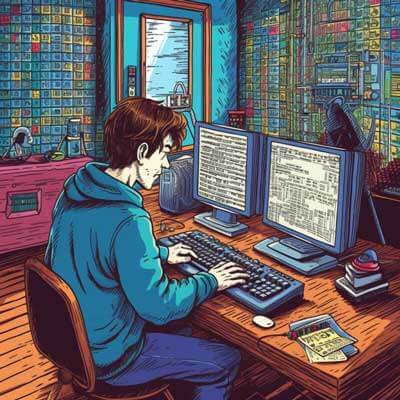
Table of Contents
Introduction to Js Date Fns
Js Date Fns is a comprehensive JavaScript library that provides a wide range of functions for working with dates and times. It offers a simple and intuitive API, making it easy to perform common date-related operations such as date arithmetic, formatting, manipulation, and more. Whether you need to calculate the difference between dates, compare dates, determine if a date is in the past or future, or handle time zones and locale-specific formatting, Js Date Fns has got you covered.
Related Article: How To Remove Duplicates From A Javascript Array
Installation of Date Fns
To start using Js Date Fns in your JavaScript project, you first need to install it. There are several ways to install the library, depending on your project setup and preferences.
1. Using npm:
npm install date-fns
2. Using yarn:
yarn add date-fns
3. Using a CDN:
Once Js Date Fns is installed, you can import the required functions into your project and start using them.
Example: Formatting a Date String
import { format } from 'date-fns'; const date = new Date(); const formattedDate = format(date, 'dd/MM/yyyy'); console.log(formattedDate); // Output: 25/12/2022
Example: Calculating the Difference Between Two Dates
import { differenceInDays } from 'date-fns'; const startDate = new Date(2022, 0, 1); const endDate = new Date(2022, 11, 31); const daysDifference = differenceInDays(endDate, startDate); console.log(daysDifference); // Output: 364
Related Article: How to Implement Functional Programming in JavaScript: A Practical Guide
Date Arithmetic with Date Fns
Js Date Fns provides a set of functions for performing date arithmetic operations such as adding or subtracting days, months, years, hours, minutes, and seconds from a given date.
Example: Adding and Subtracting Dates
import { addDays, subMonths } from 'date-fns'; const currentDate = new Date(); const futureDate = addDays(currentDate, 7); const pastDate = subMonths(currentDate, 3); console.log(futureDate); console.log(pastDate);
Example: Calculating Age
import { differenceInYears } from 'date-fns'; const birthDate = new Date(1990, 0, 1); const currentDate = new Date(); const age = differenceInYears(currentDate, birthDate); console.log(age);
Formatting Dates with Date Fns
Formatting dates is a common task when working with dates in JavaScript. Js Date Fns provides various functions to format dates according to different patterns and locale-specific formats.
Related Article: How to Copy to the Clipboard in Javascript
Example: Formatting Date Strings
import { format } from 'date-fns'; const date = new Date(); const formattedDate = format(date, 'dd/MM/yyyy'); console.log(formattedDate); const formattedDateTime = format(date, 'dd/MM/yyyy HH:mm:ss'); console.log(formattedDateTime); const formattedMonthYear = format(date, 'MMMM yyyy'); console.log(formattedMonthYear);
Example: Getting the Current Day of the Week
import { format, getDay } from 'date-fns'; const date = new Date(); const formattedDate = format(date, 'dd/MM/yyyy'); const dayOfWeek = getDay(date); console.log(`Today is ${formattedDate}, and it's a ${dayOfWeek}`);
Manipulating Dates and Times
Js Date Fns provides a rich set of functions for manipulating dates and times. Whether you need to set or adjust specific parts of a date, find the start or end of a period, or determine if a date falls within a range, Js Date Fns has you covered.
Example: Finding the Start and End of a Day
import { startOfDay, endOfDay } from 'date-fns'; const date = new Date(); const startOfDayDate = startOfDay(date); const endOfDayDate = endOfDay(date); console.log(startOfDayDate); console.log(endOfDayDate);
Related Article: How to Check if a String Matches a Regex in JS
Example: Checking if a Date Is Between Two Dates
import { isWithinInterval } from 'date-fns'; const startDate = new Date(2022, 0, 1); const endDate = new Date(2022, 11, 31); const currentDate = new Date(); const isWithinRange = isWithinInterval(currentDate, { start: startDate, end: endDate }); console.log(isWithinRange);
Time Zones and Locale Support
Js Date Fns provides comprehensive support for handling time zones and locale-specific formatting. You can easily convert dates between different time zones, retrieve the current user's local time zone, and format dates according to the user's locale.
Example: Converting Between Time Zones
import { utcToZonedTime, format } from 'date-fns-tz'; const date = new Date(); const timeZone = 'America/New_York'; const zonedDate = utcToZonedTime(date, timeZone); const formattedDate = format(zonedDate, 'dd/MM/yyyy HH:mm:ss', { timeZone }); console.log(formattedDate);
Example: Getting the User's Local Time Zone
import { getZonedTimeZone, format } from 'date-fns-tz'; const date = new Date(); const localTimeZone = getZonedTimeZone(); const formattedDate = format(date, 'dd/MM/yyyy HH:mm:ss', { timeZone: localTimeZone }); console.log(formattedDate);
Related Article: How to Access Cookies Using Javascript
Difference Between Dates Using Date Fns
Calculating the difference between two dates is a common task in many applications. Js Date Fns provides various functions for calculating the difference between dates in terms of years, months, days, hours, minutes, seconds, and more.
Example: Calculating the Difference in Days
import { differenceInDays } from 'date-fns'; const startDate = new Date(2022, 0, 1); const endDate = new Date(2022, 11, 31); const daysDifference = differenceInDays(endDate, startDate); console.log(daysDifference);
Example: Calculating the Difference in Years
import { differenceInYears } from 'date-fns'; const birthDate = new Date(1990, 0, 1); const currentDate = new Date(); const age = differenceInYears(currentDate, birthDate); console.log(age);
Comparing Dates with Date Fns
Comparing dates is a common operation when working with dates. Js Date Fns provides functions to compare two dates and determine if one is before, after, or equal to another.
Related Article: How to Work with Big Data using JavaScript
Example: Comparing Two Dates
import { isBefore, isAfter, isEqual } from 'date-fns'; const date1 = new Date(2022, 0, 1); const date2 = new Date(2022, 6, 1); console.log(isBefore(date1, date2)); // true console.log(isAfter(date1, date2)); // false console.log(isEqual(date1, date2)); // false
Example: Checking If a Date Is Today
import { isToday } from 'date-fns'; const date = new Date(); console.log(isToday(date)); // true or false
Determining if a Date Is in the Past or Future
Determining if a date is in the past or future is a common task in many applications. Js Date Fns provides functions to check if a given date is in the past or future.
Example: Checking If a Date Is in the Past
import { isPast } from 'date-fns'; const date = new Date(2022, 0, 1); console.log(isPast(date)); // true or false
Related Article: How to Shuffle a JavaScript Array
Example: Checking If a Date Is in the Future
import { isFuture } from 'date-fns'; const date = new Date(2022, 0, 1); console.log(isFuture(date)); // true or false
Working with Unix Timestamps in Date Fns
Unix timestamps represent a point in time as the number of seconds or milliseconds since January 1, 1970. Js Date Fns provides functions to convert Unix timestamps to JavaScript dates and vice versa.
Example: Converting Unix Timestamp to JavaScript Date
import { fromUnixTime } from 'date-fns'; const timestamp = 1640438400; // Unix timestamp in seconds const date = fromUnixTime(timestamp); console.log(date);
Example: Converting JavaScript Date to Unix Timestamp
import { getUnixTime } from 'date-fns'; const date = new Date(); const timestamp = getUnixTime(date); console.log(timestamp);
Related Article: How to Convert a Unix Timestamp to Time in Javascript
Use Case: Scheduling and Planning with Date Fns
Js Date Fns is a powerful tool for scheduling and planning applications. Whether you need to calculate the next occurrence of a recurring event, determine available time slots for appointments, or create calendars, Js Date Fns can simplify your development process.
Example: Calculating the Next Occurrence of a Weekly Meeting
import { addWeeks } from 'date-fns'; const lastMeetingDate = new Date(2022, 0, 1); const recurrenceInterval = 1; // Weekly recurrence const nextMeetingDate = addWeeks(lastMeetingDate, recurrenceInterval); console.log(nextMeetingDate);
Example: Finding Available Time Slots for Appointments
import { eachHourOfInterval, subMinutes } from 'date-fns'; const startDate = new Date(); const endDate = addDays(startDate, 7); const bookedAppointments = [ { start: new Date(2022, 0, 1, 9, 0), end: new Date(2022, 0, 1, 10, 0) }, { start: new Date(2022, 0, 2, 13, 0), end: new Date(2022, 0, 2, 14, 0) }, // ... other booked appointments ]; const availableSlots = eachHourOfInterval({ start: startDate, end: endDate }) .filter((slot) => !bookedAppointments.some((appointment) => slot >= appointment.start && slot ({ start: slot, end: subMinutes(slot, -59) })); console.log(availableSlots);
Use Case: Creating Calendars with Date Fns
Js Date Fns provides functions to work with calendars and dates. You can easily create calendar views, navigate between months, highlight specific dates, and more using Js Date Fns.
Related Article: Integrating JavaScript Functions in React Components
Example: Generating a Calendar for a Specific Month
import { eachDayOfInterval, startOfMonth, endOfMonth } from 'date-fns'; const currentDate = new Date(); const firstDayOfMonth = startOfMonth(currentDate); const lastDayOfMonth = endOfMonth(currentDate); const calendarDays = eachDayOfInterval({ start: firstDayOfMonth, end: lastDayOfMonth }); console.log(calendarDays);
Example: Highlighting Specific Dates in a Calendar
import { eachDayOfInterval, startOfMonth, endOfMonth, isToday, isWeekend } from 'date-fns'; const currentDate = new Date(); const firstDayOfMonth = startOfMonth(currentDate); const lastDayOfMonth = endOfMonth(currentDate); const calendarDays = eachDayOfInterval({ start: firstDayOfMonth, end: lastDayOfMonth }) .map((day) => ({ date: day, isToday: isToday(day), isWeekend: isWeekend(day), })); console.log(calendarDays);
Best Practices: Managing Date Inputs and Outputs
When working with dates in JavaScript, it's important to follow some best practices to ensure accurate and reliable date inputs and outputs. Here are a few tips to consider:
1. Always validate user input: When accepting date input from users, validate the input to ensure it matches the expected format and falls within valid ranges.
2. Use consistent date formats: Use a consistent date format throughout your application to avoid confusion and prevent errors. Consider using ISO 8601 format (YYYY-MM-DD) for date strings.
3. Handle time zones correctly: When working with dates and times in different time zones, make sure to handle time zone conversions properly. Use Js Date Fns functions to convert between time zones and display dates in the user's local time zone.
4. Be aware of daylight saving time changes: Daylight saving time changes can affect the accuracy of date calculations. Take into account the potential shift in time when performing calculations or displaying dates.
Example: Parsing Dates from User Input
import { parse } from 'date-fns'; const userInput = '2022-12-31'; const parsedDate = parse(userInput, 'yyyy-MM-dd', new Date()); console.log(parsedDate);
Related Article: How to Set Checked for a Checkbox with jQuery
Example: Checking Date Validity
import { isValid } from 'date-fns'; const date = new Date(2022, 1, 30); console.log(isValid(date)); // true or false
Best Practices: Avoiding Common Date Mistakes
When working with dates, it's important to be aware of common pitfalls and mistakes that can lead to incorrect results or unexpected behavior. Here are some best practices to avoid common date mistakes:
1. Avoid using the deprecated Date constructor: Instead of using the Date constructor with year, month, and day arguments, consider using functions like new Date(year, monthIndex, day)
or new Date(year, monthIndex, day, hour, minute, second)
to create dates.
2. Be cautious with date manipulation: When manipulating dates, be aware that some operations can mutate the original date object. Consider using Js Date Fns functions that return a new date object instead of modifying the original one.
3. Handle time components appropriately: When working with dates that include time components, make sure to handle time correctly. Be aware of time zone differences, daylight saving time changes, and potential rounding errors.
4. Use appropriate date comparison methods: When comparing dates, use the appropriate comparison functions provided by Js Date Fns, such as isBefore
, isAfter
, or isEqual
, instead of direct equality or inequality comparisons.
Example: Checking for Invalid Dates
import { isValid } from 'date-fns'; const date = new Date(2022, 1, 30); console.log(isValid(date)); // true or false
Example: Avoiding Mutations with Date Manipulation
import { addDays } from 'date-fns'; const currentDate = new Date(); const futureDate = addDays(currentDate, 7); console.log(currentDate); // Original date remains unchanged console.log(futureDate); // New date object with 7 days added
Related Article: The Most Common JavaScript Errors and How to Fix Them
Real World Example: Building a Countdown Timer
A countdown timer is a common feature in many applications. Js Date Fns can be used to build a countdown timer by calculating the remaining time between the current date and a target date.
Example: Building a Countdown Timer
import { differenceInSeconds, format } from 'date-fns'; const targetDate = new Date(2022, 11, 31); function updateCountdown() { const currentDate = new Date(); const remainingTime = differenceInSeconds(targetDate, currentDate); if (remainingTime < 0) { console.log('Countdown has ended'); } else { const hours = Math.floor(remainingTime / 3600); const minutes = Math.floor((remainingTime % 3600) / 60); const seconds = remainingTime % 60; const formattedTime = format(new Date(0, 0, 0, hours, minutes, seconds), 'HH:mm:ss'); console.log(`Countdown: ${formattedTime}`); } } setInterval(updateCountdown, 1000);
Real World Example: Handling Recurring Events
Handling recurring events is a common requirement in many applications. Js Date Fns provides functions to calculate the next occurrence of a recurring event based on a given schedule.
Example: Calculating the Next Occurrence of a Weekly Meeting
import { addWeeks } from 'date-fns'; const lastMeetingDate = new Date(2022, 0, 1); const recurrenceInterval = 1; // Weekly recurrence const nextMeetingDate = addWeeks(lastMeetingDate, recurrenceInterval); console.log(nextMeetingDate);
Related Article: How to Use Window Load and Document Ready Functions
Performance Consideration: Minimizing Date Calculations
Performing date calculations can be computationally expensive, especially when dealing with large date ranges or frequent calculations. To optimize performance, consider minimizing unnecessary date calculations and caching results when possible.
Example: Caching Date Conversions
import { format } from 'date-fns'; const date = new Date(); const formattedDate = format(date, 'dd/MM/yyyy'); console.log(formattedDate); // Cache the formatted date for future use const cachedFormattedDate = formattedDate;
Advanced Technique: Extending Date Fns with Custom Functions
Js Date Fns provides a rich set of functions, but you can also extend it by creating your own custom functions to meet specific requirements. By extending Js Date Fns, you can add new functionality or enhance existing functions to suit your needs.
Example: Creating a Custom Date Function
import { format } from 'date-fns'; function formatToCustomPattern(date, pattern) { // Custom implementation return format(date, pattern); } const date = new Date(); const formattedDate = formatToCustomPattern(date, 'yyyy/MM/dd'); console.log(formattedDate);
Related Article: JavaScript Spread and Rest Operators Explained
Advanced Technique: Combining Date Fns with Promises
Combining Js Date Fns with Promises allows you to perform asynchronous date-related operations, such as fetching remote data based on a date or waiting for a specific date or time to execute a task.
Example: Using Promises with Date Fns
import { addDays } from 'date-fns'; function fetchDataForDate(date) { return new Promise((resolve) => { // Simulating an asynchronous data fetch setTimeout(() => { resolve(`Data for ${date.toISOString()} fetched successfully`); }, 2000); }); } const currentDate = new Date(); const futureDate = addDays(currentDate, 7); fetchDataForDate(futureDate).then((data) => { console.log(data); });
Code Snippet: Formatting Date Strings
import { format } from 'date-fns'; const date = new Date(); const formattedDate = format(date, 'dd/MM/yyyy'); console.log(formattedDate); const formattedDateTime = format(date, 'dd/MM/yyyy HH:mm:ss'); console.log(formattedDateTime); const formattedMonthYear = format(date, 'MMMM yyyy'); console.log(formattedMonthYear);
Code Snippet: Adding and Subtracting Dates
import { addDays, subMonths } from 'date-fns'; const currentDate = new Date(); const futureDate = addDays(currentDate, 7); const pastDate = subMonths(currentDate, 3); console.log(futureDate); console.log(pastDate);
Related Article: How to Work with Async Calls in JavaScript
Code Snippet: Checking Date Validity
import { isValid } from 'date-fns'; const date = new Date(2022, 1, 30); console.log(isValid(date)); // true or false
Code Snippet: Converting Between Time Zones
import { utcToZonedTime, format } from 'date-fns-tz'; const date = new Date(); const timeZone = 'America/New_York'; const zonedDate = utcToZonedTime(date, timeZone); const formattedDate = format(zonedDate, 'dd/MM/yyyy HH:mm:ss', { timeZone }); console.log(formattedDate);
Code Snippet: Parsing Dates from User Input
import { parse } from 'date-fns'; const userInput = '2022-12-31'; const parsedDate = parse(userInput, 'yyyy-MM-dd', new Date()); console.log(parsedDate);
Error Handling: Dealing with Invalid Dates
When working with dates, it's important to handle invalid dates to prevent errors or unexpected behavior. Js Date Fns provides functions to check the validity of a date and handle invalid dates gracefully.
Related Article: Using the JavaScript Fetch API for Data Retrieval
Example: Checking for Invalid Dates
import { isValid } from 'date-fns'; const date = new Date(2022, 1, 30); console.log(isValid(date)); // true or false
Error Handling: Managing Time Zone Issues
Handling time zone issues is crucial when working with dates in different time zones. Js Date Fns provides functions to convert dates between time zones, retrieve the user's local time zone, and handle time zone-related errors.
Example: Getting the User's Local Time Zone
import { getZonedTimeZone, format } from 'date-fns-tz'; const date = new Date(); const localTimeZone = getZonedTimeZone(); const formattedDate = format(date, 'dd/MM/yyyy HH:mm:ss', { timeZone: localTimeZone }); console.log(formattedDate);