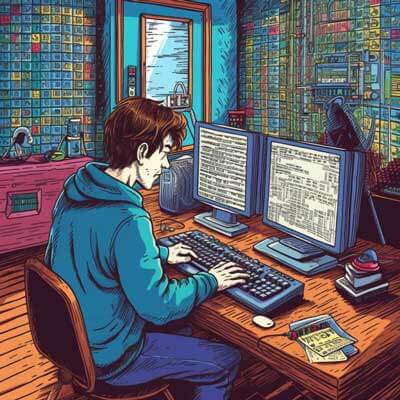
- Introduction to File Operations
- Opening and Closing Files
- Reading Files
- Writing to Files
- Deleting Files
- Copying Files
- Working with Directories
- Error Handling in File Operations
- File Modes and Their Uses
- Using the With Statement in File Handling
- File Object Attributes
- Use Case: Logging System Events
- Use Case: Automated Data Backup
- Best Practice: Safe File Handling
- Best Practice: Efficient File I/O
- Real World Example: Configuration File Parser
- Real World Example: CSV Data Processor
- Performance Consideration: Large File Handling
- Performance Consideration: File I/O and Memory Usage
- Advanced Technique: Binary File Operations
- Advanced Technique: File Serialization
- Code Snippet: Read File Line by Line
- Code Snippet: Write List to File
- Code Snippet: Delete File if Exists
- Code Snippet: Copy File to Another Directory
- Code Snippet: Recursive Directory Traversal
Introduction to File Operations
File operations are an integral part of any programming language, including Python. They allow us to interact with files stored on our computer’s file system. In this chapter, we will explore various file operations in Python, including opening and closing files, reading from files, writing to files, deleting files, and copying files.
Related Article: String Comparison in Python: Best Practices and Techniques
Opening and Closing Files
To perform any operation on a file, we first need to open it. Python provides a built-in function called open()
for this purpose. The open()
function takes two parameters: the file name or path, and the mode in which we want to open the file.
Here’s an example of opening a file in read mode:
file = open("example.txt", "r")
In this example, we open a file named “example.txt” in read mode (“r”). Other common modes include write mode (“w”), append mode (“a”), and binary mode (“b”). The default mode is read mode if no mode is specified.
Once we have finished working with a file, it is important to close it to free up system resources. We can use the close()
method of the file object to close the file.
Here’s an example:
file = open("example.txt", "r") # Perform operations on the file file.close()
Reading Files
Python provides several methods for reading from a file. The most common method is to read the entire contents of a file using the read()
method. This method returns the contents of the file as a string.
Here’s an example:
file = open("example.txt", "r") contents = file.read() print(contents) file.close()
Another useful method is readline()
, which reads a single line from the file. We can use a loop to read multiple lines.
Here’s an example:
file = open("example.txt", "r") line = file.readline() while line: print(line) line = file.readline() file.close()
Writing to Files
To write to a file, we need to open it in write mode (“w”) or append mode (“a”). Write mode overwrites the entire file, while append mode appends to the existing content.
Here’s an example of writing to a file:
file = open("example.txt", "w") file.write("Hello, World!") file.close()
In this example, we open the file in write mode and write the string “Hello, World!” to it. If the file doesn’t exist, Python will create it.
We can also write multiple lines to a file by using the writelines()
method.
Here’s an example:
lines = ["Line 1\n", "Line 2\n", "Line 3\n"] file = open("example.txt", "w") file.writelines(lines) file.close()
Related Article: How To Limit Floats To Two Decimal Points In Python
Deleting Files
To delete a file in Python, we can use the os.remove()
function from the os
module. This function takes the file path as a parameter and deletes the file from the file system.
Here’s an example:
import os os.remove("example.txt")
In this example, we delete the file named “example.txt” using the os.remove()
function.
Copying Files
To copy a file in Python, we can use the shutil
module, which provides a high-level interface for file operations. The shutil.copy()
function allows us to copy a file from one location to another.
Here’s an example:
import shutil shutil.copy("source.txt", "destination.txt")
In this example, we copy the file “source.txt” to “destination.txt” using the shutil.copy()
function.
Working with Directories
In addition to working with files, Python also provides various functions and methods for working with directories. We can create directories, list the contents of a directory, rename directories, and delete directories using these functions and methods.
Here’s an example of creating a directory:
import os os.mkdir("my_directory")
In this example, we create a directory named “my_directory” using the os.mkdir()
function.
To list the contents of a directory, we can use the os.listdir()
function.
Here’s an example:
import os contents = os.listdir("my_directory") print(contents)
In this example, we list the contents of the “my_directory” directory using the os.listdir()
function.
Related Article: How To Rename A File With Python
Error Handling in File Operations
When working with files, it is important to handle errors that may occur. Python provides a mechanism for error handling using try-except blocks. By wrapping our file operations inside a try block, we can catch and handle any exceptions that may occur.
Here’s an example:
try: file = open("example.txt", "r") contents = file.read() print(contents) file.close() except FileNotFoundError: print("File not found.") except Exception as e: print("An error occurred:", str(e))
In this example, we use a try-except block to handle the FileNotFoundError exception that may occur if the file is not found. We also have a generic except block to catch any other exceptions and print an error message.
File Modes and Their Uses
Python provides several file modes that we can use when opening a file. Each mode has a specific purpose and determines how the file can be accessed.
– “r”: Read mode. Allows reading from the file. Raises an error if the file doesn’t exist.
– “w”: Write mode. Allows writing to the file. Creates a new file if it doesn’t exist, and overwrites the existing content.
– “a”: Append mode. Allows appending to the file. Creates a new file if it doesn’t exist.
– “b”: Binary mode. Allows reading or writing binary data.
– “t”: Text mode. Allows reading or writing text data. This is the default mode if no mode is specified.
Here’s an example:
file = open("example.txt", "r") # Perform read operations file.close() file = open("example.txt", "w") # Perform write operations file.close() file = open("example.txt", "a") # Perform append operations file.close()
In this example, we demonstrate the usage of different file modes when opening a file.
Using the With Statement in File Handling
Python provides a convenient way to handle files using the with
statement. When working with files, it is recommended to use the with
statement as it automatically takes care of opening and closing the file, even if an exception occurs.
Here’s an example:
with open("example.txt", "r") as file: contents = file.read() print(contents)
In this example, we use the with
statement to open the file “example.txt” in read mode. The file is automatically closed when the with
block is exited, ensuring that system resources are properly released.
Related Article: How To Check If List Is Empty In Python
File Object Attributes
When a file is opened in Python, a file object is created. This file object has several attributes that provide information about the file.
– name
: Returns the name of the file.
– mode
: Returns the mode in which the file was opened.
– closed
: Returns a boolean value indicating whether the file is closed or not.
Here’s an example:
file = open("example.txt", "r") print(file.name) print(file.mode) print(file.closed) file.close()
In this example, we open a file and access its attributes using the file object.
Use Case: Logging System Events
One common use case for file operations is logging system events. We can use file operations to write log messages to a file, which can later be analyzed for debugging or auditing purposes.
Here’s an example of logging system events to a file:
def log_event(event): with open("log.txt", "a") as file: file.write(event + "\n") log_event("Application started") log_event("User logged in")
In this example, we define a log_event()
function that takes an event message and writes it to a file named “log.txt” in append mode. We call this function to log different system events.
Use Case: Automated Data Backup
Another use case for file operations is automated data backup. We can use file operations to copy files from one location to another, creating backups of important data.
Here’s an example of automating data backup using file operations:
import shutil shutil.copy("data.txt", "backup/data.txt")
In this example, we use the shutil.copy()
function to copy the file “data.txt” to a backup directory named “backup”.
Related Article: How To Check If a File Exists In Python
Best Practice: Safe File Handling
When working with files, it is important to follow best practices to ensure safe file handling. Here are some best practices to consider:
– Always close files after use to free up system resources.
– Use the with
statement to automatically handle file opening and closing.
– Validate user input before using it to access or manipulate files.
– Handle errors and exceptions gracefully to prevent crashes and data corruption.
Best Practice: Efficient File I/O
Efficient file I/O can significantly improve the performance of file operations. Here are some best practices for efficient file I/O:
– Minimize the number of file operations by reading or writing in larger chunks.
– Use buffered I/O for improved performance. Python’s file objects are buffered by default.
– Avoid unnecessary file operations by caching or storing frequently accessed data in memory.
– Use appropriate file modes and methods for the specific task to avoid unnecessary overhead.
Real World Example: Configuration File Parser
A common real-world example of file operations is parsing configuration files. Configuration files contain settings and options that can be read and modified using file operations.
Here’s an example of parsing a configuration file:
config = {} with open("config.ini", "r") as file: for line in file: if "=" in line: key, value = line.strip().split("=") config[key] = value print(config)
In this example, we read a configuration file named “config.ini” and parse it line by line. Each line containing a key-value pair is added to a dictionary.
Related Article: How to Use Inline If Statements for Print in Python
Real World Example: CSV Data Processor
Another real-world example of file operations is processing CSV (Comma-Separated Values) files. CSV files store tabular data, with each line representing a row and each value separated by commas.
Here’s an example of processing a CSV file:
import csv with open("data.csv", "r") as file: reader = csv.reader(file) for row in reader: print(row)
In this example, we use the csv
module to read a CSV file named “data.csv”. We iterate over each row and print it.
Performance Consideration: Large File Handling
When working with large files, it is important to consider performance implications. Reading or writing large files can consume a significant amount of memory and may impact the performance of your application.
To handle large files efficiently, consider the following techniques:
– Use buffered I/O for improved performance. Python’s file objects are buffered by default.
– Read or write data in smaller chunks instead of loading the entire file into memory.
– Process data incrementally instead of storing it all in memory.
– Use generators or iterators to process large files in a memory-efficient manner.
Performance Consideration: File I/O and Memory Usage
File I/O operations can consume a significant amount of memory, especially when dealing with large files. It is important to optimize memory usage when performing file operations to avoid memory-related issues.
To optimize memory usage during file operations, consider the following techniques:
– Use buffered I/O for improved performance. Python’s file objects are buffered by default.
– Read or write data in smaller chunks instead of loading the entire file into memory.
– Use memory-efficient data structures or techniques, such as generators or iterators, to process file data incrementally.
– Close files after use to free up system resources and release memory.
Related Article: How to Use Stripchar on a String in Python
Advanced Technique: Binary File Operations
In addition to text files, Python also supports binary file operations. Binary files contain non-textual data, such as images, audio, video, or binary data formats.
To perform binary file operations, use the appropriate file mode (“rb” for reading, “wb” for writing, “ab” for appending) and read or write binary data using the read()
and write()
methods.
Here’s an example of binary file operations:
with open("image.jpg", "rb") as file: data = file.read() with open("copy.jpg", "wb") as file: file.write(data)
In this example, we read a binary file named “image.jpg” and write its contents to another file named “copy.jpg”.
Advanced Technique: File Serialization
File serialization is the process of converting data objects into a format that can be stored and reconstructed later. Python provides built-in modules, such as pickle
and json
, for serializing and deserializing objects.
Here’s an example of file serialization using the pickle
module:
import pickle data = {"name": "John", "age": 30} with open("data.pickle", "wb") as file: pickle.dump(data, file) with open("data.pickle", "rb") as file: loaded_data = pickle.load(file) print(loaded_data)
In this example, we serialize a Python dictionary object using the pickle
module and write it to a file named “data.pickle”. We then read the file and deserialize the object using the pickle.load()
function.
Code Snippet: Read File Line by Line
with open("example.txt", "r") as file: for line in file: print(line)
This code snippet demonstrates how to read a file line by line using the with
statement. Each line is printed as it is read.
Related Article: How To Delete A File Or Folder In Python
Code Snippet: Write List to File
lines = ["Line 1\n", "Line 2\n", "Line 3\n"] with open("example.txt", "w") as file: file.writelines(lines)
This code snippet shows how to write a list of strings to a file. Each string in the list is written as a separate line.
Code Snippet: Delete File if Exists
import os if os.path.exists("example.txt"): os.remove("example.txt")
This code snippet demonstrates how to delete a file if it exists. It checks if the file exists using the os.path.exists()
function before deleting it using the os.remove()
function.
Code Snippet: Copy File to Another Directory
import shutil shutil.copy("source.txt", "destination/directory/")
This code snippet shows how to copy a file to another directory using the shutil.copy()
function. The source file is specified as the first argument, and the destination directory is specified as the second argument.
Related Article: How To Move A File In Python
Code Snippet: Recursive Directory Traversal
import os def traverse_directory(path): for root, dirs, files in os.walk(path): for file in files: print(os.path.join(root, file)) traverse_directory("my_directory")
This code snippet demonstrates how to recursively traverse a directory and print the paths of all files within the directory and its subdirectories. It uses the os.walk()
function to iterate over the directory tree.