes for retrieving users (/api/users
) and creating a new user (/api/users
). The get_users()
function returns a list of users in JSON format. The create_user()
function receives JSON data from the request payload and creates a new user object.
Flask provides various decorators (@app.route()
) to map URL patterns to view functions. You can specify different HTTP methods (GET, POST, PUT, DELETE) to handle different types of requests.
You can also use Flask's request object to access data sent in the request payload or query parameters. In the create_user()
function above, we use request.get_json()
to retrieve JSON data from the POST request.
Flask supports other features such as error handling, file uploads, and cookie handling. You can explore the Flask documentation for more details on these features.
Integrating MongoDB with Flask
MongoDB is a popular NoSQL database that can be easily integrated with Flask to store and retrieve data.
To use MongoDB with Flask, you'll need to install the pymongo
package, which is the official MongoDB driver for Python. Run the following command to install it:
pip install pymongo
Here's an example of integrating MongoDB with Flask:
from flask import Flask, jsonify, request from pymongo import MongoClient app = Flask(__name__) client = MongoClient('mongodb://localhost:27017/') db = client['mydatabase'] users_collection = db['users'] @app.route('/api/users', methods=['GET']) def get_users(): users = users_collection.find() user_list = [] for user in users: user_list.append({'id': user['id'], 'name': user['name']}) return jsonify(user_list) @app.route('/api/users', methods=['POST']) def create_user(): data = request.get_json() new_user = {'id': data['id'], 'name': data['name']} users_collection.insert_one(new_user) return jsonify(new_user), 201 if __name__ == '__main__': app.run()
In this example, we first import the MongoClient
class from the pymongo
module. We create a connection to the local MongoDB server using MongoClient('mongodb://localhost:27017/')
. We then specify the database name (mydatabase
) and collection name (users
).
In our view functions, we use users_collection.find()
to retrieve all documents from the users
collection. We iterate over each document and construct a list of users in JSON format.
To insert a new user, we use users_collection.insert_one(new_user)
, where new_user
is a dictionary containing the user data.
You can perform various other operations with MongoDB such as updating documents, deleting documents, and querying based on specific criteria. The pymongo
package provides a comprehensive API for interacting with MongoDB.
Make sure you have MongoDB installed and running on your machine before running the Flask application. You can download and install MongoDB from the official website (https://www.mongodb.com/).
Related Article: How to Append One String to Another in Python
Building the Frontend with Reactjs and Bootstrap
Reactjs and Bootstrap are popular frontend technologies that can be used together to build responsive and interactive user interfaces. Reactjs is a JavaScript library for building UI components, while Bootstrap provides CSS styles and components for creating visually appealing web pages.
To use Reactjs in your Flask application, you'll need to set up a separate frontend project using tools like Create React App (https://create-react-app.dev/). Once you've created your React project, you can integrate it with Flask by serving the built static files from Flask's routes.
Here's an example of integrating Reactjs and Bootstrap into a Flask application:
1. Create a new directory called frontend
in your Flask project's root directory.
2. Inside the frontend
directory, run the following command to create a new React app:
npx create-react-app chat-app
3. Once the app is created, navigate into the chat-app
directory:
cd chat-app
4. Install Bootstrap as a dependency:
npm install bootstrap
5. Open the file src/App.js
in your preferred code editor and replace its contents with the following code:
import React from 'react'; import './App.css'; import 'bootstrap/dist/css/bootstrap.css'; function App() { return ( <div> <h1>Chat Web App</h1> <div>Welcome to the chat!</div> </div> ); } export default App;
In this example, we import the necessary dependencies (React, CSS file for styling, and Bootstrap CSS). Inside the App
component, we render a simple heading and a Bootstrap alert component.
6. Run the following command to build the static files for your React app:
npm run build
7. Now, in your Flask application's main Python file, add a route to serve the built static files. Update your code as follows:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('index.html') if __name__ == '__main__': app.run()
In this example, we import the render_template
function from flask
module. The index()
function returns the rendered template index.html
, which will be served as the entry point for our React app.
8. Create a new directory called templates
in your Flask project's root directory.
9. Move the generated build
directory from your React app into the newly created templates
directory.
10. Rename the moved folder from build
to match your desired template name (e.g., chat-app
). Make sure it matches with what you specified in step 7.
11. Start your Flask application and navigate to http://localhost:5000/. You should see your React app running with Bootstrap styles applied.
Implementing User Authentication and Authorization
User authentication and authorization are essential components of a secure web application. Flask provides various libraries and extensions that make it easy to implement user management features.
One popular extension for user authentication in Flask is Flask-Login. Flask-Login provides a simple way to handle user sessions, login/logout functionality, and access control.
Here's an example of implementing user authentication and authorization using Flask-Login:
1. Install the flask-login
package:
pip install flask-login
2. In your Flask application's main Python file, import the necessary modules:
from flask import Flask, render_template, redirect, url_for from flask_login import LoginManager, UserMixin, login_user, logout_user, login_required
3. Create an instance of the LoginManager
class:
app = Flask(__name__) app.config['SECRET_KEY'] = 'your-secret-key' login_manager = LoginManager(app)
4. Define a User model that implements the UserMixin
class from flask_login
. This model represents your application's users:
class User(UserMixin): def __init__(self, id): self.id = id @staticmethod def get(user_id): # Retrieve user from the database based on ID # ... return User(user_id)
5. Implement the load_user
callback function required by Flask-Login to load users from their ID:
@login_manager.user_loader def load_user(user_id): return User.get(user_id)
6. Create routes for login and logout:
@app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': # Validate username/password from form data username = request.form['username'] password = request.form['password'] # Check if username/password is valid # ... user = User(username) # Create a User instance with the ID login_user(user) # Log in the user next_page = request.args.get('next') return redirect(next_page or url_for('index')) return render_template('login.html') @app.route('/logout') @login_required def logout(): logout_user() # Log out the user return redirect(url_for('index'))
In this example, we define a login()
route that handles both GET and POST requests. When a user submits the login form, we validate their credentials and create a User
instance with their ID. We then log in the user using login_user()
.
The logout()
route logs out the authenticated user by calling logout_user()
.
7. Protect routes that require authentication using the @login_required
decorator:
@app.route('/profile') @login_required def profile(): return render_template('profile.html')
In this example, the /profile
route can only be accessed by authenticated users. If an unauthenticated user tries to access it, they will be redirected to the login page.
Flask-Login provides many other features such as remember me functionality, session management, and more. You can refer to Flask-Login's documentation for further details on customization and advanced usage.
Designing the Chat Interface
Designing an intuitive and visually appealing chat interface is crucial for providing a great user experience. With HTML, CSS, and JavaScript libraries like Reactjs, you can create interactive chat interfaces with real-time updates.
Here's an example of designing a basic chat interface using HTML, CSS, and Reactjs:
1. Create a new file called ChatInterface.js
in your React app's source directory (src/components
). Open the file and add the following code:
import React, { useState } from 'react'; const ChatInterface = () => { const [messages, setMessages] = useState([]); const [newMessage, setNewMessage] = useState(''); const handleSendMessage = () => { if (newMessage.trim() !== '') { setMessages([...messages, newMessage]); setNewMessage(''); } }; return ( <div> <div> {messages.map((message, index) => ( <div>{message}</div> ))} </div> <div> setNewMessage(e.target.value)} placeholder="Type your message..." /> <button>Send</button> </div> </div> ); }; export default ChatInterface;
In this example, we define a functional component called ChatInterface
that represents the chat interface. It utilizes React's useState
hook to manage the state of messages and the input field.
The handleSendMessage
function is called when the user clicks the "Send" button. It adds a new message to the messages
state array and clears the input field.
2. In your main app component (App.js
), import and use the ChatInterface
component:
import React from 'react'; import './App.css'; import 'bootstrap/dist/css/bootstrap.css'; import ChatInterface from './components/ChatInterface'; function App() { return ( <div> <h1>Chat Web App</h1> </div> ); } export default App;
In this example, we import the ChatInterface
component and render it below the heading.
3. Style the chat interface using CSS. Create a new file called ChatInterface.css
in your component's directory (src/components
) and add the following styles:
.chat-interface { display: flex; flex-direction: column; height: 400px; } .message-container { flex-grow: 1; overflow-y: auto; } .message { margin-bottom: 10px; } .input-container { display: flex; } .input-container input { flex-grow: 1; } .input-container button { margin-left: 10px; }
These styles define a basic layout for the chat interface with a scrollable message container and an input field/button for sending messages.
Real-time Communication with Websockets
Real-time communication is essential for building interactive chat applications where messages are instantly delivered to all connected users. Websockets provide a bidirectional communication channel between clients (browsers) and servers.
To implement real-time communication in Flask, you can use libraries like Flask-SocketIO or Flask-Sockets. These libraries enable you to establish websocket connections and handle events on both the server-side and client-side.
Here's an example of implementing real-time communication with websockets using Flask-SocketIO:
1. Install flask-socketio
package:
pip install flask-socketio
2. In your Flask application's main Python file, import the necessary modules:
from flask import Flask, render_template from flask_socketio import SocketIO, emit
3. Create an instance of SocketIO
:
app = Flask(__name__) app.config['SECRET_KEY'] = 'your-secret-key' socketio = SocketIO(app)
4. Define a route for the main page:
@app.route('/') def index(): return render_template('index.html')
5. Define event handlers for websocket events:
@socketio.on('connect') def handle_connect(): emit('connected', {'data': 'Connected'}) @socketio.on('disconnect') def handle_disconnect(): print('Client disconnected') @socketio.on('message') def handle_message(message): emit('message', message, broadcast=True)
In this example, we define three event handlers: handle_connect()
, handle_disconnect()
, and handle_message()
. The connect
event is triggered when a client connects to the server via websocket. The disconnect
event is triggered when a client disconnects. The message
event is triggered when a client sends a message.
6. Create an HTML file called index.html
in your Flask application's templates directory (templates
). Add the following code:
<title>Chat Web App</title> <h1>Chat Web App</h1> <div id="messages-container"></div> <button type="submit">Send</button> var socket = io(); socket.on('connect', function() { console.log('Connected'); }); socket.on('disconnect', function() { console.log('Disconnected'); }); socket.on('message', function(data) { var messageDiv = document.createElement('div'); messageDiv.textContent = data; document.getElementById('messages-container').appendChild(messageDiv); }); document.getElementById('chat-form').addEventListener('submit', function(event) { event.preventDefault(); var input = document.getElementById('message-input'); var message = input.value.trim(); if (message !== '') { socket.emit('message', message); input.value = ''; } });
In this example, we create a simple chat interface with a container for displaying messages and a form for sending new messages. The JavaScript code establishes a websocket connection and handles events like connect
, disconnect
, and message
. When the form is submitted, it emits a message
event with the entered text.
7. Start your Flask application with SocketIO:
if __name__ == '__main__': socketio.run(app)
Now, when multiple clients connect to your Flask app's main page (/
), they can send and receive real-time messages using websockets. The server broadcasts received messages to all connected clients.
You can extend this example to implement more features such as user authentication, private messaging, typing indicators, etc., to create a fully functional real-time chat application.
Related Article: Python Data Types Tutorial
Deploying the Application using Docker-compose
Docker-compose is a tool that allows you to define and manage multi-container Docker applications. It simplifies the deployment process by specifying services, networks, volumes, and other configuration details in a single YAML file.
To deploy your Flask chat web application using docker-compose:
1. Install Docker: Visit the official Docker website (https://www.docker.com/) and follow the instructions to install Docker on your machine.
2. Create a file called docker-compose.yml
in your project's root directory. Add the following content:
version: '3' services: app: build: context: . dockerfile: Dockerfile ports: - 5000:5000 depends_on: - mongodb mongodb: image: mongo ports: - 27017:27017
In this example, we define two services: app
and mongodb
. The app
service is built using the specified Dockerfile and exposes port 5000 for accessing the Flask application. The mongodb
service uses the official MongoDB image and exposes port 27017 for MongoDB connections.
3. Create a file called Dockerfile
in your project's root directory. Add the following content:
# Base image FROM python:3.9-slim # Set working directory WORKDIR /app # Copy requirements file COPY requirements.txt . # Install dependencies RUN pip install --no-cache-dir -r requirements.txt # Copy project files to working directory COPY . . # Expose port for Flask app EXPOSE 5000 # Command to run the Flask app CMD ["python", "app.py"]
In this example, we use a base Python image, set the working directory to /app
, copy the requirements.txt
file, install dependencies, copy all project files, expose port 5000 for Flask, and specify the command to run the Flask application.
4. Build and start the containers using docker-compose:
docker-compose up --build -d
The -d
flag runs containers in detached mode (in the background).
5. Access your Flask chat web application by navigating to http://localhost:5000 in your browser.
With docker-compose, you can easily deploy your Flask chat web application along with its dependencies (such as MongoDB) in a self-contained environment. This simplifies deployment and ensures consistency across different environments.
Testing and Debugging the Application
Testing and debugging are crucial steps in the software development process to ensure the reliability and correctness of your application. Flask provides various tools and libraries that make testing and debugging easier.
To test and debug your Flask chat web application, you can follow these guidelines:
1. Writing Unit Tests: Unit tests are used to verify the behavior of individual components or functions in isolation. You can use libraries like unittest
or pytest
to write unit tests for your Flask application's backend code. Test cases typically cover scenarios such as handling different HTTP requests, validating input data, interacting with the database, etc.
Here's an example of a unit test using unittest
:
import unittest from flask import Flask from myapp import app class MyAppTestCase(unittest.TestCase): def setUp(self): self.app = app.test_client() def test_homepage(self): response = self.app.get('/') self.assertEqual(response.status_code, 200) self.assertIn(b'Hello', response.data) if __name__ == '__main__': unittest.main()
In this example, we import unittest
and create a test case class that inherits from unittest.TestCase
. The setUp()
method sets up the test client for making requests. The test_homepage()
method sends a GET request to the root URL (/
) and checks if the response status code is 200 (OK) and contains the string "Hello".
2. Integration Testing: Integration tests focus on verifying interactions between different components or modules of your system. They can be used to test the integration of Flask with other technologies such as databases, external APIs, or frontend frameworks. Integration tests ensure that the different parts of your application work together correctly.
Here's an example of an integration test using pytest
:
import pytest from flask import Flask from myapp import app @pytest.fixture def client(): app.config['TESTING'] = True with app.test_client() as client: yield client def test_homepage(client): response = client.get('/') assert response.status_code == 200 assert b'Hello' in response.data
In this example, we use pytest
and define a fixture called client
to set up the test client. The test_homepage()
function sends a GET request to the root URL (/
) and asserts that the response status code is 200 (OK) and contains the string "Hello".
3. Debugging: Flask provides a built-in debugger that can be enabled during development to assist in identifying and fixing errors. By enabling debug mode, you can get detailed error messages and traceback information in your browser whenever an exception occurs.
To enable debug mode, modify your Flask application's main Python file:
app = Flask(__name__) app.config['SECRET_KEY'] = 'your-secret-key' if __name__ == '__main__': app.run(debug=True)
With debug mode enabled, Flask will automatically reload the server when changes are made to your code.
4. Logging: Logging is a useful technique for capturing runtime information about your application's behavior. You can use Python's built-in logging module or third-party libraries like loguru
or structlog
to log important events or errors during the execution of your Flask application.
Here's an example of using Python's logging module:
import logging from flask import Flask app = Flask(__name__) app.config['SECRET_KEY'] = 'your-secret-key' @app.route('/') def index(): app.logger.info('Rendering index page') return 'Hello, World!' if __name__ == '__main__': logging.basicConfig(level=logging.INFO) app.run()
In this example, we import the logging
module and configure it to log messages at the INFO
level. We use app.logger.info()
to log an informational message when the root URL is accessed.
5. Continuous Integration: Continuous Integration (CI) is a practice that involves automatically building and testing your application whenever changes are pushed to a version control repository. CI tools like Jenkins, Travis CI, or CircleCI can be used to set up automated builds and tests for your Flask chat web application. These tools help ensure that your codebase remains stable and functional as new features are added or bugs are fixed.
Performance Considerations and Best Practices
Optimizing performance is crucial for delivering a fast and responsive chat web application. Here are some performance considerations and best practices to follow:
1. Caching: Implement caching mechanisms to reduce database or computation overhead for frequently accessed data or computations. Use tools like Redis or Memcached to cache query results, session data, or expensive calculations.
2. Database Optimization: Optimize database queries by using appropriate indexes, minimizing unnecessary queries, utilizing database-specific features like aggregation pipelines (in MongoDB), or denormalizing data for faster reads.
3. Asynchronous Tasks: Offload time-consuming tasks from the main request-response cycle by using task queues or background job systems like Celery or RQ (Redis Queue). This helps improve response times and scalability.
4. Minify and Bundle Assets: Minify and bundle CSS and JavaScript assets to reduce file sizes and minimize network latency. Tools like Webpack or Parcel can be used to automate this process.
5. CDN (Content Delivery Network): Utilize a CDN to serve static assets (CSS, JavaScript, images) from edge locations closer to your users. This reduces the latency of asset downloads and improves overall page load times.
6. Load Testing: Perform load testing using tools like Apache JMeter or Locust to simulate concurrent users and identify performance bottlenecks. Optimize your application based on the results of load testing.
7. Gzip Compression: Enable Gzip compression to reduce the size of HTTP responses sent by your Flask application. This can significantly improve network transfer times for textual content.
8. Image Optimization: Optimize images by compressing them without significant loss in quality. Use tools like ImageMagick or libraries like Pillow in Python to resize, compress, or convert image formats when necessary.
9. Proper Error Handling: Implement proper error handling mechanisms to gracefully handle exceptions and errors in your Flask application. Avoid exposing detailed error messages or stack traces in production environments.
10. Scalability: Design your application with scalability in mind from the beginning. Consider horizontal scaling by utilizing load balancers, clustering, container orchestration (e.g., Kubernetes), or cloud-based solutions like AWS ECS or Google Kubernetes Engine.
11. Security Considerations: Ensure that your Flask application follows security best practices such as input validation, secure session management, protection against common web vulnerabilities (e.g., cross-site scripting), and proper user authentication/authorization mechanisms.
12. Monitoring and Logging: Set up monitoring tools (e.g., Prometheus) and logging frameworks (e.g., ELK stack) to gather performance metrics, detect anomalies, track errors, and troubleshoot issues in production environments.