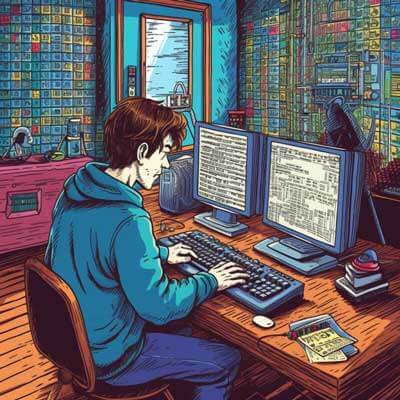
Table of Contents
Iterating over rows in a Pandas DataFrame is a common operation when working with tabular data in Python. There are several ways to accomplish this task, depending on the specific requirements of your code. In this answer, we will explore different approaches to iterate over rows in a Pandas DataFrame and discuss their advantages and disadvantages.
Why is this question asked?
The question of how to iterate over rows in a Pandas DataFrame is often asked by Python programmers who are new to the library or who are working on a specific task that requires row-wise iteration. There can be several reasons why someone might need to iterate over rows in a DataFrame:
1. Data processing: Sometimes, you may need to perform row-wise calculations or transformations on the data in a DataFrame. For example, you might need to calculate the sum of values in each row, apply a function to each row, or filter rows based on certain conditions.
2. Data validation: In some cases, you may need to validate the data in each row against certain criteria. This could involve checking for missing values, detecting outliers, or ensuring that the data conforms to a specific format.
Related Article: How to Use Matplotlib for Chinese Text in Python
Possible Answers
Answer 1: Using the iterrows() method
One way to iterate over rows in a Pandas DataFrame is to use the iterrows()
method. This method returns an iterator that yields pairs of index and row data as Pandas Series objects. Here's an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Emma', 'Michael'], 'Age': [28, 32, 45], 'City': ['New York', 'San Francisco', 'Chicago']} df = pd.DataFrame(data) # Iterate over rows using iterrows() for index, row in df.iterrows(): print(f"Index: {index}") print(f"Name: {row['Name']}, Age: {row['Age']}, City: {row['City']}") print()
Output:
Index: 0 Name: John, Age: 28, City: New York Index: 1 Name: Emma, Age: 32, City: San Francisco Index: 2 Name: Michael, Age: 45, City: Chicago
In this example, we create a DataFrame with three columns: Name, Age, and City. We then use the iterrows()
method to iterate over the rows of the DataFrame. For each row, we print the index and the values of the Name, Age, and City columns.
While the iterrows()
method is straightforward to use, it should be used with caution when working with large DataFrames. This is because the method creates a new Pandas Series object for each row, which can be memory-intensive and slow for large datasets. If performance is a concern, it is recommended to consider alternative approaches.
Answer 2: Using the itertuples() method
Another approach to iterate over rows in a Pandas DataFrame is to use the itertuples()
method. This method returns an iterator that yields namedtuples, which are similar to regular tuples but with named fields. Here's an example:
import pandas as pd # Create a sample DataFrame data = {'Name': ['John', 'Emma', 'Michael'], 'Age': [28, 32, 45], 'City': ['New York', 'San Francisco', 'Chicago']} df = pd.DataFrame(data) # Iterate over rows using itertuples() for row in df.itertuples(index=False): print(f"Name: {row.Name}, Age: {row.Age}, City: {row.City}") print()
Output:
Name: John, Age: 28, City: New York Name: Emma, Age: 32, City: San Francisco Name: Michael, Age: 45, City: Chicago
In this example, we create a DataFrame with three columns: Name, Age, and City. We then use the itertuples()
method to iterate over the rows of the DataFrame. For each row, we access the values of the Name, Age, and City fields using dot notation.
Compared to the iterrows()
method, the itertuples()
method is generally faster and more memory-efficient, especially for large DataFrames. However, it returns namedtuples instead of Pandas Series objects, which may require some adjustments in your code if you are using specific Series methods.
Related Article: How to Use Double Precision Floating Values in Python
Suggestions and Alternative Ideas
When working with Pandas DataFrames, it is often recommended to avoid explicit row-wise iteration whenever possible. This is because Pandas provides powerful vectorized operations that can efficiently process data in bulk, without the need for explicit loops. Here are some suggestions and alternative ideas to consider:
1. Vectorized operations: Instead of iterating over rows, consider using Pandas' built-in vectorized operations to perform calculations or transformations on entire columns or subsets of data. For example, you can use the apply()
or map()
methods to apply a function to each element or column of a DataFrame, respectively.
2. Conditional filtering: If you need to filter rows based on certain conditions, consider using boolean indexing or the query()
method instead of explicit iteration. Boolean indexing allows you to create a boolean mask that selects rows based on a condition, while the query()
method allows you to filter rows using a SQL-like syntax.
3. Grouping and aggregation: If you need to perform calculations on groups of rows, consider using the groupby()
method to group the data by one or more columns and then apply aggregate functions to each group. This can be much faster than iterating over rows individually.
4. Pandas DataFrame methods: Pandas provides a rich set of methods for common data manipulation tasks. Before resorting to explicit iteration, check the Pandas documentation and explore the available methods to see if there is a built-in solution that fits your needs.
Best Practices
When iterating over rows in a Pandas DataFrame, keep the following best practices in mind:
1. Avoid modifying the DataFrame during iteration: Modifying a DataFrame while iterating over it can lead to unexpected behavior and errors. If you need to modify the data, consider creating a new DataFrame or using methods that allow safe modification, such as apply()
.
2. Use vectorized operations whenever possible: As mentioned earlier, vectorized operations are generally more efficient than explicit iteration. Whenever you can express your data manipulation task as a vectorized operation, it is recommended to do so.
3. Consider the performance implications: Explicit iteration can be slow and memory-intensive for large DataFrames. If performance is a concern, consider using alternative approaches, such as vectorized operations or Pandas methods.
4. Be mindful of data types and missing values: When iterating over rows, be aware of the data types of your columns and handle missing values appropriately. Pandas provides methods like dropna()
and fillna()
to handle missing values before or during iteration.
Overall, while iterating over rows in a Pandas DataFrame can be necessary in certain situations, it is generally recommended to explore alternative approaches that leverage the power of vectorized operations and built-in Pandas methods.