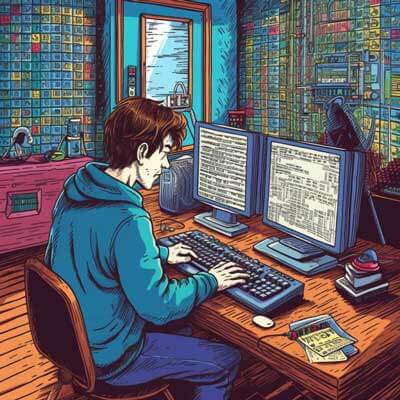
Shuffling an array is a common task in JavaScript, and there are several ways to achieve it. In this answer, we will explore two popular methods for shuffling a JavaScript array.
Method 1: Using the Fisher-Yates Algorithm
The Fisher-Yates algorithm is a simple and efficient method for shuffling an array. It works by iterating over the array from the last element to the first and swapping each element with a randomly selected element before it. This process is repeated for each element in the array, resulting in a shuffled array.
Here’s an example implementation of the Fisher-Yates algorithm:
function shuffleArray(array) { for (let i = array.length - 1; i > 0; i--) { const j = Math.floor(Math.random() * (i + 1)); [array[i], array[j]] = [array[j], array[i]]; } return array; } const myArray = [1, 2, 3, 4, 5]; const shuffledArray = shuffleArray(myArray); console.log(shuffledArray);
In this example, we define a shuffleArray
function that takes an array as an argument. The function uses a for
loop to iterate over the array from the last element to the first. Inside the loop, we generate a random index j
using Math.random()
and swap the current element with the element at index j
using destructuring assignment.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Method 2: Using the Array.sort() method
Another method for shuffling an array in JavaScript is to use the Array.sort()
method with a custom sorting function that randomly orders the elements. This approach works by assigning a random sort value to each element and letting the sort()
method rearrange the elements based on these values.
Here’s an example implementation using the Array.sort()
method:
function shuffleArray(array) { return array.sort(() => Math.random() - 0.5); } const myArray = [1, 2, 3, 4, 5]; const shuffledArray = shuffleArray(myArray); console.log(shuffledArray);
In this example, we define a shuffleArray
function that takes an array as an argument. The function uses the sort()
method with a custom sorting function that returns a random value between -0.5 and 0.5. This random value effectively shuffles the array by changing the order of its elements.
Best Practices
When shuffling an array in JavaScript, it’s important to keep a few best practices in mind:
1. Use a reliable shuffling algorithm: The Fisher-Yates algorithm is a commonly used and efficient method for shuffling arrays. It ensures a uniform distribution of shuffled elements and is easy to implement.
2. Avoid modifying the original array: When shuffling an array, it’s generally a good practice to create a new shuffled array rather than modifying the original array. This helps maintain data integrity and allows you to reuse the original array if needed.
3. Test your implementation: Before using a shuffle function in production code, it’s important to thoroughly test its correctness and performance. Test the function with different input sizes and edge cases to ensure its reliability.
Alternative Ideas
While the Fisher-Yates algorithm and the Array.sort()
method are the most common approaches for shuffling an array in JavaScript, there are other alternative ideas worth exploring. Here are a few:
1. Using a library: JavaScript libraries like Lodash provide utility functions for shuffling arrays. These libraries often have optimized implementations and additional features that can be useful in other parts of your codebase.
2. Using recursion: You can implement a recursive shuffling function that randomly selects an element from the array and recursively shuffles the remaining elements. This approach can be interesting to explore for educational purposes or when dealing with smaller arrays.
3. Using a random number generator: Instead of relying on the built-in Math.random()
function, you can use a more advanced random number generator library like random-js
or seedrandom
. These libraries offer better control over the randomness of the shuffled array.
Related Article: How to Use the forEach Loop with JavaScript