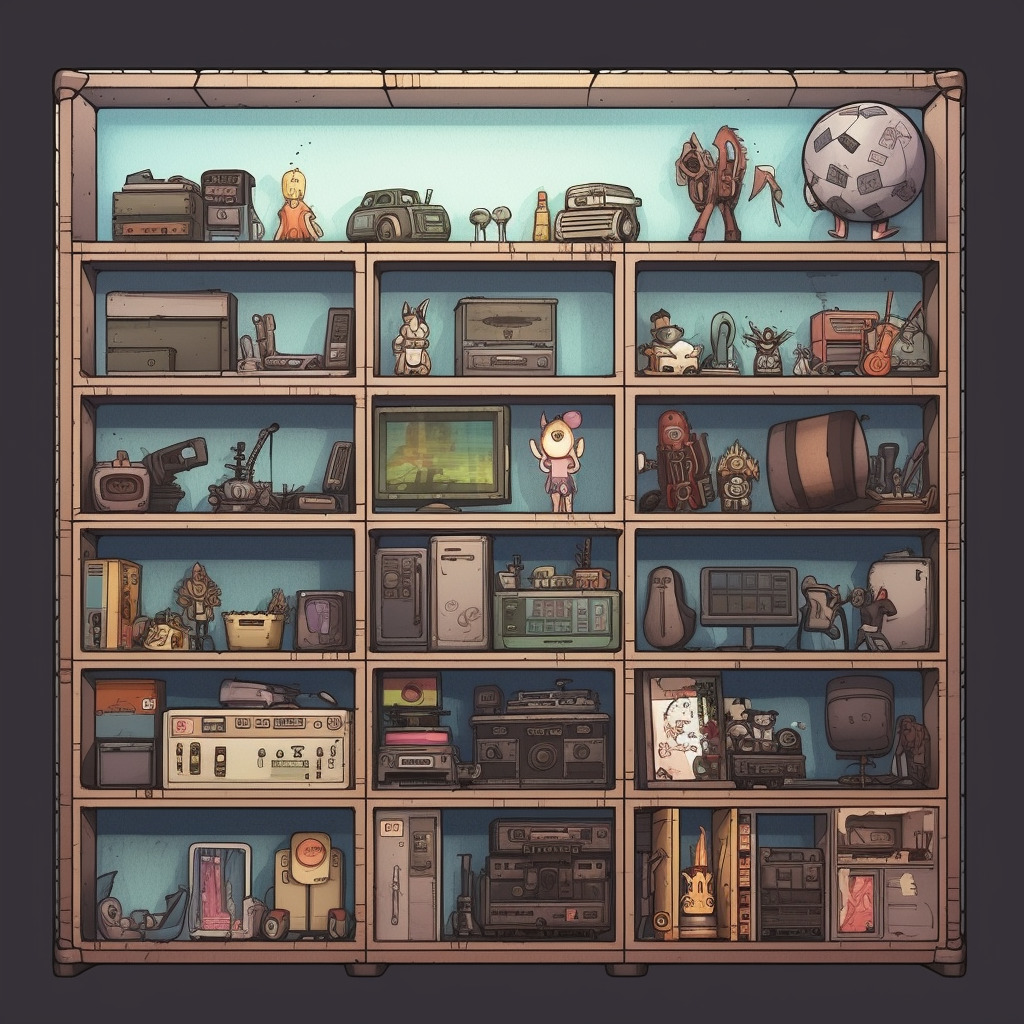
- Getting Started with Python Collections
- Lists
- Tuples
- Sets
- Dictionaries
- Lists
- Creating Lists
- Accessing List Elements
- Modifying List Elements
- List Operations
- Exploring Tuples and Sets
- Tuples
- Sets
- Diving into Dictionaries
- Working with Arrays
- Creating an Array
- Accessing Array Elements
- Modifying Array Elements
- Working with Array Methods
- Iterating over an Array
- Manipulating Stacks and Queues
- Stacks
- Queues
- Implementing Linked Lists
- Using Heaps and Priority Queues
- Creating a Heap
- Inserting and Removing Elements
- Getting the Smallest Element
- Heapify vs. Sorted
- Priority Queues
- Harnessing Trees and Graphs
- Trees
- Graphs
- Applying Collections in Real World Scenarios
- 1. Data Analysis
- 2. Task Management
- 3. Web Scraping
- Advanced Techniques for Python Collections
- Sorting Collections
- Filtering Collections
- Manipulating Collections
- Optimizing Performance with Collections
- 1. Lists vs. Tuples
- 2. Sets
- 3. Dictionaries
- 4. Collections Module
- Handling Large Data Sets with Collections
- DefaultDict
- Counter
- Deque
- Understanding Memory Management in Collections
- Exploring Parallel Processing with Collections
- Multiprocessing
- Threadpool
Getting Started with Python Collections
Python Collections are containers that are used to store and manage collections of data efficiently. These collections provide various built-in functions and methods to manipulate and access the data stored in them.
Python provides several types of collections, including lists, tuples, sets, and dictionaries. Each collection type has its own unique features and use cases. In this chapter, we will explore these different collection types and learn how to use them effectively in Python programming.
Related Article: How To Convert a Dictionary To JSON In Python
Lists
A list in Python is an ordered collection of items, enclosed in square brackets ([]). It can store elements of different data types, such as numbers, strings, or even other lists. Lists are mutable, which means that you can modify their elements after creation.
Here is an example of creating a list in Python:
fruits = ["apple", "banana", "orange"]
You can access individual elements of a list using their index. The index of the first element is 0, the second element is 1, and so on. Negative indexing is also supported, where -1 refers to the last element, -2 refers to the second last element, and so on.
print(fruits[0]) # Output: apple print(fruits[-1]) # Output: orange
Lists also support various operations, such as appending elements, removing elements, and slicing.
Tuples
A tuple is similar to a list, but it is immutable, meaning that its elements cannot be modified after creation. Tuples are defined using round brackets (()).
Here is an example of creating a tuple in Python:
colors = ("red", "green", "blue")
You can access individual elements of a tuple using their index, similar to lists.
print(colors[0]) # Output: red print(colors[-1]) # Output: blue
Since tuples are immutable, they are useful in situations where you want to ensure that the data remains constant and cannot be accidentally modified.
Sets
A set in Python is an unordered collection of unique elements. It is defined using curly braces ({}) or the built-in set()
function.
Here is an example of creating a set in Python:
fruits = {"apple", "banana", "orange"}
Sets do not allow duplicate elements, so if you try to add a duplicate element, it will be ignored.
You can perform various set operations, such as union, intersection, difference, and more.
Related Article: How to Sort a Dictionary by Key in Python
Dictionaries
A dictionary in Python is an unordered collection of key-value pairs. Each key-value pair is separated by a colon (:), and the pairs are enclosed in curly braces ({}).
Here is an example of creating a dictionary in Python:
person = {"name": "John", "age": 30, "city": "New York"}
You can access the value associated with a particular key using the key itself.
print(person["name"]) # Output: John print(person["age"]) # Output: 30
Dictionaries are commonly used to store and retrieve data based on some unique identifier (the key).
In this chapter, we have covered the basic introduction to Python collections, including lists, tuples, sets, and dictionaries. These collection types provide different ways to store and manage data in Python, depending on the requirements of your program. Now that you have a basic understanding of these collection types, you can start using them in your Python programs to store and manipulate data efficiently.
Lists
In Python, a list is a versatile and commonly used data structure that allows you to store and manipulate a collection of items. Lists are mutable, ordered, and can contain elements of different data types. They are one of the fundamental building blocks in Python programming.
Creating Lists
To create a list in Python, you can enclose a comma-separated sequence of values within square brackets []. For example:
fruits = ['apple', 'banana', 'orange']
You can create an empty list by simply using empty square brackets:
empty_list = []
Lists can also contain elements of different data types:
mixed_list = [42, 'hello', 3.14, True]
Related Article: How to Remove a Key from a Python Dictionary
Accessing List Elements
List elements can be accessed using their indices. Python uses zero-based indexing, which means the first element has an index of 0. You can access elements of a list by specifying the index within square brackets. For example:
fruits = ['apple', 'banana', 'orange'] print(fruits[0]) # Output: 'apple' print(fruits[1]) # Output: 'banana'
You can also use negative indices to access elements from the end of the list. For instance:
print(fruits[-1]) # Output: 'orange' print(fruits[-2]) # Output: 'banana'
Modifying List Elements
Lists are mutable, which means you can modify their elements. You can assign a new value to a specific index in a list to change its element. For example:
fruits = ['apple', 'banana', 'orange'] fruits[1] = 'grape' print(fruits) # Output: ['apple', 'grape', 'orange']
List Operations
Python provides various operations to manipulate lists. Here are some commonly used ones:
Appending Elements: You can use the append()
method to add an element to the end of a list. For example:
fruits = ['apple', 'banana'] fruits.append('orange') print(fruits) # Output: ['apple', 'banana', 'orange']
Removing Elements: The remove()
method allows you to remove an element from a list. It takes the value of the element you want to remove as an argument. For example:
fruits = ['apple', 'banana', 'orange'] fruits.remove('banana') print(fruits) # Output: ['apple', 'orange']
Sorting Elements: The sort()
method sorts the elements of a list in ascending order. For example:
numbers = [5, 2, 8, 1] numbers.sort() print(numbers) # Output: [1, 2, 5, 8]
Length of a List: You can use the len()
function to determine the number of elements in a list. For example:
fruits = ['apple', 'banana', 'orange'] print(len(fruits)) # Output: 3
Related Article: How to Remove an Element from a List by Index in Python
Exploring Tuples and Sets
In this chapter, we will dive into two important collections in Python: tuples and sets. These collections allow you to store multiple values in a single variable and provide different functionalities depending on your needs.
Tuples
A tuple is an ordered and immutable collection of elements. This means that once a tuple is created, you cannot modify its elements. Tuples are enclosed in parentheses and can contain elements of different data types.
To create a tuple, you can simply separate the elements with commas and enclose them in parentheses:
fruits = ('apple', 'banana', 'orange')
You can access individual elements of a tuple using indexing. Indexing starts from 0, so the first element is at index 0, the second at index 1, and so on:
first_fruit = fruits[0] # 'apple' second_fruit = fruits[1] # 'banana'
Tuples also support negative indexing, where -1 refers to the last element, -2 refers to the second last element, and so on:
last_fruit = fruits[-1] # 'orange' second_last_fruit = fruits[-2] # 'banana'
You can also use slicing to extract a portion of the tuple:
selected_fruits = fruits[1:3] # ('banana', 'orange')
Tuples are commonly used to group related values together, such as coordinates or RGB color codes. They are also used as keys in dictionaries because of their immutability.
Sets
A set is an unordered collection of unique elements. Unlike tuples, sets are mutable, meaning you can add or remove elements from them. Sets are enclosed in curly braces or can be created using the set()
function.
To create a set, you can define it using curly braces:
fruits = {'apple', 'banana', 'orange'}
You can also create a set from a list by using the set()
function:
fruits_list = ['apple', 'banana', 'orange'] fruits = set(fruits_list)
Sets automatically remove duplicate elements, so if you try to add the same element multiple times, it will only appear once in the set.
Sets provide various operations like union, intersection, and difference. You can perform these operations using methods or operators.
set1 = {1, 2, 3} set2 = {3, 4, 5} union = set1.union(set2) # {1, 2, 3, 4, 5} intersection = set1.intersection(set2) # {3} difference = set1 - set2 # {1, 2}
Sets are commonly used when you want to store a collection of items without any particular order and without any duplicates.
Now that you have an understanding of tuples and sets, you can start using them in your Python programs to store and manipulate collections of data in a more efficient way.
Related Article: How to Solve a Key Error in Python
Diving into Dictionaries
In the previous chapter, we explored the concept of lists in Python and how they can be used to store and organize data. Now, we will dive into another essential data structure in Python – dictionaries.
Dictionaries in Python are unordered collections of key-value pairs. Unlike lists, which are indexed by a range of numbers, dictionaries are indexed by keys, which can be of any immutable type. This means that dictionaries allow us to store and retrieve values using a unique key instead of a numerical index.
To create a dictionary, we use curly braces {} and separate the keys and values with a colon (:). Let’s take a look at an example:
person = {"name": "John", "age": 25, "city": "New York"}
In this example, we have created a dictionary called person
, which contains three key-value pairs. The keys are “name”, “age”, and “city”, and the corresponding values are “John”, 25, and “New York” respectively.
We can access the values in a dictionary by using the corresponding key. For example:
print(person["name"]) # Output: John
In this example, we use the key “name” to access the value “John” from the person
dictionary.
One of the advantages of dictionaries is that they allow us to store different types of values. For instance, we can have a dictionary with keys of type string and values of type integer, string, or even other dictionaries. Here’s an example:
my_dict = {"key1": 123, "key2": "value2", "key3": {"nested_key": "nested_value"}}
In this case, the value associated with the key “key1” is an integer, the value associated with the key “key2” is a string, and the value associated with the key “key3” is another dictionary.
Dictionaries also provide several useful methods to manipulate and retrieve data. Some of the commonly used methods include:
– keys()
: Returns a list of all the keys in the dictionary.
– values()
: Returns a list of all the values in the dictionary.
– items()
: Returns a list of tuples, where each tuple contains a key-value pair.
Here’s an example that demonstrates these methods:
my_dict = {"name": "Alice", "age": 30, "city": "London"} print(my_dict.keys()) # Output: dict_keys(['name', 'age', 'city']) print(my_dict.values()) # Output: dict_values(['Alice', 30, 'London']) print(my_dict.items()) # Output: dict_items([('name', 'Alice'), ('age', 30), ('city', 'London')])
In this example, we use the keys()
, values()
, and items()
methods on the my_dict
dictionary to retrieve the keys, values, and key-value pairs respectively.
Dictionaries are incredibly versatile and widely used in Python. They provide a flexible way to store and manipulate data, making them an indispensable tool for many programming tasks.
Working with Arrays
Arrays are a fundamental data structure in Python that allow you to store multiple values in a single variable. They are similar to lists but have some key differences. In this chapter, we will explore how to work with arrays in Python.
Creating an Array
To create an array in Python, you need to import the array
module from the array
package. Then, you can use the array()
function to create an array by specifying the type code and the initial values.
import array my_array = array.array('i', [1, 2, 3, 4, 5])
In the example above, we create an array of integers ('i'
) with the initial values [1, 2, 3, 4, 5]
. You can specify different type codes depending on the type of values you want to store in the array.
Related Article: How to Add New Keys to a Python Dictionary
Accessing Array Elements
You can access individual elements of an array using square brackets []
and the index of the element you want to access. Array indices start from 0.
import array my_array = array.array('i', [1, 2, 3, 4, 5]) print(my_array[0]) # Output: 1 print(my_array[2]) # Output: 3
In the example above, we access the first element of the array (1
) using my_array[0]
and the third element (3
) using my_array[2]
.
Modifying Array Elements
You can modify the value of an element in an array by assigning a new value to it using the assignment operator =
.
import array my_array = array.array('i', [1, 2, 3, 4, 5]) my_array[0] = 10 my_array[2] = 30 print(my_array) # Output: array('i', [10, 2, 30, 4, 5])
In the example above, we modify the value of the first element of the array (1
) to 10
and the value of the third element (3
) to 30
. The output shows the updated array.
Working with Array Methods
Arrays in Python come with several useful methods that allow you to manipulate and perform operations on arrays. Some commonly used methods include:
– append()
: Adds an element to the end of the array.
– insert()
: Inserts an element at a specified position in the array.
– remove()
: Removes the first occurrence of an element from the array.
– pop()
: Removes and returns the element at a specified position in the array.
– index()
: Returns the index of the first occurrence of an element in the array.
import array my_array = array.array('i', [1, 2, 3, 4, 5]) my_array.append(6) my_array.insert(0, 0) my_array.remove(3) my_array.pop(2) index = my_array.index(4) print(my_array) # Output: array('i', [0, 1, 4, 5, 6]) print(index) # Output: 2
In the example above, we use various array methods to append an element (6
) to the end of the array, insert an element (0
) at the beginning of the array, remove the first occurrence of an element (3
), remove and return an element at a specified position (2
), and find the index of an element (4
).
Related Article: How to Read a File Line by Line into a List in Python
Iterating over an Array
You can iterate over the elements of an array using a for
loop.
import array my_array = array.array('i', [1, 2, 3, 4, 5]) for element in my_array: print(element)
In the example above, we iterate over the elements of the array and print each element on a new line.
Arrays are a powerful tool for storing and manipulating multiple values in Python. In this chapter, we covered the basics of working with arrays, including creating arrays, accessing and modifying array elements, using array methods, and iterating over arrays. With these skills, you can start using arrays in your Python programs effectively.
Manipulating Stacks and Queues
In this chapter, we will explore two commonly used data structures in Python: stacks and queues. Both of these data structures are collections of items that can be manipulated using specific operations.
Stacks
A stack is a Last-In-First-Out (LIFO) data structure, which means that the last element added to the stack will be the first one to be removed. Think of a stack as a pile of books, where the last book you added is the first one you can take out.
Python provides a built-in class called list
that can be used as a stack. Let’s create a stack and perform some operations on it:
stack = [] # Pushing elements onto the stack stack.append(1) stack.append(2) stack.append(3) # Popping elements from the stack print(stack.pop()) # Output: 3 print(stack.pop()) # Output: 2 print(stack.pop()) # Output: 1
In the code snippet above, we create an empty list stack
and use the append()
method to add elements to the stack. The pop()
method is then used to remove and return the last element added to the stack.
Related Article: How to Check If Something Is Not In A Python List
Queues
A queue is a First-In-First-Out (FIFO) data structure, which means that the first element added to the queue will be the first one to be removed. Think of a queue as a line of people waiting for a bus, where the person who arrives first is the first one to board the bus.
Python does not provide a built-in class for queues, but we can use the deque
class from the collections
module. Let’s create a queue and perform some operations on it:
from collections import deque queue = deque() # Enqueuing elements into the queue queue.append(1) queue.append(2) queue.append(3) # Dequeuing elements from the queue print(queue.popleft()) # Output: 1 print(queue.popleft()) # Output: 2 print(queue.popleft()) # Output: 3
In the code snippet above, we import the deque
class from the collections
module and create an empty deque object queue
. We use the append()
method to enqueue elements into the queue and the popleft()
method to dequeue elements from the queue.
Implementing Linked Lists
A linked list is a popular data structure in computer science that consists of a sequence of nodes. Each node contains a data element and a reference (or link) to the next node in the sequence. Linked lists are commonly used to implement other data structures such as stacks, queues, and hash tables.
In Python, we can implement a linked list using classes. Let’s start by creating a Node class that represents a single node in the linked list:
class Node: def __init__(self, data): self.data = data self.next = None
The Node
class has two attributes: data
, which holds the data element of the node, and next
, which is a reference to the next node in the sequence.
To create a linked list, we need to define a LinkedList class that manages the nodes:
class LinkedList: def __init__(self): self.head = None
The LinkedList
class has a single attribute head
, which is a reference to the first node in the list. Initially, the list is empty, so head
is set to None
.
To add a new node to the linked list, we can use the insert
method:
class LinkedList: # ... def insert(self, data): new_node = Node(data) if self.head is None: self.head = new_node else: current = self.head while current.next is not None: current = current.next current.next = new_node
The insert
method takes a data
parameter and creates a new node with that data. If the list is empty (i.e., head
is None
), the new node becomes the first node in the list. Otherwise, we iterate through the list until we reach the last node and append the new node to the next
attribute of the last node.
To retrieve the data elements in the linked list, we can use the traverse
method:
class LinkedList: # ... def traverse(self): current = self.head while current is not None: print(current.data) current = current.next
The traverse
method starts from the head
of the list and iterates through each node, printing the data element.
Let’s see an example of creating and using a linked list:
# Create a new linked list my_list = LinkedList() # Insert elements into the list my_list.insert(10) my_list.insert(20) my_list.insert(30) # Traverse the list and print the data elements my_list.traverse()
This will output:
10 20 30
Linked lists are flexible and efficient data structures for certain types of operations. However, they have some drawbacks, such as slower access to elements and more memory usage compared to arrays. It’s important to consider the requirements of your program before choosing a data structure.
Using Heaps and Priority Queues
Heaps and priority queues are useful data structures for managing and retrieving elements based on their priority. In Python, these data structures are implemented using the heapq
module.
A heap is a binary tree that satisfies the heap property: for any given node, its value is greater than or equal to the values of its child nodes. This property allows the smallest or largest element to be efficiently accessed. Heaps are commonly used to implement priority queues.
To use heaps and priority queues in Python, you need to import the heapq
module:
import heapq
Related Article: How to Pretty Print Nested Dictionaries in Python
Creating a Heap
To create a heap, you can use the heapify
function provided by the heapq
module. This function rearranges the elements of a list in such a way that it satisfies the heap property:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] heapq.heapify(numbers) print(numbers)
Output:
[1, 1, 2, 3, 3, 9, 4, 6, 5, 5, 5]
Inserting and Removing Elements
Once you have created a heap, you can insert elements using the heappush
function:
heap = [] heapq.heappush(heap, 4) heapq.heappush(heap, 1) heapq.heappush(heap, 7) print(heap)
Output:
[1, 4, 7]
To remove the smallest element from the heap, you can use the heappop
function:
smallest = heapq.heappop(heap) print(smallest) print(heap)
Output:
1 [4, 7]
Getting the Smallest Element
To retrieve the smallest element from a heap without removing it, you can use the heappushpop
function. This function pushes a new element onto the heap and returns the smallest element:
smallest = heapq.heappushpop(heap, 2) print(smallest) print(heap)
Output:
1 [2, 4, 7]
Related Article: How to Find a Value in a Python List
Heapify vs. Sorted
The heapify
function is more efficient than sorting a list using the sorted
function because it has a time complexity of O(n), whereas sorting has a time complexity of O(n log n). However, if you need to retrieve all the elements in sorted order, using the sorted
function on the heap is more efficient:
sorted_numbers = sorted(numbers) print(sorted_numbers)
Output:
[1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
Priority Queues
A priority queue is a variation of a heap where each element is associated with a priority. The element with the highest priority is always at the front of the queue. In Python, you can use tuples to represent elements with their priorities.
To create a priority queue, you can use the heappush
and heappop
functions as shown earlier. However, instead of inserting a single element, you insert a tuple containing both the priority and the element:
queue = [] heapq.heappush(queue, (2, 'Task 1')) heapq.heappush(queue, (1, 'Task 2')) heapq.heappush(queue, (3, 'Task 3')) print(queue)
Output:
[(1, 'Task 2'), (2, 'Task 1'), (3, 'Task 3')]
To retrieve the highest priority element, you can use the same heappop
function:
highest_priority = heapq.heappop(queue) print(highest_priority) print(queue)
Output:
(1, 'Task 2') [(2, 'Task 1'), (3, 'Task 3')]
Harnessing Trees and Graphs
Trees and graphs are powerful data structures that can be used to represent complex relationships between objects. In this chapter, we will explore how to harness the power of trees and graphs in Python.
Related Article: How to Extract Unique Values from a List in Python
Trees
A tree is a hierarchical data structure consisting of nodes connected by edges. The topmost node in a tree is called the root, and each node can have zero or more child nodes. Trees are widely used in computer science and are particularly useful for representing hierarchical relationships.
In Python, we can represent a tree using classes. Each node in the tree can be represented as an instance of a class, which contains data and references to its child nodes. Here’s an example of how to define a simple binary tree:
class Node: def __init__(self, data): self.data = data self.left = None self.right = None # Create the root node root = Node(1) # Create the child nodes root.left = Node(2) root.right = Node(3)
This code defines a Node
class with a constructor that takes a data
parameter. Each node has a left
and right
attribute, which can be used to reference its child nodes. We create a binary tree by creating instances of the Node
class and assigning them as child nodes to the root node.
Graphs
A graph is a collection of nodes, also known as vertices, connected by edges. Unlike trees, graphs can have cycles and can be more complex in structure. Graphs are used to model relationships between objects, such as social networks or transportation networks.
In Python, we can represent a graph using various data structures. One common approach is to use an adjacency list, which is a dictionary that maps each node to a list of its neighboring nodes. Here’s an example of how to define a simple graph using an adjacency list:
graph = { 'A': ['B', 'C'], 'B': ['C', 'D'], 'C': ['D'], 'D': ['C'], 'E': ['F'], 'F': ['C'] }
This code defines a dictionary graph
where the keys represent the nodes and the values represent the neighboring nodes. For example, the node ‘A’ is connected to nodes ‘B’ and ‘C’. The node ‘C’ is connected to nodes ‘D’ and ‘F’. This representation allows us to easily traverse the graph and explore its relationships.
Applying Collections in Real World Scenarios
In the previous chapters, we have explored various collections available in Python, such as lists, tuples, sets, and dictionaries. We have seen how these collections can be used to store and manipulate data efficiently. In this chapter, we will dive deeper into real-world scenarios where collections play a crucial role.
Related Article: How to Remove Duplicates From Lists in Python
1. Data Analysis
Python collections are widely used in data analysis tasks. Let’s consider a scenario where we have a dataset of student grades. We can use a dictionary to store the grades of each student, where the student ID is the key and the grade is the value.
grades = { 101: 85, 102: 92, 103: 78, 104: 90, 105: 87 }
With this data structure, we can easily access and manipulate the grades. For example, we can calculate the average grade of all students:
average_grade = sum(grades.values()) / len(grades) print(f"Average Grade: {average_grade}")
2. Task Management
Collections are also useful in managing tasks or to-do lists. Let’s consider a scenario where we need to keep track of tasks and their respective deadlines. We can use a list of dictionaries to represent each task, where each dictionary contains the task description and deadline.
tasks = [ { "description": "Write article", "deadline": "2022-12-31" }, { "description": "Prepare presentation", "deadline": "2022-12-15" }, { "description": "Submit report", "deadline": "2022-11-30" } ]
We can loop through the list and perform operations on each task, such as checking if a task is overdue or sorting the tasks based on the deadline.
for task in tasks: deadline = task["deadline"] if deadline < datetime.now().strftime("%Y-%m-%d"): print(f"Task '{task['description']}' is overdue!") else: print(f"Task '{task['description']}' is on track.")
3. Web Scraping
Collections are also essential in web scraping tasks, where we extract data from websites. Let’s consider a scenario where we want to scrape a web page and extract all the links present on that page. We can use a set to store the links, ensuring that each link is unique.
import requests from bs4 import BeautifulSoup url = "https://www.example.com" response = requests.get(url) soup = BeautifulSoup(response.text, "html.parser") links = set() for link in soup.find_all("a"): href = link.get("href") links.add(href) print(links)
In this example, we use the requests
library to send an HTTP request to the specified URL and the BeautifulSoup
library to parse the HTML content. We then find all the <a>
tags on the page and extract the href
attribute to get the links.
These are just a few examples of how collections can be applied in real-world scenarios. Python collections provide a versatile and powerful way to handle data in various domains, making Python an excellent choice for many applications.
Keep exploring the different collections and experiment with them to deepen your understanding and proficiency in Python.
Related Article: How to Compare Two Lists in Python and Return Matches
Advanced Techniques for Python Collections
In this chapter, we will explore some advanced techniques for working with Python collections. We will delve into topics such as sorting, filtering, and manipulating collections in more complex ways. Let’s get started!
Sorting Collections
Sorting a collection is a common operation in programming. Python provides several methods to sort collections, such as lists, tuples, and dictionaries. One common method is to use the sorted()
function, which returns a new sorted list based on the given collection.
Here’s an example of sorting a list of integers in ascending order:
numbers = [5, 2, 8, 1, 9] sorted_numbers = sorted(numbers) print(sorted_numbers) # Output: [1, 2, 5, 8, 9]
You can also sort collections in descending order by passing the reverse=True
argument to the sorted()
function.
numbers = [5, 2, 8, 1, 9] sorted_numbers = sorted(numbers, reverse=True) print(sorted_numbers) # Output: [9, 8, 5, 2, 1]
Filtering Collections
Filtering a collection allows you to extract specific elements based on certain conditions. Python provides the filter()
function, which can be used to filter elements from a collection.
Here’s an example of filtering a list to only include even numbers:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4, 6, 8, 10]
The filter()
function takes a function and an iterable as arguments. The function is applied to each element in the iterable, and only the elements for which the function returns True
are included in the filtered collection.
Related Article: How to Print a Python Dictionary Line by Line
Manipulating Collections
Python provides a powerful set of methods to manipulate collections. These methods include operations such as mapping, reducing, and flattening collections.
The map()
function applies a given function to each element of a collection and returns a new collection with the results. Here’s an example of doubling each element in a list:
numbers = [1, 2, 3, 4, 5] doubled_numbers = list(map(lambda x: x * 2, numbers)) print(doubled_numbers) # Output: [2, 4, 6, 8, 10]
The reduce()
function takes a function and an iterable and applies the function to the first two elements, then to the result and the next element, and so on, until a single value is obtained. Here’s an example of summing all elements in a list:
from functools import reduce numbers = [1, 2, 3, 4, 5] sum_of_numbers = reduce(lambda x, y: x + y, numbers) print(sum_of_numbers) # Output: 15
The flatten()
function can be used to flatten a nested collection into a single-level collection. Here’s an example of flattening a list of lists:
nested_list = [[1, 2], [3, 4], [5, 6]] flattened_list = [item for sublist in nested_list for item in sublist] print(flattened_list) # Output: [1, 2, 3, 4, 5, 6]
These advanced techniques for manipulating collections can be incredibly useful in various programming scenarios, allowing you to transform, filter, and combine data in powerful ways.
Optimizing Performance with Collections
In this chapter, we will explore how to optimize the performance of your Python code using various collections available in the Python standard library. Collections are data structures that are designed to efficiently store and manipulate groups of related data.
1. Lists vs. Tuples
Lists and tuples are two commonly used collection types in Python. However, there are some key differences between them that can impact performance.
Lists are mutable, meaning their elements can be modified after creation. Tuples, on the other hand, are immutable, meaning their elements cannot be modified once they are assigned. This immutability makes tuples more memory-efficient and faster to access compared to lists.
If you have a collection of items that you do not need to modify, using a tuple instead of a list can provide a performance boost. Additionally, tuples can be used as keys in dictionaries or elements in sets, while lists cannot.
Related Article: How to Use Hash Map In Python
2. Sets
Sets are another collection type in Python that can be used to store a collection of unique elements. They are implemented using a hash table, which allows for constant-time average case complexity for operations like adding, removing, and checking for the existence of an element.
If you need to perform operations like membership testing or removing duplicates from a list, using a set can be more efficient than using a list or a tuple. However, sets are unordered, meaning the order of elements is not preserved.
Here’s an example of using a set to remove duplicates from a list:
my_list = [1, 2, 3, 3, 4, 5, 5] my_set = set(my_list) unique_list = list(my_set) print(unique_list) # Output: [1, 2, 3, 4, 5]
3. Dictionaries
Dictionaries are key-value pairs that allow you to store and retrieve data efficiently. They are implemented using a hash table, providing constant-time average case complexity for operations like inserting, deleting, and retrieving elements.
To optimize performance when working with dictionaries, you can:
– Use the get()
method instead of direct indexing to avoid KeyError exceptions if a key does not exist.
– Use dictionary comprehension instead of traditional loops for creating dictionaries from other collections.
– Use the items()
method to efficiently iterate over key-value pairs.
– Use the in
operator to check for the existence of a key in a dictionary.
Here’s an example of using dictionary comprehension to create a dictionary from a list:
my_list = [1, 2, 3, 4, 5] my_dict = {x: x**2 for x in my_list} print(my_dict) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
4. Collections Module
The Python standard library provides the collections
module, which offers additional high-performance data structures compared to the built-in collections. Some notable collections in this module include deque
, Counter
, and defaultdict
.
– deque
: A double-ended queue that allows efficient insertion and removal from both ends. It is especially useful when implementing algorithms that require a queue or stack.
– Counter
: A dictionary subclass that allows you to count the occurrences of elements in a collection. It provides efficient methods for common operations like counting, adding, and subtracting.
– defaultdict
: A dictionary subclass that provides a default value for keys that do not exist. This can be useful when working with nested dictionaries or counting occurrences of elements.
To use the collections module, you need to import it first:
import collections
In this chapter, we have explored various collections available in Python and how they can be optimized for performance. By choosing the right collection type and utilizing the methods and features provided by these collections, you can write more efficient and faster Python code.
Related Article: How to Detect Duplicates in a Python List
Handling Large Data Sets with Collections
In this chapter, we will explore how to handle large data sets using the powerful collections module in Python.
When working with large data sets, it is important to efficiently store and manipulate the data to avoid performance issues. The collections module provides specialized data structures that are optimized for specific tasks, such as storing large amounts of data and performing operations on them.
DefaultDict
The defaultdict class is a subclass of the built-in dictionary class. It allows us to define a default value for keys that are not present in the dictionary. This can be useful when working with large data sets, as it eliminates the need to check if a key exists before performing operations on it.
Here’s an example of how to use a defaultdict to count the occurrences of words in a large text file:
from collections import defaultdict word_counts = defaultdict(int) with open('large_text_file.txt', 'r') as file: for line in file: words = line.split() for word in words: word_counts[word] += 1 print(word_counts)
In this example, we create a defaultdict with a default value of 0 using the int
function. We then iterate over each line in the file, split it into words, and increment the count of each word in the dictionary.
Counter
The Counter class is another useful tool for handling large data sets. It is a subclass of defaultdict and is specifically designed for counting hashable objects. It provides a convenient way to count the occurrences of elements in a collection.
Here’s an example of how to use a Counter to count the occurrences of elements in a large list:
from collections import Counter data = [1, 2, 3, 4, 5, 1, 2, 3, 4, 1, 2, 1, 1, 1] counter = Counter(data) print(counter)
In this example, we create a Counter object by passing a list to it. The Counter object counts the occurrences of each element in the list and stores them in a dictionary-like format.
Related Article: How To Find Index Of Item In Python List
Deque
The deque class is a double-ended queue implementation in Python. It provides efficient insertion and deletion from both ends of the queue, making it suitable for handling large data sets where frequent modifications are required.
Here’s an example of how to use a deque to process a large data set in chunks:
from collections import deque data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] chunk_size = 3 chunks = deque(maxlen=chunk_size) for item in data: chunks.append(item) if len(chunks) == chunk_size: process_chunk(chunks)
In this example, we create a deque with a maximum length of chunk_size
. We iterate over the data set and append each item to the deque. When the length of the deque reaches the chunk size, we process the chunk and remove the oldest item from the deque.
Using a deque for processing large data sets in chunks helps to conserve memory and improve performance by only keeping a limited number of items in memory at a time.
Understanding Memory Management in Collections
Memory management is an important concept in programming, especially when working with collections in Python. Understanding how memory is allocated and released can help you write more efficient and optimized code.
In Python, memory management is handled by the Python interpreter’s memory manager. The memory manager is responsible for allocating memory to objects and releasing memory when it is no longer needed.
Python uses a technique called reference counting to manage memory. Reference counting keeps track of the number of references to an object. When the reference count of an object reaches zero, meaning there are no more references to it, the memory manager frees the memory occupied by that object.
Let’s take a look at an example to understand this concept better. Suppose we have a list called my_list
:
my_list = [1, 2, 3]
In this example, my_list
is a reference to a list object in memory. The reference count of this object is initially 1 because my_list
is the only reference to it. If we create another reference to the same list object, the reference count will increase to 2:
another_list = my_list
Now, both my_list
and another_list
refer to the same list object, and the reference count is 2. If we remove one of the references, the reference count will decrease accordingly:
del my_list
After deleting the my_list
reference, the reference count of the list object decreases to 1. If we delete the another_list
reference as well, the reference count will become 0, and the memory manager will free the memory occupied by the list object.
Python also provides a garbage collector that deals with objects that have circular references. Circular references occur when objects refer to each other in a way that creates a loop. The garbage collector identifies these circular references and frees the memory occupied by the objects involved.
While reference counting is an efficient memory management technique, it is not perfect. It may not handle all cases, such as circular references, which is why the garbage collector is there to handle those scenarios.
Understanding memory management in collections can help you write more efficient code. When working with large collections, it is important to keep memory usage in mind and optimize your code accordingly.
Exploring Parallel Processing with Collections
Parallel processing is a technique that allows multiple tasks to be executed simultaneously, thereby improving the performance and efficiency of an application. Python provides several collections that can be used to implement parallel processing. In this chapter, we will explore some of the popular collections for parallel processing in Python.
Related Article: Extracting File Names from Path in Python, Regardless of OS
Multiprocessing
The multiprocessing
module in Python is a powerful tool for parallel processing. It allows you to spawn multiple processes, each running in its own Python interpreter. These processes can run in parallel and can be used for a wide range of tasks, such as CPU-intensive computations, network programming, and more.
To use the multiprocessing
module, you need to import it first:
import multiprocessing
One of the main components of the multiprocessing
module is the Process
class, which represents a process. You can create a new process by instantiating the Process
class and passing a target function to it. The target function will be executed in a separate process.
Here’s a simple example that demonstrates how to use the multiprocessing
module:
import multiprocessing def square(n): return n * n if __name__ == '__main__': # Create a new process p = multiprocessing.Process(target=square, args=(5,)) # Start the process p.start() # Wait for the process to finish p.join()
In this example, we define a function square
that takes a number n
as input and returns its square. We then create a new process p
and pass the square
function as the target. We also pass the argument 5
to the function using the args
parameter. Finally, we start the process using the start
method and wait for it to finish using the join
method.
Threadpool
The concurrent.futures
module in Python provides a high-level interface for asynchronously executing callables. It includes a ThreadPoolExecutor
class that allows you to easily create and manage a pool of worker threads.
To use the ThreadPoolExecutor
class, you need to import it from the concurrent.futures
module:
from concurrent.futures import ThreadPoolExecutor
Here’s an example that demonstrates how to use the ThreadPoolExecutor
class:
from concurrent.futures import ThreadPoolExecutor def square(n): return n * n if __name__ == '__main__': # Create a thread pool with 5 worker threads with ThreadPoolExecutor(max_workers=5) as executor: # Submit a task to the thread pool future = executor.submit(square, 5) # Get the result of the task result = future.result() print(result)
In this example, we define a function square
that takes a number n
as input and returns its square. We then create a ThreadPoolExecutor
with a maximum of 5 worker threads. We submit a task to the thread pool using the submit
method and pass the square
function as the callable. We also pass the argument 5
to the function. Finally, we use the result
method of the future
object to get the result of the task.