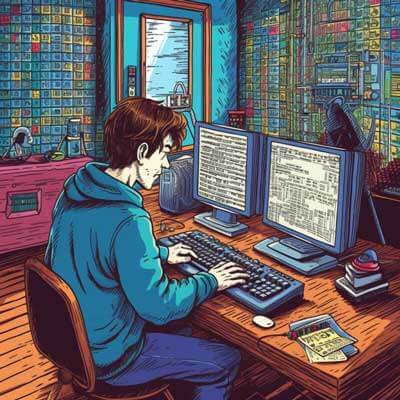
Table of Contents
To write a Pandas DataFrame to a CSV file in Python, you can use the to_csv()
function provided by the Pandas library. This function allows you to specify the file path and name, as well as various optional parameters to control the output format.
Step 1: Install Pandas
Before we can use the to_csv()
function, we need to make sure that the Pandas library is installed. If you haven't already installed it, you can do so by running the following command:
pip install pandas
Related Article: FastAPI Enterprise Basics: SSO, RBAC, and Auditing
Step 2: Import the Pandas Library
Once Pandas is installed, you need to import it into your Python script or interactive session. You can do this using the import
statement:
import pandas as pd
This statement imports the Pandas library and assigns it the alias pd
, which is the most commonly used alias for Pandas.
Step 3: Create a DataFrame
Before we can write a DataFrame to a CSV file, we need to have a DataFrame to work with. You can create a DataFrame in various ways, such as reading data from a file, querying a database, or manually constructing it.
For example, let's say we have the following data representing students and their grades:
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'], 'Math': [90, 85, 92, 78], 'Science': [88, 80, 95, 82]} df = pd.DataFrame(data)
This code creates a DataFrame with three columns: "Name", "Math", and "Science", and four rows representing four students and their grades.
Step 4: Write the DataFrame to a CSV File
To write the DataFrame to a CSV file, we can use the to_csv()
function. This function takes the file path and name as the first argument and has various optional parameters to control the output format.
For example, to write the DataFrame to a file named "students.csv" in the current directory, you can use the following code:
df.to_csv('students.csv', index=False)
This code writes the DataFrame to a CSV file named "students.csv" and sets the index
parameter to False
to exclude the row index from the output.
If you want to include the row index in the output, you can omit the index
parameter or set it to True
:
df.to_csv('students.csv') # or df.to_csv('students.csv', index=True)
By default, the to_csv()
function uses a comma (,
) as the field delimiter and a quote character ("
) to enclose fields that contain special characters. If you want to use a different delimiter or disable quoting altogether, you can use the sep
and quotechar
parameters, respectively:
df.to_csv('students.csv', sep=';', quotechar="'")
This code writes the DataFrame to a CSV file using a semicolon (;
) as the field delimiter and a single quote ('
) as the quote character.
Related Article: How to Drop All Duplicate Rows in Python Pandas
Step 5: Specify Additional Parameters
The to_csv()
function provides many more optional parameters that allow you to customize the output format. Here are a few examples:
- header
: Specifies whether to include the column names as the first line in the output. By default, this parameter is set to True
. You can set it to False
to exclude the header line.
- columns
: Specifies which columns to include in the output. By default, all columns are included. You can pass a list of column names to include only specific columns.
- na_rep
: Specifies the string representation of missing values. By default, missing values are represented as an empty string. You can set this parameter to a custom value, such as "NA"
.
- date_format
: Specifies the format string for date columns. By default, date columns are formatted as ISO 8601 strings. You can use a custom format string to specify a different date format.
For a complete list of parameters and their descriptions, you can refer to the [Pandas documentation on to_csv()
](https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.to_csv.html).
Why is this question asked?
The question "How to write a Pandas DataFrame to a CSV file?" is a common one because CSV (Comma-Separated Values) is a widely used file format for storing tabular data. Many data analysis and data processing tasks involve reading data from various sources, manipulating it using Pandas DataFrames, and then saving the results to CSV files for further analysis or sharing with others.
Being able to write Pandas DataFrames to CSV files is a fundamental skill for any data scientist, data engineer, or anyone working with data in Python. It allows for easy integration with other tools and systems that can consume CSV files, such as spreadsheet applications, databases, and data processing pipelines.
Alternative Ideas and Suggestions
While writing Pandas DataFrames to CSV files is a straightforward and commonly used approach, there are alternative ideas and suggestions depending on the specific use case and requirements:
1. Using Other File Formats: In addition to CSV, Pandas supports writing DataFrames to various other file formats, such as Excel, SQL databases, JSON, and more. Depending on your needs, you may consider using a different file format that better suits your data structure or the tools you are working with.
2. Compression: If the resulting CSV file is large or storage space is a concern, you can consider compressing the output using compression libraries or formats, such as gzip, zip, or parquet. This can significantly reduce the file size and improve storage efficiency.
3. Appending Data: If you need to write multiple DataFrames to the same CSV file or append new data to an existing file, you can use the mode
parameter of the to_csv()
function. By setting mode='a'
, you can append the DataFrame to an existing file instead of overwriting it.
4. Specifying Data Types: When writing DataFrames to CSV files, Pandas infers the data types of the columns based on the actual data. However, in some cases, you may want to explicitly specify the data types for better control and compatibility. You can use the dtype
parameter of the to_csv()
function to specify the desired data types for the columns.
Best Practices
When writing Pandas DataFrames to CSV files, it is good practice to keep the following points in mind:
1. Consider File Encoding: By default, the to_csv()
function uses the UTF-8 encoding for the output file. However, if your data contains non-ASCII characters or you need to work with a different encoding, you can specify the encoding
parameter to ensure proper encoding and decoding of the data.
2. Handle Missing Values: By default, missing values in Pandas DataFrames are represented as empty strings in the output CSV file. If you prefer a different representation, you can use the na_rep
parameter to specify a custom value, such as "NA"
, "NULL"
, or "NaN"
.
3. Validate Output: After writing the DataFrame to a CSV file, it is a good practice to verify the output file to ensure that it matches your expectations. Open the file in a text editor or a spreadsheet application and check the column names, data values, and any custom formatting or options you have specified.
4. Keep File Size in Mind: If your DataFrame is large or contains a significant amount of data, writing it to a CSV file may result in a large output file. Make sure you have enough disk space available and consider compression or alternative file formats if storage efficiency is a concern.
5. Document Your Code: When writing code that includes saving DataFrames to CSV files, it is a good practice to add comments or documentation to explain the purpose of the code, the expected output, and any special considerations or requirements.