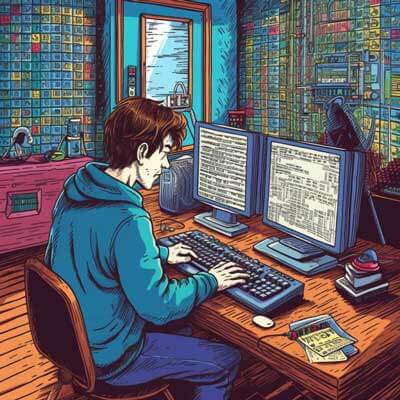
- Introduction to Fetch API
- Setting up the Fetch API
- Fetching Data from a Server
- Case Study: Fetching JSON Data
- Breaking Down Fetch API Responses
- Case Study: Posting Data to a Server
- Handling Fetch API Errors
- Code Snippet: Error Handling
- Best Practices: Using Promises with Fetch API
- Code Snippet: Fetching with Async/Await
- Advanced Fetch API Techniques: Headers and Modes
- Code Snippet: Fetching with Headers
- Performance Considerations: Network Latency
- Performance Considerations: Large Data Fetching
- Code Snippet: Basic GET Request
- Code Snippet: Basic POST Request
- Case Study: Real-World Fetch API Application
- Troubleshooting Common Fetch API Issues
Introduction to Fetch API
The Fetch API is a modern JavaScript API that provides a way to make HTTP requests and handle responses. It is a replacement for the older XMLHttpRequest object and offers a more powerful and flexible feature set. With the Fetch API, you can easily retrieve data from a server and handle it in your JavaScript code.
Related Article: How To Check If a Key Exists In a Javascript Object
Setting up the Fetch API
To use the Fetch API, you don’t need to install any additional libraries or frameworks. It is built into modern browsers and can be used directly in your JavaScript code. Simply create a new instance of the fetch
function and pass in the URL of the resource you want to fetch.
Here’s an example of setting up the Fetch API to make a basic GET request:
fetch('https://api.example.com/data') .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { console.log(data); }) .catch(error => { console.error(error); });
In this example, we use the fetch
function to make a GET request to the URL https://api.example.com/data
. We then chain a series of Promise-based functions to handle the response. If the response is successful (with a status code of 200-299), we call the json
method on the response object to parse the response body as JSON. If there is an error, we throw an error with the corresponding status code. Finally, we log the parsed data or the error to the console.
By default, the Fetch API uses the GET method for requests. If you need to use other HTTP methods like POST, PUT, or DELETE, you can pass an optional second argument to the fetch
function with the desired options, such as the method and body.
Fetching Data from a Server
Fetching data from a server is one of the most common use cases of the Fetch API. Whether you’re retrieving JSON data, HTML content, or any other type of resource, the Fetch API provides a simple and consistent way to fetch data and handle the response.
Case Study: Fetching JSON Data
Let’s say you want to fetch JSON data from a server and display it on a web page. Here’s an example:
fetch('https://api.example.com/users') .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { // Process the JSON data for (const user of data) { console.log(user.name); } }) .catch(error => { console.error(error); });
In this example, we make a GET request to the URL https://api.example.com/users
to fetch a list of users in JSON format. If the response is successful, we parse the response body as JSON and loop through the data to log each user’s name to the console. If there is an error, we log the error to the console.
The response.json()
method returns a Promise that resolves to the parsed JSON data. This allows you to easily work with the fetched data in your JavaScript code.
Related Article: How To Use the Javascript Void(0) Operator
Breaking Down Fetch API Responses
When working with the Fetch API, it’s important to understand how to handle different types of responses. The API provides several methods to access the response data and extract the information you need.
Case Study: Posting Data to a Server
In addition to fetching data from a server, the Fetch API also allows you to send data to a server using different HTTP methods like POST, PUT, or DELETE. Let’s take a look at an example of how to post data to a server using the Fetch API:
const data = { name: 'John Doe', email: 'john@example.com' }; fetch('https://api.example.com/users', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(data) }) .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { console.log(data); }) .catch(error => { console.error(error); });
In this example, we create an object data
with the user’s name and email. We then make a POST request to the URL https://api.example.com/users
and pass in the data object as the request body. We also set the Content-Type
header to application/json
to indicate that we’re sending JSON data.
If the response is successful, we parse the response body as JSON and log the data to the console. If there is an error, we log the error to the console.
Handling Fetch API Errors
Error handling is an important aspect of working with the Fetch API. When making requests, there are several types of errors you may encounter, such as network errors, server errors, or client-side errors. The Fetch API provides a consistent way to handle these errors and take appropriate action.
Related Article: How to Check If a Value is an Object in JavaScript
Code Snippet: Error Handling
fetch('https://api.example.com/data') .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { console.log(data); }) .catch(error => { console.error(error); });
In this example, we handle errors by checking if the response is not successful (with a status code outside the range of 200-299). If there is an error, we throw a new Error object with the corresponding status code. This will trigger the catch
block and allow us to handle the error appropriately.
By logging the error to the console, we can easily see what went wrong and take appropriate action, such as displaying an error message to the user or retrying the request.
Best Practices: Using Promises with Fetch API
The Fetch API uses Promises to handle asynchronous operations, making it easier to write and manage asynchronous code. Promises provide a clean and straightforward way to handle asynchronous operations and avoid callback hell.
Code Snippet: Fetching with Async/Await
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); if (response.ok) { const data = await response.json(); console.log(data); } else { throw new Error('Error: ' + response.status); } } catch (error) { console.error(error); } } fetchData();
In this example, we use the async/await
syntax to make the code more readable and easier to understand. The await
keyword allows us to pause the execution of the function until the Promise is resolved or rejected. This eliminates the need for chaining .then
and .catch
methods.
By wrapping the code in a try/catch
block, we can handle any errors that occur during the fetching process. If there is an error, it will be caught in the catch
block and we can log the error to the console.
Related Article: How To Capitalize the First Letter In Javascript
Advanced Fetch API Techniques: Headers and Modes
The Fetch API provides advanced techniques for customizing the request headers and modes. Headers allow you to send additional information with your request, such as authentication tokens or custom headers. Modes allow you to control the behavior of the request, such as enabling or disabling CORS.
Code Snippet: Fetching with Headers
const headers = new Headers(); headers.append('Authorization', 'Bearer token'); fetch('https://api.example.com/data', { headers: headers }) .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { console.log(data); }) .catch(error => { console.error(error); });
In this example, we create a new Headers
object and append a custom Authorization
header with a bearer token. We then pass the headers
object as an option when making the fetch request.
This allows us to include the authentication token in the request headers and send it to the server for authentication.
Performance Considerations: Network Latency
When using the Fetch API, it’s important to consider network latency and optimize your code to minimize the impact of network delays. Network latency refers to the time it takes for a request to reach the server and for the response to return to the client.
Related Article: How To Get A Timestamp In Javascript
Performance Considerations: Large Data Fetching
Fetching large amounts of data can impact performance, especially if the data is not efficiently transferred or processed. To improve performance when fetching large data sets, you can use techniques like pagination or lazy loading.
Code Snippet: Basic GET Request
fetch('https://api.example.com/data') .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { console.log(data); }) .catch(error => { console.error(error); });
Code Snippet: Basic POST Request
const data = { name: 'John Doe', email: 'john@example.com' }; fetch('https://api.example.com/users', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(data) }) .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { console.log(data); }) .catch(error => { console.error(error); });
Related Article: How To Validate An Email Address In Javascript
Case Study: Real-World Fetch API Application
Let’s take a look at a real-world example of using the Fetch API in a web application. In this example, we’ll build a simple weather app that fetches weather data from a weather API and displays it on a web page.
const apiKey = 'YOUR_API_KEY'; const city = 'London'; fetch(`https://api.example.com/weather?city=${city}&apiKey=${apiKey}`) .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error: ' + response.status); } }) .then(data => { // Display weather data on the web page const temperature = data.main.temp; const description = data.weather[0].description; document.getElementById('temperature').textContent = temperature; document.getElementById('description').textContent = description; }) .catch(error => { console.error(error); });
In this example, we fetch weather data from a weather API using the city name and an API key. We then parse the response body as JSON and extract the temperature and weather description. Finally, we update the temperature and description elements on the web page with the fetched data.
Troubleshooting Common Fetch API Issues
When working with the Fetch API, you may encounter some common issues. Here are a few troubleshooting tips to help you resolve these issues:
– Ensure that the URL you’re fetching from is correct and accessible.
– Check the response status code to determine if the request was successful or if there are any errors.
– Verify that the response data is in the expected format and structure.
– Check for any network errors or CORS-related issues.
– Review the documentation of the API you’re working with to ensure you’re using the correct endpoints and parameters.
By following these troubleshooting tips, you can identify and resolve common issues when using the Fetch API.