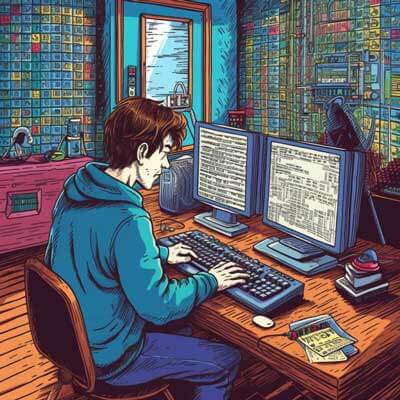
- How to Call a JavaScript Function from a React Component
- Can a React Component Invoke a JavaScript Function?
- How Do I Execute a JavaScript Function from a React Component?
- What Is the Syntax to Call a JavaScript Function in a React Component?
- How to Use a JavaScript Function as an Event Handler in a React Component
- Can I Pass a JavaScript Function as a Prop to a React Component?
- What Are the Steps to Call a JavaScript Function from a React Component?
- How Does a React Component Interact with JavaScript Functions?
- Is It Possible to Call a JavaScript Function Using Props in a React Component?
- Can a React Component Access and Modify the State of a JavaScript Function?
How to Call a JavaScript Function from a React Component
In React, it is possible to call a JavaScript function from a React component. This can be useful when you want to perform certain actions or calculations that are not directly related to the component’s rendering or state management.
To call a JavaScript function from a React component, you can simply invoke the function by using its name followed by parentheses. Here’s an example:
import React from 'react'; class MyComponent extends React.Component { handleClick() { console.log('Button clicked!'); // Call your JavaScript function here myFunction(); } render() { return ( <button> Click me </button> ); } } function myFunction() { console.log('JavaScript function called!'); } export default MyComponent;
In this example, we have a React component called MyComponent
. Inside the component, there is a method called handleClick
which is triggered when the button is clicked. Inside this method, we call the JavaScript function myFunction
by simply writing its name followed by parentheses.
When the button is clicked, the console will log both “Button clicked!” and “JavaScript function called!”.
Related Article: Accessing Parent State from Child Components in Next.js
Can a React Component Invoke a JavaScript Function?
Yes, a React component can invoke a JavaScript function. React components are built using JavaScript, so they have access to all the features and capabilities of the language.
When a React component invokes a JavaScript function, it can pass parameters to the function and receive a return value if the function returns one. This allows the component to perform complex calculations, interact with external APIs, or manipulate data in any way required.
Here’s an example of a React component invoking a JavaScript function with parameters:
import React from 'react'; class MyComponent extends React.Component { handleClick() { const result = calculateSum(5, 10); console.log('Sum:', result); } render() { return ( <button> Click me </button> ); } } function calculateSum(a, b) { return a + b; } export default MyComponent;
In this example, the React component MyComponent
has a method called handleClick
that invokes the JavaScript function calculateSum
with the parameters 5 and 10. The function calculateSum
returns the sum of the two numbers, which is then logged to the console.
When the button is clicked, the console will log “Sum: 15”.
How Do I Execute a JavaScript Function from a React Component?
To execute a JavaScript function from a React component, you can simply call the function using its name followed by parentheses. This can be done within any method of the component, such as render
, componentDidMount
, or custom methods defined by the developer.
Here’s an example of executing a JavaScript function from a React component’s componentDidMount
method:
import React from 'react'; class MyComponent extends React.Component { componentDidMount() { initializeApp(); } render() { return ( <div> {/* Component content */} </div> ); } } function initializeApp() { console.log('App initialized!'); } export default MyComponent;
In this example, the JavaScript function initializeApp
is executed when the component is mounted using the componentDidMount
lifecycle method. The function can perform any initialization tasks required by the application.
When the component is mounted, the console will log “App initialized!”.
What Is the Syntax to Call a JavaScript Function in a React Component?
The syntax to call a JavaScript function in a React component is straightforward. You can simply write the function name followed by parentheses. Optionally, you can pass arguments to the function within the parentheses.
Here’s an example of the syntax to call a JavaScript function in a React component:
import React from 'react'; class MyComponent extends React.Component { handleClick() { myFunction(); // Calling a function with no arguments myOtherFunction('Hello, world!'); // Calling a function with an argument } render() { return ( <button> Click me </button> ); } } function myFunction() { console.log('Function called!'); } function myOtherFunction(message) { console.log('Message:', message); } export default MyComponent;
In this example, the React component MyComponent
has a method called handleClick
which calls two JavaScript functions: myFunction
with no arguments, and myOtherFunction
with the argument 'Hello, world!'
.
When the button is clicked, the console will log “Function called!” and “Message: Hello, world!”.
Related Article: How to Apply Ngstyle Conditions in Angular
How to Use a JavaScript Function as an Event Handler in a React Component
In React, you can use a JavaScript function as an event handler in a React component by assigning the function to the corresponding event attribute of the element. This allows you to trigger the function when a specific event occurs, such as a button click or a form submission.
Here’s an example of using a JavaScript function as an event handler in a React component:
import React from 'react'; class MyComponent extends React.Component { handleClick() { console.log('Button clicked!'); } render() { return ( <button> Click me </button> ); } } export default MyComponent;
In this example, the JavaScript function handleClick
is used as an event handler for the onClick
event of the button
element. When the button is clicked, the function is triggered and the message “Button clicked!” is logged to the console.
Can I Pass a JavaScript Function as a Prop to a React Component?
Yes, you can pass a JavaScript function as a prop to a React component. This allows you to pass behavior or functionality from a parent component to its child components, enabling communication and interaction between them.
To pass a JavaScript function as a prop, you simply include it as a value of a prop when rendering the child component. The child component can then access and invoke the function as needed.
Here’s an example of passing a JavaScript function as a prop to a React component:
import React from 'react'; class ParentComponent extends React.Component { handleClick() { console.log('Button clicked!'); } render() { return ( ); } } class ChildComponent extends React.Component { render() { return ( <button> Click me </button> ); } } export default ParentComponent;
In this example, the JavaScript function handleClick
is defined in the parent component ParentComponent
. The function is passed as a prop called onClick
to the child component ChildComponent
. Inside the child component, the function is invoked when the button is clicked using this.props.onClick
.
When the button is clicked, the console will log “Button clicked!”.
What Are the Steps to Call a JavaScript Function from a React Component?
To call a JavaScript function from a React component, you can follow these steps:
1. Define the JavaScript function that you want to call.
2. Import the necessary JavaScript files or libraries that contain the function.
3. Inside the React component, invoke the JavaScript function using its name followed by parentheses.
4. Optionally, pass arguments to the function within the parentheses.
5. Run the React component to trigger the function.
Here’s an example of the steps to call a JavaScript function from a React component:
import React from 'react'; import { myFunction } from './utils'; // Assuming myFunction is defined in utils.js class MyComponent extends React.Component { handleClick() { myFunction(); // Calling the function } render() { return ( <button> Click me </button> ); } } export default MyComponent;
In this example, the JavaScript function myFunction
is defined in a separate file called utils.js
. The function is imported into the React component MyComponent
and invoked when the button is clicked.
Related Article: How to Integrate Redux with Next.js
How Does a React Component Interact with JavaScript Functions?
A React component can interact with JavaScript functions by invoking them, passing arguments to them, and receiving return values from them. This allows the component to perform actions or calculations that are not directly related to rendering or state management.
When a React component interacts with a JavaScript function, it can trigger the function at specific times or in response to user events. The component can also pass data to the function and use the return value to update its state or perform other operations.
Here’s an example of a React component interacting with a JavaScript function:
import React from 'react'; class MyComponent extends React.Component { handleClick() { const result = calculateSum(5, 10); console.log('Sum:', result); } render() { return ( <button> Click me </button> ); } } function calculateSum(a, b) { return a + b; } export default MyComponent;
In this example, the React component MyComponent
interacts with the JavaScript function calculateSum
. The component invokes the function with the arguments 5 and 10, and logs the return value to the console.
When the button is clicked, the console will log “Sum: 15”.
Is It Possible to Call a JavaScript Function Using Props in a React Component?
Yes, it is possible to call a JavaScript function using props in a React component. By passing a JavaScript function as a prop from a parent component to a child component, the child component can call the function and execute it as needed.
To call a JavaScript function using props, you need to pass the function as a prop from the parent component and access it in the child component using this.props
. Once you have access to the function, you can call it and pass any necessary arguments.
Here’s an example of calling a JavaScript function using props in a React component:
import React from 'react'; class ParentComponent extends React.Component { handleClick() { console.log('Button clicked!'); } render() { return ( ); } } class ChildComponent extends React.Component { handleClick() { this.props.onClick(); // Calling the function passed as a prop } render() { return ( <button> Click me </button> ); } } export default ParentComponent;
In this example, the parent component ParentComponent
has a method called handleClick
which is passed as a prop called onClick
to the child component ChildComponent
. Inside the child component, the function is called using this.props.onClick
.
When the button is clicked, the console will log “Button clicked!”.
Can a React Component Access and Modify the State of a JavaScript Function?
No, a React component cannot directly access or modify the state of a JavaScript function. The state in a React component refers to the internal data of the component that can be updated using the setState
method.
A JavaScript function, on the other hand, is a separate entity that does not have an associated state. Functions can be invoked and perform actions based on their input parameters, but they do not have their own state that can be modified.
If you need to access or modify the state in a React component from a JavaScript function, you can pass the necessary state values as arguments to the function and return any updated values as needed.
Here’s an example of passing state values to a JavaScript function and returning updated values:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } handleClick() { const updatedCount = incrementCount(this.state.count); this.setState({ count: updatedCount }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button> Increment </button> </div> ); } } function incrementCount(count) { return count + 1; } export default MyComponent;
In this example, the React component MyComponent
has a state property called count
which is initially set to 0. When the button is clicked, the JavaScript function incrementCount
is called with the current count value as an argument. The function returns the updated count value, which is then set as the new state using this.setState
.
Each time the button is clicked, the count value will be incremented by 1 and displayed on the screen.