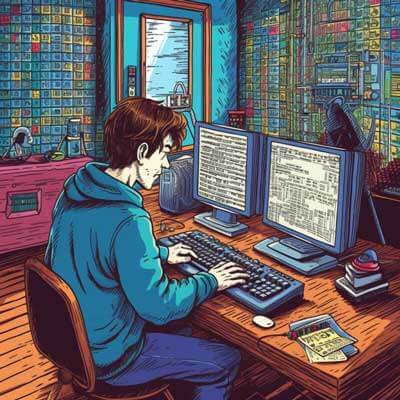
Table of Contents
Introduction
Cookies are small pieces of data that websites store on a user's device. They are commonly used for various purposes, such as remembering login information, tracking user preferences, and providing personalized experiences. In JavaScript, you can access and manipulate cookies to retrieve or modify their values.
Related Article: How to Encode a String to Base64 in Javascript
Accessing Cookies with JavaScript
To access cookies using JavaScript, you can use the document.cookie
property. It returns a string containing all the cookies associated with the current document.
Here's an example that demonstrates how to access a specific cookie:
const cookieValue = document.cookie .split('; ') .find(row => row.startsWith('cookieName=')) ?.split('=')[1]; console.log(cookieValue);
In this example, we split the document.cookie
string into an array of individual cookie strings. Then, we use the find()
method to locate the desired cookie by checking if it starts with the specified name (cookieName=
in this case). Finally, we extract the value of the cookie using split('=')[1]
.
Setting Cookies with JavaScript
To set a cookie using JavaScript, you can assign a new value to the document.cookie
property.
Here's an example that demonstrates how to set a cookie:
document.cookie = 'cookieName=cookieValue; expires=Thu, 01 Jan 2023 00:00:00 UTC; path=/';
In this example, we assign a string in the format 'name=value'
to document.cookie
. Additionally, we specify an expiration date using the expires
attribute and set it to January 1st, 2023. The path
attribute determines which paths on your website can access the cookie.
Deleting Cookies with JavaScript
To delete a cookie using JavaScript, you can set its expiration date to a time in the past.
Here's an example that demonstrates how to delete a cookie:
document.cookie = 'cookieName=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/;';
In this example, we set the expires
attribute to January 1st, 1970, which effectively removes the cookie from the user's device. Remember to specify the same path used when setting the cookie to ensure you are deleting the correct one.
Related Article: How to Resolve Nodemon App Crash and File Change Wait
Updating Cookies with JavaScript
To update a cookie using JavaScript, you can set a new value and/or adjust its attributes.
Here's an example that demonstrates how to update a cookie:
document.cookie = 'cookieName=newValue; expires=Thu, 01 Jan 2023 00:00:00 UTC; path=/';
In this example, we assign a new value (newValue
) to the cookieName
cookie. We also keep the same expiration date and path as before. By setting a new value for an existing cookie with the same name and path, we effectively update it.
All Cookie Features with JavaScript
JavaScript provides several attributes that can be used when working with cookies. These attributes allow you to customize their behavior and control how they are stored and accessed. Here are some commonly used attributes:
- expires
: Specifies the expiration date of the cookie.
- path
: Determines which paths on your website can access the cookie.
- domain
: Specifies which domain(s) can access the cookie.
- secure
: Restricts sending the cookie over unencrypted connections (HTTPS).
- sameSite
: Prevents cross-site request forgery attacks by restricting when the cookie is sent.
To use these attributes, you can include them as part of the cookie string when setting or updating a cookie.
Code Examples for Cookie Manipulation
Here are two code examples that demonstrate different scenarios of cookie manipulation:
Example 1: Retrieving and displaying a user's preferred theme:
const preferredTheme = document.cookie .split('; ') .find(row => row.startsWith('theme=')) ?.split('=')[1]; if (preferredTheme) { document.body.classList.add(preferredTheme); }
In this example, we retrieve the value of the theme
cookie and add it as a CSS class to the body
element. This allows us to apply the user's preferred theme dynamically.
Example 2: Creating a simple "Remember Me" functionality:
const rememberMeCheckbox = document.getElementById('remember-me'); const loginForm = document.getElementById('login-form'); loginForm.addEventListener('submit', function(event) { if (rememberMeCheckbox.checked) { document.cookie = 'rememberMe=true; expires=Thu, 01 Jan 2023 00:00:00 UTC; path=/'; // Additional logic for handling login with "Remember Me" } else { document.cookie = 'rememberMe=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/;'; // Additional logic for handling regular login without "Remember Me" } });
In this example, we listen for the form submission event and check if the "Remember Me" checkbox is selected. If it is, we set a rememberMe
cookie with an expiration date in the future. Otherwise, we delete the rememberMe
cookie. This allows users to have their login information remembered across sessions.
Best Practices for Working with Cookies in JavaScript
When working with cookies in JavaScript, it's important to follow some best practices to ensure security and efficiency:
1. Sanitize and validate cookie values: Always sanitize and validate any user input before using it as a cookie value to prevent security vulnerabilities.
2. Use secure and sameSite attributes: Set the secure
attribute to restrict sending cookies over unencrypted connections (HTTPS) for sensitive information. Additionally, consider using the sameSite
attribute to prevent cross-site request forgery attacks.
3. Limit cookie size: Keep the size of your cookies as small as possible to minimize network traffic and improve performance.
4. Encrypt sensitive information: If you need to store sensitive information in a cookie, consider encrypting it first to enhance security.
5. Be mindful of privacy regulations: Ensure compliance with privacy regulations, such as GDPR or CCPA, when handling user data through cookies.
Related Article: How to Get Selected Value in Dropdown List Using Javascript
Performance Considerations for Cookie Handling in JavaScript
While working with cookies in JavaScript, keep the following performance considerations in mind:
1. Minimize the number of cookies: Having an excessive number of cookies can increase overhead during requests, impacting performance. Try consolidating multiple related values into a single cookie whenever possible.
2. Use efficient string manipulation techniques: When parsing or modifying the document.cookie
string, use optimized string manipulation techniques (e.g., splitting by specific delimiters) for better performance.
3. Cache frequently accessed cookie values: If you frequently access the same cookie value within your code, consider caching it instead of repeatedly parsing document.cookie
.
4. Leverage browser caching mechanisms: Leverage browser caching mechanisms when setting expiration dates for cookies that do not require immediate changes.
5. Monitor network traffic: Keep an eye on the network traffic generated by cookies, especially if you have large or frequently changing cookies. Excessive network traffic can impact performance.
Use Cases for Using Cookies in JavaScript
Cookies offer various use cases in JavaScript-based web applications. Some common scenarios where cookies are used include:
1. User authentication: Storing authentication tokens or session IDs in cookies to maintain user sessions.
2. Remembering user preferences: Storing user preferences, such as theme selection or language preference, to provide a personalized experience across visits.
3. Tracking user behavior: Using cookies to track and analyze user behavior, such as page views or interactions, for analytics purposes.
4. E-commerce functionality: Managing shopping carts, wishlist items, or recently viewed products using cookies.
5. A/B testing: Storing experiment group assignments in cookies to consistently show the same version of a webpage to a user during an A/B test.
These are just a few examples of how cookies can be utilized within JavaScript applications. The flexibility and versatility of cookies make them valuable for various functionalities and enhancements.
Code Snippet Ideas - Basic Cookie Operations
Here are some code snippet ideas that cover basic cookie operations:
Snippet 1: Checking if a cookie exists:
function hasCookie(cookieName) { return document.cookie.split('; ').some(row => row.startsWith(`${cookieName}=`)); } console.log(hasCookie('myCookie')); // true or false
Snippet 2: Getting all available cookies:
function getAllCookies() { return document.cookie.split('; '); } console.log(getAllCookies());
These snippets provide simple utility functions that allow you to check if a specific cookie exists and retrieve an array of all available cookies.
Code Snippet Ideas - Advanced Cookie Operations
Here are some code snippet ideas that cover advanced cookie operations:
Snippet 1: Setting a cookie with additional attributes:
function setCookie(name, value, daysToExpire, path = '/') { const expirationDate = new Date(); expirationDate.setDate(expirationDate.getDate() + daysToExpire); const cookieString = `${name}=${value}; expires=${expirationDate.toUTCString()}; path=${path}`; document.cookie = cookieString; } setCookie('myCookie', 'myValue', 7, '/myPath');
Snippet 2: Deleting all cookies:
function deleteAllCookies() { const cookies = document.cookie.split('; '); for (const cookie of cookies) { const [cookieName] = cookie.split('='); document.cookie = `${cookieName}=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/`; } } deleteAllCookies();
These snippets demonstrate more advanced operations such as setting a cookie with customizable attributes (e.g., expiration date and path) and deleting all existing cookies.
With these code snippets, you can extend your JavaScript cookie manipulation capabilities to handle more complex scenarios.